在上一个 SSM 配置的基础上运用 SSM 框架对数据库进行增删改查操作
具体操作与 MVC 练习时结合 JSP 页面对数据库进行增删改查的操作相似
目录结构
1、对 ProductMapper 文件进行修改,补全对数据库的增删改查操作
这些方法名与 Product.xml 文件中的标签 id 相对应
package com.mr.mapper;
import java.util.List;
import java.util.Map;
import com.mr.pojo.Product;
public interface ProductMapper {
public List<Product> list(Map<String, Object> map);
public void add(Product product);
public void delete(Product product);
public Product get(int id);
public void update(Product product);
public int getTotal();
}
2、修改 Product.xml 文档
增加了 获取数据的个数、以及修改了查询标签,让它分页显示,用 Map 类型的对象来传递参数
<?xml version="1.0" encoding="UTF-8"?>
<!DOCTYPE mapper
PUBLIC "-//mybatis.org//DTD Mapper 3.0//EN"
"http://mybatis.org/dtd/mybatis-3-mapper.dtd">
<mapper namespace="com.mr.mapper.ProductMapper">
<insert id="add" parameterType="Product" >
insert into product ( name , price ) values (#{name},#{price})
</insert>
<delete id="delete" parameterType="Product" >
delete from product where id= #{id}
</delete>
<select id="get" parameterType="_int" resultType="Product">
select * from product where id= #{id}
</select>
<update id="update" parameterType="Product" >
update product
<set>
<if test="name!=null">name=#{name},</if>
<if test="price!=null">price=#{price}</if>
</set>
where id=#{id}
</update>
<select id="list" resultType="Product" parameterType="map">
select * from product
<if test="start!=null">
order by id asc limit #{start},#{count}
</if>
</select>
<select id="getTotal" resultType="_int">
select count(*) from product
</select>
</mapper>
3、修改接口 ProductService,新增对数据库操作的方法,与映射类 ProductMapper相对应
package com.mr.service;
import java.util.List;
import java.util.Map;
import com.mr.pojo.Product;
public interface ProductService {
List<Product> list(Map<String, Object> map);
void add(Product product);
void delete(Product product);
Product get(int id);
void update(Product product);
int getTotal();
}
4、修改业务类 ProductServiceImpl,与接口 ProductService 相对应
package com.mr.service.impl;
import java.util.List;
import java.util.Map;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.stereotype.Service;
import com.mr.mapper.ProductMapper;
import com.mr.pojo.Product;
import com.mr.service.ProductService;
@Service
public class ProductServiceImpl implements ProductService {
@Autowired
public ProductMapper productMapper;
public List<Product> list(Map<String, Object> map) {
return productMapper.list(map);
}
public void add(Product product) {
productMapper.add(product);
}
public void delete(Product product) {
productMapper.delete(product);
}
public Product get(int id) {
return productMapper.get(id);
}
public void update(Product product) {
productMapper.update(product);
}
public int getTotal() {
return productMapper.getTotal();
}
}
5、修改控制器类,新增相应的控制器
编写控制器方法的时候,一个控制器对应的一个 RequestMapping,在其控制器类中编写其对应的逻辑代码
分页查询:分析 start 与 count,并从页面中获取值,并以 Map 的形式传递给映射 xml 文件中相应的标签,从而执行相应的 sql 语句。
package com.mr.controller;
import java.io.IOException;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
import javax.servlet.http.HttpServletRequest;
import javax.servlet.http.HttpServletResponse;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.stereotype.Controller;
import org.springframework.web.bind.annotation.RequestMapping;
import org.springframework.web.servlet.ModelAndView;
import com.mr.pojo.Product;
import com.mr.service.ProductService;
@Controller
@RequestMapping("")
public class ProductController {
@Autowired
ProductService productService;
// 查询数据
@RequestMapping("listProduct")
public ModelAndView listProduct(HttpServletRequest request, HttpServletResponse response) throws IOException {
ModelAndView mav = new ModelAndView();
int start = 0, count = 5;
int last;
int total = productService.getTotal();
System.out.println("总共有" + total + "条数据");
try {
start = Integer.parseInt(request.getParameter("start"));
} catch (NumberFormatException e) {
System.out.println("找不到提交的start");
}
// 下一页
int next = start + count;
// 上一页
int prime = start - count;
if (total % count == 0) {
last = total - start;
} else {
last = total - total % count;
}
next = next > total ? last : next;
prime = prime > 0 ? prime : 0;
Map<String, Object> map = new HashMap<>();
System.out.println("当前页面的start值:" + start);
map.put("start", start);
map.put("count", count);
List<Product> csList = productService.list(map);
mav.addObject("next", next);
mav.addObject("prime", prime);
mav.addObject("last", last);
mav.addObject("cs", csList);
mav.setViewName("listProduct");
return mav;
}
// 增添数据
@RequestMapping("addProduct")
public void addProduct(HttpServletRequest request, HttpServletResponse response) throws IOException {
ModelAndView modelAndView = new ModelAndView();
String name = request.getParameter("name");
System.out.println("name 为:" + name);
float price = Float.parseFloat(request.getParameter("price"));
System.out.println("price 为:" + price);
Product product = new Product();
product.setName(name);
product.setPrice(price);
productService.add(product);
System.out.println("成功添加!");
response.sendRedirect("listProduct");
}
// 删除数据
@RequestMapping("deleteProduct")
public void deleteProduct(HttpServletRequest request, HttpServletResponse response) throws IOException {
int id = Integer.parseInt(request.getParameter("id"));
System.out.println(id);
Product product = new Product();
product.setId(id);
productService.delete(product);
System.out.println("成功删除!");
response.sendRedirect("listProduct");
}
// 修改数据
@RequestMapping("updateProduct")
public ModelAndView updateProduct(HttpServletRequest request, HttpServletResponse response) throws IOException {
ModelAndView modelAndView = new ModelAndView();
int id = Integer.parseInt(request.getParameter("id"));
System.out.println(id);
Product product = productService.get(id);
try {
float price = Float.parseFloat(request.getParameter("price"));
System.out.println("price 为:" + price);
String name = request.getParameter("name");
System.out.println("name 为:" + name);
product.setName(name);
product.setPrice(price);
productService.update(product);
System.out.println("修改成功!");
response.sendRedirect("listProduct");
} catch (Exception e) {
}
modelAndView.addObject(product);
modelAndView.setViewName("updateProduct");
return modelAndView;
}
}
6、修改 listProduct.jsp页面,实现数据的查询、分页效果、以及增添、删除效果
<%@ page language="java" contentType="text/html; charset=UTF-8"
pageEncoding="UTF-8" import="java.util.*"%>
<%@ taglib uri="http://java.sun.com/jsp/jstl/core" prefix="c"%>
<style>
table{
position:relative;
top:20px;
left:500px;
padding-bottom: 50px;
}
td{
border:1px grey solid;
padding: 10px;
text-align: center;
}
a {
text-decoration: none;
font-size: larger;
}
.box{
position: relative;
left:543px;
padding-bottom: 50px;
}
</style>
<table>
<tr>
<td>id</td>
<td>name</td>
<td>price</td>
<td>编辑</td>
<td>删除</td>
</tr>
<c:forEach items="${cs}" var="c" varStatus="st">
<tr>
<td>${c.id}</td>
<td>${c.name}</td>
<td>${c.price }</td>
<td><a href="updateProduct?id=${c.id }">编辑</a></td>
<td><a href="deleteProduct?id=${c.id }">删除</a></td>
</tr>
</c:forEach>
</table>
<span class="box">
<span><a href="listProduct?start=0">首页</a></span>
<span><a href="listProduct?start=${prime }">上一页</a></span>
<span><a href="listProduct?start=${next }">下一页</a></span>
<span><a href="listProduct?start=${last }">末页</a></span>
</span>
<form action="addProduct">
<table>
<tr><td colspan="2">要增加的数据</td></tr>
<tr>
<td>name:</td>
<td><input type="text" name="name"></td>
</tr>
<tr>
<td>price:</td>
<td><input type="text" name="price"></td>
</tr>
<tr><td colspan="2"><input type="submit" value="提交"></a></td></tr>
</table>
</form>
7、建立 updateProduct.jsp 页面,实现数据的修改功能
<%@ page language="java" import="java.sql.*" contentType="text/html; charset=utf-8"
pageEncoding="utf-8"%>
<%@ taglib uri="http://java.sun.com/jsp/jstl/core" prefix="c"%>
<!DOCTYPE html>
<html>
<head>
<meta charset="utf-8">
<title></title>
</head>
<body>
<form action="updateProduct">
<table>
<tr><td colspan="2">填写要修改的数据</td></tr>
<tr>
<td>name:</td>
<td><input type="text" name="name"></td>
</tr>
<tr>
<td>price:</td>
<td><input type="text" name="price"></td>
</tr>
<tr><td colspan="2"><input type="submit" value="提交"></a></td></tr>
<input type="hidden" name="id" value=${product.id}>
</table>
</form>
</body>
</html>
最后,SSM 练习就完成了j,具体运行效果如下
初始效果:
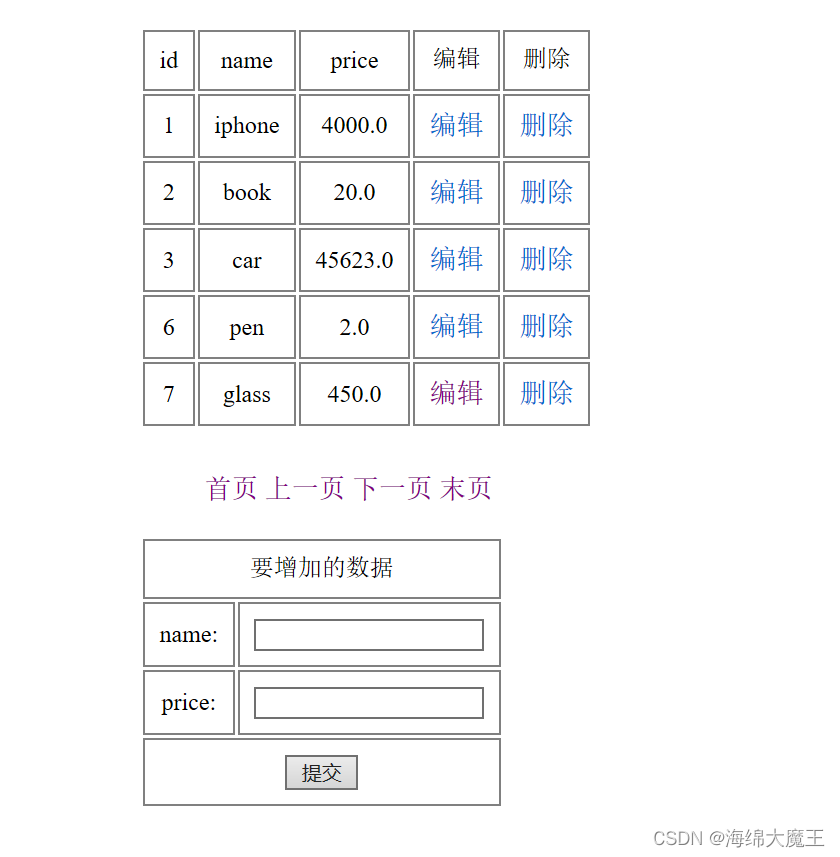
新增数据:
修改数据:
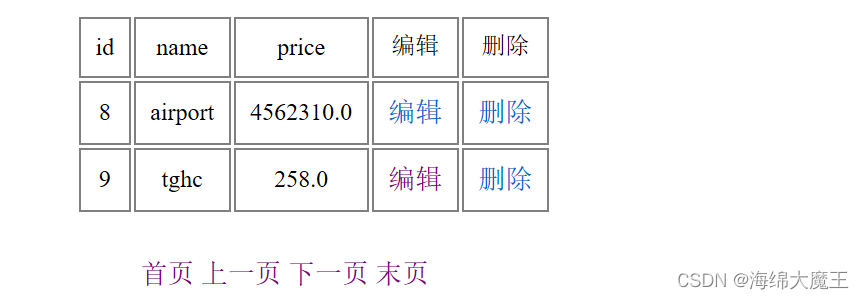
删除数据:
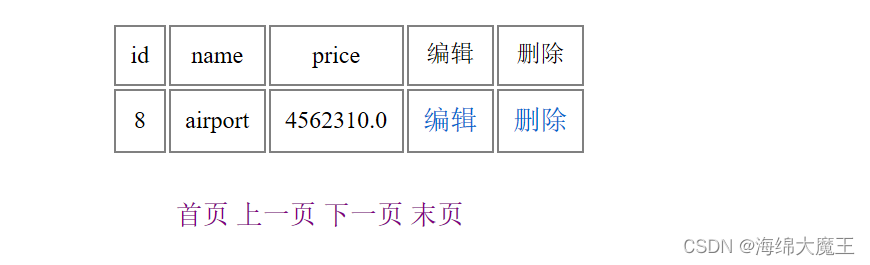
分页显示:
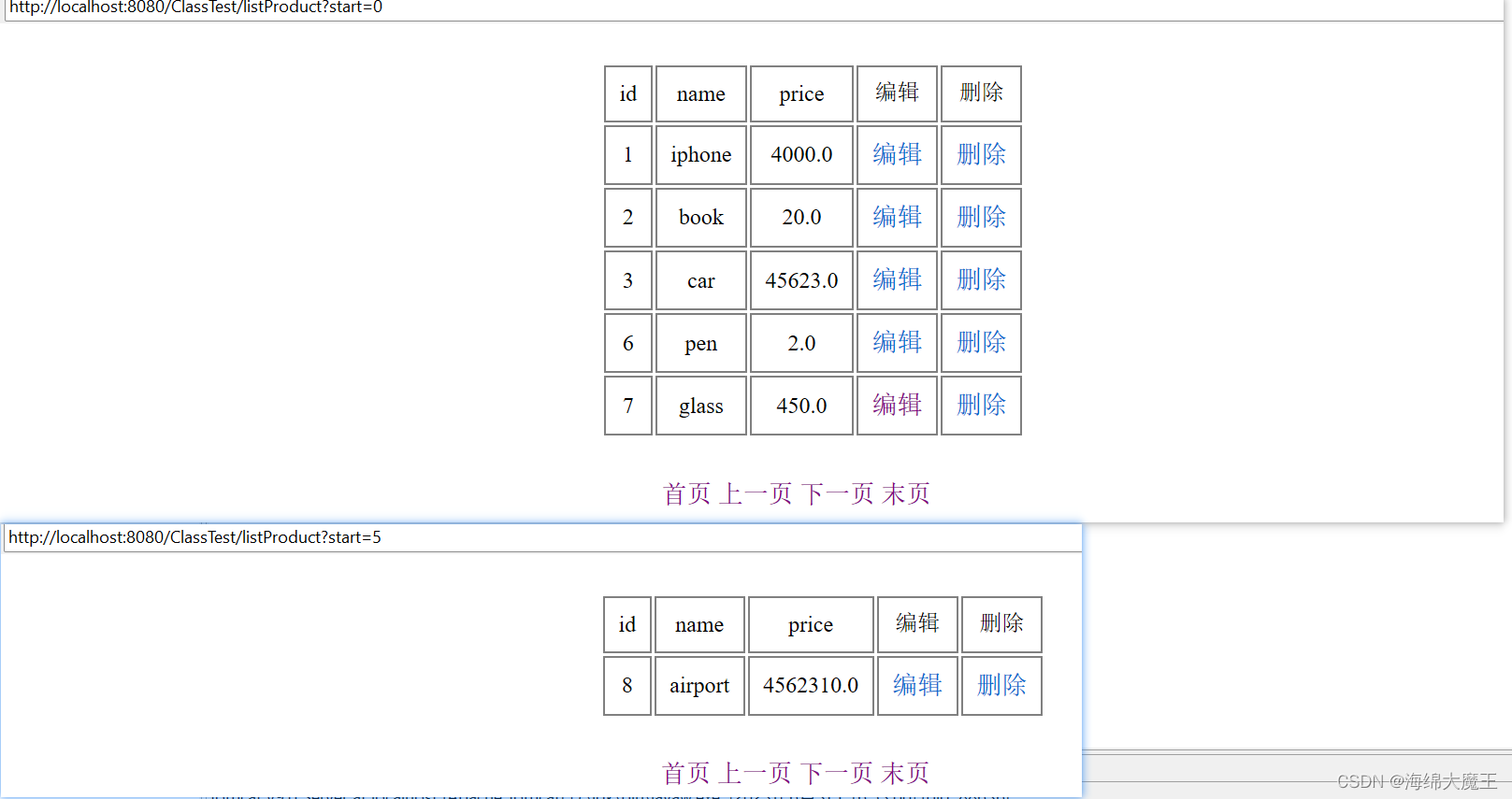