一、简单习题部分
1.1简单判断一个质数
import java.util.Scanner;
public class panduanzhishu {
public static void main(String[] args) {
Scanner sc = new Scanner(System.in);
System.out.println("请输入一个正整数");
int num = sc.nextInt();
boolean flag = true;
for(int i = 2;i < num / 2;i++){
if (num % i == 0){
flag = false;
break;
}
}
if(flag){
System.out.println(num + "是一个质数");
}
else{
System.out.println(num + "不是质数");
}
}
}
1.2判断数字回文
import java.util.Scanner;
public class sumhuiwen {
public static void main(String[] args) {
Scanner sc = new Scanner(System.in);
System.out.println("请输入数字");
int num = sc.nextInt();
int sum = 0;
int temp = num;
while (num != 0)
{
int ge = num%10;
num = num / 10;
sum = sum*10+ge;
}
System.out.println(sum);
if(temp == sum)
{
System.out.println(true);
}
else{
System.out.println(false);
}
}
}
1.3生成随机数,并猜大小
Random用法
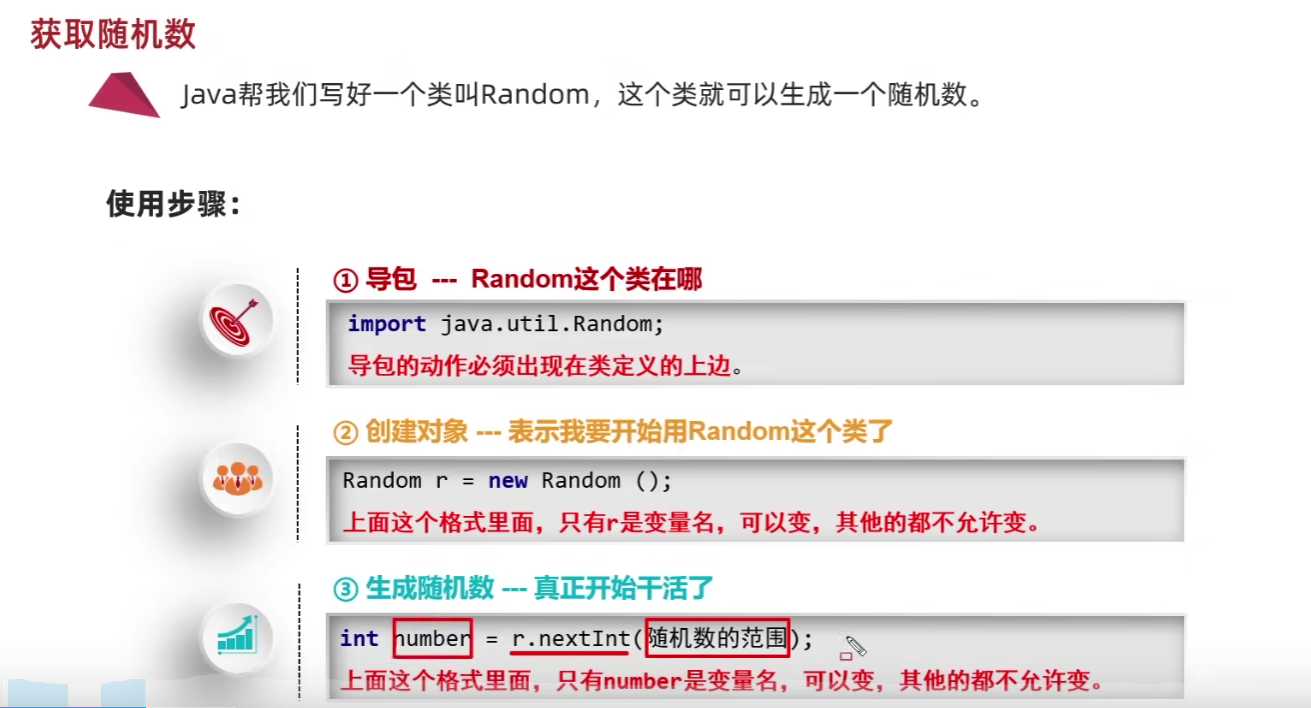
题目如下

import java.util.Random;
import java.util.Scanner;
public class suijishu {
public static void main(String[] args) {
//生成一个1~100的随机数
Random r = new Random();
int num = r.nextInt(100)+1;
//判断大小
while(true){
//猜这个数字是多少
Scanner sc =new Scanner(System.in);
System.out.println("请输入你要猜的数字");
int guessnum = sc.nextInt();
if(guessnum > num){
System.out.println("猜大了");
}
else if (guessnum < num){
System.out.println("猜小了");
}
else{
System.out.println("猜中了");
break;
}
}
}
}
二、动态及静态数组
2.1静态数组的几种初始化方式
静态数组其余功能与C类似,不再做太多说明。
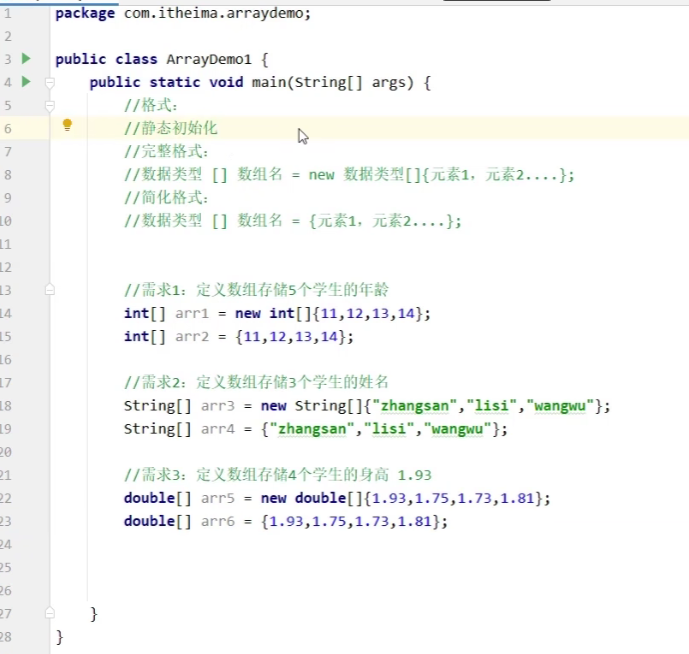
2.2静态数组遍历快捷方式
(数组名.length) 或者 (数组名.fori)
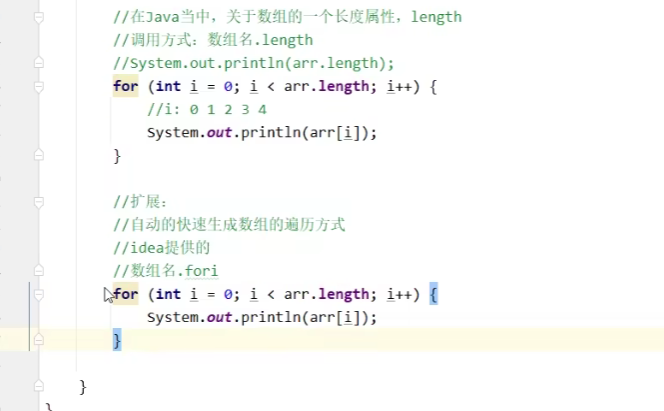
2.3动态数组初始化
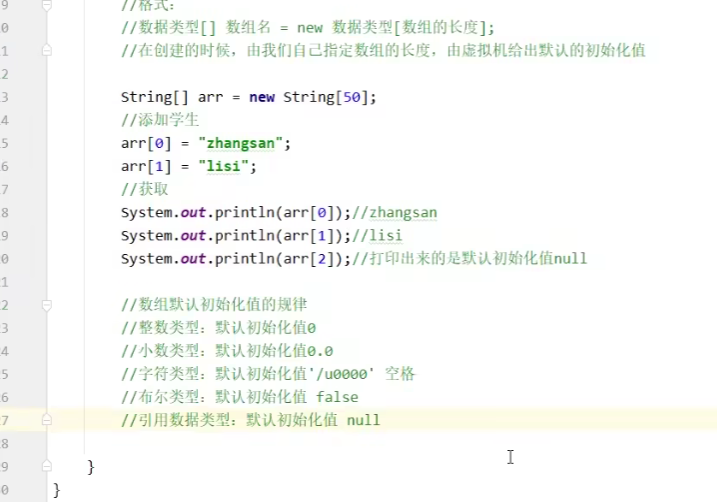
运行结果如下
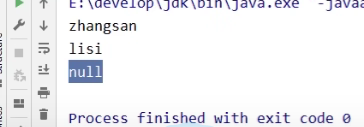
2.4求数组元素最大值
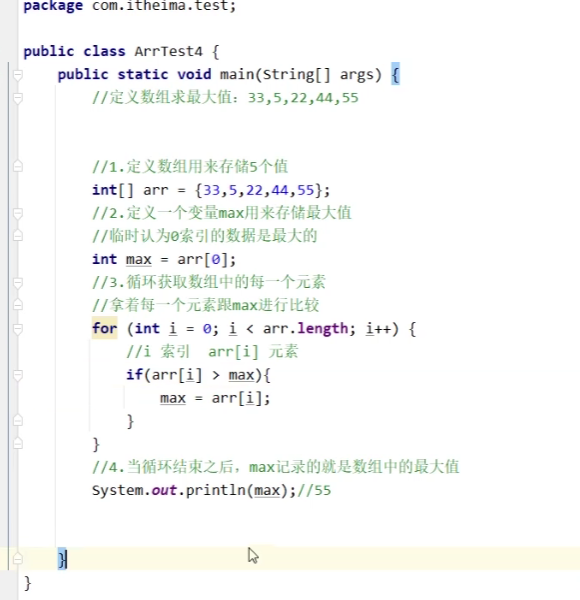
2.5将数组中的元素全部倒过来
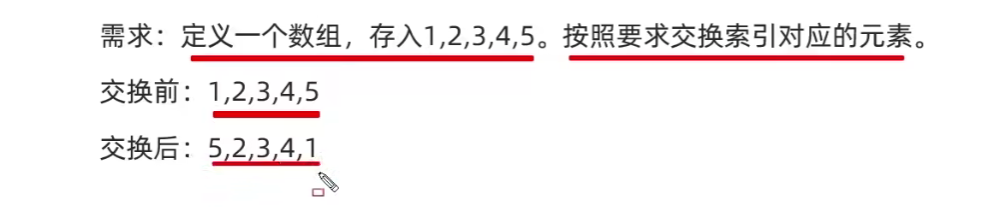
public class jiaohuanshuju {
public static void main(String[] args) {
int arr[] = {1,2,3,4,5};
for (int i = 0,j=arr.length-1; i < j; i++,j--) {
int temp = arr[i];
arr[i] = arr[j];
arr[j] = temp;
}
for (int i = 0; i < arr.length; i++) {
System.out.print(arr[i] + " ");
}
}
}
2.6将数组元素全部打乱
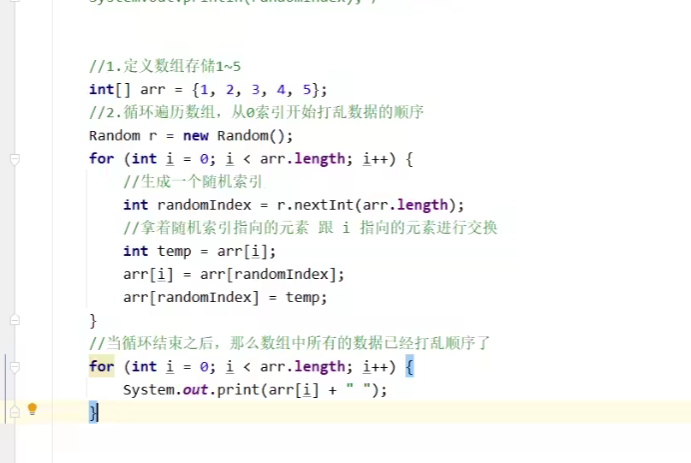
mport java.lang.reflect.Array;
import java.util.Random;
public class jingtaishuzu {
public static void main(String[] args) {
int []array={1,2,3,4,5};
Random r = new Random();
int ppsuc = r.nextInt(array.length);
for (int i = 0; i < array.length; i++) {
int temp = array[i];
array[i] = array[ppsuc];
array[ppsuc] = temp;
}
for (int i = 0; i < array.length; i++) {
System.out.print(array[i]+" ");
}
}
}
2.7数组内存的存储方式
2.7.1第一种情况
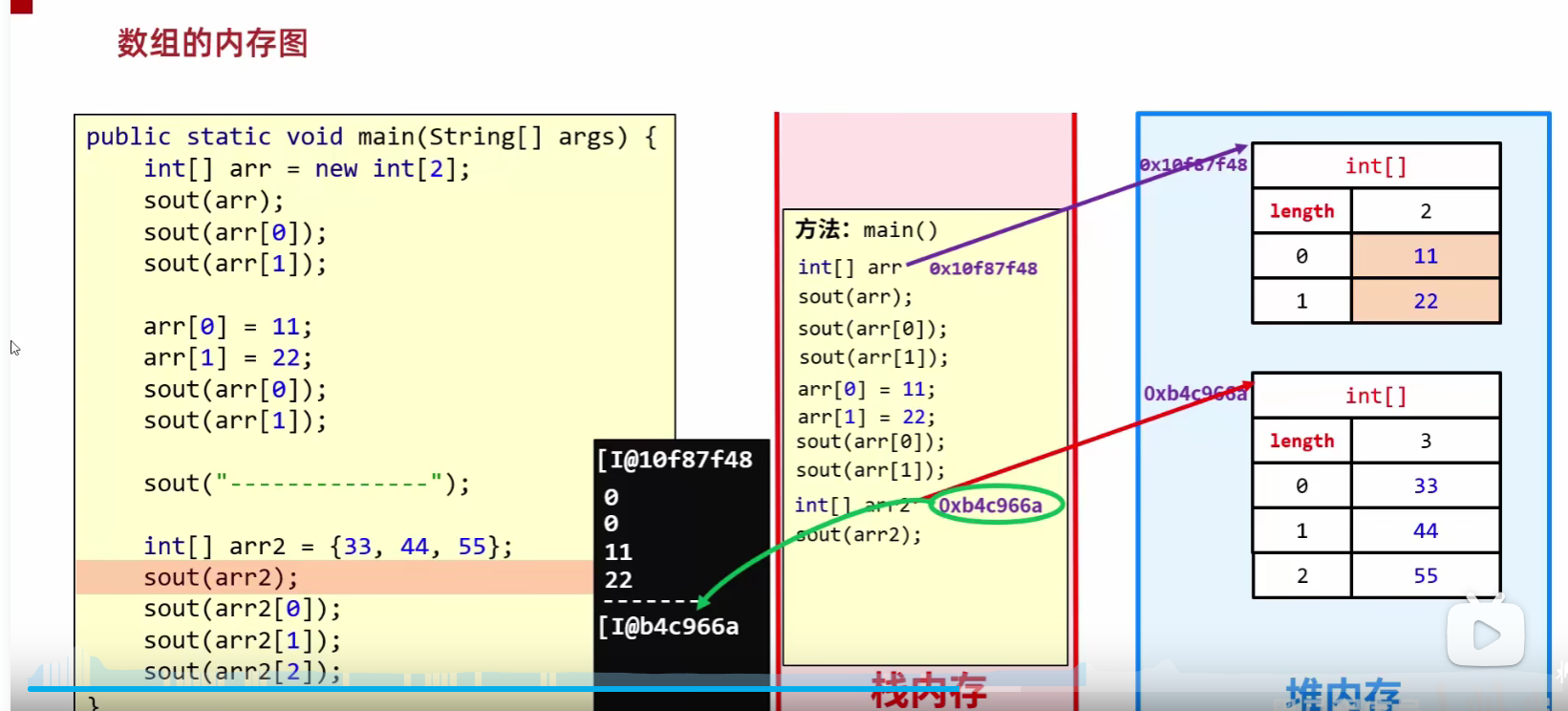
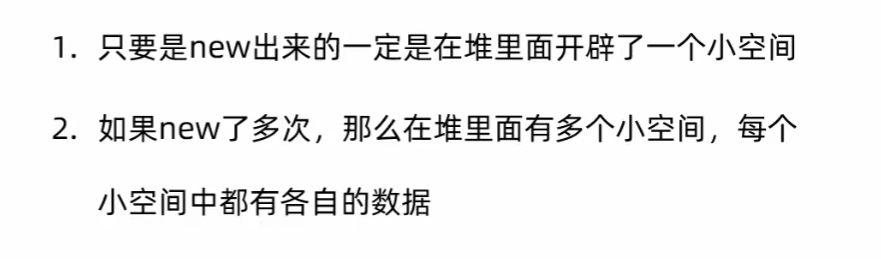
2.7.2第二种情况(共享数组)
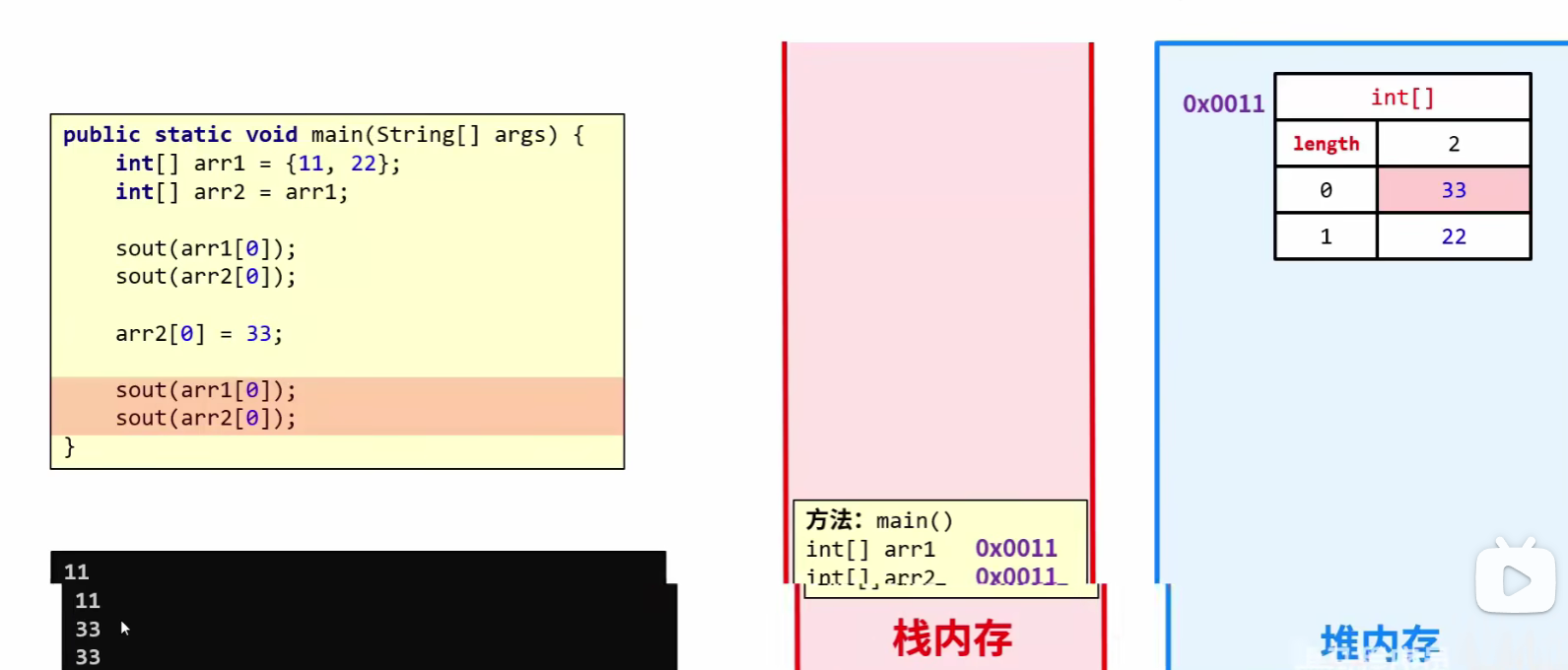
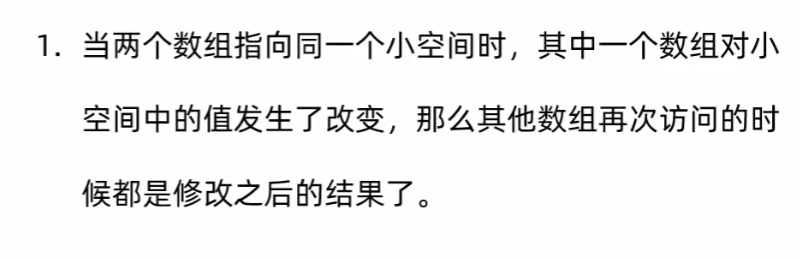
2.8二维数组初始化
2.8.1 维数组静态初始化
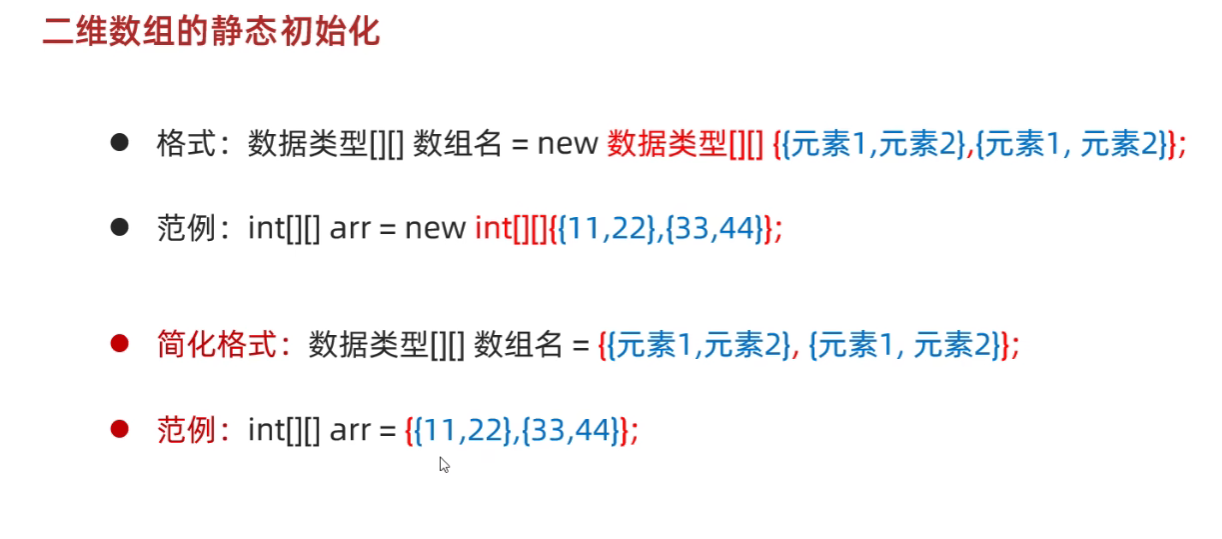
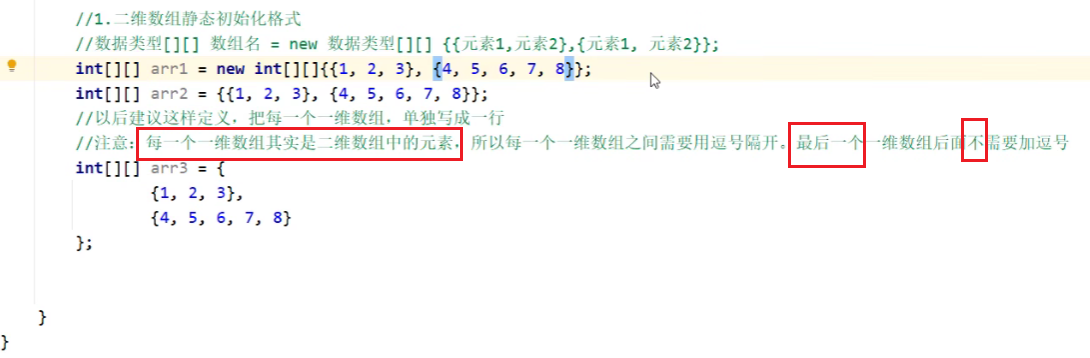
2.8.2二维数组动态初始化
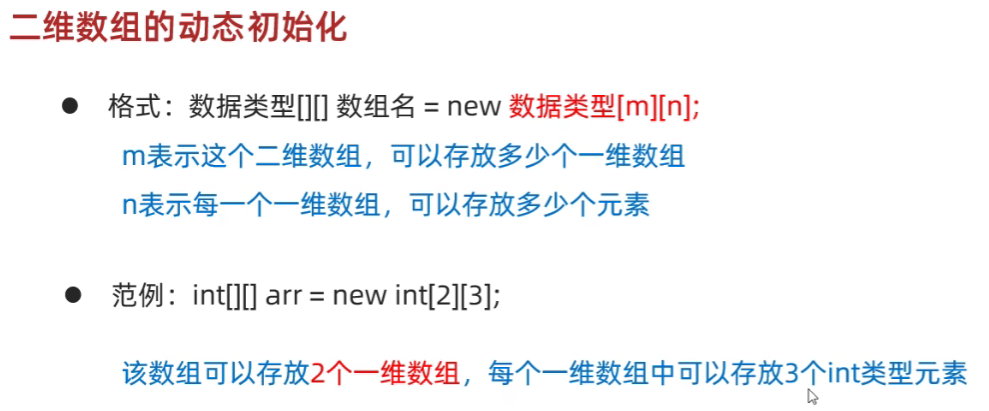
2.8.3遍历二维数组
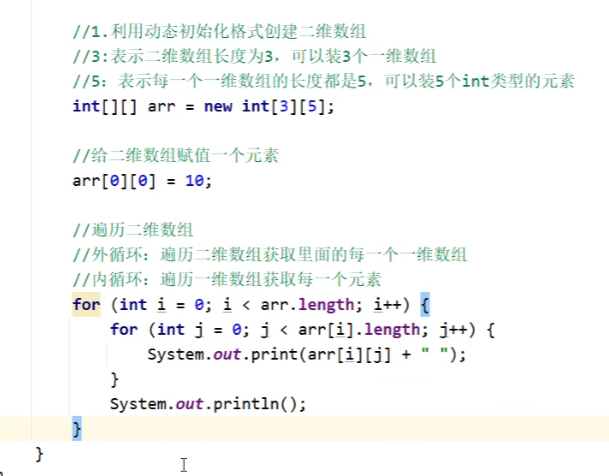
三、函数
3.1方法

方法的定义格式如下(就是C语言中的函数!)
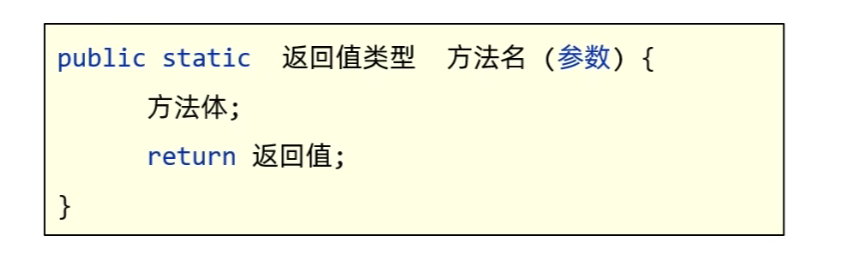
3.1.1方法的重载
什么是方法的重载??
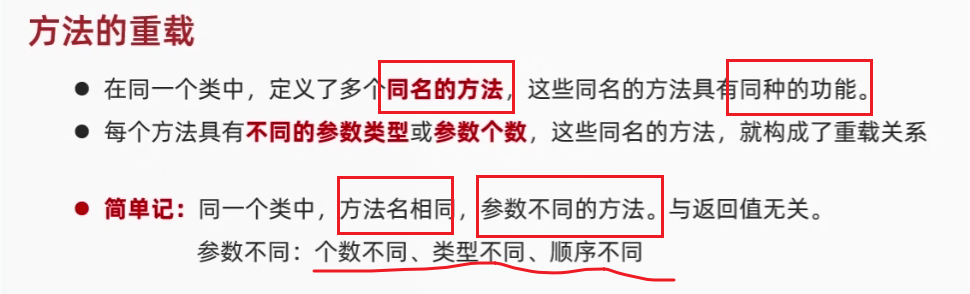
例如:
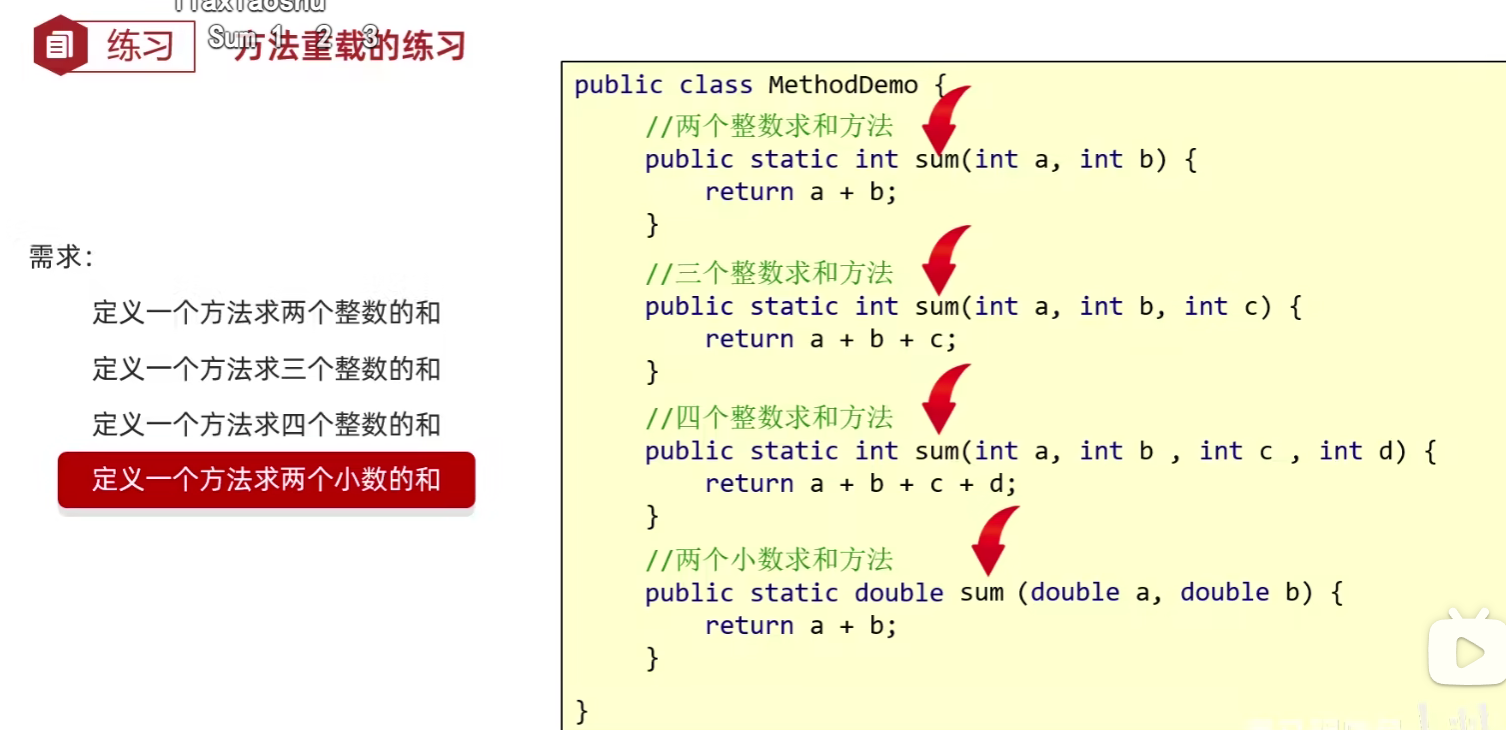
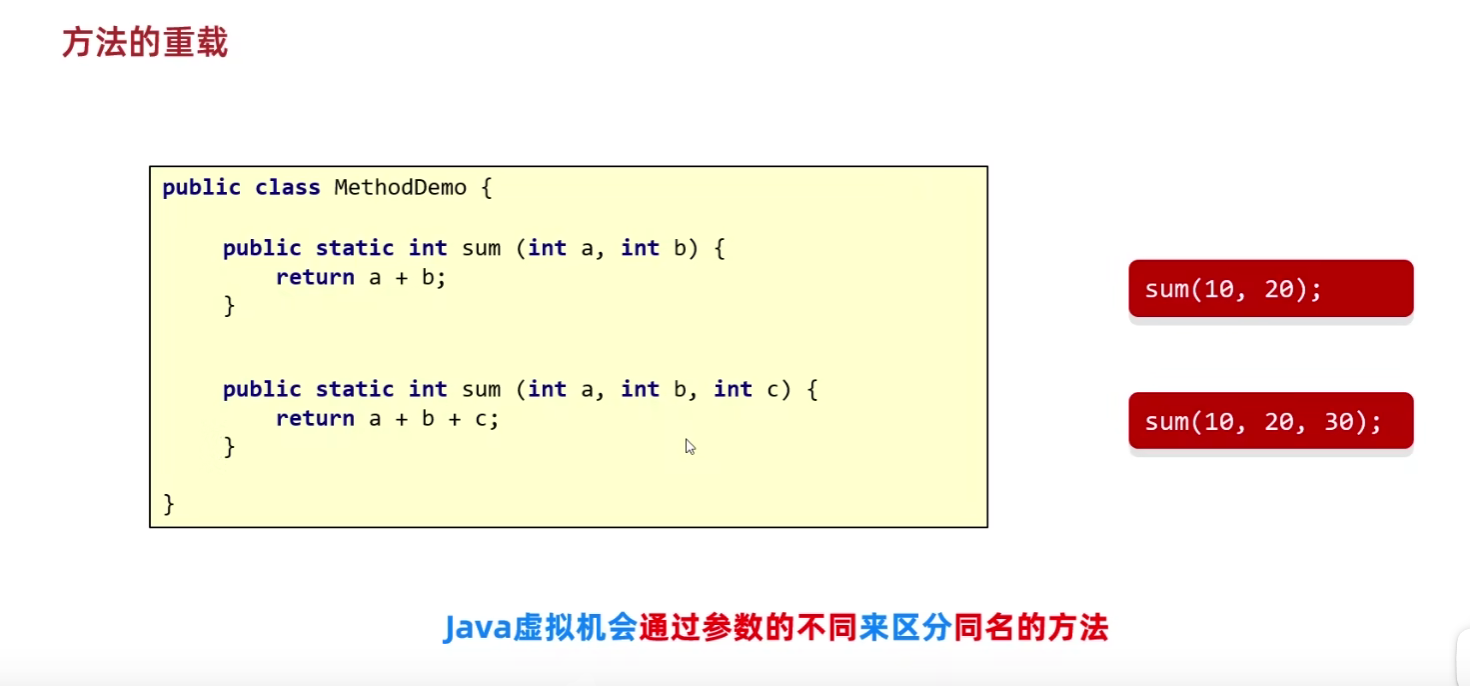
3.1.2判断是否构成重载关系
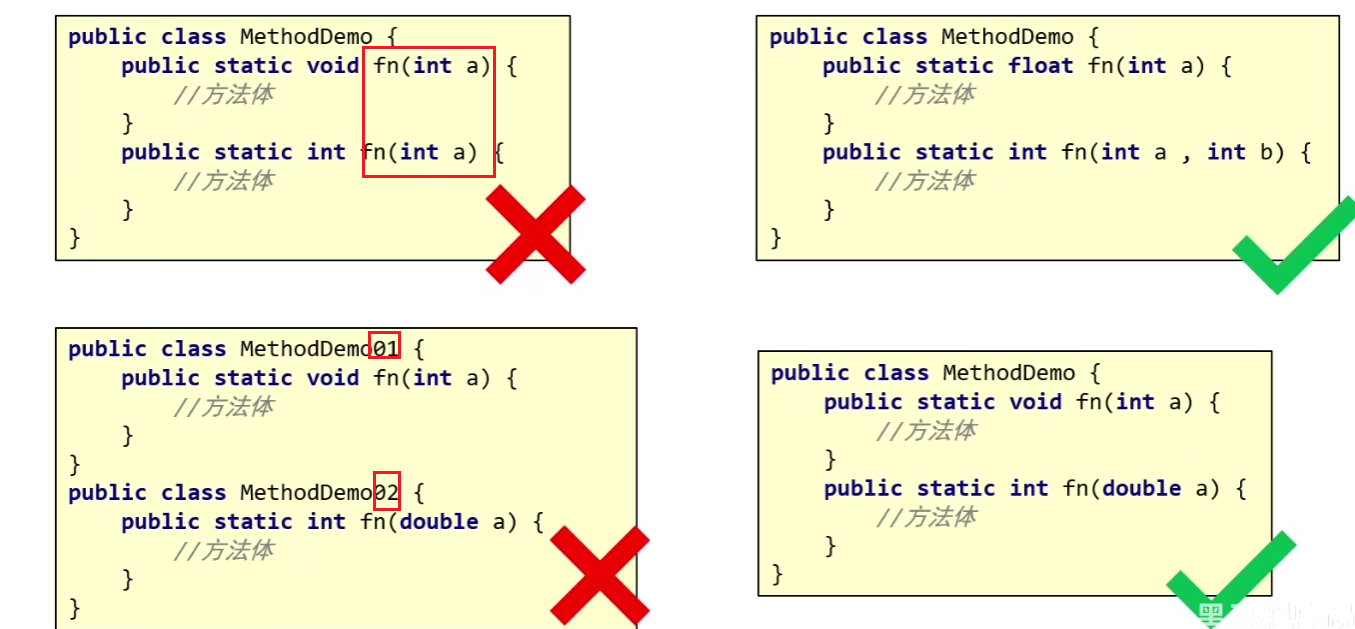
四、面向对象
4.1创建一个类
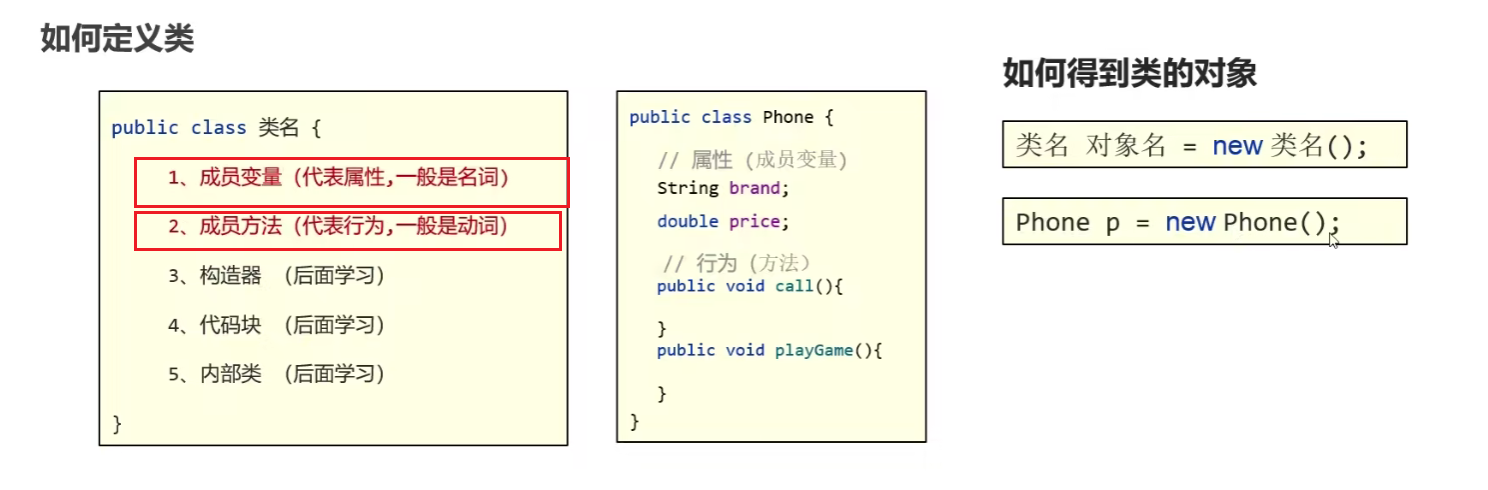
如何使用这个类?
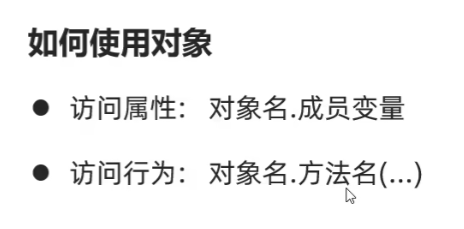
例如:
先定义一个手机对象
public class Phone {
//属性
String brand;
double price;
//行为
public void call(){
System.out.println("手机在打电话");
}
public void playGame(){
System.out.println("手机在打游戏");
}
}
创建实体对象,并使用
public class PhoneTest {
public static void main(String[] args) {
//创建手机的对象
Phone p = new Phone();
//使用新创建的对象
p.brand = "小米";
p.price = 998;
p.call();
p.playGame();
}
}
4.2封装
4.2.1关键字Private
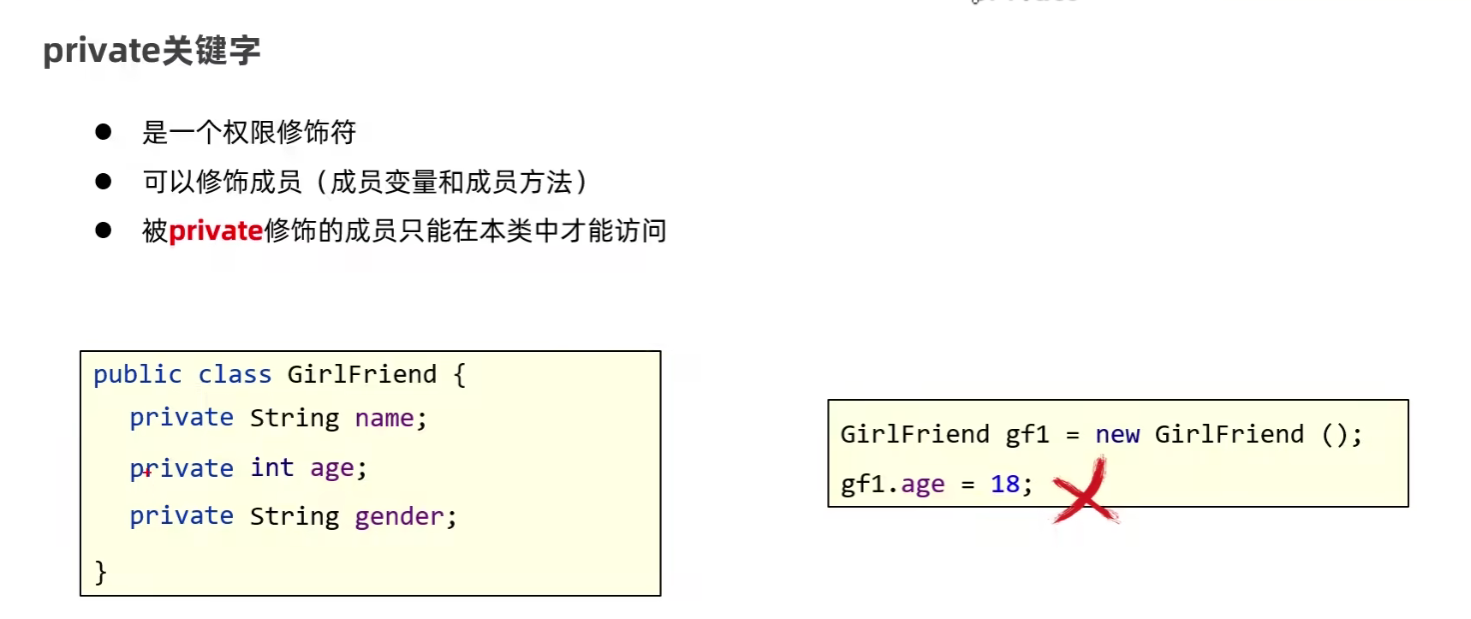
4.2.2使用Set和Get进行条件封装
例如先创建一个手机类,用Private和set、get进行封装
public class Phone {
//属性
private String brand;
private double price;
public void SetBrand(String n){
brand = n;
}
public String GetBrand(){
return brand;
}
public void setPrice(int a){
if(a > 500 && a<=1500){
price = a;
}
else{
System.out.println("非法参数");
}
}
public double GetPrice(){
return price;
}
//行为
public void call(){
System.out.println("手机在打电话");
}
public void playGame(){
System.out.println("手机在打游戏");
}
}
然后主函数进行使用,注意使用set和get方法
public class PhoneTest {
public static void main(String[] args) {
//创建手机的对象
Phone p = new Phone();
//使用新创建的对象
//创建第一个对象
p.setPrice(1299);
System.out.println(p.GetPrice());
p.SetBrand("vivo");
System.out.println(p.GetBrand());
p.call();
p.playGame();
}
}
运行结果
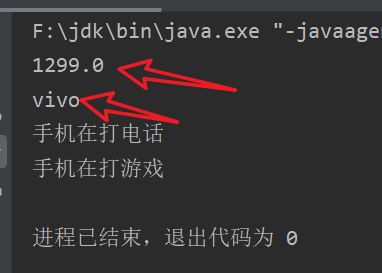
对封装进行总结
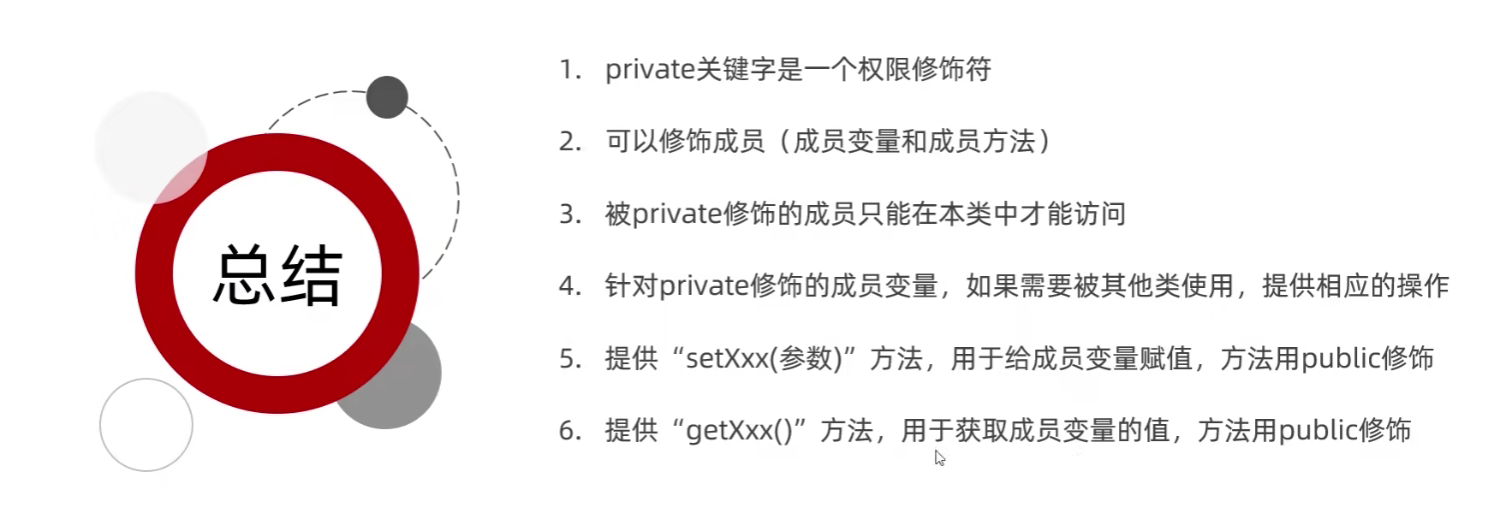
4.3this语句
this语句相当于跳过局部变量,直接访问成员变量
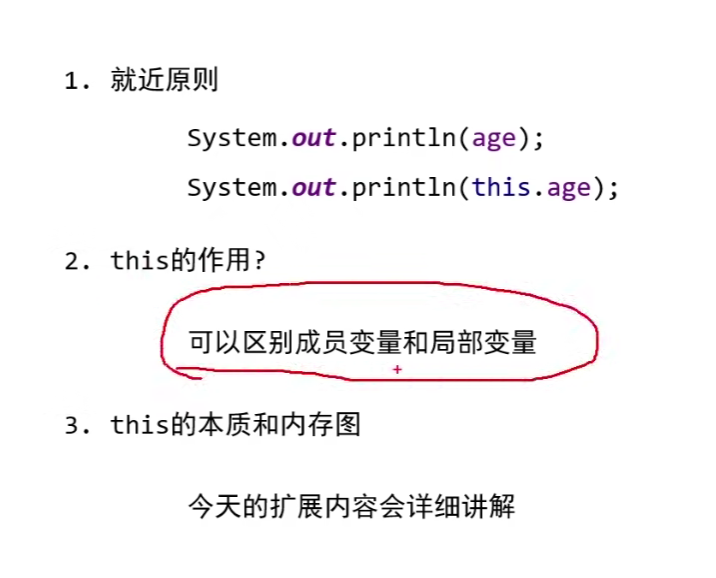
4.4构造方法
4.4.1构造方法的格式
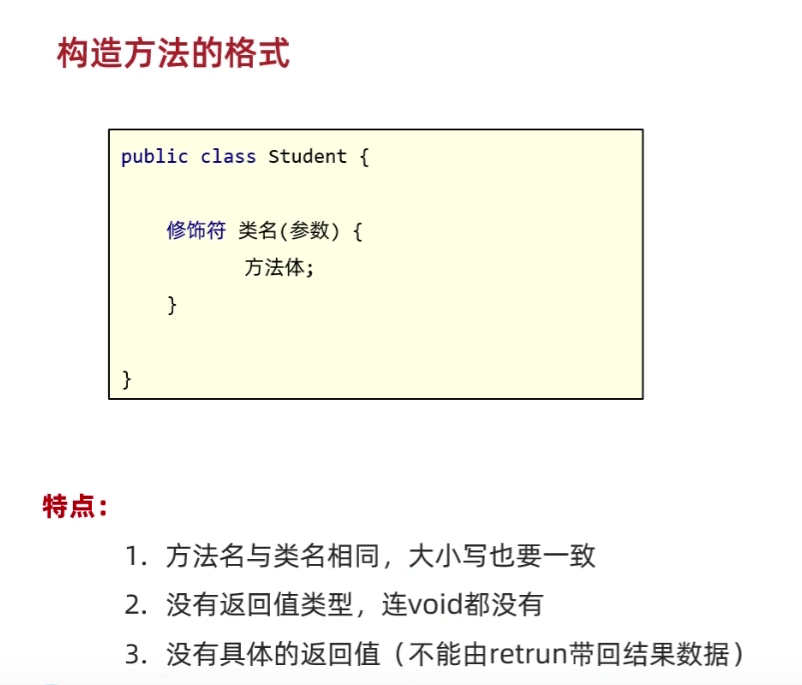
无参构造例子:

有参构造例子:
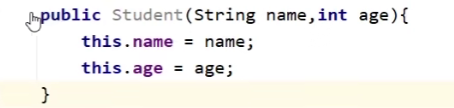
!!!!!注意事项!!!!!!
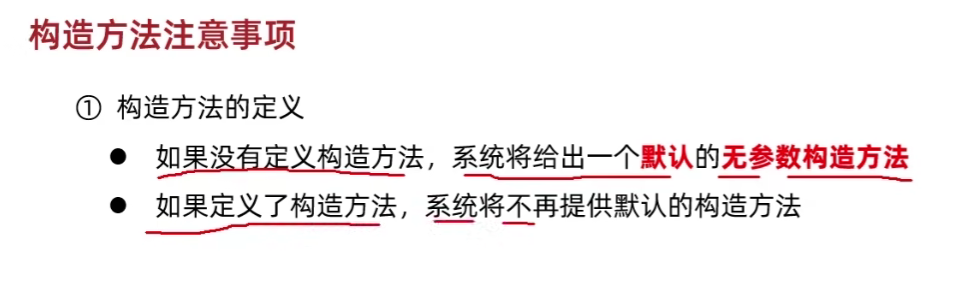
4.4.2总结
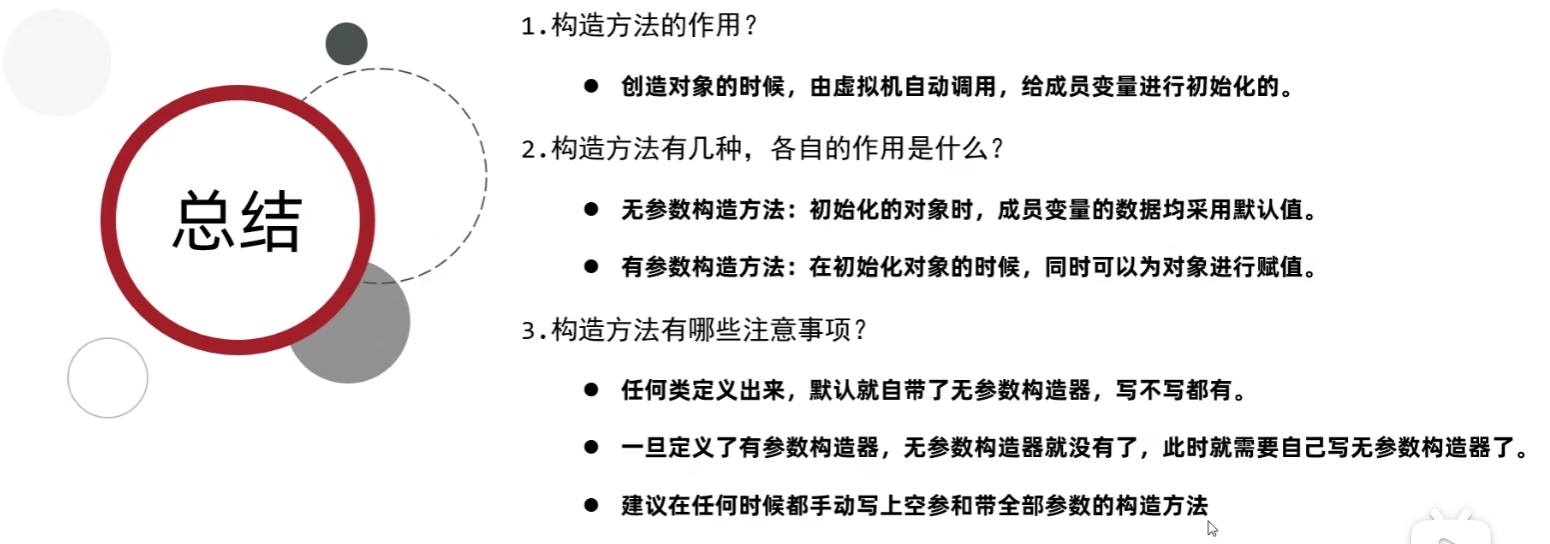
4.5标准的javabean类
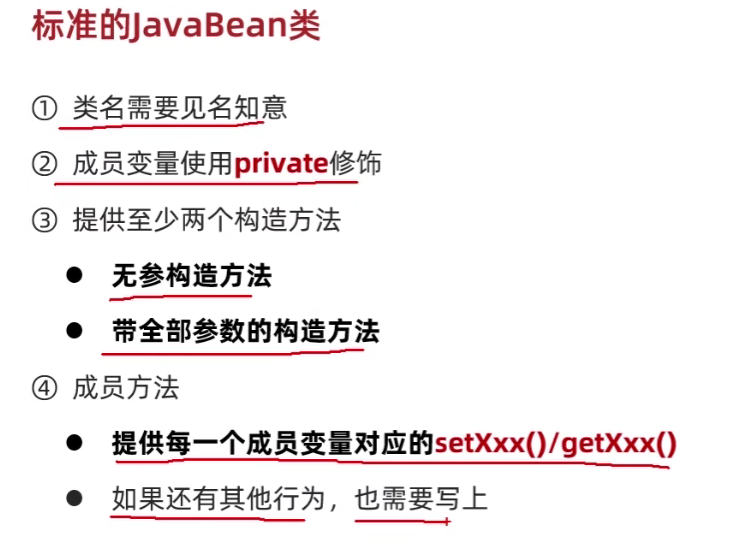
PS: 可以直接用PTG插件进行初始化构造