基于javaweb+mysql的springboot就业信息管理系统(java+springboot+vue+maven+mybatis+mysql)
运行环境
Java≥8、MySQL≥5.7、Node.js≥10
开发工具
后端:eclipse/idea/myeclipse/sts等均可配置运行
前端:WebStorm/VSCode/HBuilderX等均可
适用
课程设计,大作业,毕业设计,项目练习,学习演示等
功能说明
基于javaweb+mysql的SpringBoot就业信息管理系统(java+springboot+vue+maven+mybatis+mysql)
一、项目运行 环境配置:
Jdk1.8 + Tomcat8.5 + Mysql + HBuilderX(Webstorm也行)+ Eclispe(IntelliJ IDEA,Eclispe,MyEclispe,Sts都支持)。
项目技术:
Spring + SpringBoot+ mybatis + Maven + Vue 等等组成,B/S模式 + Maven管理等等。
* @email
*/
@RestController
@RequestMapping("/messages")
public class MessagesController {
@Autowired
private MessagesService messagesService;
/**
* 后端列表
*/
@RequestMapping("/page")
public R page(@RequestParam Map<String, Object> params,MessagesEntity messages, HttpServletRequest request){
EntityWrapper<MessagesEntity> ew = new EntityWrapper<MessagesEntity>();
PageUtils page = messagesService.queryPage(params, MPUtil.sort(MPUtil.between(MPUtil.likeOrEq(ew, messages), params), params));
return R.ok().put("data", page);
}
/**
* 前端列表
*/
@RequestMapping("/list")
public R list(@RequestParam Map<String, Object> params,MessagesEntity messages, HttpServletRequest request){
EntityWrapper<MessagesEntity> ew = new EntityWrapper<MessagesEntity>();
PageUtils page = messagesService.queryPage(params, MPUtil.sort(MPUtil.between(MPUtil.likeOrEq(ew, messages), params), params));
return R.ok().put("data", page);
}
/**
* 列表
*/
@RequestMapping("/lists")
public R list( MessagesEntity messages){
EntityWrapper<MessagesEntity> ew = new EntityWrapper<MessagesEntity>();
ew.allEq(MPUtil.allEQMapPre( messages, "messages"));
return R.ok().put("data", messagesService.selectListView(ew));
}
/**
* 查询
*/
@RequestMapping("/query")
public R query(MessagesEntity messages){
EntityWrapper< MessagesEntity> ew = new EntityWrapper< MessagesEntity>();
ew.allEq(MPUtil.allEQMapPre( messages, "messages"));
MessagesView messagesView = messagesService.selectView(ew);
return R.ok();
}
/**
* 前端保存
*/
@RequestMapping("/add")
public R add(@RequestBody DiscussqiuzhizhexinxiEntity discussqiuzhizhexinxi, HttpServletRequest request){
discussqiuzhizhexinxi.setId(new Date().getTime()+new Double(Math.floor(Math.random()*1000)).longValue());
//ValidatorUtils.validateEntity(discussqiuzhizhexinxi);
discussqiuzhizhexinxiService.insert(discussqiuzhizhexinxi);
return R.ok();
}
/**
* 修改
*/
@RequestMapping("/update")
public R update(@RequestBody DiscussqiuzhizhexinxiEntity discussqiuzhizhexinxi, HttpServletRequest request){
//ValidatorUtils.validateEntity(discussqiuzhizhexinxi);
discussqiuzhizhexinxiService.updateById(discussqiuzhizhexinxi);//全部更新
return R.ok();
}
/**
* 删除
*/
@RequestMapping("/delete")
public R delete(@RequestBody Long[] ids){
discussqiuzhizhexinxiService.deleteBatchIds(Arrays.asList(ids));
return R.ok();
}
/**
* 提醒接口
*/
@RequestMapping("/remind/{columnName}/{type}")
public R remindCount(@PathVariable("columnName") String columnName, HttpServletRequest request,
@PathVariable("type") String type,@RequestParam Map<String, Object> map) {
map.put("column", columnName);
map.put("type", type);
if(type.equals("2")) {
SimpleDateFormat sdf = new SimpleDateFormat("yyyy-MM-dd");
Calendar c = Calendar.getInstance();
Date remindStartDate = null;
/**
* 招聘信息评论表
* 后端接口
* @email
*/
@RestController
@RequestMapping("/discusszhaopinxinxi")
public class DiscusszhaopinxinxiController {
@Autowired
private DiscusszhaopinxinxiService discusszhaopinxinxiService;
/**
* 后端列表
*/
@RequestMapping("/page")
public R page(@RequestParam Map<String, Object> params,DiscusszhaopinxinxiEntity discusszhaopinxinxi, HttpServletRequest request){
EntityWrapper<DiscusszhaopinxinxiEntity> ew = new EntityWrapper<DiscusszhaopinxinxiEntity>();
PageUtils page = discusszhaopinxinxiService.queryPage(params, MPUtil.sort(MPUtil.between(MPUtil.likeOrEq(ew, discusszhaopinxinxi), params), params));
return R.ok().put("data", page);
}
/**
* 前端列表
*/
@IgnoreAuth
@RequestMapping("/list")
public R list(@RequestParam Map<String, Object> params,DiscusszhaopinxinxiEntity discusszhaopinxinxi, HttpServletRequest request){
EntityWrapper<DiscusszhaopinxinxiEntity> ew = new EntityWrapper<DiscusszhaopinxinxiEntity>();
PageUtils page = discusszhaopinxinxiService.queryPage(params, MPUtil.sort(MPUtil.between(MPUtil.likeOrEq(ew, discusszhaopinxinxi), params), params));
return R.ok().put("data", page);
}
/**
* 列表
*/
@RequestMapping("/lists")
public R list( DiscusszhaopinxinxiEntity discusszhaopinxinxi){
EntityWrapper<DiscusszhaopinxinxiEntity> ew = new EntityWrapper<DiscusszhaopinxinxiEntity>();
ew.allEq(MPUtil.allEQMapPre( discusszhaopinxinxi, "discusszhaopinxinxi"));
return R.ok().put("data", discusszhaopinxinxiService.selectListView(ew));
}
}
int count = discusszhaopinxinxiService.selectCount(wrapper);
return R.ok().put("count", count);
}
}
@RequestMapping("/session")
public R getCurrUser(HttpServletRequest request){
Long id = (Long)request.getSession().getAttribute("userId");
YonghuEntity user = yonghuService.selectById(id);
return R.ok().put("data", user);
}
/**
* 密码重置
*/
@IgnoreAuth
@RequestMapping(value = "/resetPass")
public R resetPass(String username, HttpServletRequest request){
YonghuEntity user = yonghuService.selectOne(new EntityWrapper<YonghuEntity>().eq("yonghuming", username));
if(user==null) {
return R.error("账号不存在");
}
user.setMima("123456");
yonghuService.updateById(user);
return R.ok("密码已重置为:123456");
}
/**
* 后端列表
*/
@RequestMapping("/page")
public R page(@RequestParam Map<String, Object> params,YonghuEntity yonghu, HttpServletRequest request){
EntityWrapper<YonghuEntity> ew = new EntityWrapper<YonghuEntity>();
PageUtils page = yonghuService.queryPage(params, MPUtil.sort(MPUtil.between(MPUtil.likeOrEq(ew, yonghu), params), params));
return R.ok().put("data", page);
}
/**
* 前端列表
*/
@RequestMapping("/list")
public R list(@RequestParam Map<String, Object> params,YonghuEntity yonghu, HttpServletRequest request){
EntityWrapper<YonghuEntity> ew = new EntityWrapper<YonghuEntity>();
PageUtils page = yonghuService.queryPage(params, MPUtil.sort(MPUtil.between(MPUtil.likeOrEq(ew, yonghu), params), params));
return R.ok().put("data", page);
}
/**
* 列表
*/
@RequestMapping("/lists")
* 友情链接
* 后端接口
* @email
*/
@RestController
@RequestMapping("/youqinglianjie")
public class YouqinglianjieController {
@Autowired
private YouqinglianjieService youqinglianjieService;
/**
* 后端列表
*/
@RequestMapping("/page")
public R page(@RequestParam Map<String, Object> params,YouqinglianjieEntity youqinglianjie, HttpServletRequest request){
EntityWrapper<YouqinglianjieEntity> ew = new EntityWrapper<YouqinglianjieEntity>();
PageUtils page = youqinglianjieService.queryPage(params, MPUtil.sort(MPUtil.between(MPUtil.likeOrEq(ew, youqinglianjie), params), params));
return R.ok().put("data", page);
}
/**
* 前端列表
*/
@IgnoreAuth
@RequestMapping("/list")
public R list(@RequestParam Map<String, Object> params,YouqinglianjieEntity youqinglianjie, HttpServletRequest request){
EntityWrapper<YouqinglianjieEntity> ew = new EntityWrapper<YouqinglianjieEntity>();
PageUtils page = youqinglianjieService.queryPage(params, MPUtil.sort(MPUtil.between(MPUtil.likeOrEq(ew, youqinglianjie), params), params));
return R.ok().put("data", page);
}
/**
* 列表
*/
@RequestMapping("/lists")
public R list( YouqinglianjieEntity youqinglianjie){
EntityWrapper<YouqinglianjieEntity> ew = new EntityWrapper<YouqinglianjieEntity>();
ew.allEq(MPUtil.allEQMapPre( youqinglianjie, "youqinglianjie"));
return R.ok().put("data", youqinglianjieService.selectListView(ew));
}
/**
* 查询
*/
@RequestMapping("/query")
public R query(YouqinglianjieEntity youqinglianjie){
EntityWrapper< YouqinglianjieEntity> ew = new EntityWrapper< YouqinglianjieEntity>();
}
/**
* 后端列表
*/
@RequestMapping("/page")
public R page(@RequestParam Map<String, Object> params,YonghuEntity yonghu, HttpServletRequest request){
EntityWrapper<YonghuEntity> ew = new EntityWrapper<YonghuEntity>();
PageUtils page = yonghuService.queryPage(params, MPUtil.sort(MPUtil.between(MPUtil.likeOrEq(ew, yonghu), params), params));
return R.ok().put("data", page);
}
/**
* 前端列表
*/
@RequestMapping("/list")
public R list(@RequestParam Map<String, Object> params,YonghuEntity yonghu, HttpServletRequest request){
EntityWrapper<YonghuEntity> ew = new EntityWrapper<YonghuEntity>();
PageUtils page = yonghuService.queryPage(params, MPUtil.sort(MPUtil.between(MPUtil.likeOrEq(ew, yonghu), params), params));
return R.ok().put("data", page);
}
/**
* 列表
*/
@RequestMapping("/lists")
public R list( YonghuEntity yonghu){
EntityWrapper<YonghuEntity> ew = new EntityWrapper<YonghuEntity>();
ew.allEq(MPUtil.allEQMapPre( yonghu, "yonghu"));
return R.ok().put("data", yonghuService.selectListView(ew));
}
/**
* 查询
*/
@RequestMapping("/query")
public R query(YonghuEntity yonghu){
EntityWrapper< YonghuEntity> ew = new EntityWrapper< YonghuEntity>();
ew.allEq(MPUtil.allEQMapPre( yonghu, "yonghu"));
YonghuView yonghuView = yonghuService.selectView(ew);
return R.ok("查询用户成功").put("data", yonghuView);
}
/**
* 后端详情
return R.ok("密码已重置为:123456");
}
/**
* 列表
*/
@RequestMapping("/page")
public R page(@RequestParam Map<String, Object> params,UserEntity user){
EntityWrapper<UserEntity> ew = new EntityWrapper<UserEntity>();
PageUtils page = userService.queryPage(params, MPUtil.sort(MPUtil.between(MPUtil.allLike(ew, user), params), params));
return R.ok().put("data", page);
}
/**
* 列表
*/
@RequestMapping("/list")
public R list( UserEntity user){
EntityWrapper<UserEntity> ew = new EntityWrapper<UserEntity>();
ew.allEq(MPUtil.allEQMapPre( user, "user"));
return R.ok().put("data", userService.selectListView(ew));
}
/**
* 信息
*/
@RequestMapping("/info/{id}")
public R info(@PathVariable("id") String id){
UserEntity user = userService.selectById(id);
return R.ok().put("data", user);
}
/**
* 获取用户的session用户信息
*/
@RequestMapping("/session")
public R getCurrUser(HttpServletRequest request){
Long id = (Long)request.getSession().getAttribute("userId");
UserEntity user = userService.selectById(id);
return R.ok().put("data", user);
if(map.get("remindend")!=null) {
Integer remindEnd = Integer.parseInt(map.get("remindend").toString());
c.setTime(new Date());
c.add(Calendar.DAY_OF_MONTH,remindEnd);
remindEndDate = c.getTime();
map.put("remindend", sdf.format(remindEndDate));
}
}
Wrapper<YingpinxinxiEntity> wrapper = new EntityWrapper<YingpinxinxiEntity>();
if(map.get("remindstart")!=null) {
wrapper.ge(columnName, map.get("remindstart"));
}
if(map.get("remindend")!=null) {
wrapper.le(columnName, map.get("remindend"));
}
String tableName = request.getSession().getAttribute("tableName").toString();
if(tableName.equals("qiyexinxi")) {
wrapper.eq("qiyebianhao", (String)request.getSession().getAttribute("username"));
}
if(tableName.equals("yonghu")) {
wrapper.eq("yonghuming", (String)request.getSession().getAttribute("username"));
}
int count = yingpinxinxiService.selectCount(wrapper);
return R.ok().put("count", count);
}
}
YingpinxinxiEntity yingpinxinxi = yingpinxinxiService.selectById(id);
return R.ok().put("data", yingpinxinxi);
}
/**
* 前端详情
*/
@RequestMapping("/detail/{id}")
public R detail(@PathVariable("id") String id){
YingpinxinxiEntity yingpinxinxi = yingpinxinxiService.selectById(id);
return R.ok().put("data", yingpinxinxi);
}
/**
* 后端保存
*/
@RequestMapping("/save")
public R save(@RequestBody YingpinxinxiEntity yingpinxinxi, HttpServletRequest request){
yingpinxinxi.setId(new Date().getTime()+new Double(Math.floor(Math.random()*1000)).longValue());
//ValidatorUtils.validateEntity(yingpinxinxi);
yingpinxinxiService.insert(yingpinxinxi);
return R.ok();
}
/**
* 前端保存
*/
@RequestMapping("/add")
public R add(@RequestBody YingpinxinxiEntity yingpinxinxi, HttpServletRequest request){
yingpinxinxi.setId(new Date().getTime()+new Double(Math.floor(Math.random()*1000)).longValue());
//ValidatorUtils.validateEntity(yingpinxinxi);
yingpinxinxiService.insert(yingpinxinxi);
return R.ok();
}
/**
* 修改
*/
@RequestMapping("/update")
public R update(@RequestBody YingpinxinxiEntity yingpinxinxi, HttpServletRequest request){
//ValidatorUtils.validateEntity(yingpinxinxi);
yingpinxinxiService.updateById(yingpinxinxi);//全部更新
return R.ok();
}
/**
* 删除
return R.ok("投票成功");
}
/**
* 后端保存
*/
@RequestMapping("/save")
public R save(@RequestBody QiuzhizhexinxiEntity qiuzhizhexinxi, HttpServletRequest request){
qiuzhizhexinxi.setId(new Date().getTime()+new Double(Math.floor(Math.random()*1000)).longValue());
//ValidatorUtils.validateEntity(qiuzhizhexinxi);
qiuzhizhexinxiService.insert(qiuzhizhexinxi);
return R.ok();
}
/**
* 前端保存
*/
@RequestMapping("/add")
public R add(@RequestBody QiuzhizhexinxiEntity qiuzhizhexinxi, HttpServletRequest request){
qiuzhizhexinxi.setId(new Date().getTime()+new Double(Math.floor(Math.random()*1000)).longValue());
//ValidatorUtils.validateEntity(qiuzhizhexinxi);
qiuzhizhexinxiService.insert(qiuzhizhexinxi);
return R.ok();
}
/**
* 修改
*/
@RequestMapping("/update")
public R update(@RequestBody QiuzhizhexinxiEntity qiuzhizhexinxi, HttpServletRequest request){
//ValidatorUtils.validateEntity(qiuzhizhexinxi);
qiuzhizhexinxiService.updateById(qiuzhizhexinxi);//全部更新
return R.ok();
}
/**
* 删除
*/
@RequestMapping("/delete")
public R delete(@RequestBody Long[] ids){
qiuzhizhexinxiService.deleteBatchIds(Arrays.asList(ids));
return R.ok();
*/
@RequestMapping("/page")
public R page(@RequestParam Map<String, Object> params,QiuzhizhexinxiEntity qiuzhizhexinxi, HttpServletRequest request){
String tableName = request.getSession().getAttribute("tableName").toString();
if(tableName.equals("yonghu")) {
qiuzhizhexinxi.setYonghuming((String)request.getSession().getAttribute("username"));
}
EntityWrapper<QiuzhizhexinxiEntity> ew = new EntityWrapper<QiuzhizhexinxiEntity>();
PageUtils page = qiuzhizhexinxiService.queryPage(params, MPUtil.sort(MPUtil.between(MPUtil.likeOrEq(ew, qiuzhizhexinxi), params), params));
return R.ok().put("data", page);
}
/**
* 前端列表
*/
@IgnoreAuth
@RequestMapping("/list")
public R list(@RequestParam Map<String, Object> params,QiuzhizhexinxiEntity qiuzhizhexinxi, HttpServletRequest request){
EntityWrapper<QiuzhizhexinxiEntity> ew = new EntityWrapper<QiuzhizhexinxiEntity>();
PageUtils page = qiuzhizhexinxiService.queryPage(params, MPUtil.sort(MPUtil.between(MPUtil.likeOrEq(ew, qiuzhizhexinxi), params), params));
return R.ok().put("data", page);
}
/**
* 列表
*/
@RequestMapping("/lists")
public R list( QiuzhizhexinxiEntity qiuzhizhexinxi){
EntityWrapper<QiuzhizhexinxiEntity> ew = new EntityWrapper<QiuzhizhexinxiEntity>();
ew.allEq(MPUtil.allEQMapPre( qiuzhizhexinxi, "qiuzhizhexinxi"));
return R.ok().put("data", qiuzhizhexinxiService.selectListView(ew));
}
/**
* 查询
*/
@RequestMapping("/query")
public R query(QiuzhizhexinxiEntity qiuzhizhexinxi){
EntityWrapper< QiuzhizhexinxiEntity> ew = new EntityWrapper< QiuzhizhexinxiEntity>();
ew.allEq(MPUtil.allEQMapPre( qiuzhizhexinxi, "qiuzhizhexinxi"));
public class MessagesController {
@Autowired
private MessagesService messagesService;
/**
* 后端列表
*/
@RequestMapping("/page")
public R page(@RequestParam Map<String, Object> params,MessagesEntity messages, HttpServletRequest request){
EntityWrapper<MessagesEntity> ew = new EntityWrapper<MessagesEntity>();
PageUtils page = messagesService.queryPage(params, MPUtil.sort(MPUtil.between(MPUtil.likeOrEq(ew, messages), params), params));
return R.ok().put("data", page);
}
/**
* 前端列表
*/
@RequestMapping("/list")
public R list(@RequestParam Map<String, Object> params,MessagesEntity messages, HttpServletRequest request){
EntityWrapper<MessagesEntity> ew = new EntityWrapper<MessagesEntity>();
PageUtils page = messagesService.queryPage(params, MPUtil.sort(MPUtil.between(MPUtil.likeOrEq(ew, messages), params), params));
return R.ok().put("data", page);
}
/**
* 列表
*/
@RequestMapping("/lists")
public R list( MessagesEntity messages){
EntityWrapper<MessagesEntity> ew = new EntityWrapper<MessagesEntity>();
ew.allEq(MPUtil.allEQMapPre( messages, "messages"));
return R.ok().put("data", messagesService.selectListView(ew));
}
/**
* 查询
*/
@RequestMapping("/query")
public R query(MessagesEntity messages){
EntityWrapper< MessagesEntity> ew = new EntityWrapper< MessagesEntity>();
ew.allEq(MPUtil.allEQMapPre( messages, "messages"));
MessagesView messagesView = messagesService.selectView(ew);
return R.ok("查询留言板成功").put("data", messagesView);
}
/**
* 后端详情
*/
@RequestMapping("/info/{id}")
/**
* 通用接口
*/
@RestController
public class CommonController{
@Autowired
private CommonService commonService;
@Autowired
private ConfigService configService;
private static AipFace client = null;
/**
* 获取table表中的column列表(联动接口)
* @param
* @param
* @return
*/
@IgnoreAuth
@RequestMapping("/option/{tableName}/{columnName}")
public R getOption(@PathVariable("tableName") String tableName, @PathVariable("columnName") String columnName,String level,String parent) {
Map<String, Object> params = new HashMap<String, Object>();
params.put("table", tableName);
params.put("column", columnName);
if(StringUtils.isNotBlank(level)) {
params.put("level", level);
}
if(StringUtils.isNotBlank(parent)) {
params.put("parent", parent);
}
List<String> data = commonService.getOption(params);
return R.ok().put("data", data);
}
/**
* 根据table中的column获取单条记录
* @param
* @param
* @return
*/
@IgnoreAuth
}
/**
* 单列求和
*/
@IgnoreAuth
@RequestMapping("/cal/{tableName}/{columnName}")
public R cal(@PathVariable("tableName") String tableName, @PathVariable("columnName") String columnName) {
Map<String, Object> params = new HashMap<String, Object>();
params.put("table", tableName);
params.put("column", columnName);
Map<String, Object> result = commonService.selectCal(params);
return R.ok().put("data", result);
}
/**
* 分组统计
*/
@IgnoreAuth
@RequestMapping("/group/{tableName}/{columnName}")
public R group(@PathVariable("tableName") String tableName, @PathVariable("columnName") String columnName) {
Map<String, Object> params = new HashMap<String, Object>();
params.put("table", tableName);
params.put("column", columnName);
List<Map<String, Object>> result = commonService.selectGroup(params);
return R.ok().put("data", result);
}
/**
* (按值统计)
*/
@IgnoreAuth
@RequestMapping("/value/{tableName}/{xColumnName}/{yColumnName}")
public R value(@PathVariable("tableName") String tableName, @PathVariable("yColumnName") String yColumnName, @PathVariable("xColumnName") String xColumnName) {
Map<String, Object> params = new HashMap<String, Object>();
params.put("table", tableName);
params.put("xColumn", xColumnName);
params.put("yColumn", yColumnName);
List<Map<String, Object>> result = commonService.selectValue(params);
return R.ok().put("data", result);
}
}
/**
* 列表
*/
@RequestMapping("/lists")
public R list( StoreupEntity storeup){
EntityWrapper<StoreupEntity> ew = new EntityWrapper<StoreupEntity>();
ew.allEq(MPUtil.allEQMapPre( storeup, "storeup"));
return R.ok().put("data", storeupService.selectListView(ew));
}
/**
* 查询
*/
@RequestMapping("/query")
public R query(StoreupEntity storeup){
EntityWrapper< StoreupEntity> ew = new EntityWrapper< StoreupEntity>();
ew.allEq(MPUtil.allEQMapPre( storeup, "storeup"));
StoreupView storeupView = storeupService.selectView(ew);
return R.ok("查询收藏表成功").put("data", storeupView);
}
/**
* 后端详情
*/
@RequestMapping("/info/{id}")
public R info(@PathVariable("id") Long id){
StoreupEntity storeup = storeupService.selectById(id);
return R.ok().put("data", storeup);
}
/**
* 前端详情
*/
@RequestMapping("/detail/{id}")
public R detail(@PathVariable("id") Long id){
StoreupEntity storeup = storeupService.selectById(id);
return R.ok().put("data", storeup);
}
/**
* 后端保存
/**
* 留言板
* 后端接口
* @email
*/
@RestController
@RequestMapping("/messages")
public class MessagesController {
@Autowired
private MessagesService messagesService;
/**
* 后端列表
*/
@RequestMapping("/page")
public R page(@RequestParam Map<String, Object> params,MessagesEntity messages, HttpServletRequest request){
EntityWrapper<MessagesEntity> ew = new EntityWrapper<MessagesEntity>();
PageUtils page = messagesService.queryPage(params, MPUtil.sort(MPUtil.between(MPUtil.likeOrEq(ew, messages), params), params));
return R.ok().put("data", page);
}
/**
* 前端列表
*/
@RequestMapping("/list")
public R list(@RequestParam Map<String, Object> params,MessagesEntity messages, HttpServletRequest request){
EntityWrapper<MessagesEntity> ew = new EntityWrapper<MessagesEntity>();
PageUtils page = messagesService.queryPage(params, MPUtil.sort(MPUtil.between(MPUtil.likeOrEq(ew, messages), params), params));
return R.ok().put("data", page);
}
/**
* 列表
*/
@RequestMapping("/lists")
public R list( MessagesEntity messages){
EntityWrapper<MessagesEntity> ew = new EntityWrapper<MessagesEntity>();
ew.allEq(MPUtil.allEQMapPre( messages, "messages"));
return R.ok().put("data", messagesService.selectListView(ew));
}
/**
@RequestMapping("/save")
public R save(@RequestBody QiyexinxiEntity qiyexinxi, HttpServletRequest request){
qiyexinxi.setId(new Date().getTime()+new Double(Math.floor(Math.random()*1000)).longValue());
//ValidatorUtils.validateEntity(qiyexinxi);
QiyexinxiEntity user = qiyexinxiService.selectOne(new EntityWrapper<QiyexinxiEntity>().eq("qiyebianhao", qiyexinxi.getQiyebianhao()));
if(user!=null) {
return R.error("用户已存在");
}
qiyexinxi.setId(new Date().getTime());
qiyexinxiService.insert(qiyexinxi);
return R.ok();
}
/**
* 前端保存
*/
@RequestMapping("/add")
public R add(@RequestBody QiyexinxiEntity qiyexinxi, HttpServletRequest request){
qiyexinxi.setId(new Date().getTime()+new Double(Math.floor(Math.random()*1000)).longValue());
//ValidatorUtils.validateEntity(qiyexinxi);
QiyexinxiEntity user = qiyexinxiService.selectOne(new EntityWrapper<QiyexinxiEntity>().eq("qiyebianhao", qiyexinxi.getQiyebianhao()));
if(user!=null) {
return R.error("用户已存在");
}
qiyexinxi.setId(new Date().getTime());
qiyexinxiService.insert(qiyexinxi);
return R.ok();
}
/**
* 修改
*/
@RequestMapping("/update")
public R update(@RequestBody QiyexinxiEntity qiyexinxi, HttpServletRequest request){
//ValidatorUtils.validateEntity(qiyexinxi);
qiyexinxiService.updateById(qiyexinxi);//全部更新
return R.ok();
}
/**
* 删除
*/
@RequestMapping("/delete")
public R delete(@RequestBody Long[] ids){
qiyexinxiService.deleteBatchIds(Arrays.asList(ids));
return R.ok();
}
/**
* 提醒接口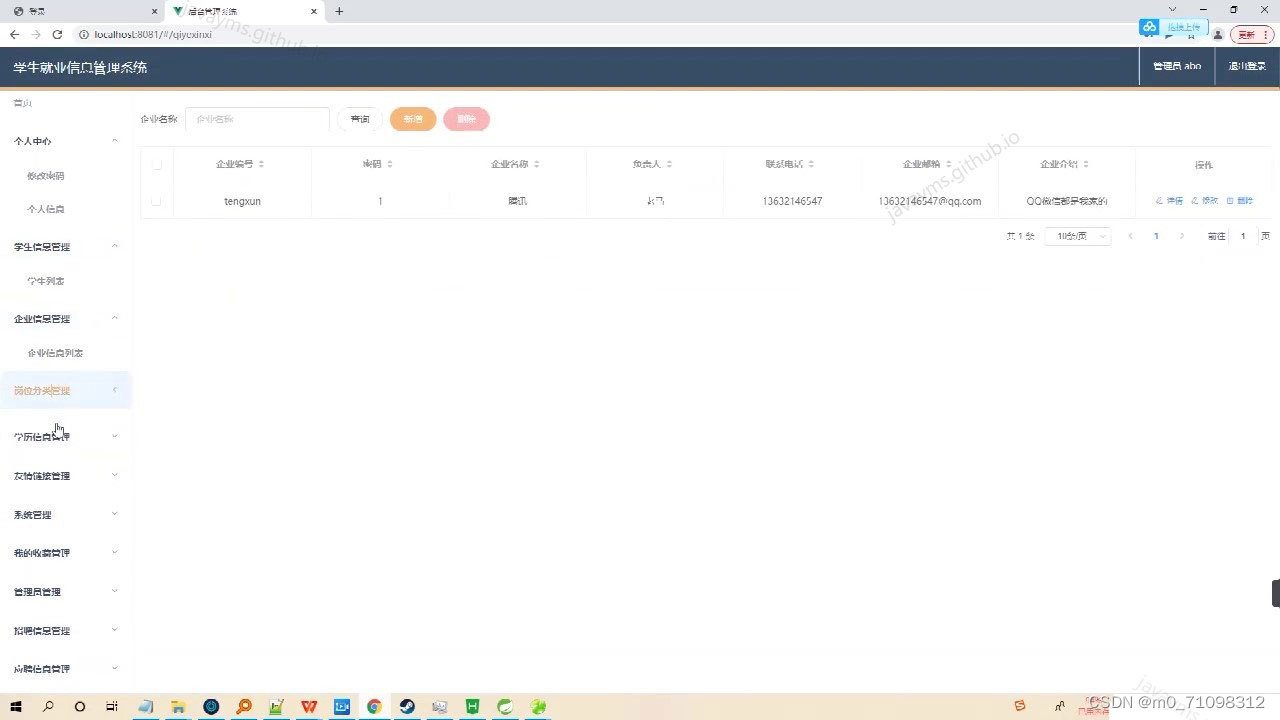