目录
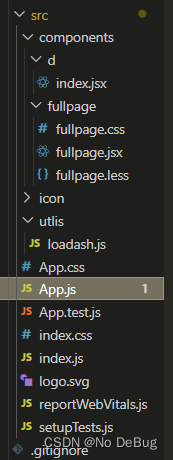
index.jsx
import React from "react";
import { Fullpage, FullpageItem } from "../fullpage/fullpage";
class LoginPage extends React.Component {
constructor(props) {
super(props);
this.state = {};
}
render() {
return (
<div className="box_container">
<Fullpage>
<FullpageItem>
<div>
<img src={require("../.././icon/国旗杆.webp")} alt="" />
</div>
</FullpageItem>
<FullpageItem>
<div>
<img src={require("../.././icon/壁纸.jpg")} alt="" />
</div>
</FullpageItem>
<FullpageItem>
<div>
<img src={require("../.././icon/青铜鼎.jpg")} alt="" />
</div>
</FullpageItem>
<FullpageItem>
<div>
<img src={require("../.././icon/天安门.webp")} alt="" />
</div>
</FullpageItem>
<FullpageItem>
<div>
<img src={require("../.././icon/国旗.webp")} alt="" />
</div>
</FullpageItem>
<FullpageItem>
<div>
<img src={require("../.././icon/勿忘国耻.webp")} alt="" />
</div>
</FullpageItem>
</Fullpage>
</div>
);
}
}
export default LoginPage
fullpage.jsx
import React from "react";
import "./fullpage.css";
import { throttle } from "../../utlis/loadash";
class Fullpage extends React.Component {
constructor(props) {
super(props);
this.state = {
currentPage: 1,
pageList: this.props.children,
};
this.initPageListFromPropsChildren(); // 类似于vue的插槽,props带过来的children(FullpageItem)
this.handleScroll = throttle(this.handleScroll, 600, true); //屏幕滚动时的节流函数
}
debounceHandleScroll = (e) => {
e.persist();
this.handleScroll(e);
};
changeCurrentPage(index) {
this.setState({ currentPage: index });
}
handleScroll(e) {
if (e.deltaY > 0) {
if (this.state.currentPage === this.state.pageList.length) return;
this.changeCurrentPage(this.state.currentPage + 1);
} else {
if (this.state.currentPage === 1) return;
this.changeCurrentPage(this.state.currentPage - 1);
}
}
initPageListFromPropsChildren() {
// 初始化pageList(插槽)
if (this.state.pageList === undefined) this.setState({ pageList: [] });
if (
typeof pageList === "object" &&
this.state.pageList.length === undefined
)
this.setState({ pageList: [this.state.pageList] });
}
render() {
return (
<div className="fullpage_container" onWheel={this.debounceHandleScroll}>
{this.state.pageList.map((x, i) => (
<div
className={`fullpage_item item_${i + 1}`}
key={i}
style={{
top: -this.state.currentPage + i + 1 + "00vh",
}}
>
{x}
</div>
))}
<div className="point_nav">
{this.state.pageList.map((x, i) => (
<div
className="point_nav_item"
style={{
background:
i + 1 === this.state.currentPage ? "#333" : "#cccaca",
}}
key={i}
onMouseEnter={() => this.changeCurrentPage(i + 1)}
></div>
))}
</div>
</div>
);
}
}
const FullpageItem = (props) => {
let { children } = props;
return <div style={{ height: "100%", width: "100%" }}>{children}</div>;
};
export { Fullpage, FullpageItem };
fullpage.css
.fullpage_container {
width: 100%;
height: 100vh;
overflow: hidden;
position: relative;
}
.fullpage_container .fullpage_item {
width: 100%;
height: 100vh;
position: absolute;
transition: top 500ms;
}
img {
width: 100%;
height: 100vh;
}
.point_nav {
position: fixed;
right: 20px;
top: 50%;
transform: translateY(-50%);
z-index: 100;
}
.point_nav .point_nav_item {
width: 10px;
height: 10px;
border-radius: 50%;
margin-bottom: 10px;
transition: background 0.5s;
}
loadash.js
export function throttle(func, wait, immediate) {
let previous = 0;
let timeout;
return function (...args) {
if (immediate) {
let now = Date.now();
if (now - previous > wait) {
func.apply(this, args);
previous = now;
}
} else {
if (!timeout) {
timeout = setTimeout(() => {
timeout = null;
func.apply(this, args);
}, wait);
}
}
};
}