目录
上篇已经熟悉了ArrayList的使用,ArrayList底层使用数组来存储元素。由于其底层是一段连续空间,当在ArrayList任意位置插入或者删除元素时,就需要将后序元素整体往前或者往后搬移,时间复杂度为O(n),效率比较低,因此ArrayList不适合做任意位置插入和删除比较多的场景。
因此,java集合中又引入了LinkedList,即链表结构。
链表
1、链表的概念及结构
链表
是一种
物理存储结构上非连续
存储结构,数据元素的
逻辑顺序是通过链表中的引用链接次序实现的 。
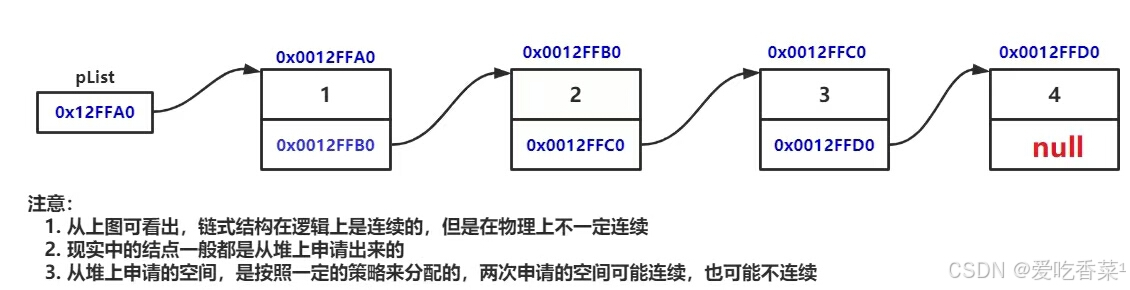
实际中链表的结构非常多样,以下情况组合起来就有
8
种链表结构:
链表大分类
- 单向或者双向
- 带头或者不带头
- 循环或者非循环
虽然有这么多的链表的结构,但是我们重点掌握两种
:
无头单向非循环链表
:
结构简单
,一般不会单独用来存数据。实际中更多是作为
其他数据结构的子结构
,如 哈希桶、图的邻接表等等。另外这种结构在笔试面试
中出现很多。
无头双向链表
:在
Java
的集合框架库中
LinkedList
底层实现就是无头双向循环链表。
2、LinkedList的使用
2、1 什么是LinkedList
LinkedList的底层是双向链表结构(
链表后面介绍
)
,由于链表没有将元素存储在连续的空间中,元素存储在单独的节 点中,然后通过引用将节点连接起来了,因此在在任意位置插入或者删除元素时,不需要搬移元素,效率比较高。
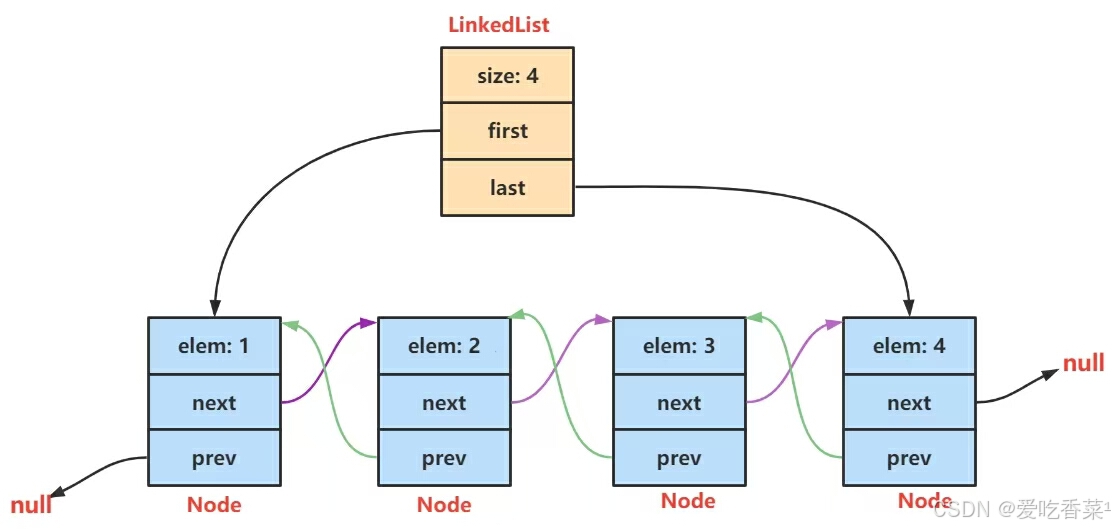
在集合框架中,LinkedList也实现了List接口,具体如下:
【说明】
- LinkedList实现了List接口
- LinkedList的底层使用了双向链表
- LinkedList没有实现RandomAccess接口,因此LinkedList不支持随机访问
- LinkedList的任意位置插入和删除元素时效率比较高,时间复杂度为O(1)
- LinkedList比较适合任意位置插入的场景
2、2 LinkedList的使用
LinkedList 的构造
方法 | 解释 |
LinkedList ( ) | 无参构造 |
Public LinkedList ( Collection<? extends E> c ) |
使用其他集合容器中元素构造List
|
public static void main ( String [] args ) {// 构造一个空的 LinkedListList < Integer > list1 = new LinkedList <> ();List < String > list2 = new java . util . ArrayList <> ();list2 . add ( "JavaSE" );list2 . add ( "JavaWeb" );list2 . add ( "JavaEE" );// 使用 ArrayList 构造 LinkedListList < String > list3 = new LinkedList <> ( list2 );}
LinkedList常用方法
方法 | 解释 |
boolean
add
(E e)
| 尾插 e |
void add(int index, E element) | 将 e 插入到 index 位置 |
boolean addAll(Collection<? extends E> c) | 尾插 c 中的元素 |
E
remove
(int index)
| 删除 index 位置元素 |
boolean remove(Object o) | 删除遇到的第一个 o |
E get(int index) | 获取下标 index 位置元素 |
E set(int index, E element) | 将下标 index 位置元素设置为element |
void clear() | 清空 |
boolean contains(Object o) | 判断 o 是否在线性表中 |
int indexOf(Object o) |
返回第一个 o
所在下标
|
int lastIndexOf(Obeject o) | 返回最后一个o 所在下标 |
List<E> subList(int fromIndex, int toIndex) | 截取部分 list |
以上就是 LinkedList常用方法 ,需要多练熟悉用法
3、LinkedList的遍历
LinkedList
的遍历有三种;for循环
+
下标、
foreach
、使用迭代器
LinkedList<Integer> list1=new LinkedList<>();
list1.add(1);
list1.add(2);
list1.add(3);
list1.add(4);
1、for循环遍历
for (int i = 0; i <list1.size() ; i++) {
System.out.print(list1.get(i)+" ");
}
System.out.println(" ");
2、foreach遍历
for (Integer i:list1){
System.out.print(i+" ");
}
System.out.println(" ");
3、使用迭代器遍历---正向遍历
ListIterator<Integer> it= list1.listIterator();
while(it.hasNext())
System.out.print(it.next()+" ");
System.out.println(" ");
4、使用反向迭代器---反向遍历
ListIterator<Integer> rit= list1.listIterator(4);
while(rit.hasPrevious())
System.out.print(rit.previous()+" ");
System.out.println(" ");
}
4、LinkedList的模拟实现
模拟实现 LinkedList链表。
public class MyLinkedList {
static class ListNode{
public int val;
public ListNode next;
public ListNode prev;
public ListNode(int val) {
this.val = val;
}
}
public ListNode head;
public ListNode last;
//头插法
public void addFirst(int data){
ListNode node=new ListNode(data);
if(head==null) {
head = last = node;
return;
}
node.next=head;
head.prev=node;
head=node;
}
//尾插法
public void addLast(int data){
ListNode node=new ListNode(data);
if(head==null) {
head = last = node;
return;
}
last=head;
while(last.next!=null){
last=last.next;
}
last.next=node;
node.prev=last;
last=last.next;
}
//任意位置插入,第一个数据节点为0号下标
public boolean addIndex(int index,int data){
int len=size();
if(index<0||index>len) {
System.out.println("index位置不合法");
return false;
}
if(index==0) {
addFirst(data);
return true;
}
if(index==len) {
addLast(data);
return true;
}
ListNode cur=findnode(index);
ListNode node=new ListNode(data);
node.next=cur;
cur.prev.next=node;
node.prev=cur.prev;
cur.prev=node;
return true;
}
private ListNode findnode(int index){
ListNode cur=head;
while(index!=0){
index--;
cur=cur.next;
}
return cur;
}
//查找是否包含关键字key是否在单链表当中
public boolean contains(int key){
ListNode cur=head;
while(cur!=null){
if(cur.val==key)
return true;
cur=cur.next;
}
return false;
}
//删除第一次出现关键字为key的节点
public void remove(int key){
ListNode cur=head;
while(cur!=null){
if(cur.val==key){
if(cur==head){
head=head.next;
if(head==null)
return;
}else {
cur.prev.next=cur.next;
if(cur.next==null){
last=last.prev;
}else{
cur.next.prev=cur.prev;
}
}
return;
}
cur=cur.next;
}
}
//删除所有值为key的节点
public void removeAllKey(int key){
ListNode cur=head;
while(cur!=null){
if(cur.val==key){
if(cur==head){
head=head.next;
if(head!=null)
head.prev=null;
}else{
cur.prev.next=cur.next;
if(cur.next==null){
last=last.prev;
}else{
cur.next.prev=cur.prev;
}
}
}
cur=cur.next;
}
}
//得到单链表的长度
public int size(){
int count=0;
ListNode cur=head;
if(head==null)
return 0;
while(cur!=null){
count++;
cur=cur.next;
}
return count;
}
public void display(){
ListNode cur=head;
if(head==null)
return;
while(cur!=null){
System.out.print(cur.val+" ");
cur=cur.next;
}
System.out.println(" ");
}
public void clear(){
ListNode cur=head,curN=head;
while(cur!=null){
curN=cur.next;
cur.next=null;cur.prev=null;
cur=curN;
}
head=last=null;
}
}
5、ArrayList和LinkedList的区别
不同点 | ArrayList | LinkedList |
存储空间上 | 物理上一定连续 | 逻辑上连续,物理上不一定连续 |
随机访问 | 支持O(1) | 不支持 O ( n ) |
头插 | 需要搬移元素,效率低 O( n ) | 只需修改元素指向,时间复杂度 O(1) |
插入 | 空间不够可以扩容 | 没有容量概念 |
应用场景 | 元素高效存储+频繁访问 | 任意位置插入和频繁删除 |
【数据结构】LinkedList与链表学习到这里