目录
2.2.输出流指数据从程序(内存)到数据源(文件)的路径编辑
3.4 处理流___BufferedReader和BufferedWriter
3.5 处理流___BufferedInputStream和BufferedOutputStream
3.6对象流___ObjectInputStream和ObjectOutputStream
3.8 转换流___InputStreamReader和OutputSreamWriter
3.9 打印流___PrintStream和PrintWriter
一 文件
1.文件的概念: 文件是保存数据的地方
2.文件流:文件在程序中是以流的形式来操作的
2.1.输入流指数据从数据源(文件)到程序(内存)的路径
2.2.输出流指数据从程序(内存)到数据源(文件)的路径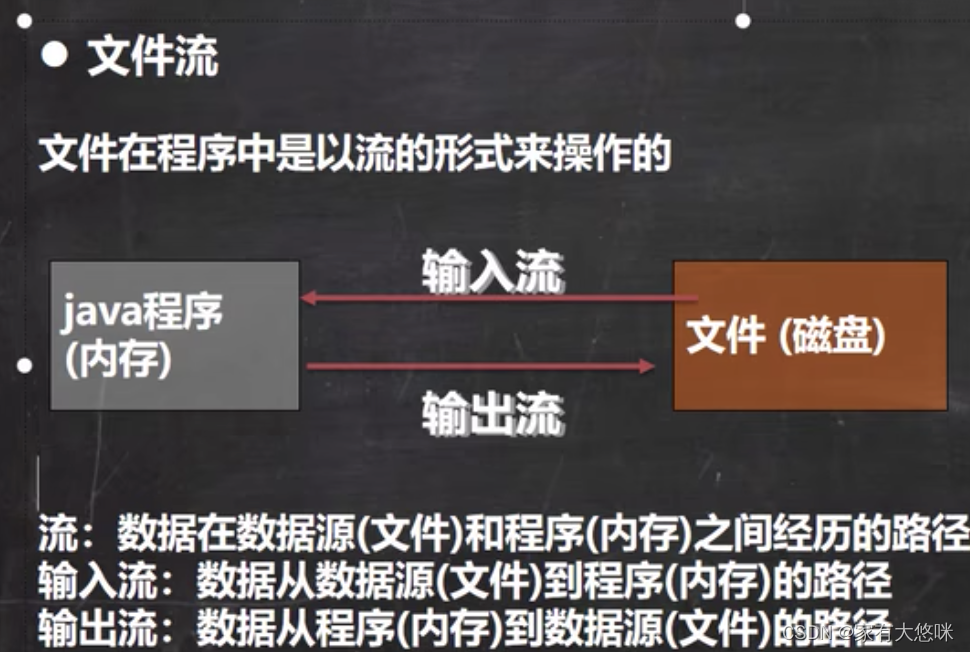
3.常用的文件操作
3.1.创建文件的三种方式
new File(String pathname)//根据路径构建一个File对象
new File(File parent, String child)//根据父目录文件+子路径构建
new File(String parent, String child)//根据父目录+子路径构建
//方式1
public static void create01(){
String filePath = "Desktop/test1.txt";
File file = new File(filePath);
try{
file.createNewFile();
System.out.println("文件创建成功");
}catch(IOException e){
e.printStackTrace();
}
}
//方式2
public static void create02(){
//just an object
File parent = new File("Desktop/");
String fileName = "test2.txt";
File file2 = new File(parent, fileName);
try {
file2.createNewFile(); //save in disk
} catch (IOException e) {
throw new RuntimeException(e);
}
}
//方式3
public static void create03(){
String parentPath = "Desktop/";
String fileName = "test3.txt";
File file = new File(parentPath, fileName);
try {
file.createNewFile();
} catch (IOException e) {
throw new RuntimeException(e);
}
}
3.2. 获取文件的相关信息
File file = new File("Desktop/test4.txt");
System.out.println(file.getName());
System.out.println(file.getAbsolutePath());
System.out.println(file.getParent());
System.out.println(file.length());
System.out.println(file.exists());//whether the file exists
System.out.println(file.isFile());
System.out.println(file.isDirectory());
3.3. 目录的操作和文件的删除
mkdir创建一级目录
mkdirs创建多级目录
delete删除空目录或文件
public static void m1(){
String filePath = "Desktop/demo"; //directory is also a type of file
File file = new File(filePath);
if (file.exists()){
if (file.delete()){
System.out.println(filePath+"delete successfully");
}else{
System.out.println("delete wrongly");
}
}else{
System.out.println("the file doesn't exist");
}
}
public static void m2(){
String directoryPath = "Desktop/demo";
File file = new File(directoryPath);
if (file.exists()){
System.out.println(directoryPath+"exists");
}else{
if (file.mkdirs()){//create multi level directory
//file.mkdir create a level directory
System.out.println(directoryPath+"is created successfully");
}
}
}
二 IO流原理及流的分类
1. Java IO流原理
I:input
O:output
2. 流的分类
3. FileInputStream介绍
3.1结构
3.2常用方法
3.3案例
public static void readFile01() throws IOException {
String filePath = "Desktop/test2.txt";
int read = 0;
FileInputStream fileInputStream = null;
try{
fileInputStream = new FileInputStream(filePath);
while((read = fileInputStream.read()) != -1){
System.out.print((char)read);
}
} catch (IOException e) {
e.printStackTrace();
}finally {
fileInputStream.close();
}
}
//use read(byte[] b) return actual read byte number
public static void readFile02() throws IOException {
String filePath = "Desktop/test2.txt";
byte[] buf = new byte[8]; //字节数组长度可自定义
int readLen = 0;
FileInputStream fileInputStream = null;
try{
fileInputStream = new FileInputStream(filePath);
while((readLen = fileInputStream.read(buf)) != -1){
System.out.print(new String(buf,0, readLen));
}
} catch (IOException e) {
e.printStackTrace();
}finally {
fileInputStream.close();
}
}
4. FileOutputStream介绍
4.1结构
4.2方法
4.3案例
public static void writeData() throws IOException {
String filePath = "/Users/antihu/Desktop/test3.txt";
FileOutputStream fileOutputStream = null;
try {
//1.if override: new FileOutputStream(filePath);
//2.if append: new FileOutputStream(filePath, true);
fileOutputStream = new FileOutputStream(filePath,true);
// fileOutputStream.write('H');//char->int
String str = "hsp, world";
// fileOutputStream.write(str.getBytes());//string->byte[]
fileOutputStream.write(str.getBytes(),0,3);//可以根据索引截取字符
} catch (FileNotFoundException e) {
throw new RuntimeException(e);
}finally {
fileOutputStream.close();
}
}
5.FileReader和FileWrtier(字符流)
5.1 结构
5.2 相关方法及案例
FileReader:
public static void read() throws IOException {
String filePath = "Desktop/test3.txt";
FileReader fileReader = null;
char[] buf = new char[8];
int readLen = 0;
try {
fileReader = new FileReader(filePath);
//2. read char[]
while((readLen = fileReader.read(buf)) != -1){
System.out.print(new String(buf, 0 ,readLen));
};
} catch (IOException e) {
e.printStackTrace();
}finally {
if (fileReader != null){
fileReader.close();
}
}
}
FileWriter:
public static void write01() throws IOException {
FileWriter fileWriter = null;
int data = 0;
String filePath = "Desktop/test3.txt";
char[] chars = {'a','b','c'};
try {
fileWriter = new FileWriter(filePath);
fileWriter.write('H'); //1. write single char
fileWriter.write(chars); //2. write char[]
fileWriter.write("hanshunping".toCharArray(),0,3);//3. write (char[] off, len)
fileWriter.write(" nihao beijing~"); //4. write(string)
fileWriter.write("sdfaf", 0 ,2); //5. wtire(string, off, len)
//huge data: can loop
}catch(IOException e){
e.printStackTrace();
}finally{
try {
fileWriter.close();// =filewriter.flush();
} catch (IOException e) {
e.printStackTrace();
}
}
}
三 节点流和处理流
3.1 概念
3.2 节点流和处理流的区别和联系:
3.3 处理流的功能
1. 提高性能,以增加缓冲的方式提高输入输出效率
2. 操作便捷,处理流提供了一系列便捷的方法来一次输入输出大批量的数据,使用更加灵活方便
3.4 处理流___BufferedReader和BufferedWriter
BufferedReader:
String filePath = "Desktop/test3.txt";
BufferedReader bufferedReader = new BufferedReader(new FileReader(filePath));
String line;//read according to rows
//1. read by rows;return null means read completely
while((line = bufferedReader.readLine()) != null){
System.out.println(line);
}
bufferedReader.close();
BufferedWriter:
String filePath = "Desktop/test4.txt";
BufferedWriter bufferedWriter = new BufferedWriter(new FileWriter(filePath));
bufferedWriter.write("hello bufferedWriter");
//insert a empty line
bufferedWriter.newLine();
bufferedWriter.write("hello");
bufferedWriter.close();
3.5 处理流___BufferedInputStream和BufferedOutputStream
BufferedInputStream:
BufferedOutputStream:
copy案例:
String filePath = "Desktop/ad.jpg";
String targetPath = "Desktop/copy.jpg";
BufferedInputStream bis = null;
BufferedOutputStream bos = null;
try {
bis = new BufferedInputStream(new FileInputStream(filePath));
bos = new BufferedOutputStream(new FileOutputStream(targetPath));
byte[] buf = new byte[1024];
int readLen = 0;
//return -1 means completion
while((readLen = bis.read(buf))!= -1){
bos.write(buf,0,readLen);
}
} catch (IOException e) {
throw new RuntimeException(e);
}finally {
if (bis != null){
bis.close();
}
if (bos != null){
bos.close();
}
}
3.6对象流___ObjectInputStream和ObjectOutputStream
ObjectOutputStream 序列化
String filePath = "Desktop/test3.dat"; //dat is a required type to save data
ObjectOutputStream os = new ObjectOutputStream( new FileOutputStream(filePath));
os.writeInt(100);
os.writeBoolean(true);
os.writeChar('a');
os.writeDouble(9.5);
os.writeUTF("Han");
os.writeObject(new Dog("wang", 10)); //save a dog object
os.close();
System.out.println("success");
public static class Dog implements Serializable {
String name;
int age;
public Dog(String name, int age) {
this.name = name;
this.age = age;
}
public String getName() {
return name;
}
public void setName(String name) {
this.name = name;
}
public int getAge() {
return age;
}
public void setAge(int age) {
this.age = age;
}
@Override
public String toString() {
return "Dog{" +
"name='" + name + '\'' +
", age=" + age +
'}';
}
}
ObjectInputStream 反序列化
String filePath = "Desktop/test3.dat";
ObjectInputStream ois = new ObjectInputStream(new FileInputStream(filePath));
//read order needs to be consistent to the order of serialization
System.out.println(ois.readInt());
System.out.println(ois.readBoolean());
System.out.println(ois.readChar());
System.out.println(ois.readDouble());
System.out.println(ois.readUTF());
//dog
Object dog = ois.readObject();
System.out.println(dog.getClass());
System.out.println(dog);
//向下转型
ObjectOutputStream_.Dog dog2 = (ObjectOutputStream_.Dog)dog;
ois.close();
3.7 标准输入输出流
System.in 标准输入
System.out标准输出
3.8 转换流___InputStreamReader和OutputSreamWriter
转换流可以将字节流转换成字符流,减少乱码,提高效率
InputStreamReader:
//将字节流FileInputStream转成字符流InputStreamReader
String fp = "";
InputStreamReader isr = new InputStreamReader(new FileInputStream(fp), "gbk");
//把inputstreamReader转成bufferedReader
BufferedReader br = new BufferedReader(isr);
//合起来
BufferedReader br2 = new BufferedReader(new InputStreamReader(
new FileInputStream(fp),"gbk"));
//read
String s = br.readLine();
System.out.println(s);
br.close();
OutputStreamReader:
String filePath = "";
OutputStreamWriter outputStreamWriter = new OutputStreamWriter(new FileOutputStream(filePath), "gbk");
outputStreamWriter.write("jdkf");
outputStreamWriter.close();
3.9 打印流___PrintStream和PrintWriter
Note: 打印流只有输出流没有输入流
PrintStream:
PrintStream out = System.out;
//default situation: printStream will output data on standard outputer:screen
out.print("hello");
//also can use write(),input byte ;could use string.getBytes()
out.write("hello".getBytes());
out.close();
//can modify the default output place
System.setOut(new PrintStream("Desktop/test2.txt")); //source code: native method-> modify out
//native means code is not created by java
System.out.println("hello,han");
PrintWriter:
PrintWriter printWriter = new PrintWriter(new FileWriter("Desktop/test2.txt",true));
printWriter.print("hello78722");
printWriter.close();
四 Properties类
Properties类是专门用于读写配置文件的集合类,格式为键=值,默认类型是String,不用引号.
4.1 常用方法
4.2 案例
读取配置文件mysql.properties
1.传统方法读取配置文件:
BufferedReader bufferedReader = new BufferedReader(new FileReader("src/mysql.properties"));
String line = "";
while((line = bufferedReader.readLine()) != null){
String[] split = line.split("=");
//if require get ip value
if ("ip".equals(split[0])){
System.out.println(split[1]);}
}
bufferedReader.close();
2.使用Properties类读取配置文件
//use properties to read mysql.properties file
//1. create properties object
Properties properties = new Properties();
//2. load file
properties.load(new FileReader("src/mysql.properties"));
//3. show kv to controller
properties.list(System.out);
//4.according to key get value
String user = properties.getProperty("user");
String pwd = properties.getProperty("pwd");
System.out.println(user);
System.out.println(pwd);
3.使用Properties类创建配置文件并修改内容
//use properties to create peroperties file and modify content
Properties properties = new Properties();
//CREATE
//setProperty: if not exists,create; if exists, modify value
//properties parent is Hashtable
properties.setProperty("charset","utf8");
properties.setProperty("user","Tom");
properties.setProperty("pwd","abc123456");
//save kv to file
properties.store(new FileOutputStream("src/mysql2.properties"), null);
System.out.println("save successfully");
五 作业(练习题)
5.1题目
5.2答案
Homework01:
String directoryPath = "/Users/antihu/Desktop/go0";
File file = new File(directoryPath);
if (!file.exists()){
if (file.mkdirs()){
System.out.println("create" + directoryPath + "successfully");
}else {
System.out.println("create" + directoryPath + "fail");
}
}
String filePath = directoryPath + "/hell.txt";
file = new File(filePath);
if (!file.exists()){
if (file.createNewFile()){
System.out.println(filePath +"create successfully");
//if create successfully,use bufferedwriter to write
BufferedWriter bufferedWriter = new BufferedWriter(new FileWriter(filePath));
bufferedWriter.write("hello,world~~");
bufferedWriter.close();
}else {
System.out.println(filePath+"create fail");
}
}else {
System.out.println("file exists");
}
Homework02:
BufferedReader dd = new BufferedReader(new FileReader("dd"));
String line;
int lineNum = 0;
try {
while((line = dd.readLine()) != null ){
System.out.println(++lineNum + line);
}
} catch (IOException e) {
throw new RuntimeException(e);
}finally{
if (dd != null){
dd.close();}
}
Homework03:
Properties properties = new Properties();
properties.setProperty("name","tom");
properties.setProperty("age", "5");
properties.setProperty("color","red");
properties.store(new FileWriter("src/dog.properties"),null);
properties.load(new FileReader("src/dog.properties"));
Dog dog = new Dog();
dog.setName(properties.getProperty("name"));
dog.setName(properties.getProperty("age"));
dog.setName(properties.getProperty("color"));
System.out.println(dog);