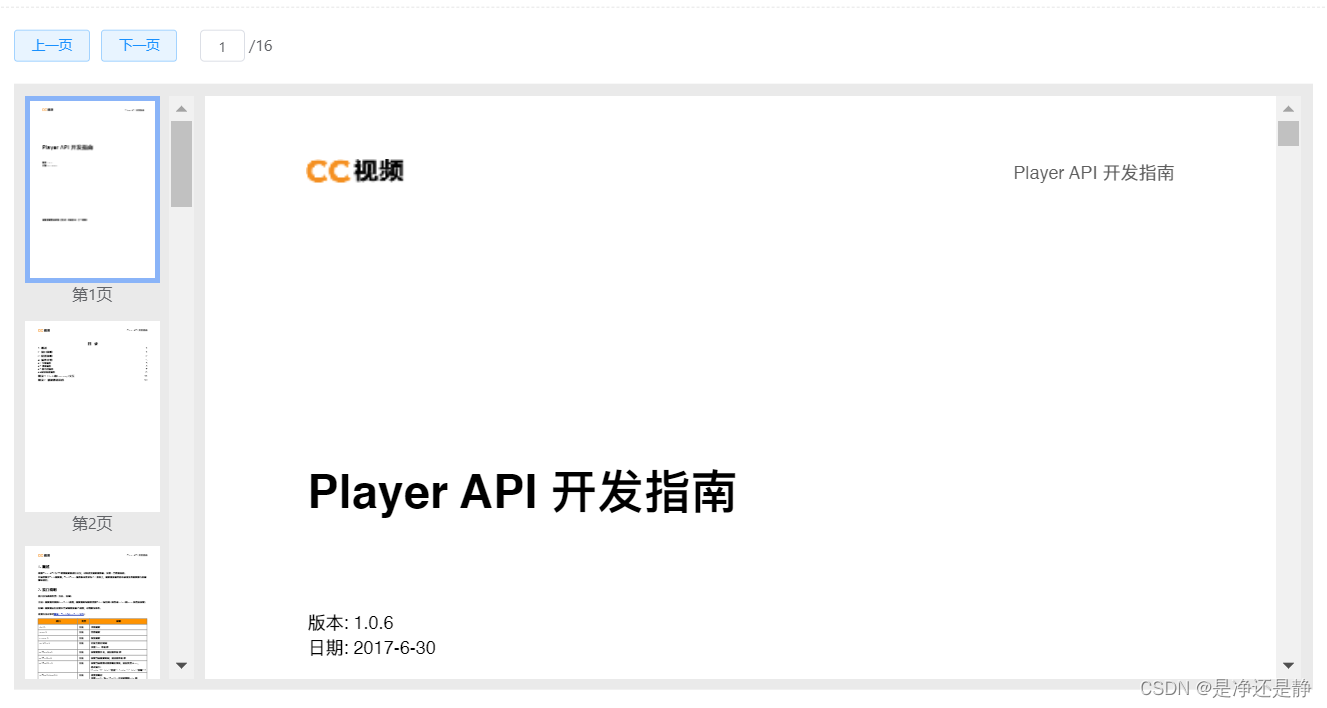
<div class="overflowWrap"
customClass="pdfLoading"
v-loading="loading"
element-loading-text="正在加载中..."
element-loading-spinner="el-icon-loading"
>
<div class="tools" style="display: flex;justify-content: flex-start;margin:0 0 20px">
<el-button type="primary" plain @click.stop="prePage" size="mini"> 上一页</el-button>
<el-button type="primary" plain @click.stop="nextPage" size="mini"> 下一页</el-button>
<div class="pageShow">
<el-input
style="width: 40px;"
v-model.trim="pageNum"
@input="pageNum==0?pageNum=1:(pageNum>pageTotalNum?pageNum=pageTotalNum:pageNum=pageNum.replace(/[^\d]/g,''))"
@change="inputPageNum()"
size="mini"
/>
/{{pageTotalNum}} </div>
</div>
<div class="pdfDiv" style="display: flex;padding: 10px;background: #eaeaea;justify-content: space-between;">
<div ref="pdfNav" style="height: calc(75vh - 75px);width: 150px; overflow-y: scroll;overflow-x: hidden;">
<div v-for="i in pageTotalNum" :key="i" style="width: 120px;height: 200px;text-align: center; cursor: pointer;" @click="changePageNum(i)">
<div :style="i==pageNum?'border: 5px solid #8ab4f8;':''"><pdf :src="pdfUrls" :page="i"></pdf></div>
<div>第{{ i }}页</div>
</div>
</div>
<div ref="pdfWrap" v-scroll="handleScroll" style="height: calc(75vh - 75px);width: calc(100% - 160px);overflow-y: scroll;overflow-x: hidden;">
<div v-for="i in pageTotalNum" :key="i" style="border-bottom: 5px solid #eaeaea;">
<pdf :src="pdfUrls" :page="i" @progress="loadedRatio = $event"></pdf>
</div>
</div>
</div>
</div>
import pdf from 'vue-pdf'
import axios from 'axios'
export default {
name: "dialog",
data(){
return{
loading:true,
title: "",
pdfUrls:'',
pdfName:'',
pageTotalNum: 1,
pageNum:1,
}
},
components:{
pdf
},
directives: {
scroll: {
inserted(el, binding) {
// 为元素添加滚动事件监听
el.addEventListener('scroll', () => {
// 判断用户滚动的距离
if (el.scrollTop > 0) {
binding.value(); // 调用绑定的方法
}
});
}
}
},
methods : {
/* 初始化弹框*/
init(annex,annexUrl){
this.pdfUrls=annexUrl;
this.pdfName=annex;
this.getNumPages(annexUrl)
this.pageNum=1;
this.loading=true;
},
//滚动
handleScroll () {
// console.log("滚动条为:",this.$refs.pdfWrap.scrollTop)
// console.log("滚动条高度为:",this.$refs.pdfWrap.scrollHeight)
// console.log("客户区高度为:",this.$refs.pdfWrap.clientHeight)
// console.log(Math.ceil(16*(this.$refs.pdfWrap.scrollTop / (this.$refs.pdfWrap.scrollHeight - this.$refs.pdfWrap.clientHeight))))
// this.scrollTop=this.$refs.pdfWrap.scrollTop
//this.$refs.pdfWrap.scrollTop+1这里是因为滚动的时候和切换页码有问题,简单粗暴的加了一点高度
const scrollPercentage=(this.$refs.pdfWrap.scrollTop+1) / (this.$refs.pdfWrap.scrollHeight/this.pageTotalNum)
this.pageNum=Math.ceil(scrollPercentage)
const scrollHeight1=this.$refs.pdfNav.scrollHeight
this.$refs.pdfNav.scrollTop=(this.pageNum-1) *(scrollHeight1/this.pageTotalNum)
},
//获取pdf页码
getNumPages(url) {
var loadingTask = pdf.createLoadingTask(url)
// var loadingTask = this.pdf.default.createLoadingTask(url)
loadingTask.promise.then(pdf => {
this.url = loadingTask
this.pageTotalNum = pdf.numPages
setTimeout(()=>{
this.loading=false;
},3000)
// console.log(this.pageTotalNum)
}).catch((err) => {
console.error('pdf加载失败')
})
},
//输入框切换
inputPageNum(){
const scrollHeight=this.$refs.pdfWrap.scrollHeight
this.$refs.pdfWrap.scrollTop=(this.pageNum-1) *(scrollHeight/this.pageTotalNum)
const scrollHeight1=this.$refs.pdfNav.scrollHeight
this.$refs.pdfNav.scrollTop=(this.pageNum-1) *(scrollHeight1/this.pageTotalNum)
},
//点击左侧小图切换
changePageNum(num) {
this.pageNum = num
const scrollHeight=this.$refs.pdfWrap.scrollHeight
this.$refs.pdfWrap.scrollTop=(this.pageNum-1) *(scrollHeight/this.pageTotalNum)
},
// 上一页函数,
prePage() {
var page = this.pageNum
page = page > 1 ? page - 1 : this.pageTotalNum
this.pageNum = page
const scrollHeight=this.$refs.pdfWrap.scrollHeight
this.$refs.pdfWrap.scrollTop=(this.pageNum-1) *(scrollHeight/this.pageTotalNum)
const scrollHeight1=this.$refs.pdfNav.scrollHeight
this.$refs.pdfNav.scrollTop=(this.pageNum-1) *(scrollHeight1/this.pageTotalNum)
},
// 下一页函数
nextPage() {
var page = this.pageNum
page = page < this.pageTotalNum ? page + 1 : 1
this.pageNum = page
console.log(this.pageNum)
// const scrollHeight=this.$refs.pdfWrap.scrollHeight - this.$refs.pdfWrap.clientHeight
const scrollHeight=this.$refs.pdfWrap.scrollHeight
this.$refs.pdfWrap.scrollTop=(this.pageNum-1) *(scrollHeight/this.pageTotalNum)
const scrollHeight1=this.$refs.pdfNav.scrollHeight
this.$refs.pdfNav.scrollTop=(this.pageNum-1) *(scrollHeight1/this.pageTotalNum)
},
//下载
async downloadPDF(pdfUrl) {
const response = await axios({
url: pdfUrl,
method: 'GET',
responseType: 'blob', // 必须指定为blob类型才能下载
});
const url = window.URL.createObjectURL(new Blob([response.data]));
const link = document.createElement('a');
link.href = url;
link.setAttribute('download', this.pdfName);
document.body.appendChild(link);
link.click();
},
handleDownLoad(){
this.downloadPDF(this.pdfUrls);
this.open=false;
}
},
mounted : function(){
}
}