A. Sum
You are given three integers a a a, b b b, and c c c. Determine if one of them is the sum of the other two.
Input
The first line contains a single integer t t t ( 1 ≤ t ≤ 9261 1 \leq t \leq 9261 1≤t≤9261) — the number of test cases.
The description of each test case consists of three integers a a a, b b b, c c c ( 0 ≤ a , b , c ≤ 20 0 \leq a, b, c \leq 20 0≤a,b,c≤20).
Output
For each test case, output “YES” if one of the numbers is the sum of the other two, and “NO” otherwise.
You can output the answer in any case (for example, the strings “yEs”, “yes”, “Yes” and “YES” will be recognized as a positive answer).
Example
input
7
1 4 3
2 5 8
9 11 20
0 0 0
20 20 20
4 12 3
15 7 8
output
YES
NO
YES
YES
NO
NO
YES
Note
In the first test case, 1 + 3 = 4 1 + 3 = 4 1+3=4.
In the second test case, none of the numbers is the sum of the other two.
In the third test case, 9 + 11 = 20 9 + 11 = 20 9+11=20.
Tutorial
直接判断
Solution
for _ in range(int(input())):
a, b, c = map(int, input().split())
print("YES" if a + b == c or a + c == b or b + c == a else "NO")
B. Increasing
You are given an array a a a of n n n positive integers. Determine if, by rearranging the elements, you can make the array strictly increasing. In other words, determine if it is possible to rearrange the elements such that a 1 < a 2 < ⋯ < a n a_1 < a_2 < \dots < a_n a1<a2<⋯<an holds.
Input
The first line contains a single integer t t t ( 1 ≤ t ≤ 100 1 \leq t \leq 100 1≤t≤100) — the number of test cases.
The first line of each test case contains a single integer n n n ( 1 ≤ n ≤ 100 1 \leq n \leq 100 1≤n≤100) — the length of the array.
The second line of each test case contains n n n integers a i a_i ai ( 1 ≤ a i ≤ 1 0 9 1 \leq a_i \leq 10^9 1≤ai≤109) — the elements of the array.
Output
For each test case, output “YES” (without quotes) if the array satisfies the condition, and “NO” (without quotes) otherwise.
You can output the answer in any case (for example, the strings “yEs”, “yes”, “Yes” and “YES” will be recognized as a positive answer).
Example
input
3
4
1 1 1 1
5
8 7 1 3 4
1
5
output
NO
YES
YES
Note
In the first test case any rearrangement will keep the array [ 1 , 1 , 1 , 1 ] [1,1,1,1] [1,1,1,1], which is not strictly increasing.
In the second test case, you can make the array [ 1 , 3 , 4 , 7 , 8 ] [1,3,4,7,8] [1,3,4,7,8].
Tutorial
若需要数组严格递增,则需要数组没有两个相同的元素,可以排序后判断相邻元素是否相等,也可以直接用一个计时器判断一个数有没有出现第二次
Solution
for _ in range(int(input())):
flag = True
n = int(input())
a = list(map(int, input().split()))
a.sort()
for i in range(1, n):
if a[i] == a[i - 1]:
flag = False
break
print("YES" if flag else "NO")
C. Stripes
On an 8 × 8 8 \times 8 8×8 grid, some horizontal rows have been painted red, and some vertical columns have been painted blue, in some order. The stripes are drawn sequentially, one after the other. When the stripe is drawn, it repaints all the cells through which it passes.
Determine which color was used last.
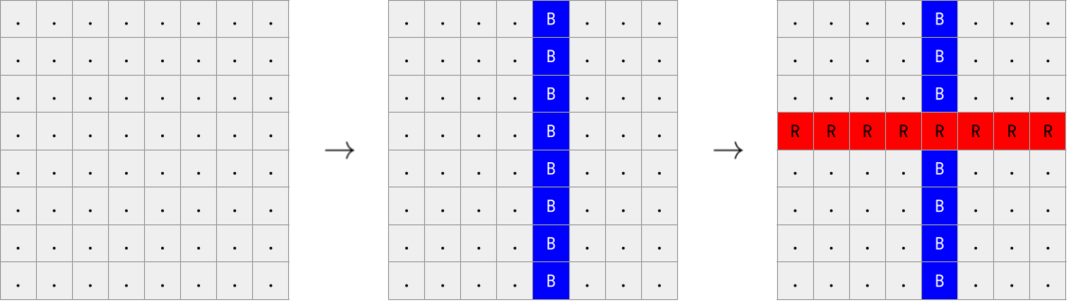
The red stripe was painted after the blue one, so the answer is R.
Input
The first line of the input contains a single integer t t t ( 1 ≤ t ≤ 4000 1 \leq t \leq 4000 1≤t≤4000) — the number of test cases. The description of test cases follows. There is an empty line before each test case.
Each test case consists of 8 8 8 lines, each containing 8 8 8 characters. Each of these characters is either ‘R’, ‘B’, or ‘.’, denoting a red square, a blue square, and an unpainted square, respectively.
It is guaranteed that the given field is obtained from a colorless one by drawing horizontal red rows and vertical blue columns.
At least one stripe is painted.
Output
For each test case, output ‘R’ if a red stripe was painted last, and ‘B’ if a blue stripe was painted last (without quotes).
Example
input
4
....B...
....B...
....B...
RRRRRRRR
....B...
....B...
....B...
....B...
RRRRRRRB
B......B
B......B
B......B
B......B
B......B
B......B
RRRRRRRB
RRRRRRBB
.B.B..BB
RRRRRRBB
.B.B..BB
.B.B..BB
RRRRRRBB
.B.B..BB
.B.B..BB
........
........
........
RRRRRRRR
........
........
........
........
output
R
B
B
R
Note
The first test case is pictured in the statement.
In the second test case, the first blue column is painted first, then the first and last red rows, and finally the last blue column. Since a blue stripe is painted last, the answer is B.
Tutorial
题目表明红色只会横向排布,蓝色只会纵向排布,而题目又保证至少有一个颜色,则只需要判断有没有哪一行是完整的红色就可以,否则就是蓝色
Solution
for _ in range(int(input())):
input()
g = [input() for __ in range(8)]
red = False
for s in g:
if s == "RRRRRRRR":
red = True
break
print("R" if red else "B")
D. Coprime
Given an array of n n n positive integers a 1 , a 2 , … , a n a_1, a_2, \dots, a_n a1,a2,…,an ( 1 ≤ a i ≤ 1000 1 \le a_i \le 1000 1≤ai≤1000). Find the maximum value of i + j i + j i+j such that a i a_i ai and a j a_j aj are coprime, † ^{\dagger} † or − 1 -1 −1 if no such i i i, j j j exist.
For example consider the array [ 1 , 3 , 5 , 2 , 4 , 7 , 7 ] [1, 3, 5, 2, 4, 7, 7] [1,3,5,2,4,7,7]. The maximum value of i + j i + j i+j that can be obtained is 5 + 7 5 + 7 5+7, since a 5 = 4 a_5 = 4 a5=4 and a 7 = 7 a_7 = 7 a7=7 are coprime.
† ^{\dagger} † Two integers p p p and q q q are coprime if the only positive integer that is a divisor of both of them is 1 1 1 (that is, their greatest common divisor is 1 1 1).
Input
The input consists of multiple test cases. The first line contains an integer t t t ( 1 ≤ t ≤ 10 1 \leq t \leq 10 1≤t≤10) — the number of test cases. The description of the test cases follows.
The first line of each test case contains an integer n n n ( 2 ≤ n ≤ 2 ⋅ 1 0 5 2 \leq n \leq 2\cdot10^5 2≤n≤2⋅105) — the length of the array.
The following line contains n n n space-separated positive integers a 1 a_1 a1, a 2 a_2 a2,…, a n a_n an ( 1 ≤ a i ≤ 1000 1 \leq a_i \leq 1000 1≤ai≤1000) — the elements of the array.
It is guaranteed that the sum of n n n over all test cases does not exceed 2 ⋅ 1 0 5 2\cdot10^5 2⋅105.
Output
For each test case, output a single integer — the maximum value of i + j i + j i+j such that i i i and j j j satisfy the condition that a i a_i ai and a j a_j aj are coprime, or output − 1 -1 −1 in case no i i i, j j j satisfy the condition.
Example
input
6
3
3 2 1
7
1 3 5 2 4 7 7
5
1 2 3 4 5
3
2 2 4
6
5 4 3 15 12 16
5
1 2 2 3 6
output
6
12
9
-1
10
7
Note
For the first test case, we can choose i = j = 3 i = j = 3 i=j=3, with sum of indices equal to 6 6 6, since 1 1 1 and 1 1 1 are coprime.
For the second test case, we can choose i = 7 i = 7 i=7 and j = 5 j = 5 j=5, with sum of indices equal to 7 + 5 = 12 7 + 5 = 12 7+5=12, since 7 7 7 and 4 4 4 are coprime.
Tutorial
根据题意得
1
≤
a
i
≤
1000
1 \leq a_i \leq 1000
1≤ai≤1000,所以只需要开一个长度为
1000
1000
1000 的数组用来存
a
i
a_i
ai 的出现过的最大位置就可以,然后对这
1000
1000
1000 个数进行一个时间复杂度为
O
(
100
0
2
)
O(1000 ^ 2)
O(10002) 的循环暴力寻找最大值就可以
Solution
import math
for _ in range(int(input())):
n = int(input())
ans = -1
idx = [0 for __ in range(1001)]
a = list(map(int, input().split()))
for i, x in enumerate(a):
idx[x] = i + 1
for i in range(1, 1001):
if idx[i]:
for j in range(i, 1001):
if idx[j] and math.gcd(i, j) == 1:
ans = max(ans, idx[i] + idx[j])
print(ans)
E. Scuza
Timur has a stairway with n n n steps. The i i i-th step is a i a_i ai meters higher than its predecessor. The first step is a 1 a_1 a1 meters higher than the ground, and the ground starts at 0 0 0 meters.
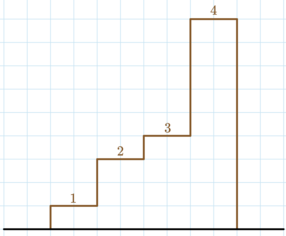
The stairs for the first test case.
Timur has q q q questions, each denoted by an integer k 1 , … , k q k_1, \dots, k_q k1,…,kq. For each question k i k_i ki, you have to print the maximum possible height Timur can achieve by climbing the steps if his legs are of length k i k_i ki. Timur can only climb the j j j-th step if his legs are of length at least a j a_j aj. In other words, k i ≥ a j k_i \geq a_j ki≥aj for each step j j j climbed.
Note that you should answer each question independently.
Input
The first line contains a single integer t t t ( 1 ≤ t ≤ 100 1 \leq t \leq 100 1≤t≤100) — the number of test cases.
The first line of each test case contains two integers n , q n, q n,q ( 1 ≤ n , q ≤ 2 ⋅ 1 0 5 1 \leq n, q \leq 2\cdot10^5 1≤n,q≤2⋅105) — the number of steps and the number of questions, respectively.
The second line of each test case contains n n n integers ( 1 ≤ a i ≤ 1 0 9 1 \leq a_i \leq 10^9 1≤ai≤109) — the height of the steps.
The third line of each test case contains q q q integers ( 0 ≤ k i ≤ 1 0 9 0 \leq k_i \leq 10^9 0≤ki≤109) — the numbers for each question.
It is guaranteed that the sum of n n n does not exceed 2 ⋅ 1 0 5 2\cdot10^5 2⋅105, and the sum of q q q does not exceed 2 ⋅ 1 0 5 2\cdot10^5 2⋅105.
Output
For each test case, output a single line containing q q q integers, the answer for each question.
Please note, that the answer for some questions won’t fit into 32-bit integer type, so you should use at least 64-bit integer type in your programming language (like long long for C++).
Example
input
3
4 5
1 2 1 5
1 2 4 9 10
2 2
1 1
0 1
3 1
1000000000 1000000000 1000000000
1000000000
output
1 4 4 9 9
0 2
3000000000
Note
Consider the first test case, pictured in the statement.
- If Timur’s legs have length 1 1 1, then he can only climb stair 1 1 1, so the highest he can reach is 1 1 1 meter.
- If Timur’s legs have length 2 2 2 or 4 4 4, then he can only climb stairs 1 1 1, 2 2 2, and 3 3 3, so the highest he can reach is 1 + 2 + 1 = 4 1+2+1=4 1+2+1=4 meters.
- If Timur’s legs have length 9 9 9 or 10 10 10, then he can climb the whole staircase, so the highest he can reach is 1 + 2 + 1 + 5 = 9 1+2+1+5=9 1+2+1+5=9 meters.
In the first question of the second test case, Timur has no legs, so he cannot go up even a single step.
Tutorial
开两个数组,其中一个用来存储前缀最大阶梯高度差,另一个用来存储当前位置的高度,这两个数组一定都是非递减序列,最后可以根据步长通过二分查找找出最多可以到达哪一级阶梯,直接输出当前阶梯的高度
Solution
#include <bits/stdc++.h>
using namespace std;
#define endl '\n'
#define int long long
void solve() {
int n, q, x;
cin >> n >> q;
vector<int> a(n + 1), mx(n + 1), high(n + 1);
for (int i = 1; i <= n; ++i) {
cin >> a[i];
mx[i] = max(mx[i - 1], a[i]);
high[i] = high[i - 1] + a[i];
}
while (q--) {
cin >> x;
int idx = ranges::upper_bound(mx, x) - mx.begin() - 1;
cout << high[idx] << " ";
}
cout << endl;
}
signed main() {
ios::sync_with_stdio(false);
cin.tie(0), cout.tie(0);
int test; cin >> test; while (test--)
solve();
return 0;
}
F. Smaller
Alperen has two strings, s s s and t t t which are both initially equal to “a”.
He will perform q q q operations of two types on the given strings:
- 1 k x 1 \;\; k \;\; x 1kx — Append the string x x x exactly k k k times at the end of string s s s. In other words, s : = s + x + ⋯ + x ⏟ k times s := s + \underbrace{x + \dots + x}_{k \text{ times}} s:=s+k times x+⋯+x.
- 2 k x 2 \;\; k \;\; x 2kx — Append the string x x x exactly k k k times at the end of string t t t. In other words, t : = t + x + ⋯ + x ⏟ k times t := t + \underbrace{x + \dots + x}_{k \text{ times}} t:=t+k times x+⋯+x.
After each operation, determine if it is possible to rearrange the characters of s s s and t t t such that s s s is lexicographically smaller † ^{\dagger} † than t t t.
Note that the strings change after performing each operation and don’t go back to their initial states.
† ^{\dagger} † Simply speaking, the lexicographical order is the order in which words are listed in a dictionary. A formal definition is as follows: string p p p is lexicographically smaller than string q q q if there exists a position i i i such that p i < q i p_i < q_i pi<qi, and for all j < i j < i j<i, p j = q j p_j = q_j pj=qj. If no such i i i exists, then p p p is lexicographically smaller than q q q if the length of p p p is less than the length of q q q. For example, abdc < abe \texttt{abdc} < \texttt{abe} abdc<abe and abc < abcd \texttt{abc} < \texttt{abcd} abc<abcd, where we write p < q p < q p<q if p p p is lexicographically smaller than q q q.
Input
The first line of the input contains an integer t t t ( 1 ≤ t ≤ 1 0 4 1 \leq t \leq 10^4 1≤t≤104) — the number of test cases.
The first line of each test case contains an integer q q q ( 1 ≤ q ≤ 1 0 5 ) (1 \leq q \leq 10^5) (1≤q≤105) — the number of operations Alperen will perform.
Then q q q lines follow, each containing two positive integers d d d and k k k ( 1 ≤ d ≤ 2 1 \leq d \leq 2 1≤d≤2; 1 ≤ k ≤ 1 0 5 1 \leq k \leq 10^5 1≤k≤105) and a non-empty string x x x consisting of lowercase English letters — the type of the operation, the number of times we will append string x x x and the string we need to append respectively.
It is guaranteed that the sum of q q q over all test cases doesn’t exceed 1 0 5 10^5 105 and that the sum of lengths of all strings x x x in the input doesn’t exceed 5 ⋅ 1 0 5 5 \cdot 10^5 5⋅105.
Output
For each operation, output “YES”, if it is possible to arrange the elements in both strings in such a way that s s s is lexicographically smaller than t t t and “NO” otherwise.
Example
input
3
5
2 1 aa
1 2 a
2 3 a
1 2 b
2 3 abca
2
1 5 mihai
2 2 buiucani
3
1 5 b
2 3 a
2 4 paiu
output
YES
NO
YES
NO
YES
NO
YES
NO
NO
YES
Note
In the first test case, the strings are initially $s = $ “a” and $t = $ “a”.
After the first operation the string t t t becomes “aaa”. Since “a” is already lexicographically smaller than “aaa”, the answer for this operation should be “YES”.
After the second operation string s s s becomes “aaa”, and since t t t is also equal to “aaa”, we can’t arrange s s s in any way such that it is lexicographically smaller than t t t, so the answer is “NO”.
After the third operation string t t t becomes “aaaaaa” and s s s is already lexicographically smaller than it so the answer is “YES”.
After the fourth operation s s s becomes “aaabb” and there is no way to make it lexicographically smaller than “aaaaaa” so the answer is “NO”.
After the fifth operation the string t t t becomes “aaaaaaabcaabcaabca”, and we can rearrange the strings to: “bbaaa” and “caaaaaabcaabcaabaa” so that s s s is lexicographically smaller than t t t, so we should answer “YES”.
Tutorial
根据题意分析,当
t
t
t 中出现不是 a
的元素后,必定可以使
s
s
s 的字典序小与
t
t
t 的字典序,因为
s
s
s 可以始终根据字母表顺序排列,而
t
t
t 可以根据字母表倒序排列,所以只需要记录
s
s
s 和
t
t
t 有没有出现过不是 a
的元素,如果都没有出现过不是 a
的元素就需要对两者的 a
的数量进行判断,根据两者的长度进行字典序判断
Solution
#include <bits/stdc++.h>
using namespace std;
#define endl '\n'
#define int long long
void solve() {
int q, d, k;
string s;
cin >> q;
bool flag[2] = {false};
int cnt[2] = {0};
while(q--) {
cin >> d >> k >> s;
d--;
for (char c : s) {
if (c == 'a') {
cnt[d] += k;
} else {
flag[d] = true;
}
}
if (flag[1]) {
cout << "YES\n";
} else if (flag[0]) {
cout << "NO\n";
} else {
cout << (cnt[0] < cnt[1] ? "YES" : "NO") << endl;
}
}
}
signed main() {
ios::sync_with_stdio(false);
cin.tie(0), cout.tie(0);
int test; cin >> test; while (test--)
solve();
return 0;
}
G. Orray
You are given an array a a a consisting of n n n nonnegative integers.
Let’s define the prefix OR array b b b as the array b i = a 1 O R a 2 O R … O R a i b_i = a_1~\mathsf{OR}~a_2~\mathsf{OR}~\dots~\mathsf{OR}~a_i bi=a1 OR a2 OR … OR ai, where O R \mathsf{OR} OR represents the bitwise OR operation. In other words, the array b b b is formed by computing the O R \mathsf{OR} OR of every prefix of a a a.
You are asked to rearrange the elements of the array a a a in such a way that its prefix OR array is lexicographically maximum.
An array x x x is lexicographically greater than an array y y y if in the first position where x x x and y y y differ, x i > y i x_i > y_i xi>yi.
Input
The first line of the input contains a single integer t t t ( 1 ≤ t ≤ 100 1 \le t \le 100 1≤t≤100) — the number of test cases. The description of test cases follows.
The first line of each test case contains a single integer n n n ( 1 ≤ n ≤ 2 ⋅ 1 0 5 1 \leq n \leq 2 \cdot 10^5 1≤n≤2⋅105) — the length of the array a a a.
The second line of each test case contains n n n nonnegative integers a 1 , … , a n a_1, \ldots, a_n a1,…,an ( 0 ≤ a i ≤ 1 0 9 0 \leq a_i \leq 10^9 0≤ai≤109).
It is guaranteed that the sum of n n n over all test cases does not exceed 2 ⋅ 1 0 5 2 \cdot 10^5 2⋅105.
Output
For each test case print n n n integers — any rearrangement of the array a a a that obtains the lexicographically maximum prefix OR array.
Example
input
5
4
1 2 4 8
7
5 1 2 3 4 5 5
2
1 101
6
2 3 4 2 3 4
8
1 4 2 3 4 5 7 1
output
8 4 2 1
5 2 1 3 4 5 5
101 1
4 3 2 2 3 4
7 1 4 2 3 4 5 1
Tutorial
由于本题是求前缀或,所以前缀或数组必定是一个非递减序列,由于需要构建出一个字典序最大的前缀或序列,所以原数组中最大值必定是前缀和数组的第一个值,后面再一次次遍历判断能不能通过或运算构造出出一个更大的值,否则后续的前缀或数组都是一样,即后面的元素可以任意排列
Solution
#include <bits/stdc++.h>
using namespace std;
#define endl '\n'
#define int long long
void solve() {
int n, mx = 0;
cin >> n;
vector<int> a(n + 1), ans;
vector<int> memo(n + 1);
for (int i = 1; i <= n; ++i) {
cin >> a[i];
}
while (true) {
int mid = mx, idx = 0;
for (int i = 1; i <= n; ++i) {
if (!memo[i] && (mx | a[i]) > mid) {
mid = mx | a[i];
idx = i;
}
}
if (!idx) {
break;
}
ans.emplace_back(a[idx]);
memo[idx] = true;
mx = mid;
}
for (int x : ans) {
cout << x << " ";
}
for (int i = 1; i <= n; ++i) {
if (!memo[i]) {
cout << a[i] << " ";
}
}
cout << endl;
}
signed main() {
ios::sync_with_stdio(false);
cin.tie(0), cout.tie(0);
int test; cin >> test; while (test--)
solve();
return 0;
}
写在后面
- D D D 题实际上是一个诈骗题,看似 2 ⋅ 1 0 5 2\cdot10^5 2⋅105 的数据量,但是实际上只有 1000 1000 1000 个数据,只需要对这 1000 1000 1000 个数据进行暴力比较就可以了
- E E E 题有意思的点在于要判断每一次询问在哪一个位置将不能继续往后爬,就需要将实际问题模型化,先转换为用代码可实现的数组再去解决这个问题
- F F F 题是一个脑筋急转弯式的构造题,需要想到 s s s 是按字母表顺序排列, t t t 是按照字母表倒序排列
- G G G 题需要想到 或运算 的不会变小的性质,这样就可以减少要排列的数量,很轻松排列整个数组了