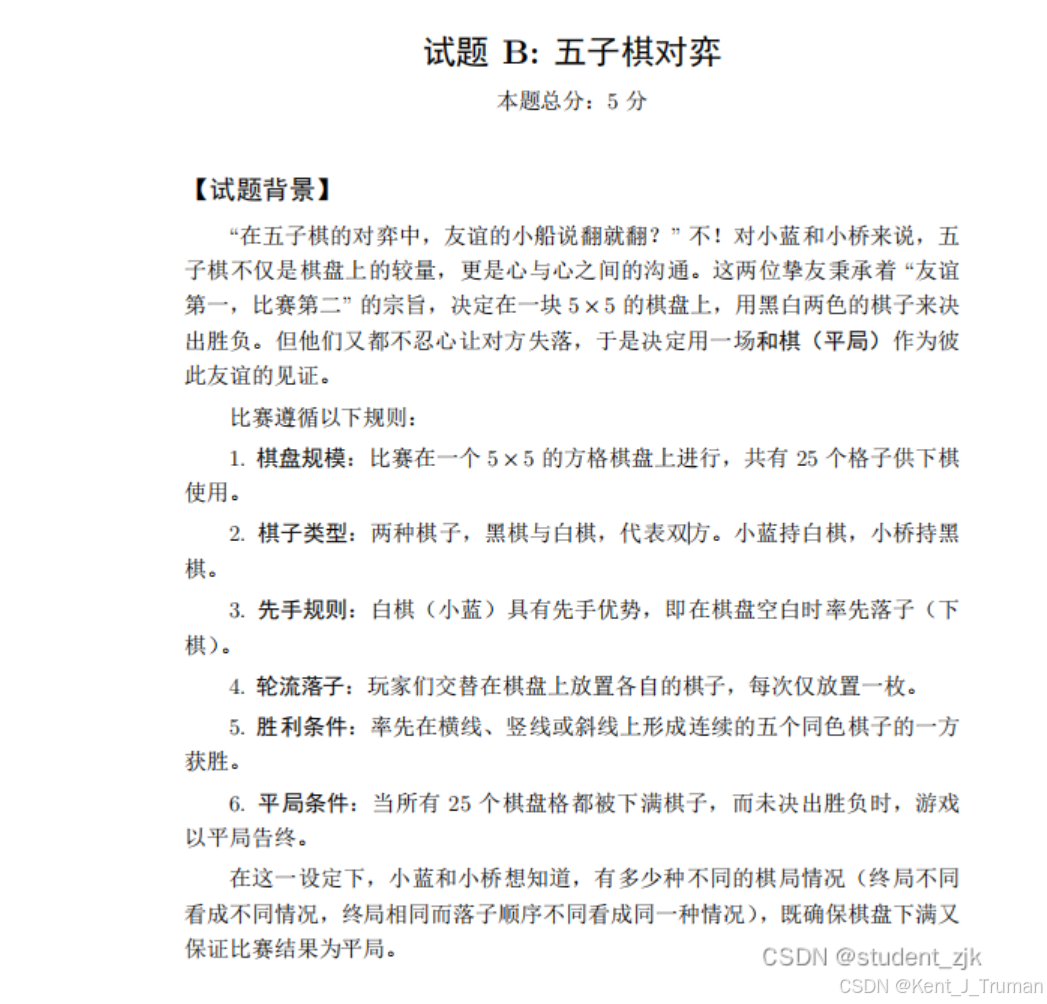
代码:
#include<bits/stdc++.h>
using namespace std;
int row[20];
int col[20];
int dia[20]; //对角线
int ndia[20]; //反对角线
int result;
void dfs(int n, int white, int black) //white+1, black-1
{
if(n > 25)
{
result++;
return;
}
int x = (n+5-1) / 5; //一维的n映射到二维的(x,y)
int y = n%5;
if(!y) y = 5;
if(white && row[x] < 4 && col[y] < 4 && dia[x-y+5] < 4 && ndia[x+y] < 4)
{
row[x]++;
col[y]++;
dia[x-y+5]++;
ndia[x+y]++;
dfs(n+1, white-1, black);
row[x]--;
col[y]--;
dia[x-y+5]--;
ndia[x+y]--;
}
if(black && row[x] > -4 && col[y] > -4 && dia[x-y+5] > -4 && ndia[x+y] > -4)
{
row[x]--;
col[y]--;
dia[x-y+5]--;
ndia[x+y]--;
dfs(n+1, white, black-1);
row[x]++;
col[y]++;
dia[x-y+5]++;
ndia[x+y]++;
}
return;
}
int main()
{
dfs(1, 13, 12);
cout << result << endl;
return 0;
}