Aspose.Imaging for .NET是一个可以让开发人员可以创建、编辑、画图、转换图像的图像处库,提供了一些开发平台原有功能基础之上的一些新特性。它独立于其他应用程序, Aspose.Imaging for .NET允许你在不安装PhotoShop的情况下保存PSD格式。
Aspose.Imaging for .NET非常灵活、稳定和强大。它的很多特性和图像处理例程能够满足绝大多数图像制作的要求。像所有的Aspose组件一样, Aspose.Imaging for .NET能很好地在Win Form and Web Form 下面运行,并且还支持Silverlight。
Aspose API支持流行文件格式处理,并允许将各类文档导出或转换为固定布局文件格式和最常用的图像/多媒体格式。
Aspose.lmaging 最新下载(qun:761297826)https://www.evget.com/resource/detail-download-22338
经常需要通过绘制形状来创建不同的图形对象,包括圆形、直线、矩形等。这些形状也可以用于图像的注释。在本文中,您将学习如何在 C# 中以编程方式绘制不同的形状。我们将演示如何绘制直线、椭圆、圆弧和矩形并生成它们的图像。
- 用于绘制形状的 C# API - 免费下载
- 使用 C# 画一条线
- 使用 C# 绘制椭圆
- 使用 C# 绘制圆弧
- 使用C#画一个矩形
用于绘制形状的 C# API - 免费下载
要绘制不同类型的形状,我们将使用Aspose.Imaging for .NET。这是一个了不起的图像编辑 API,提供了广泛的功能来处理图像和创建绘图。您可以下载API 或从 NuGet 安装它。
PM> Install-Package Aspose.Imaging
使用 C# 画一条线
下面是在C#中画线的步骤。
- 首先,创建BmpOptions类的对象并使用BitsPerPixel属性设置每像素位数。
- 然后,使用Source属性分配StreamSource。
- 创建一个新图像并使用BmpOptions对象和图像的高度和宽度对其进行初始化。
- 创建Graphics类的对象并使用Image对象对其进行初始化。
- 使用Graphics.Clear()方法用一些颜色清除图像表面。
- 使用Graphics.DrawLine(Pen, int, int, int, int)方法画一条线。
- 使用Image.Save()方法生成并保存图像。
下面的代码示例显示了如何在 C# 中绘制一条线。
// Create BmpOptions BmpOptions bmpCreateOptions = new BmpOptions(); bmpCreateOptions.BitsPerPixel = 32; // Define the source property for the instance of BmpOptions bmpCreateOptions.Source = new StreamSource(); // Creates an instance of Image and call create method by passing the // bmpCreateOptions object Image image = Image.Create(bmpCreateOptions, 500, 500); // Create and initialize an instance of Graphics class Graphics graphic = new Graphics(image); // Clear the image surface with White color graphic.Clear(Color.White); // Draw a dotted line by specifying the Pen object having blue color and // co-ordinate Points graphic.DrawLine(new Pen(Color.Blue, 3), 18, 18, 200, 200); graphic.DrawLine(new Pen(Color.Blue, 3), 18, 200, 200, 18); // Draw a continuous line by specifying the Pen object having Solid // Brush with red color and two point structures graphic.DrawLine(new Pen(new SolidBrush(Color.Red), 3), new Point(18, 18), new Point(18, 200)); // Draw a continuous line by specifying the Pen object having Solid // Brush with white color and two point structures graphic.DrawLine(new Pen(new SolidBrush(Color.Orange), 3), new Point(200, 18), new Point(18, 18)); // Save all changes image.Save("draw_lines.bmp");
以下是上述代码示例的输出。
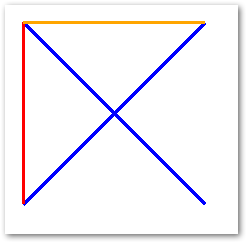
使用 C# 绘制椭圆
下面是在C#中绘制椭圆的步骤。
- 首先,创建BmpOptions类的对象并使用BitsPerPixel属性设置每像素位数。
- 然后,使用Source属性分配StreamSource。
- 创建一个新图像并使用BmpOptions对象和图像的高度和宽度对其进行初始化。
- 创建Graphics类的对象并使用Image对象对其进行初始化。
- 使用Graphics.Clear()方法用一些颜色清除图像表面。
- 使用Graphics.DrawEllipse(Pen, Rectangle)方法绘制一个椭圆。
- 使用Image.Save()方法生成并保存图像。
下面的代码示例显示了如何在 C# 中的图像上绘制椭圆。
// Create BmpOptions BmpOptions bmpCreateOptions = new BmpOptions(); bmpCreateOptions.BitsPerPixel = 32; // Define the source property for the instance of BmpOptions bmpCreateOptions.Source = new StreamSource(); // Creates an instance of Image and call create method by passing the // bmpCreateOptions object Image image = Image.Create(bmpCreateOptions, 500, 500); // Create and initialize an instance of Graphics class Graphics graphic = new Graphics(image); // Clear the image surface with White color graphic.Clear(Color.White); // Draw a dotted ellipse shape by specifying the Pen object having red // color and a surrounding Rectangle graphic.DrawEllipse(new Pen(Color.Red, 3), new Rectangle(60, 40, 70, 120)); // Draw a continuous ellipse shape by specifying the Pen object having // solid brush with blue color and a surrounding Rectangle graphic.DrawEllipse(new Pen(new SolidBrush(Color.Blue), 3), new Rectangle(40, 60, 120, 70)); // Save all changes image.Save("draw_ellipse.bmp");
以下是上述代码示例的输出。
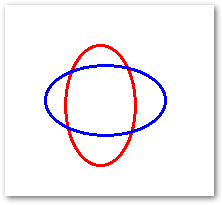
使用 C# 绘制圆弧
下面是在C#中绘制圆弧的步骤。
- 首先,创建BmpOptions类的对象并使用BitsPerPixel属性设置每像素位数。
- 然后,使用Source属性分配StreamSource。
- 创建一个新图像并使用BmpOptions对象和图像的高度和宽度对其进行初始化。
- 创建Graphics类的对象并使用Image对象对其进行初始化。
- 使用Graphics.Clear()方法用一些颜色清除图像表面。
- 使用Graphics.DrawArc(Pen, float x, float y, float width, float height, float startAngle, float sweepAngle)方法绘制圆弧。
- 使用Image.Save()方法生成并保存图像。
下面的代码示例显示了如何在 C# 中的图像上绘制圆弧。
// Create BmpOptions BmpOptions bmpCreateOptions = new BmpOptions(); bmpCreateOptions.BitsPerPixel = 32; // Define the source property for the instance of BmpOptions bmpCreateOptions.Source = new StreamSource(); // Creates an instance of Image and call create method by passing the // bmpCreateOptions object Image image = Image.Create(bmpCreateOptions, 500, 500); // Create and initialize an instance of Graphics class Graphics graphic = new Graphics(image); // Clear the image surface with White color graphic.Clear(Color.White); // Draw a dotted arc shape by specifying the Pen object having red black // color and coordinates, height, width, start & end angles int width = 200; int height = 300; int startAngle = 45; int sweepAngle = 270; // Draw arc to screen graphic.DrawArc(new Pen(Color.Black, 3), 0, 0, width, height, startAngle, sweepAngle); // Save all changes image.Save("draw_arc.bmp");
以下是上述代码示例的输出。
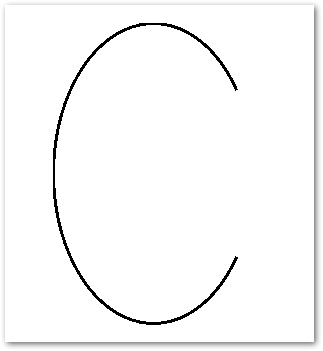
使用 C# 绘制矩形
下面是在C#中绘制矩形的步骤。
- 首先,创建BmpOptions类的对象并使用BitsPerPixel属性设置每像素位数。
- 然后,使用Source属性分配StreamSource。
- 创建一个新图像并使用BmpOptions对象和图像的高度和宽度对其进行初始化。
- 创建Graphics类的对象并使用Image对象对其进行初始化。
- 使用Graphics.Clear()方法用一些颜色清除图像表面。
- 使用Graphics.DrawRectangle(Pen, Rectangle)方法绘制一个矩形。
- 使用Image.Save()方法生成并保存图像。
下面的代码示例显示了如何在 C# 中的图像上绘制矩形。
// Create BmpOptions BmpOptions bmpCreateOptions = new BmpOptions(); bmpCreateOptions.BitsPerPixel = 32; // Define the source property for the instance of BmpOptions bmpCreateOptions.Source = new StreamSource(); // Creates an instance of Image and call create method by passing the // bmpCreateOptions object Image image = Image.Create(bmpCreateOptions, 500, 500); // Create and initialize an instance of Graphics class Graphics graphic = new Graphics(image); // Clear the image surface with White color graphic.Clear(Color.White); // Draw a dotted rectangle shape by specifying the Pen object having red // color and a rectangle structure graphic.DrawRectangle(new Pen(Color.Red, 3), new Rectangle(60, 40, 70, 120)); // Draw a continuous rectangle shape by specifying the Pen object having // solid brush with blue color and a rectangle structure graphic.DrawRectangle(new Pen(new SolidBrush(Color.Blue), 3), new Rectangle(40, 60, 120, 70)); // Save all changes image.Save("draw_reactangle.bmp");
以下是上述代码示例的输出。
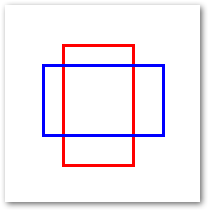
以上便是如何在 C# 中绘制形状:直线、圆弧、椭圆和矩形,要是您还有其他关于产品方面的问题,欢迎咨询我们,或者加入我们官方技术交流群761297826。