思路分析:
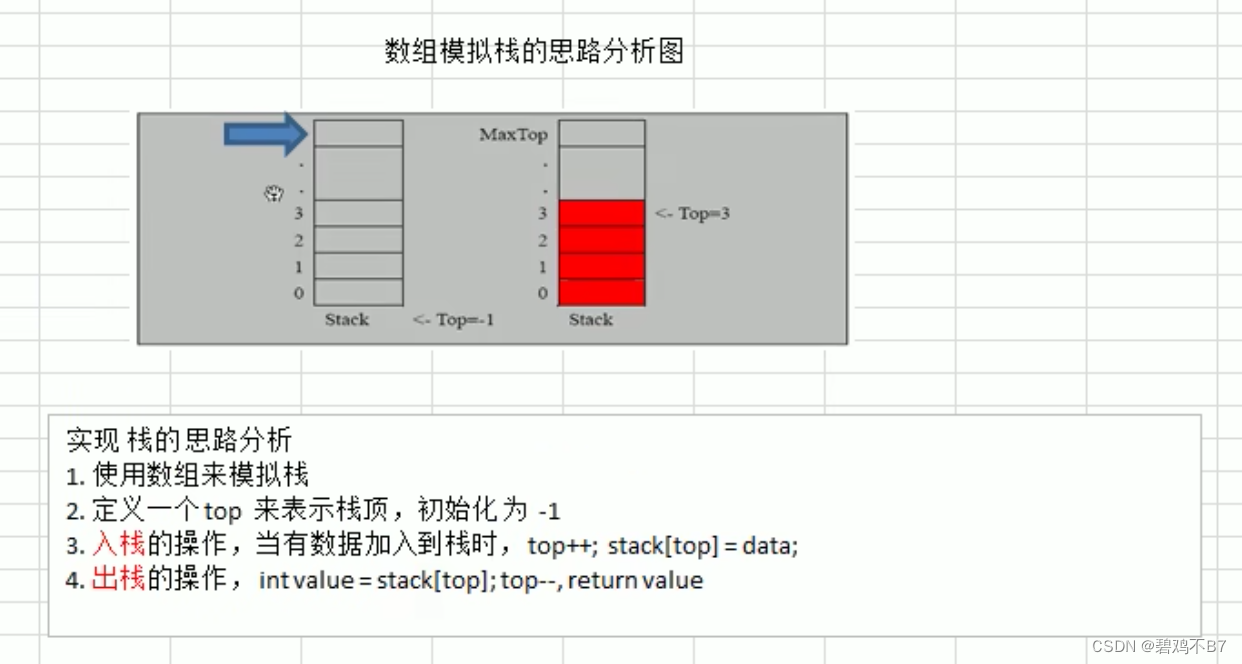
整体代码:
/*
* 项目名称:ANA
* 文件名称:ArrayStackDemo.java
* Date:2023/9/26 上午8:33
* Author:yan_Bingo
*/
package Learning;
import java.util.Scanner;
/**
* @author Yan_Bingo
* @version 1.0
* Create by 2023/9/26 08:33
*/
public class ArrayStackDemo {
public static void main(String[] args) {
ArrayStack stack = new ArrayStack(3);
boolean loop = true;
String input = "";
Scanner scanner = new Scanner(System.in);
while(loop){
System.out.println("show: 表示展示栈的情况");
System.out.println("exit: 表示退出程序");
System.out.println("push: 表示添加新的数据到栈(入栈)");
System.out.println("pop: 表示从栈中取出数据(出栈)");
System.out.print("请输入你的选择: ");
input = scanner.next();
switch (input){
case "show":{
stack.showStack();
break;
}
case "exit":{
scanner.close();
loop = false;
break;
}
case "push":{
System.out.print("请输入你要添加的数据: ");
int value = scanner.nextInt();
stack.push(value);
break;
}
case "pop":{
try {
System.out.println("出栈的数据为: " + stack.pop());
System.out.println();
}catch(Exception e){
System.out.println(e.getMessage());
}
}
default:
break;
}
}
}
}
class ArrayStack{
private int maxSize;
private int top = -1;
private int [] stack;
// 构造器
public ArrayStack(int maxSize){
this.maxSize = maxSize;
stack = new int[this.maxSize];
}
// 判断栈是否为空
public boolean isEmpty(){
return top == -1;
}
// 判断栈是否已满
public boolean isFull(){
return top == maxSize - 1;
}
// 入栈
public void push(int value){
if(isFull()){
System.out.println("当前栈已满,无法加入数据~~");
return;
}
top++;
stack[top] = value;
System.out.println();
}
// 出栈
public int pop(){
if(isEmpty()){
throw new RuntimeException("当前栈为空,没有数据~~");
}
int value = stack[top];
top--;
return value;
}
// 遍历栈
public void showStack(){
if(isEmpty()){
System.out.println("当前栈为空~~");
System.out.println();
return;
}
for (int i = top; i >= 0 ; i--) {
System.out.printf("stack[%d] = %d\n", i, stack[i]);
}
System.out.println();
}
}