1. Map集合概述和特点
-
Map集合概述
interface Map<K,V> K:键的类型;V:值的类型
-
Map集合的特点
- 双列集合,一个键对应一个值
- 键不可以重复,值可以重复
public class MapDemo1 { public static void main(String[] args) { Map<Integer,String> map = new HashMap<>(); map.put(01, "社会龙"); map.put(02, "三藏哥"); map.put(03, "七头哥"); map.put(03, "社会姐"); System.out.println(map); }
运行结果
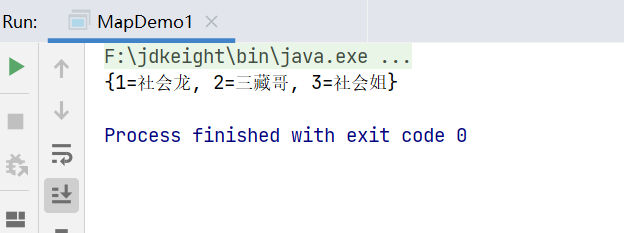
2. Map集合的基本功能
方法介绍
方法名 | 说明 |
---|---|
V put(K key,V value) | 添加元素 |
V remove(Object key) | 根据键删除键值对元素 |
void clear() | 移除所有的键值对元素 |
boolean containsKey(Object key) | 判断集合是否包含指定的键 |
boolean containsValue(Object value) | 判断集合是否包含指定的值 |
boolean isEmpty() | 判断集合是否为空 |
int size() | 集合的长度,也就是集合中键值对的个数 |
示例代码
package com.zhang.map;
import java.util.HashMap;
import java.util.Map;
/**
* map方法介绍
*/
public class MapDemo4 {
public static void main(String[] args) {
Map<Integer,String> map = new HashMap<>();
//1.添加元素
map.put(01, "社会龙");
map.put(02, "三藏哥");
map.put(03, "七头哥");
map.put(04, "社会姐");
System.out.println(map);
//2.根据建删除整个键值对
String removedEntry = map.remove(01);
//3.删除键值对元素
// map.clear();
//4.判断指定的键是否存在
boolean result1 = map.containsKey(02);
System.out.println(result1);
//5.判断指定的值是否存在
boolean result2 = map.containsValue("三藏哥");
System.out.println(result2);
//6.判断集合是否为空
boolean result3 = map.isEmpty();
System.out.println(result3);
//7.获取集合的长度,也就是集合的元素个素
int size = map.size();
System.out.println(size);
}
}
运行结果
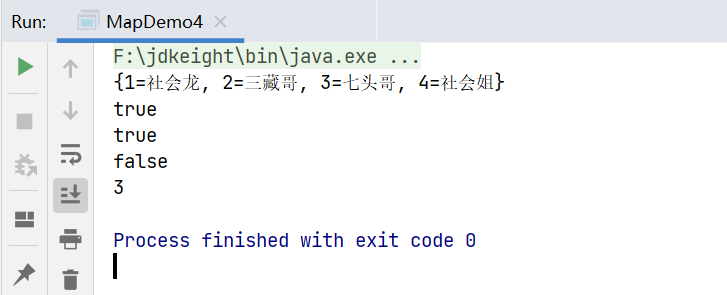
3.遍历map集合方式1:
思路:先获取全部键的集合,然后遍历集合根据键获取值
-
方法介绍
方法名 说明 V get(Object key) 根据键获取值 Set keySet() 获取所有键的集合 Collection values() 获取所有值的集合 Set<Map.Entry<K,V>> entrySet() 获取所有键值对对象的集合
package com.zhang.map;
import java.util.HashMap;
import java.util.Map;
import java.util.Set;
/**
* 遍历Map集合
* 方式一:先获取全部键的集合,然后遍历集合根据键获取值
*/
public class MapDemo2 {
public static void main(String[] args) {
Map<Integer,String> map = new HashMap<>();
map.put(01, "社会龙");
map.put(02, "三藏哥");
map.put(03, "七头哥");
map.put(04, "社会姐");
//1.获取全部的键
Set<Integer> keys = map.keySet();
for (Integer key : keys) {
//2.根据键获取值
String value = map.get(key);
System.out.println(key+ "====>>>" + value);
}
}
}
4. 遍历map集合方式2:
实现思路:
先获取全部整体键值对对象集合,遍历集合,
entry就是每一个键值对整体对象
package com.zhang.map;
import java.util.HashMap;
import java.util.Map;
import java.util.Set;
/**
* 遍历Map集合
* 方式二:先获取全部整体键值对对象集合,
* 遍历集合,entry就是每一个键值对整体对象
*/
public class MapDemo3 {
public static void main(String[] args) {
Map<Integer,String> map = new HashMap<>();
map.put(01, "社会龙");
map.put(02, "三藏哥");
map.put(03, "七头哥");
map.put(04, "社会姐");
//1.先获取全部整体键值对对象集合
Set<Map.Entry<Integer, String>> entries = map.entrySet();
//2.遍历集合,entry就是每一个键值对整体对象
for (Map.Entry<Integer, String> entry : entries) {
Integer key = entry.getKey();//获取键
String value = entry.getValue();//获取值
System.out.println(key+"=====>" +value);
}
}
}
运行结果
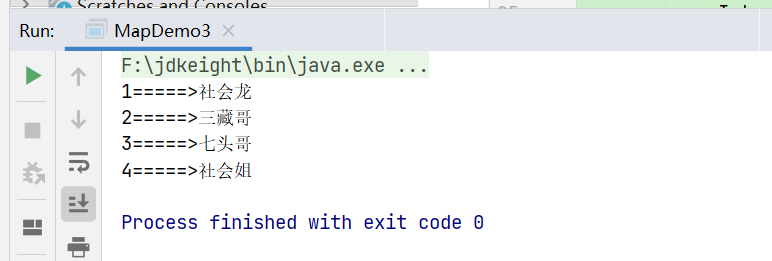
5. HashMap集合
5.1 HashMap集合概述和特点
- HashMap跟HashSet一样底层是哈希表结构的
- 依赖hashCode方法和equals方法保证键的唯一
- 如果键要存储的是自定义对象,需要重写hashCode和equals方法
5.2 HashMap集合应用案例
案例需求
- 创建一个HashMap集合,键是学生对象(Student),值是居住地 (String)。存储多个元素,并遍历。
- 要求保证键的唯一性:如果学生对象的成员变量值相同,我们就认为是同一个对象
**
* HashMap集合应用案例
* - 案例需求
*
* - 创建一个HashMap集合,键是学生对象(Student),值是居住地 (String)。存储多个元素,并遍历。
* - 要求保证键的唯一性:如果学生对象的成员变量值相同,我们就认为是同一个对象
*/
public class HashMapDemo1 {
public static void main(String[] args) {
HashMap<Student,String> hashMap = new HashMap<>();
Student student1 = new Student("社会龙",17);
Student student2 = new Student("三藏哥",18);
Student student3 = new Student("七头哥",19);
Student student4 = new Student("七头哥",19);
hashMap.put(student1, "安徽伏地魔");
hashMap.put(student2, "河源山泉水");
hashMap.put(student3, "梧州六堡茶");
hashMap.put(student4, "北海臭瓜皮");
//2.遍历集合
Set<Map.Entry<Student, String>> entries = hashMap.entrySet();
for (Map.Entry<Student, String> entry : entries) {
Student key = entry.getKey();
String value = entry.getValue();
System.out.println(key + "======>>>"+ value);
}
}
}
运行结果
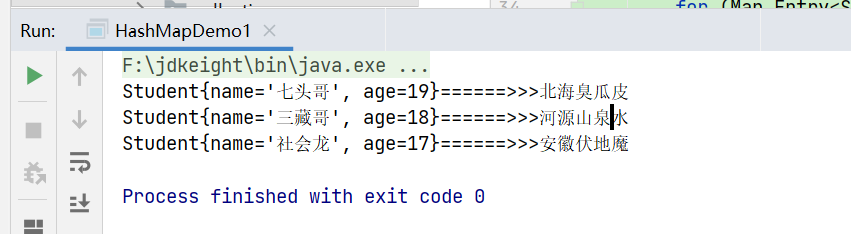
6. TreeMap集合
6.1 TreeMap集合概述和特点
- TreeMap底层是红黑树结构
- 依赖自然排序或者比较器排序,对键进行排序
- 如果键存储的是自定义对象,需要实现Comparable接口或者在创建TreeMap对象时候给出比较器排序规则
public class TreeMapDemo1 {
public static void main(String[] args) {
TreeMap<Integer,String> treeMap = new TreeMap<>();
treeMap.put(4, "社会姐");
treeMap.put(1, "社会龙");
treeMap.put(3, "七头哥");
treeMap.put(2, "三藏哥");
System.out.println(treeMap);
}
}
运行结果
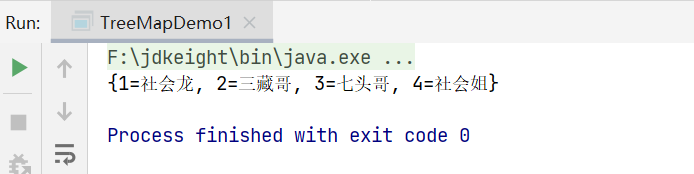
6.2 TreeMap集合应用案例
-
案例需求
- 创建一个TreeMap集合,键是学生对象(Student),值是籍贯(String),学生属性姓名和年龄,按照年龄进行排序并遍历
- 要求按照学生的年龄进行排序,如果年龄相同则按照姓名进行排序
比较器排序规则
package com.zhang.map.treemap;
import java.util.Comparator;
import java.util.TreeMap;
/**
* 案例需求
*
* + 创建一个TreeMap集合,键是学生对象(Student),值是籍贯(String),学生属性姓名和年龄,按照年龄进行排序并遍历
* + 要求按照学生的年龄进行排序,如果年龄相同则按照姓名进行排序
*/
public class TreeMapDemo2 {
public static void main(String[] args) {
TreeMap<Student,String> treeMap = new TreeMap<>(new Comparator<Student>() {
@Override
public int compare(Student o1, Student o2) {
int result = o1.getAge() - o2.getAge();
return result;
}
});
Student student1 = new Student("社会龙",56);
Student student2 = new Student("三藏哥",18);
Student student3 = new Student("七头哥",34);
Student student4 = new Student("七头哥",34);
Student student5 = new Student("社会姐",72);
treeMap.put(student1, "安徽合肥");
treeMap.put(student2, "广东河源");
treeMap.put(student3, "广西梧州");
treeMap.put(student4, "广西梧州");
treeMap.put(student5, "广西河池");
System.out.println(treeMap);
}
}
运行结果

7. 可变参数
-
可变参数介绍
- 可变参数又称参数个数可变,用作方法的形参出现,那么方法参数个数就是可变的了
- 方法的参数类型已经确定,个数不确定,我们可以使用可变参数
-
可变参数定义格式
修饰符 返回值类型 方法名(数据类型… 变量名) { } 例: public static int sum(int...a) {} 这里的变量其实是一个数组; 如果一个方法有多个参数,包含可变参数,可变参数要放在最后
需求:定义一个方法求N个数的和。
package com.zhang.exchageparam;
/**
* 求n个数的和
*/
public class Demo {
public static void main(String[] args) {
int sum = getSum(1, 2, 3, 4, 5);
System.out.println(sum);
}
public static int getSum(int...arr){
int sum = 0;
for (int i = 0; i < arr.length; i++) {
sum = sum+= arr[i];
}
return sum;
}
}
运行结果
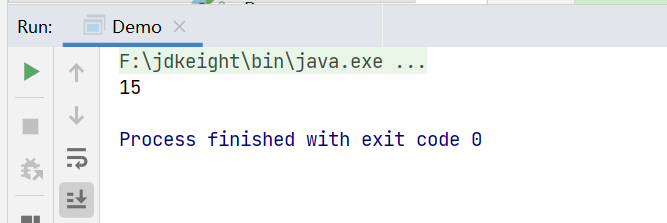
8 创建不可变集合
-
方法介绍
- 在List、Set、Map接口中,都存在of方法,可以创建一个不可变的集合
- 这个集合不能添加,不能删除,不能修改
- 但是可以结合集合的带参构造,实现集合的批量添加
- 在Map接口中,还有一个ofEntries方法可以提高代码的阅读性
- 首先会把键值对封装成一个Entry对象,再把这个Entry对象添加到集合当中
- 在List、Set、Map接口中,都存在of方法,可以创建一个不可变的集合
// static <E> List<E> of(E…elements) 创建一个具有指定元素的List集合对象
//static <E> Set<E> of(E…elements) 创建一个具有指定元素的Set集合对象
//static <K , V> Map<K,V> of(E…elements) 创建一个具有指定元素的Map集合对象
//集合的批量添加。
//首先是通过调用List.of方法来创建一个不可变的集合,of方法的形参就是一个可变参数。
//再创建一个ArrayList集合,并把这个不可变的集合中所有的数据,都添加到ArrayList中。
ArrayList<String> list3 = new ArrayList<>(List.of("a", "b", "c", "d"));
System.out.println(list3);