Othello UVA - 220
问题
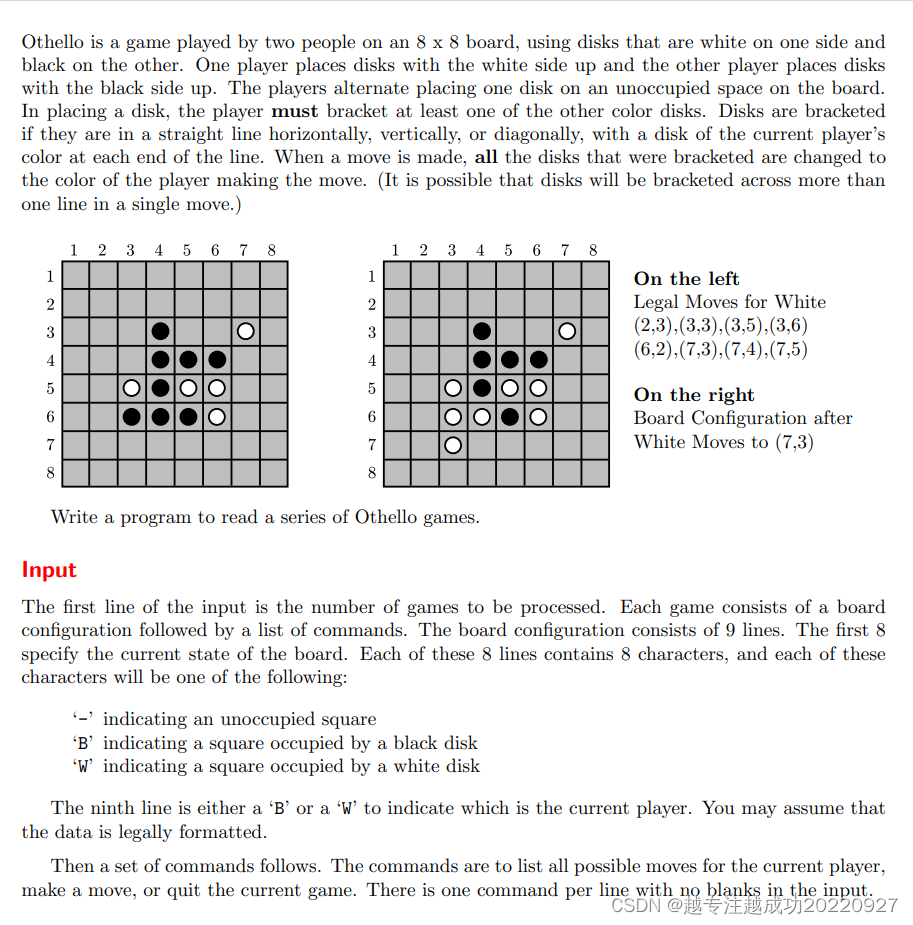
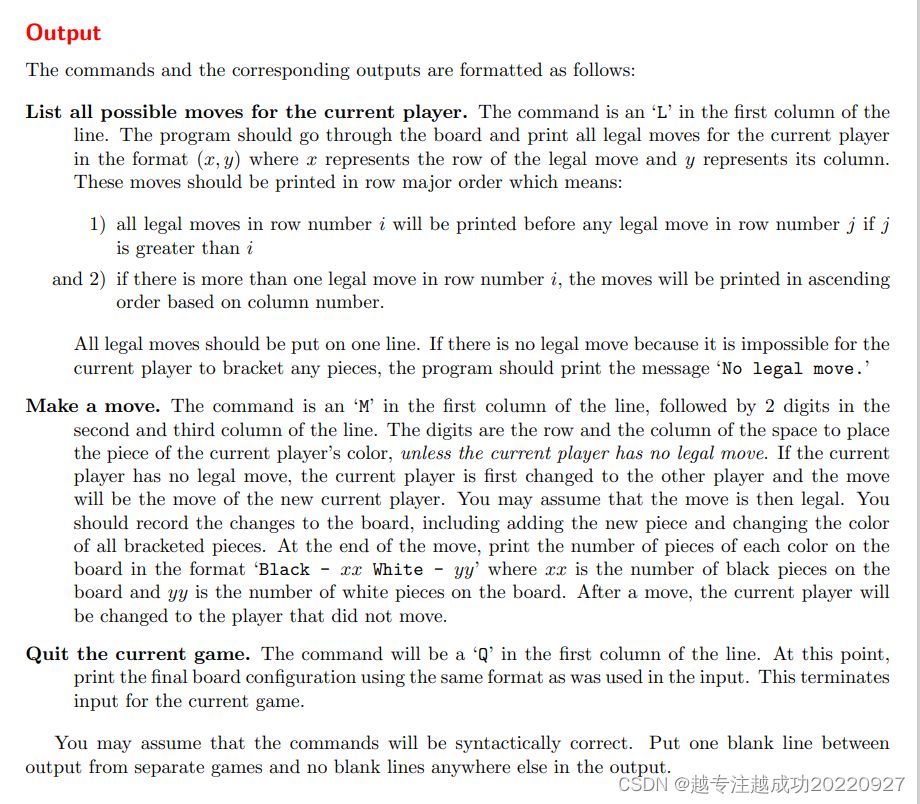
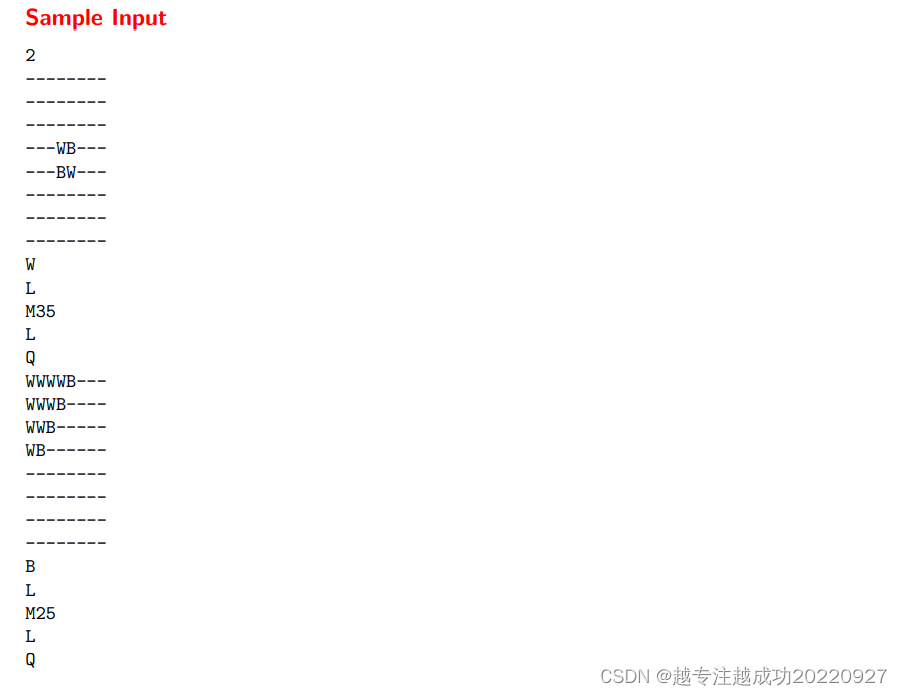
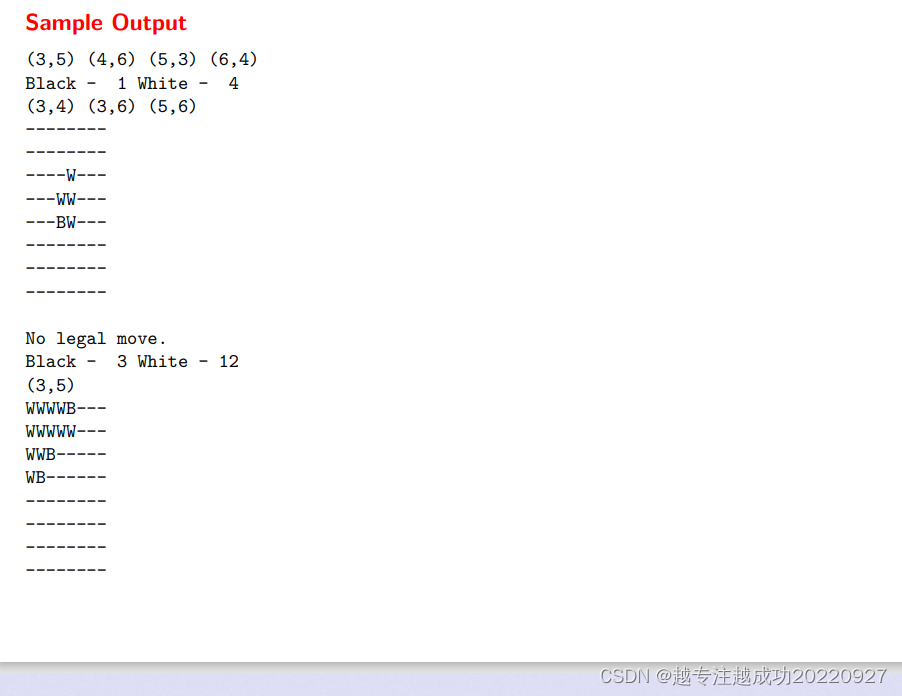
代码
#include <iostream>
#include <vector>
#include <cassert>
#include <cstring>
#include <iomanip>
using namespace std;
bool inRange(int x, int left, int right) {
if(left > right) return inRange(x, right, left);
return left <= x && x <= right;
}
struct Point {
int x, y;
Point(int x = 0, int y = 0) : x(x), y(y) {
}
};
ostream & operator << (ostream &os, const Point &p) {
return os << "(" << p.x << "," << p.y << ")"; }
template<typename T>
ostream & operator << (ostream &os, const vector<T> &v) {
for(int i = 0; i < v.size(); i++) {
if(i) os << ' ';
os << v[i];
}
return os;
}
typedef Point Vector;
Vector operator + (const Vector &A, const Vector &B) {
return Vector(A.x + B.x, A.y + B.y); }
Vector operator - (const Vector &A, const Vector &B) {
return Vector(A.x - B.x, A.y - B.y); }
Vector operator * (const Vector &A