概念引入
什么是分页:
简单来说:大量数据无法一次性全部显示在网页上?怎么办?只能选取其中的一部分,将大量数据分成好几段,每一段我们用一个网页显示,也就是一页,在页面上我们可以手动控制我们要选择的页面
分页就是将大量数据分成很多页显示的一种处理手段.
分页有什么好处?
1、通过分页,我们不用一次性将所有的数据查出来,只需先查出来一部分,可以减少数据库的IO数据量的传输,降低数据库读写压力,从而提高数据库响应速度(数据库角度)
2、页面也不用一次性显示所有的数据,可以减少浏览器和服务器之间大量数据的IO传输,从而提高服务器的响应速度(前后端角度)
3、我们可能值需要很多信息中少数的几条,那么传输其他多余的数据就是无形之中对于资源的浪费,分页可以减少资源的浪费(用户角度)
数据库如何实现分页查询?(limit)
select * from student limit 0,5
sql语句通过limit关键字实现数据的分页查询, limit后面可以放两个整数作为参数,前一个参数的意义为从那条数据开始查询,后一个参数的意义是连续取出多少条
如果查询 第n 页,每页x条 数据那么sql语句应该写成select * from student limit (n-1)*x, x
注意事项:
第一点 : index ,size start =(index-1)*size;
第二点: maxpage = if(total%size==0){
maxpage=total/size}
else {
maxpage=total/size+1}
分页思路
目标效果: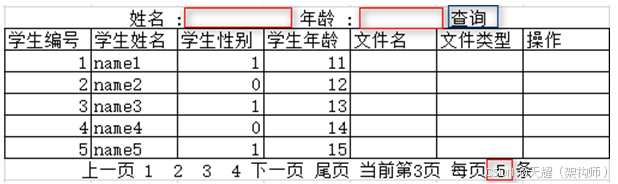
问题一:浏览器向后台发送的信息应该有什么?
1、要查询第几页(index)
2、页大小(size)
3、查询条件
问题二:服务器向浏览器应该返回什么数据?
1、当前第几页
2、信息总条数
3、总页码数
4、页大小
5、当前页的所有信息
分页实现
数据库准备:
页面效果展示:
后端代码:
DAO层
import com.msb.dao.StudentDao;
import com.msb.pojo.Student;
import java.util.List;
public class StudentDaoImpl extends BaseDao implements StudentDao {
@Override
public List<Student> findByPage(String stuname,String stuage,int currentPage, int pageSize) {
StringBuilder sql=new StringBuilder("select * from student where 1=1 ");
if(null != stuname && !"".equals(stuname)) {
sql.append("and stuname like ? ");
}
if(null != stuage && !"".equals(stuage)) {
sql.append("and stuage > ? ");
}
sql.append("limit ?,?");
System.out.println(sql.toString());
List list =null;
if(null != stuname && !"".equals(stuname) && null != stuage && !"".equals(stuage)){
list = baseQuery(Student.class, sql.toString(), "%"+stuname+"%",stuage,(currentPage - 1) * pageSize, pageSize);
}else if ((null != stuname && !"".equals(stuname)) && (null == stuage || "".equals(stuage))){
list = baseQuery(Student.class, sql.toString(), "%"+stuname+"%",(currentPage - 1) * pageSize, pageSize);
}else if ((null == stuname || "".equals(stuname))&& (null != stuage && !"".equals(stuage))){
list = baseQuery(Student.class, sql.toString(), stuage,(currentPage - 1) * pageSize, pageSize);
}else{
list = baseQuery(Student.class, sql.toString(), (currentPage - 1) * pageSize, pageSize);
}
return list;
}
@Override
public int findTotalSize(String stuname,String stuage) {
StringBuilder sql=new StringBuilder("select count(1) from student where 1=1 ");
if(null != stuname && !"".equals(stuname)) {
sql.append("and stuname like ? ");
}
if(null != stuage && !"".equals(stuage)) {
sql.append("and stuage > ? ");
}
int count =0;
if(null != stuname && !"".equals(stuname) && null != stuage && !"".equals(stuage)){
count = baseQueryInt( sql.toString(), "%"+stuname+"%",stuage);
}else if ((null != stuname && !"".equals(stuname)) && (null == stuage || "".equals(stuage))){
count = baseQueryInt( sql.toString(), "%"+stuname+"%");
}else if ((null == stuname || "".equals(stuname))&& (null != stuage && !"".equals(stuage))){
count = baseQueryInt( sql.toString(),stuage);
}else{
count = baseQueryInt(sql.toString());
}
return count;
}
}
sevice层
import com.msb.dao.StudentDao;
import com.msb.dao.impl.StudentDaoImpl;
import com.msb.pojo.PageBean;
import com.msb.pojo.Student;
import com.msb.service.StudentService;
import java.util.List;
public class StudentServiceImpl implements StudentService {
private StudentDao studentDao =new StudentDaoImpl();
// 做分页数据封装的业务处理
@Override
public PageBean<Student> findByPage(String stuname,String stuage,int currentPage, int pageSize) {
// 查询出该页所有数据
List<Student> students = studentDao.findByPage( stuname, stuage,currentPage, pageSize);
// 查询出有多少条数据
int totalSize =studentDao.findTotalSize( stuname, stuage);
// 总页数
int totalPage =totalSize%pageSize==0?totalSize/pageSize:totalSize/pageSize+1;
// 当前页
// 页大小
PageBean<Student> pageBean =new PageBean<>(students, totalSize, pageSize, totalPage, currentPage);
return pageBean;
}
}
controller层
import com.msb.pojo.PageBean;
import com.msb.pojo.Student;
import com.msb.service.StudentService;
import com.msb.service.impl.StudentServiceImpl;
import javax.servlet.ServletException;
import javax.servlet.annotation.WebServlet;
import javax.servlet.http.HttpServlet;
import javax.servlet.http.HttpServletRequest;
import javax.servlet.http.HttpServletResponse;
import java.io.IOException;
@WebServlet(urlPatterns = "/showStudentController.do")
public class ShowStudentController extends HttpServlet {
private StudentService studentService=new StudentServiceImpl();
@Override
protected void service(HttpServletRequest req, HttpServletResponse resp) throws ServletException, IOException {
// 接收数据
// 页码数
int currentPage =1;
try {
currentPage=Integer.parseInt(req.getParameter("currentPage"));
} catch (NumberFormatException e) {
}
// 页大小
int pageSize =5;
try {
pageSize =Integer.parseInt(req.getParameter("pageSize"));
} catch (NumberFormatException e) {
}
// 查询条件
String stuname = req.getParameter("stuname");
String stuage = req.getParameter("stuage");
// 调用service层服务处理业务逻辑
PageBean<Student> pageBean =studentService.findByPage(stuname,stuage,currentPage,pageSize);
// 将数据放入请求域
req.setAttribute("pageBean",pageBean);
req.setAttribute("stuname", stuname);
req.setAttribute("stuage", stuage);
// 响应数据,页面跳转
req.getRequestDispatcher("showStudent.jsp").forward(req,resp);
}
}
前端代码
<%@ page contentType="text/html;charset=UTF-8" language="java" %>
<%@taglib prefix="c" uri="http://java.sun.com/jsp/jstl/core" %>
<html>
<head>
<title>$Title%sSourceCode%lt;/title>
<style>
table{
border: 1px red solid;
margin:0px auto;
width: 80%;
}
td,th{
border:1px solid green;
}
</style>
</head>
<body>
<div style="text-align: center">
名字关键字:<input type="text" id="stuname" value="${stuname}">
年龄下限<input type="text" id="stuage" value="${stuage}">
<input type="button" value="查询" onclick="changePage(1)" >
</div>
<table>
<tr>
<th>学生编号</th>
<th>学生姓名</th>
<th>学生年龄</th>
<th>学生性别</th>
<th>照片</th>
<th>照片类型</th>
<th>操作</th>
</tr>
<%--
pageBean
public class PageBean<T> implements Serializable {
// 当前页数据
private List<T> data;
// 查询出的总记录数
private int totalSize;
// 页大小
private int pageSize;
// 总页数
private int totalPage;
// 当前页数
private int currentPage;
--%>
<c:forEach items="${pageBean.data}" var="student">
<tr>
<td>${student.stuid}</td>
<td>${student.stuname}</td>
<td>${student.stuage}</td>
<td>${student.stugender}</td>
<td>${student.filename}</td>
<td>${student.filetype}</td>
<td>
<a href="#">删除</a>
</td>
</tr>
</c:forEach>
<tr align="center">
<td colspan="7">
<a href="javascript:void(0)" onclick="changePage(${pageBean.currentPage-1})">上一页</a>
<c:forEach begin="1" end="${pageBean.totalPage}" var="num">
<c:choose>
<c:when test="${num eq pageBean.currentPage}">
[${num}]
</c:when>
<c:otherwise>
${num}
</c:otherwise>
</c:choose>
</c:forEach>
<a href="javascript:void(0)" onclick="changePage(${pageBean.currentPage+1})">下一页</a>
尾页
每页
<input id="pageSize" style="width: 40px" type="text" value="${pageBean.pageSize}">
条
当前第
${pageBean.currentPage}
页
共${pageBean.totalPage}页
共
${pageBean.totalSize}
条记录
</td>
</tr>
<script src="js/jquery.min.js"></script>
<script>
function changePage(currentPage){
if(currentPage<1){
alert("已经是第一页了")
return;
}
if(currentPage>${pageBean.totalPage}){
alert("已经是最后一页了")
return;
}
window.location.href="showStudentController.do?stuname="+$("#stuname").val()+"&stuage="+$("#stuage").val()+"¤tPage="+currentPage+"&pageSize="+$("#pageSize").val();
}
</script>
</table>
</body>
</html>