A. Plus or Minus
You are given three integers a a a, b b b, and c c c such that exactly one of these two equations is true:
- a + b = c a+b=c a+b=c
- a − b = c a-b=c a−b=c
Output + if the first equation is true, and - otherwise.
Input
The first line contains a single integer t t t ( 1 ≤ t ≤ 162 1 \leq t \leq 162 1≤t≤162) — the number of test cases.
The description of each test case consists of three integers a a a, b b b, c c c ( 1 ≤ a , b ≤ 9 1 \leq a, b \leq 9 1≤a,b≤9, − 8 ≤ c ≤ 18 -8 \leq c \leq 18 −8≤c≤18). The additional constraint on the input: it will be generated so that exactly one of the two equations will be true.
Output
For each test case, output either + or - on a new line, representing the correct equation.
Example
input
11
1 2 3
3 2 1
2 9 -7
3 4 7
1 1 2
1 1 0
3 3 6
9 9 18
9 9 0
1 9 -8
1 9 10
output
+
-
-
+
+
-
+
+
-
-
+
Note
In the first test case, 1 + 2 = 3 1+2=3 1+2=3.
In the second test case, 3 − 2 = 1 3-2=1 3−2=1.
In the third test case, 2 − 9 = − 7 2-9=-7 2−9=−7. Note that c c c can be negative.
Tutorial
if 判断
Solution
for _ in range(int(input())):
a, b, c = map(int, input().split())
print("+" if a + b == c else "-")
B. Grab the Candies
Mihai and Bianca are playing with bags of candies. They have a row a a a of n n n bags of candies. The i i i-th bag has a i a_i ai candies. The bags are given to the players in the order from the first bag to the n n n-th bag.
If a bag has an even number of candies, Mihai grabs the bag. Otherwise, Bianca grabs the bag. Once a bag is grabbed, the number of candies in it gets added to the total number of candies of the player that took it.
Mihai wants to show off, so he wants to reorder the array so that at any moment (except at the start when they both have no candies), Mihai will have strictly more candies than Bianca. Help Mihai find out if such a reordering exists.
Input
The first line of the input contains an integer t t t ( 1 ≤ t ≤ 1000 1 \leq t \leq 1000 1≤t≤1000) — the number of test cases.
The first line of each test case contains a single integer n n n ( 1 ≤ n ≤ 100 1 \leq n \leq 100 1≤n≤100) — the number of bags in the array.
The second line of each test case contains n n n space-separated integers a i a_i ai ( 1 ≤ a i ≤ 100 1 \leq a_i \leq 100 1≤ai≤100) — the number of candies in each bag.
Output
For each test case, output “YES” (without quotes) if such a reordering exists, and “NO” (without quotes) otherwise.
You can output the answer in any case (for example, the strings “yEs”, “yes”, “Yes” and “YES” will be recognized as a positive answer).
Example
input
3
4
1 2 3 4
4
1 1 1 2
3
1 4 3
output
YES
NO
NO
Note
In the first test case, Mihai can reorder the array as follows: [ 4 , 1 , 2 , 3 ] [4, 1, 2, 3] [4,1,2,3]. Then the process proceeds as follows:
- the first bag has 4 4 4 candies, which is even, so Mihai takes it — Mihai has 4 4 4 candies, and Bianca has 0 0 0.
- the second bag has 1 1 1 candies, which is odd, so Bianca takes it — Mihai has 4 4 4 candies, and Bianca has 1 1 1.
- the third bag has 2 2 2 candies, which is even, so Mihai takes it — Mihai has 6 6 6 candies, and Bianca has 1 1 1.
- the fourth bag has 3 3 3 candies, which is odd, so Bianca takes it — Mihai has 6 6 6 candies, and Bianca has 4 4 4.
Since Mihai always has more candies than Bianca, this reordering works.
Tutorial
偶数总和比奇数总和大就为
Y
E
S
YES
YES,因为可以先把偶数全部拿完,再去拿奇数
Solution
for _ in range(int(input())):
n = int(input())
a = list(map(int, input().split()))
print("YES" if sum((x if x & 1 == 0 else 0) for x in a) > sum((x if x & 1 else 0) for x in a) else "NO")
C. Find and Replace
You are given a string s s s consisting of lowercase Latin characters. In an operation, you can take a character and replace all occurrences of this character with 0 \texttt{0} 0 or replace all occurrences of this character with 1 \texttt{1} 1.
Is it possible to perform some number of moves so that the resulting string is an alternating binary string † ^{\dagger} †?
For example, consider the string abacaba \texttt{abacaba} abacaba. You can perform the following moves:
- Replace a \texttt{a} a with 0 \texttt{0} 0. Now the string is 0b 0c 0b 0 \color{red}{\texttt{0}}\texttt{b}\color{red}{\texttt{0}}\texttt{c}\color{red}{\texttt{0}}\texttt{b}\color{red}{\texttt{0}} 0b0c0b0.
- Replace b \texttt{b} b with 1 \texttt{1} 1. Now the string is 0 10c0 10 {\texttt{0}}\color{red}{\texttt{1}}{\texttt{0}}\texttt{c}{\texttt{0}}\color{red}{\texttt{1}}{\texttt{0}} 010c010.
- Replace c \texttt{c} c with 1 \texttt{1} 1. Now the string is 010 1010 {\texttt{0}}{\texttt{1}}{\texttt{0}}\color{red}{\texttt{1}}{\texttt{0}}{\texttt{1}}{\texttt{0}} 0101010. This is an alternating binary string.
† ^{\dagger} †An alternating binary string is a string of 0 \texttt{0} 0s and 1 \texttt{1} 1s such that no two adjacent bits are equal. For example, 01010101 \texttt{01010101} 01010101, 101 \texttt{101} 101, 1 \texttt{1} 1 are alternating binary strings, but 0110 \texttt{0110} 0110, 0a0a0 \texttt{0a0a0} 0a0a0, 10100 \texttt{10100} 10100 are not.
Input
The input consists of multiple test cases. The first line contains an integer t t t ( 1 ≤ t ≤ 100 1 \leq t \leq 100 1≤t≤100) — the number of test cases. The description of the test cases follows.
The first line of each test case contains an integer n n n ( 1 ≤ n ≤ 2000 1 \leq n \leq 2000 1≤n≤2000) — the length of the string s s s.
The second line of each test case contains a string consisting of n n n lowercase Latin characters — the string s s s.
Output
For each test case, output “YES” (without quotes) if you can make the string into an alternating binary string, and “NO” (without quotes) otherwise.
You can output the answer in any case (for example, the strings “yEs”, “yes”, “Yes” and “YES” will be recognized as a positive answer).
Example
input
8
7
abacaba
2
aa
1
y
4
bkpt
6
ninfia
6
banana
10
codeforces
8
testcase
output
YES
NO
YES
YES
NO
YES
NO
NO
Note
The first test case is explained in the statement.
In the second test case, the only possible binary strings you can make are 00 \texttt{00} 00 and 11 \texttt{11} 11, neither of which are alternating.
In the third test case, you can make 1 \texttt{1} 1, which is an alternating binary string.
Tutorial
先把同一个字符的坐标存在一起,最后分别判断每一种字符的坐标的奇偶性是否一致,如果一致则为
Y
E
S
YES
YES,否则为
N
O
NO
NO
Solution
for _ in range(int(input())):
n = int(input())
s = input()
flag = True
all = [[] for _ in range(26)]
for i, c in enumerate(s):
all[ord(c) - ord('a')].append(i)
for a in all:
for j, x in enumerate(a):
if j and ((x & 1) ^ (a[j - 1] & 1)):
flag = False
break
if not flag:
break
print("YES" if flag else "NO")
D. Odd Queries
You have an array a 1 , a 2 , … , a n a_1, a_2, \dots, a_n a1,a2,…,an. Answer q q q queries of the following form:
- If we change all elements in the range a l , a l + 1 , … , a r a_l, a_{l+1}, \dots, a_r al,al+1,…,ar of the array to k k k, will the sum of the entire array be odd?
Note that queries are independent and do not affect future queries.
Input
Each test contains multiple test cases. The first line contains the number of test cases t t t ( 1 ≤ t ≤ 1 0 4 1 \le t \le 10^4 1≤t≤104). The description of the test cases follows.
The first line of each test case consists of 2 2 2 integers n n n and q q q ( 1 ≤ n ≤ 2 ⋅ 1 0 5 1 \le n \le 2 \cdot 10^5 1≤n≤2⋅105; 1 ≤ q ≤ 2 ⋅ 1 0 5 1 \le q \le 2 \cdot 10^5 1≤q≤2⋅105) — the length of the array and the number of queries.
The second line of each test case consists of n n n integers a i a_i ai ( 1 ≤ a i ≤ 1 0 9 1 \le a_i \le 10^9 1≤ai≤109) — the array a a a.
The next q q q lines of each test case consists of 3 3 3 integers l , r , k l,r,k l,r,k ( 1 ≤ l ≤ r ≤ n 1 \le l \le r \le n 1≤l≤r≤n; 1 ≤ k ≤ 1 0 9 1 \le k \le 10^9 1≤k≤109) — the queries.
It is guaranteed that the sum of n n n over all test cases doesn’t exceed 2 ⋅ 1 0 5 2 \cdot 10^5 2⋅105, and the sum of q q q doesn’t exceed 2 ⋅ 1 0 5 2 \cdot 10^5 2⋅105.
Output
For each query, output “YES” if the sum of the entire array becomes odd, and “NO” otherwise.
You can output the answer in any case (upper or lower). For example, the strings “yEs”, “yes”, “Yes”, and “YES” will be recognized as positive responses.
Example
input
2
5 5
2 2 1 3 2
2 3 3
2 3 4
1 5 5
1 4 9
2 4 3
10 5
1 1 1 1 1 1 1 1 1 1
3 8 13
2 5 10
3 8 10
1 10 2
1 9 100
output
YES
YES
YES
NO
YES
NO
NO
NO
NO
YES
Note
For the first test case:
- If the elements in the range ( 2 , 3 ) (2, 3) (2,3) would get set to 3 3 3 the array would become { 2 , 3 , 3 , 3 , 2 } \{2, 3, 3, 3, 2\} {2,3,3,3,2}, the sum would be 2 + 3 + 3 + 3 + 2 = 13 2+3+3+3+2 = 13 2+3+3+3+2=13 which is odd, so the answer is “YES”.
- If the elements in the range ( 2 , 3 ) (2, 3) (2,3) would get set to 4 4 4 the array would become { 2 , 4 , 4 , 3 , 2 } \{2, 4, 4, 3, 2\} {2,4,4,3,2}, the sum would be 2 + 4 + 4 + 3 + 2 = 15 2+4+4+3+2 = 15 2+4+4+3+2=15 which is odd, so the answer is “YES”.
- If the elements in the range ( 1 , 5 ) (1, 5) (1,5) would get set to 5 5 5 the array would become { 5 , 5 , 5 , 5 , 5 } \{5, 5, 5, 5, 5\} {5,5,5,5,5}, the sum would be 5 + 5 + 5 + 5 + 5 = 25 5+5+5+5+5 = 25 5+5+5+5+5=25 which is odd, so the answer is “YES”.
- If the elements in the range ( 1 , 4 ) (1, 4) (1,4) would get set to 9 9 9 the array would become { 9 , 9 , 9 , 9 , 2 } \{9, 9, 9, 9, 2\} {9,9,9,9,2}, the sum would be 9 + 9 + 9 + 9 + 2 = 38 9+9+9+9+2 = 38 9+9+9+9+2=38 which is even, so the answer is “NO”.
- If the elements in the range ( 2 , 4 ) (2, 4) (2,4) would get set to 3 3 3 the array would become { 2 , 3 , 3 , 3 , 2 } \{2, 3, 3, 3, 2\} {2,3,3,3,2}, the sum would be 2 + 3 + 3 + 3 + 2 = 13 2+3+3+3+2 = 13 2+3+3+3+2=13 which is odd, so the answer is “YES”.
Tutorial
利用前缀和,就可以将每个区间的和记录下来,然后对每次询问进行模拟
Solution
import sys
input = sys.stdin.readline
for _ in range(int(input())):
n, q = map(int, input().split())
a = [0] + list(map(int, input().split()))
for i in range(1, n + 1):
a[i] += a[i - 1]
for __ in range(q):
l, r, k = map(int, input().split())
all = a[n] - (a[r] - a[l - 1]) + (r - l + 1) * k
print("YES" if all & 1 else "NO")
E. Interview
This is an interactive problem. If you are unsure how interactive problems work, then it is recommended to read the guide for participants.
Before the last stage of the exam, the director conducted an interview. He gave Gon n n n piles of stones, the i i i-th pile having a i a_i ai stones.
Each stone is identical and weighs 1 1 1 grams, except for one special stone that is part of an unknown pile and weighs 2 2 2 grams.

A picture of the first test case. Pile 2 2 2 has the special stone. The piles have weights of 1 , 3 , 3 , 4 , 5 1,3,3,4,5 1,3,3,4,5, respectively.
Gon can only ask the director questions of one kind: he can choose k k k piles, and the director will tell him the total weight of the piles chosen. More formally, Gon can choose an integer k k k ( 1 ≤ k ≤ n 1 \leq k \leq n 1≤k≤n) and k k k unique piles p 1 , p 2 , … , p k p_1, p_2, \dots, p_k p1,p2,…,pk ( 1 ≤ p i ≤ n 1 \leq p_i \leq n 1≤pi≤n), and the director will return the total weight m p 1 + m p 2 + ⋯ + m p k m_{p_1} + m_{p_2} + \dots + m_{p_k} mp1+mp2+⋯+mpk, where m i m_i mi denotes the weight of pile i i i.
Gon is tasked with finding the pile that contains the special stone. However, the director is busy. Help Gon find this pile in at most 30 \mathbf{30} 30 queries.
Input
The input data contains several test cases. The first line contains one integer t t t ( 1 ≤ t ≤ 1000 1 \leq t \leq 1000 1≤t≤1000) — the number of test cases. The description of the test cases follows.
The first line of each test case contains a single integer n n n ( 1 ≤ n ≤ 2 ⋅ 1 0 5 1 \leq n \leq 2 \cdot 10^5 1≤n≤2⋅105) — the number of piles.
The second line of each test case contains n n n integers a i a_i ai ( 1 ≤ a i ≤ 1 0 4 1 \leq a_i \leq 10^4 1≤ai≤104) — the number of stones in each pile.
It is guaranteed that the sum of n n n over all test cases does not exceed 2 ⋅ 1 0 5 2 \cdot 10^5 2⋅105.
After reading the input for each test case, proceed with the interaction as follows.
Interaction
You can perform the operation at most 30 \mathbf{30} 30 times to guess the pile.
To make a guess, print a line with the following format:
- ? k p 1 p 2 p 3 . . . p k − 1 p k \texttt{?}\ k \ p_1 \ p_2 \ p_3 \ ... \ p_{k-1}\ p_k ? k p1 p2 p3 ... pk−1 pk ( 1 ≤ k ≤ n 1 \leq k \leq n 1≤k≤n; 1 ≤ p i ≤ n 1 \leq p_i \leq n 1≤pi≤n; all p i p_i pi are distinct) — the indices of the piles.
After each operation, you should read a line containing a single integer x x x — the sum of weights of the chosen piles. (Formally, x = m p 1 + m p 2 + ⋯ + m p k x = m_{p_1} + m_{p_2} + \dots + m_{p_k} x=mp1+mp2+⋯+mpk.)
When you know the index of the pile with the special stone, print one line in the following format: ! m \texttt{!}\ m ! m ( 1 ≤ m ≤ n 1 \leq m \leq n 1≤m≤n).
After that, move on to the next test case, or terminate the program if there are no more test cases remaining.
If your program performs more than 30 30 30 operations for one test case or makes an invalid query, you may receive a Wrong Answer verdict.
After you print a query or the answer, please remember to output the end of the line and flush the output. Otherwise, you may get Idleness limit exceeded or some other verdict. To do this, use the following:
- fflush(stdout) or cout.flush() in C++;
- System.out.flush() in Java;
- flush(output) in Pascal;
- stdout.flush() in Python;
- see the documentation for other languages.
It is additionally recommended to read the interactive problems guide for participants.
Hacks
To make a hack, use the following format.
The first line should contain a single integer t t t ( 1 ≤ t ≤ 1000 1 \leq t \leq 1000 1≤t≤1000) — the number of test cases.
The first line of each test case should contain two integers n , m n, m n,m ( 1 ≤ n ≤ 2 ⋅ 1 0 5 1 \leq n \leq 2 \cdot 10^5 1≤n≤2⋅105) – the number of piles and the pile with the special stone.
The second line of each test case should contain n n n integers a i a_i ai ( 1 ≤ a i ≤ 1 0 4 1 \leq a_i \leq 10^4 1≤ai≤104) — the number of stones in each pile.
Note that the interactor is not adaptive, meaning that the answer is known before the participant asks the queries and doesn’t depend on the queries asked by the participant.
Example
input
2
5
1 2 3 4 5
11
6
3
7
1 2 3 5 3 4 2
12
6
output
? 4 1 2 3 4
? 2 2 3
? 1 2
! 2
? 4 2 3 5 6
? 2 1 4
! 7
Note
In the first test case, the stone with weight two is located in pile 2 2 2, as shown in the picture. We perform the following interaction:
- ? 4 1 2 3 4 \texttt{? 4 1 2 3 4} ? 4 1 2 3 4 — ask the total weight of piles 1 1 1, 2 2 2, 3 3 3, and 4 4 4. The total weight we receive back is 1 + 3 + 3 + 4 = 11 1+3+3+4=11 1+3+3+4=11.
- ? 2 2 3 \texttt{? 2 2 3} ? 2 2 3 — ask the total weight of piles 2 2 2 and 3 3 3. The total weight we receive back is 3 + 3 = 6 3+3=6 3+3=6.
- ? 1 2 \texttt{? 1 2} ? 1 2 — ask the total weight of pile 2 2 2. The total weight we receive back is 3 3 3.
- ! 2 \texttt{! 2} ! 2 — we have figured out that pile 2 2 2 contains the special stone, so we output it and move on to the next test case.
In the second test case, the stone with weight two is located on index 7 7 7. We perform the following interaction:
- ? 4 2 3 5 6 \texttt{? 4 2 3 5 6} ? 4 2 3 5 6 — ask the total weight of piles 2 2 2, 3 3 3, 5 5 5, and 6 6 6. The total weight we receive back is 2 + 3 + 3 + 4 = 12 2+3+3+4=12 2+3+3+4=12.
- ? 2 1 4 \texttt{? 2 1 4} ? 2 1 4 — ask the total weight of piles 1 1 1 and 4 4 4. The total weight we receive back is 1 + 5 = 6 1+5=6 1+5=6.
- ! 7 \texttt{! 7} ! 7 — we have somehow figured out that pile 7 7 7 contains the special stone, so we output it and end the interaction.
Tutorial
本题是道交互题,题意为:给出
n
n
n 堆石子的数量,每个石子的重量都是
1
1
1,其中有一堆石子中的一个石子重量是
2
2
2,与机器交互找出那个石子在哪一堆
由于只有一堆有特殊的石子,即用二分查找即可逐渐向那一堆特殊石子靠近,最后剩下的一个就是答案
Solution
#include <bits/stdc++.h>
using namespace std;
#define endl '\n'
#define int long long
int ask(int l, int r) {
cout << "? " << r - l + 1 << ' ';
for (int i = l; i <= r; ++i) {
cout << i << " \n"[i == r];
}
cout.flush();
int x;
cin >> x;
return x;
}
void solve() {
int n, y, mid, x;
cin >> n ;
vector<int> a(n + 1);
for (int i = 1; i <= n; i++) {
cin >> a[i];
a[i] += a[i - 1];
}
int l = 1, r = n;
while (l < r) {
int mid = (l + r) >> 1;
int val = ask(l, mid);
val -= a[mid] - a[l - 1];
if (val) {
r = mid;
} else {
l = mid + 1;
}
}
cout << "! " << l << endl;
cout.flush();
}
signed main() {
ios::sync_with_stdio(false);
cin.tie(0);
int test; cin >> test; while (test--)
solve();
return 0;
}
F. Bouncy Ball
You are given a room that can be represented by a n × m n \times m n×m grid. There is a ball at position ( i 1 , j 1 ) (i_1, j_1) (i1,j1) (the intersection of row i 1 i_1 i1 and column j 1 j_1 j1), and it starts going diagonally in one of the four directions:
- The ball is going down and right, denoted by DR \texttt{DR} DR; it means that after a step, the ball’s location goes from ( i , j ) (i, j) (i,j) to ( i + 1 , j + 1 ) (i+1, j+1) (i+1,j+1).
- The ball is going down and left, denoted by DL \texttt{DL} DL; it means that after a step, the ball’s location goes from ( i , j ) (i, j) (i,j) to ( i + 1 , j − 1 ) (i+1, j-1) (i+1,j−1).
- The ball is going up and right, denoted by UR \texttt{UR} UR; it means that after a step, the ball’s location goes from ( i , j ) (i, j) (i,j) to ( i − 1 , j + 1 ) (i-1, j+1) (i−1,j+1).
- The ball is going up and left, denoted by UL \texttt{UL} UL; it means that after a step, the ball’s location goes from ( i , j ) (i, j) (i,j) to ( i − 1 , j − 1 ) (i-1, j-1) (i−1,j−1).
After each step, the ball maintains its direction unless it hits a wall (that is, the direction takes it out of the room’s bounds in the next step). In this case, the ball’s direction gets flipped along the axis of the wall; if the ball hits a corner, both directions get flipped. Any instance of this is called a bounce. The ball never stops moving.
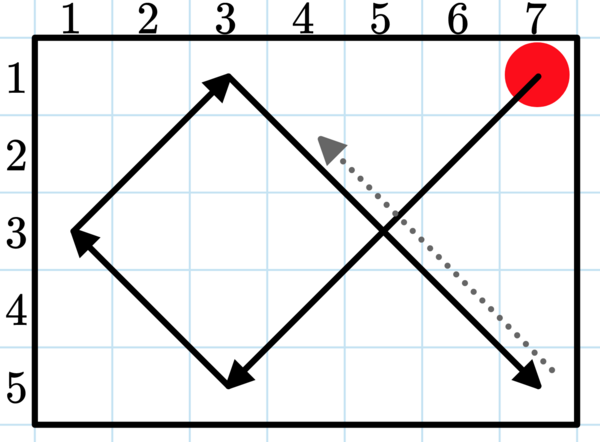
In the above example, the ball starts at ( 1 , 7 ) (1, 7) (1,7) and goes DL \texttt{DL} DL until it reaches the bottom wall, then it bounces and continues in the direction UL \texttt{UL} UL. After reaching the left wall, the ball bounces and continues to go in the direction UR \texttt{UR} UR. When the ball reaches the upper wall, it bounces and continues in the direction DR \texttt{DR} DR. After reaching the bottom-right corner, it bounces once and continues in direction UL \texttt{UL} UL, and so on.
Your task is to find how many bounces the ball will go through until it reaches cell ( i 2 , j 2 ) (i_2, j_2) (i2,j2) in the room, or report that it never reaches cell ( i 2 , j 2 ) (i_2, j_2) (i2,j2) by printing − 1 -1 −1.
Note that the ball first goes in a cell and only after that bounces if it needs to.
Input
The first line contains a single integer t t t ( 1 ≤ t ≤ 1000 1 \leq t \leq 1000 1≤t≤1000) — the number of test cases.
The first line of each test case contains six integers and a string n , m , i 1 , j 1 , i 2 , j 2 , d n, m, i_1, j_1, i_2, j_2, d n,m,i1,j1,i2,j2,d ( 2 ≤ n , m ≤ 25000 2 \leq n, m \leq 25000 2≤n,m≤25000; 1 ≤ i 1 , i 2 ≤ n 1 \leq i_1, i_2 \leq n 1≤i1,i2≤n; 1 ≤ j 1 , j 2 ≤ m 1 \leq j_1, j_2 \leq m 1≤j1,j2≤m; d ∈ { DR , DL , UR , UL } d \in\{ \texttt{DR}, \texttt{DL}, \texttt{UR}, \texttt{UL}\} d∈{DR,DL,UR,UL}) — the dimensions of the grid, the starting coordinates of the ball, the coordinates of the final cell and the starting direction of the ball.
It is guaranteed that the sum of n ⋅ m n \cdot m n⋅m over all test cases does not exceed 5 ⋅ 1 0 4 5 \cdot 10^4 5⋅104.
Output
For each test case, output a single integer — the number of bounces the ball does until it reaches cell ( i 2 , j 2 ) (i_2, j_2) (i2,j2) for the first time, or − 1 -1 −1 if the ball never reaches the final cell.
Example
input
6
5 7 1 7 2 4 DL
5 7 1 7 3 2 DL
3 3 1 3 2 2 UR
2 4 2 1 2 2 DR
4 3 1 1 1 3 UL
6 4 1 2 3 4 DR
output
3
-1
1
-1
4
0
Tutorial
没有特殊处理,直接暴力模拟,模拟时注意边界问题
Solution
#include <bits/stdc++.h>
using namespace std;
#define endl '\n'
#define int long long
void solve() {
int n, m, i1, j1, i2, j2, ans = 0;
string d;
cin >> n >> m >> i1 >> j1 >> i2 >> j2 >> d;
for (int i = 0; i < 2 * n * m; i++) {
if (i1 == i2 && j1 == j2) {
cout << ans << endl;
return;
}
bool b = 0;
if (i1 == 1 && d[0] == 'U') {
d[0] = 'D';
b = 1;
}
if (i1 == n && d[0] == 'D') {
d[0] = 'U';
b = 1;
}
if (j1 == 1 && d[1] == 'L') {
d[1] = 'R';
b = 1;
}
if (j1 == m && d[1] == 'R') {
d[1] = 'L';
b = 1;
}
ans += b;
i1 += (d[0] == 'U' ? -1 : 1);
j1 += (d[1] == 'L' ? -1 : 1);
}
cout << "-1\n";
}
signed main() {
ios::sync_with_stdio(false);
cin.tie(0), cout.tie(0);
int test; cin >> test; while (test--)
solve();
return 0;
}
G2. Subsequence Addition (Hard Version)
The only difference between the two versions is that in this version, the constraints are higher.
Initially, array a a a contains just the number 1 1 1. You can perform several operations in order to change the array. In an operation, you can select some subsequence † ^{\dagger} † of a a a and add into a a a an element equal to the sum of all elements of the subsequence.
You are given a final array c c c. Check if c c c can be obtained from the initial array a a a by performing some number (possibly 0) of operations on the initial array.
† ^{\dagger} † A sequence b b b is a subsequence of a sequence a a a if b b b can be obtained from a a a by the deletion of several (possibly zero, but not all) elements. In other words, select k k k ( 1 ≤ k ≤ ∣ a ∣ 1 \leq k \leq |a| 1≤k≤∣a∣) distinct indices i 1 , i 2 , … , i k i_1, i_2, \dots, i_k i1,i2,…,ik and insert anywhere into a a a a new element with the value equal to a i 1 + a i 2 + ⋯ + a i k a_{i_1} + a_{i_2} + \dots + a_{i_k} ai1+ai2+⋯+aik.
Input
The first line of the input contains an integer t t t ( 1 ≤ t ≤ 1000 1 \leq t \leq 1000 1≤t≤1000) — the number of test cases. The description of the test cases follows.
The first line of each test case contains a single integer n n n ( 1 ≤ n ≤ 2 ⋅ 1 0 5 1 \leq n \leq 2 \cdot 10^5 1≤n≤2⋅105) — the number of elements the final array c c c should have.
The second line of each test case contains n n n space-separated integers c i c_i ci ( 1 ≤ c i ≤ 2 ⋅ 1 0 5 1 \leq c_i \leq 2 \cdot 10^5 1≤ci≤2⋅105) — the elements of the final array c c c that should be obtained from the initial array a a a.
It is guaranteed that the sum of n n n over all test cases does not exceed 2 ⋅ 1 0 5 2 \cdot 10^5 2⋅105.
Output
For each test case, output “YES” (without quotes) if such a sequence of operations exists, and “NO” (without quotes) otherwise.
You can output the answer in any case (for example, the strings “yEs”, “yes”, “Yes” and “YES” will be recognized as a positive answer).
Example
input
6
1
1
1
2
5
5 1 3 2 1
5
7 1 5 2 1
3
1 1 1
5
1 1 4 2 1
output
YES
NO
YES
NO
YES
YES
Note
For the first test case, the initial array a a a is already equal to [ 1 ] [1] [1], so the answer is “YES”.
For the second test case, performing any amount of operations will change a a a to an array of size at least two which doesn’t only have the element 2 2 2, thus obtaining the array [ 2 ] [2] [2] is impossible and the answer is “NO”.
For the third test case, we can perform the following operations in order to obtain the final given array c c c:
- Initially, a = [ 1 ] a = [1] a=[1].
- By choosing the subsequence [ 1 ] [1] [1], and inserting 1 1 1 in the array, a a a changes to [ 1 , 1 ] [1, 1] [1,1].
- By choosing the subsequence [ 1 , 1 ] [1, 1] [1,1], and inserting 1 + 1 = 2 1+1=2 1+1=2 in the middle of the array, a a a changes to [ 1 , 2 , 1 ] [1, 2, 1] [1,2,1].
- By choosing the subsequence [ 1 , 2 ] [1, 2] [1,2], and inserting 1 + 2 = 3 1+2=3 1+2=3 after the first 1 1 1 of the array, a a a changes to [ 1 , 3 , 2 , 1 ] [1, 3, 2, 1] [1,3,2,1].
- By choosing the subsequence [ 1 , 3 , 1 ] [1, 3, 1] [1,3,1] and inserting 1 + 3 + 1 = 5 1+3+1=5 1+3+1=5 at the beginning of the array, a a a changes to [ 5 , 1 , 3 , 2 , 1 ] [5, 1, 3, 2, 1] [5,1,3,2,1] (which is the array we needed to obtain).
Tutorial
由题意得,对题目给出的字符串排序后,前两个元素必定是
1
1
1,后面的元素都不能比之前元素的和还要大,否则就不能凑出来,所以只需要对数组排序后,从前往后遍历判断即可
Solution
#include <bits/stdc++.h>
using namespace std;
#define endl '\n'
#define int long long
void solve() {
int n, cnt;
cin >> n;
vector<int> c(n);
for (int &ci : c) {
cin >> ci;
}
ranges::sort(c);
if (n < 3) {
cout << (accumulate(c.begin(), c.end(), 0ll) == n ? "YES\n" : "NO\n");
return;
}
if ((cnt = c[0] + c[1]) != 2) {
cout << "NO\n";
return;
}
for (int i = 2; i < n; ++i) {
if (c[i] > cnt) {
cout << "NO\n";
return;
}
cnt += c[i];
}
cout << "YES\n";
}
signed main() {
ios::sync_with_stdio(false);
cin.tie(0), cout.tie(0);
int test; cin >> test; while (test--)
solve();
return 0;
}