Step 1. 导入模块
In [1]:
import numpy as np import pandas as pd import plotly.graph_objects as go import plotly.express as px import jieba import jieba.analyse from stylecloud import gen_stylecloud from IPython.display import Image # 用于在jupyter lab中显示本地图片Step 2. 店铺评价数据分析及其可视化
2.1 店铺评价数据概览
In [3]:
df = pd.read_csv('/home/mw/input/luosifen4874/李子柒螺蛳粉评论.csv') df.head(6)Out[3]:
UserNick comment_time content auctionSku 0 太***4 2020-04-09 18:53:48 整体评价:还不错呦,东西蛮好的,第一次买李子柒家东西呀,不错呀,棒棒哒 胀包问题:没有呀,包... 口味:螺蛳粉3袋装 1 秘***0 2020-04-10 13:33:19 #柒家美拍达人#当吃到李子柒家第一口螺狮粉的时候,只想说,漫长的等待是值得的😁米粉爽滑Q弹,... 口味:螺蛳粉3袋装 2 m***3 2020-04-11 16:57:29 整体评价:不错 胀包问题:无 包装品质:好 口感味道:辣椒半包就够了,挺香的 新鲜度:可以 ... 口味:螺蛳粉3袋装 3 爱***6 2020-04-10 17:52:28 #柒家美拍达人# 等待了好久的美食终于到了,可以说期待了很久,拿到后激动了很久,想着一定给柒... 口味:螺蛳粉3袋装 4 t***2 2020-04-11 18:39:50 3 月11 号下单,4月11 号收到的,哈哈哈哈 不愧是我等了一个月的螺蛳粉,好吃到爆炸!!... 口味:螺蛳粉3袋装 5 t***9 2020-04-08 16:36:42 据说,螺蛳粉的臭味,是因酸笋,而螺蛳粉的汤,由螺丝肉和骨头熬成,此举使汤清甜与鲜美,后加浓郁... 口味:螺蛳粉3袋装 In [4]:
print("——" * 10) print('数据集存在重复值个数:') print(df.duplicated().sum()) print("——" * 10) print('数据集缺失值情况:') print(df.isna().sum()) print("——" * 10) print('数据集各字段类型:') print(df.dtypes) print("——" * 10) print('数据总体概览:') print(df.info())———————————————————— 数据集存在重复值个数: 20 ———————————————————— 数据集缺失值情况: UserNick 0 comment_time 0 content 0 auctionSku 0 dtype: int64 ———————————————————— 数据集各字段类型: UserNick object comment_time object content object auctionSku object dtype: object ———————————————————— 数据总体概览: <class 'pandas.core.frame.DataFrame'> RangeIndex: 2000 entries, 0 to 1999 Data columns (total 4 columns): UserNick 2000 non-null object comment_time 2000 non-null object content 2000 non-null object auctionSku 2000 non-null object dtypes: object(4) memory usage: 62.6+ KB NoneIn [5]:
df.drop_duplicates(inplace=True)2.2 商品评价数量走势图
In [6]:
df['comment_time'] = pd.to_datetime(df['comment_time']) df['comment_date'] = df['comment_time'].dt.date comment_num = df['comment_date'].value_counts().sort_index()Jan 52020Jan 19Feb 2Feb 16Mar 1Mar 15Mar 29Apr 12050100150200250300商品评价数量走势图日期热度
2.3 淘宝店铺评论数据处理
In [8]:
def judge_comment(df, result): # 创建一个空数据框 judges = pd.DataFrame(np.zeros(13 * len(df)).reshape(len(df),13), columns = ['品牌','物流正面','物流负面','包装正面','包装负面','原料正面', '原料负面','口感正面','口感负面','日期正面','日期负面', '性价比正面','性价比负面']) for i in range(len(result)): word = result[i] #李子柒的产品具有强IP属性,基本都是正面评价,这里不统计情绪,只统计提及次数 if '李子柒' in word or '子柒' in word or '小柒' in word or '李子七' in word or '小七' in word: judges.iloc[i]['品牌'] = 1 #先判断是不是物流相关的 if '物流' in word or '快递' in word or '配送' in word or '取货' in word: #再判断是正面还是负面情感 if '好' in word or '不错' in word or '棒' in word or '满意' in word or '迅速' in word: judges.iloc[i]['物流正面'] = 1 elif '慢' in word or '龟速' in word or '暴力' in word or '差' in word: judges.iloc[i]['物流负面'] = 1 #判断是否包装相关 if '包装' in word or '盒子' in word or '袋子' in word or '外观' in word: if '高端' in word or '大气' in word or '还行' in word or '完整' in word or '好' in word or\ '严实' in word or '紧' in word or '精致' in word: judges.iloc[i]['包装正面'] = 1 elif '破' in word or '破损' in word or '瘪' in word or '简陋' in word: judges.iloc[i]['包装负面'] = 1 #产品 #产品原料是牛肉为主,且评价大多会提到牛肉,因此我们把这个单独拎出来分析 if '米粉' in word or '汤' in word or '配料' in word or '腐竹' in word or '花生' in word: if '劲道' in word or '多' in word or '足' in word or '香' in word or '才' in word or\ '脆' in word or 'nice' in word: judges.iloc[i]['原料正面'] = 1 elif '小' in word or '少' in word or '没' in word: judges.iloc[i]['原料负面'] = 1 #口感的情绪 if '口味' in word or '味道' in word or '口感' in word or '吃起来' in word: if '不错' in word or '浓鲜' in word or '十足' in word or '鲜' in word or\ '可以' in word or '喜欢' in word or '符合' in word: judges.iloc[i]['口感正面'] = 1 elif '不好' in word or '不行' in word or '不鲜' in word or\ '太烂' in word: judges.iloc[i]['口感负面'] = 1 #口感方面,有些是不需要出现前置词,消费者直接评价好吃难吃的,例如: if '难吃' in word or '不好吃' in word: judges.iloc[i]['口感负面'] = 1 elif '好吃' in word or '香' in word: judges.iloc[i]['口感正面'] = 1 #日期是不是新鲜 if '日期' in word or '时间' in word or '保质期' in word: if '新鲜' in word: judges.iloc[i]['日期正面'] = 1 elif '久' in word or '长' in word: judges.iloc[i]['日期负面'] = 1 elif '过期' in word: judges.iloc[i]['日期负面'] = 1 #性价比 if '划算' in word or '便宜' in word or '赚了' in word or '囤货' in word or '超值' in word or \ '太值' in word or '物美价廉' in word or '实惠' in word or '性价比高' in word or '不贵' in word: judges.iloc[i]['性价比正面'] = 1 elif '贵' in word or '不值' in word or '亏了' in word or '不划算' in word or '不便宜' in word: judges.iloc[i]['性价比负面'] = 1 final_result = pd.concat([df,judges],axis = 1) return final_result # 得到数据框 judge = judge_comment(df, result=df.content) judge.head(5)Out[8]:
UserNick comment_time content auctionSku comment_date 品牌 物流正面 物流负面 包装正面 包装负面 原料正面 原料负面 口感正面 口感负面 日期正面 日期负面 性价比正面 性价比负面 0 太***4 2020-04-09 18:53:48 整体评价:还不错呦,东西蛮好的,第一次买李子柒家东西呀,不错呀,棒棒哒 胀包问题:没有呀,包... 口味:螺蛳粉3袋装 2020-04-09 1.0 0.0 0.0 1.0 0.0 0.0 0.0 1.0 0.0 1.0 0.0 0.0 0.0 1 秘***0 2020-04-10 13:33:19 #柒家美拍达人#当吃到李子柒家第一口螺狮粉的时候,只想说,漫长的等待是值得的😁米粉爽滑Q弹,... 口味:螺蛳粉3袋装 2020-04-10 1.0 0.0 0.0 0.0 0.0 1.0 0.0 1.0 0.0 0.0 0.0 0.0 0.0 2 m***3 2020-04-11 16:57:29 整体评价:不错 胀包问题:无 包装品质:好 口感味道:辣椒半包就够了,挺香的 新鲜度:可以 ... 口味:螺蛳粉3袋装 2020-04-11 0.0 0.0 0.0 1.0 0.0 1.0 0.0 1.0 1.0 0.0 0.0 0.0 0.0 3 爱***6 2020-04-10 17:52:28 #柒家美拍达人# 等待了好久的美食终于到了,可以说期待了很久,拿到后激动了很久,想着一定给柒... 口味:螺蛳粉3袋装 2020-04-10 1.0 0.0 0.0 0.0 0.0 1.0 0.0 1.0 0.0 0.0 0.0 0.0 0.0 4 t***2 2020-04-11 18:39:50 3 月11 号下单,4月11 号收到的,哈哈哈哈 不愧是我等了一个月的螺蛳粉,好吃到爆炸!!... 口味:螺蛳粉3袋装 2020-04-11 0.0 0.0 0.0 1.0 0.0 0.0 0.0 1.0 0.0 1.0 0.0 0.0 0.0 In [9]:
rank = judge.iloc[:,5:].sum().reset_index().sort_values(0,ascending=False) rank.columns = ['分类', '提及次数'] rank['占比'] = rank['提及次数'] / rank['提及次数'].sum() rank['高级分类'] = rank['分类'].str[:-2] rankOut[9]:
分类 提及次数 占比 高级分类 7 口感正面 1547.0 0.396565 口感 3 包装正面 718.0 0.184055 包装 5 原料正面 605.0 0.155088 原料 0 品牌 474.0 0.121507 9 日期正面 186.0 0.047680 日期 11 性价比正面 111.0 0.028454 性价比 10 日期负面 74.0 0.018969 日期 1 物流正面 62.0 0.015893 物流 6 原料负面 57.0 0.014612 原料 8 口感负面 34.0 0.008716 口感 12 性价比负面 31.0 0.007947 性价比 2 物流负面 1.0 0.000256 物流 4 包装负面 1.0 0.000256 包装 In [10]:
rank.loc[0, '高级分类'] = '品牌' rankOut[10]:
分类 提及次数 占比 高级分类 7 口感正面 1547.0 0.396565 口感 3 包装正面 718.0 0.184055 包装 5 原料正面 605.0 0.155088 原料 0 品牌 474.0 0.121507 品牌 9 日期正面 186.0 0.047680 日期 11 性价比正面 111.0 0.028454 性价比 10 日期负面 74.0 0.018969 日期 1 物流正面 62.0 0.015893 物流 6 原料负面 57.0 0.014612 原料 8 口感负面 34.0 0.008716 口感 12 性价比负面 31.0 0.007947 性价比 2 物流负面 1.0 0.000256 物流 4 包装负面 1.0 0.000256 包装 In [11]:
rank_num = rank.groupby('高级分类')['提及次数'].sum().sort_values(ascending=False).reset_index() rank_numOut[11]:
高级分类 提及次数 0 口感 1581.0 1 包装 719.0 2 原料 662.0 3 品牌 474.0 4 日期 260.0 5 性价比 142.0 6 物流 63.0 2.4 淘宝店铺消费者关注点占比分布
口感158140.5%包装71918.4%原料66217%品牌47412.2%日期2606.66%性价比1423.64%物流631.61%口感包装原料品牌日期性价比物流消费者关注点占比分布
2.7 店铺评价词云图
In [13]:
def get_cut_words(content_series): # 读入停用词表 stop_words = [] # 添加关键词 my_words = [] for i in my_words: jieba.add_word(i) # 自定义停用词 my_stop_words = ['40', 'hellip', '一袋', '一包', '一个月', '一点', '一个多月', '第一次', '哈哈哈', '螺狮粉', '螺蛳'] stop_words.extend(my_stop_words) # 分词 word_num = jieba.lcut(content_series.str.cat(sep='。'), cut_all=False) # 条件筛选 word_num_selected = [i for i in word_num if i not in stop_words and len(i)>=2] return word_num_selectedIn [14]:
text = get_cut_words(content_series=df['content']) text[:10]Building prefix dict from the default dictionary ... Dumping model to file cache C:\Users\H1638\AppData\Local\Temp\jieba.cache Loading model cost 2.150 seconds. Prefix dict has been built successfully.Out[14]:
['整体', '评价', '不错', '东西', '李子', '柒家', '东西', '不错呀', '棒棒', '问题']Out[15]:
Python数据可视化淘宝天猫店铺评论分析
最新推荐文章于 2024-06-28 11:33:51 发布
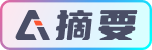