顺序表:关系线性化,结点顺序存
线性表的特点:同一性、无穷性、有序性
分为三个文件:
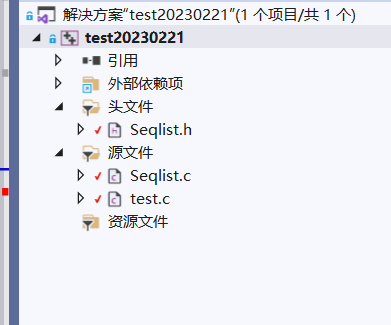
其中头文件为:
#pragma once
#define _CRT_SECURE_NO_WARNINGS 1
#include<stdio.h>
#include<string.h>
#include<errno.h>
#include<stdlib.h>
#include<assert.h>
typedef int SLDateType;
#define INIT_CAPACITY 4
//动态数据表 按需申请
typedef struct Seqlist {
int size;
int capacity;
SLDateType* a;
}SL;
//增删查改
void SLInit(SL*ps);
void SLDestory(SL*ps);fengwei
//尾插
void SLPushBack(SL* ps, SLDateType x);
void SLPopback(SL* ps);
void SLPushFront(SL* ps, SLDateType x);
void SLPopFront(SL* ps);
//打印
void SLPrint(SL* ps);
//检查库存
void SLCheckCapacity(SL* ps);
//某个位置插入
void SLInsert(SL* ps, int pos, SLDateType x);
void SLErase(SL* ps, int pos);
//查找
int SLFind(SL* ps, SLDateType x);
//删除所有的数据
void ClearList(SL* ps);
测试文件:
#include"Seqlist.h"
void TestSeqList1() {
SL s;
SLInit(&s);
SLPushBack(&s,1);
SLPushBack(&s,2);
SLPushBack(&s,3);
SLPushBack(&s,4);
SLPushBack(&s,5);
SLPushBack(&s,6);
SLPushBack(&s,7);
SLPrint(&s);
//SLDestory(&s);
SLPopback(&s);
SLPopback(&s);
SLPrint(&s);
SLPushFront(&s,11);
SLPushFront(&s,22);
SLPushFront(&s,33);
SLPushFront(&s,44);
SLPushFront(&s,55);
SLPrint(&s);
SLPopFront(&s);
SLPopFront(&s);
SLPopFront(&s);
SLPrint(&s);
SLInsert(&s, 2, 2);
SLPrint(&s);
SLErase(&s, 3);
SLPrint(&s);
int ret=SLFind(&s, 5);
if (ret!= -1) {
printf("下标为%d\n", ret);
}
else
{
printf("找不到了\n");
}
SLDestory(&s);
}
void menu()
{
printf("***********************************************\n");
printf("1、尾插数据 2、尾删数据\n");
printf("3、头插数据 4、头删数据\n");
printf("5、打印数据 6、查找数据\n");
printf("7、删除所有数据 -1、退出\n");
printf("***********************************************\n");
}
int main()
{
SL s;
SLInit(&s);
int option = 0;
int val = 0;
int ret = 0;
int a = 0;
do
{
menu();
printf("请输入你的操作:>");
scanf("%d", &option);
switch (option)
{
case 1:
printf("请依次输入你要尾插的数据,以-1结束");
scanf("%d", &val);
while (val != -1)
{
SLPushBack(&s, val);
scanf("%d", &val);
}
SLPrint(&s);
break;
case 2:
SLPopback(&s);
SLPrint(&s);
break;
case 3:
printf("请依次输入你要头插的数据,以-1结束");
scanf("%d", &val);
while (val != -1)
{
SLPushFront(&s, val);
scanf("%d", &val);
}
SLPrint(&s);
break;
case 4:
SLPopFront(&s);//头删
SLPrint(&s);
break;
case 5:
SLPrint(&s);
break;
case 6:
printf("请输入你要查找的数字");
scanf("%d", &a);
ret = SLFind(&s, a);
if (ret != -1) {
printf("下标为%d\n", ret);
}
else
{
printf("找不到了\n");
}
break;
case 7:
ClearList(&s);
break;
default:
break;
}
} while (option != -1);
SLDestory(&s);
return 0;
}
代码核心实现文件: