//子类
package com.alibaba.demo06;
public class BasePlusSalesEmployee extends SalesEmployee{
private int dixin;
public BasePlusSalesEmployee(int yueXiaoShouE, double tiChengLv, int dixin) {
super(yueXiaoShouE, tiChengLv);
this.dixin = dixin;
}
public BasePlusSalesEmployee(String name, int month, int yueXiaoShouE, double tiChengLv, int dixin) {
super(name, month, yueXiaoShouE, tiChengLv);
this.dixin = dixin;
}
public int getDixin() {
return dixin;
}
public void setDixin(int dixin) {
this.dixin = dixin;
}
public int getSalary(int month) {
if (month == getMonth()){
double v = getDixin() + (getYueXiaoShouE() * getTiChengLv());
return (int) (v+100);
}else {
double v = getDixin() + (getYueXiaoShouE() * getTiChengLv());
return (int) v;
}
}
}
//父类
package com.alibaba.demo06;
import java.util.Date;
public class Employee {
private String name;
private int month;
public Employee() {
}
public Employee(String name, int month) {
this.name = name;
this.month = month;
}
public String getName() {
return name;
}
public int getMonth() {
return month;
}
public void setName(String name) {
this.name = name;
}
public void setMonth(int month) {
this.month = month;
}
public int getSalary(int month){
return 0;
}
}
//子类
package com.alibaba.demo06;
public class HourlyEmployee extends Employee{
private int meiX;
private int meiY;
public HourlyEmployee(int meiX, int meiY) {
this.meiX = meiX;
this.meiY = meiY;
}
public HourlyEmployee(String name, int month, int meiX, int meiY) {
super(name, month);
this.meiX = meiX;
this.meiY = meiY;
}
public int getMeiX() {
return meiX;
}
public int getMeiY() {
return meiY;
}
public void setMeiX(int meiX) {
this.meiX = meiX;
}
public void setMeiY(int meiY) {
this.meiY = meiY;
}
public int getSalary(int month) {
if (getMeiY() > 160) {
if (month == getMonth()) {
double v = (getMeiY() - 160) * (1.5 * getMeiX());
int i = getMeiX() * 160;
return (int) (v + i + 100);
}else {
double v = (getMeiY() - 160) * (1.5 * getMeiX());
int i = getMeiX() * 160;
return (int) (v + i);
}
} else {
if (month == getMonth()) {
int i = (getMeiX() * getMeiY()) + 100;
return i;
} else {
int i = getMeiX() * getMeiY();
return i;
}
}
}
}
//子类
package com.alibaba.demo06;
import java.util.Date;
public class SalariedEmployee extends Employee { //拿固定工资的员工
private int yueXin;
public SalariedEmployee(int yueYin) {
this.yueXin = yueYin;
}
public SalariedEmployee(String name, int month, int yueXin) {
super(name, month);
this.yueXin = yueXin;
}
public int getYueXin() {
return yueXin;
}
public void setYueXin(int yueXin) {
this.yueXin = yueXin;
}
public int getSalary(int month) {
if (month == getMonth()) {
return getYueXin() + 100;
} else {
return getYueXin();
}
}
}
//子类
package com.alibaba.demo06;
public class SalesEmployee extends Employee {
private int yueXiaoShouE;
private double tiChengLv;
public SalesEmployee(int yueXiaoShouE, double tiChengLv) {
this.yueXiaoShouE = yueXiaoShouE;
this.tiChengLv = tiChengLv;
}
public SalesEmployee(String name, int month, int yueXiaoShouE, double tiChengLv) {
super(name, month);
this.yueXiaoShouE = yueXiaoShouE;
this.tiChengLv = tiChengLv;
}
public int getYueXiaoShouE() {
return yueXiaoShouE;
}
public void setYueXiaoShouE(int yueXiaoShouE) {
this.yueXiaoShouE = yueXiaoShouE;
}
public double getTiChengLv() {
return tiChengLv;
}
public void setTiChengLv(double tiChengLv) {
this.tiChengLv = tiChengLv;
}
public int getSalary(int month) {
if (month == getMonth()) {
double v = (getYueXiaoShouE() * getTiChengLv());
int v1 = (int) v + 100;
return v1;
} else {
double v = (getYueXiaoShouE() * getTiChengLv());
return (int) v;
}
}
}
//测试 运行结果用主方法main
package com.alibaba.demo06;
public class Test {
public static void main(String[] args) {
Employee salariedEmployee = new SalariedEmployee("小高",10,10000);
Employee hourlyEmployee = new HourlyEmployee("小刘",10,10,180);
Employee salesEmployee = new SalesEmployee("腊肠",6,20000,4);
Employee basePlusSalesEmployee = new BasePlusSalesEmployee("小艾",5,10000,2,300);
int salary = salariedEmployee.getSalary(10);
System.out.println(salariedEmployee.getName()+""+salary);
int salary1 = hourlyEmployee.getSalary(10);
System.out.println(hourlyEmployee.getName()+"的月薪是:" + salary1);
int salary2 = salesEmployee.getSalary(6);
System.out.println(salesEmployee.getName()+"的月薪是:" + salary2);
int salary3 = basePlusSalesEmployee.getSalary(5);
System.out.println(basePlusSalesEmployee.getName()+"的月薪是:" + salary3);
}
}
【无标题】
最新推荐文章于 2024-08-15 14:09:45 发布
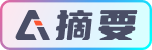