并查集例题
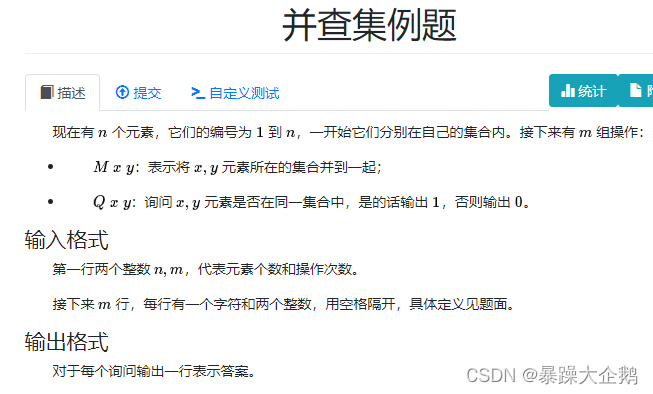
//并查集例题
#include<bits/stdc++.h>
using namespace std;
int n, m;
int fa[100001];
char str[10];
int getfa(int x){
if (x == fa[x]){
return x;
}
fa[x] = getfa(fa[x]);
return fa[x];
}
int main(){
ios::sync_with_stdio(false);
cin.tie(0);
cin >> n >> m;
for (int i = 1; i <= n; i++){
fa[i] = i;
}
for (int i = 1; i <= m; i++){
int x, y;
cin >> str >> x >> y;
if (str[0] == 'M'){
int fx = getfa(x), fy = getfa(y);
if (fx == fy){
continue;
}
fa[fx] = fy;
}
else {
int fx = getfa(x), fy = getfa(y);
if (fx == fy){
cout << "1" << '\n';
}
else {
cout << "0" << '\n';
}
}
}
}
数据量很大的时候,可以加入启发式合并
//并查集例题(加入启发式合并-按照size合并)
#include<bits/stdc++.h>
using namespace std;
int n, m;
int fa[100001], sz[100001];
char str[10];
int getfa(int x){
if (x == fa[x]){
return x;
}
fa[x] = getfa(fa[x]);
return fa[x];
}
int main(){
ios::sync_with_stdio(false);
cin.tie(0);
cin >> n >> m;
for (int i = 1; i <= n; i++){
fa[i] = i, sz[i] = 1;
}
for (int i = 1; i <= m; i++){
int x, y;
cin >> str >> x >> y;
if (str[0] == 'M'){
int fx = getfa(x), fy = getfa(y);
if (fx == fy){
continue;
}
if (sz[fx] > sz[fy]){
swap(fx, fy);
}
fa[fx] = fy;
sz[fy] += sz[fx];
}
else {
int fx = getfa(x), fy = getfa(y);
if (fx == fy){
cout << "1" << '\n';
}
else {
cout << "0" << '\n';
}
}
}
}
修路
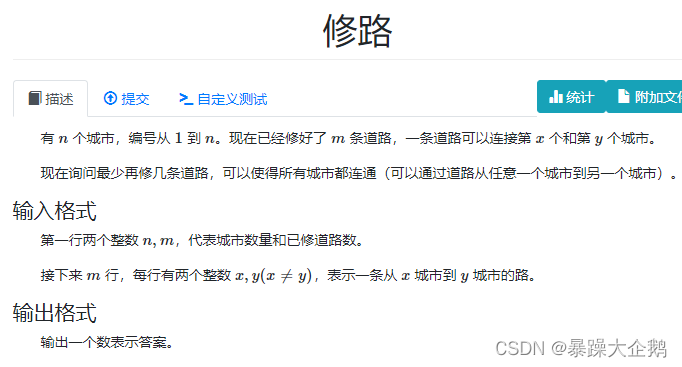
//修路
#include<bits/stdc++.h>
using namespace std;
int n, m;
int fa[100001], sz[100001];
char str[10];
inline int getfa(int x){
if (x == fa[x]){
return x;
}
fa[x] = getfa(fa[x]);
return fa[x];
}
int main(){
ios::sync_with_stdio(false);
cin.tie(0);
cin >> n >> m;
for (int i = 1; i <= n; i++){
fa[i] = i;
}
for (int i = 1; i <= m; i++){
int x, y;
cin >> x >> y;
int fx = getfa(x), fy = getfa(y);
if (fx == fy){
continue;
}
fa[fx] = fy;
}
int ans = 0;
for (int i = 1; i <= n; i++){
if (fa[i] == i){
ans++;
}
}
cout << ans - 1 << '\n';
}
行进路线
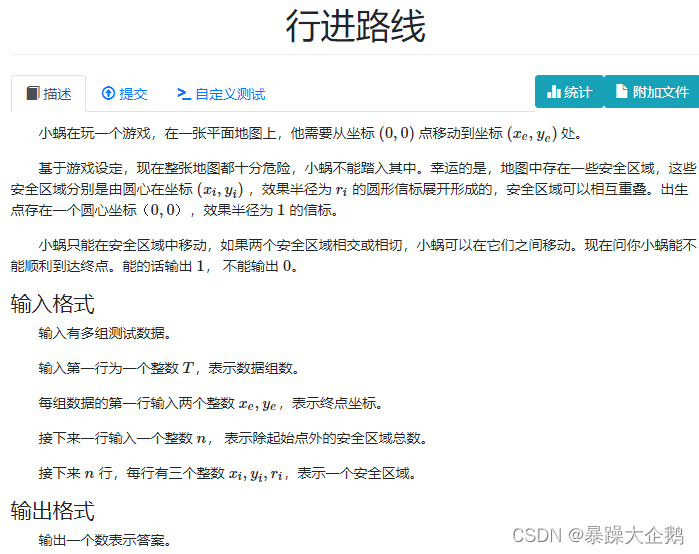
//行进路线
#include<bits/stdc++.h>
using namespace std;
long long t, n;
long long fa[1001], a[1001][3];
inline long long getfa(long long x){
if (x == fa[x]){
return x;
}
fa[x] = getfa(fa[x]);
return fa[x];
}
int main(){
ios::sync_with_stdio(false);
cin.tie(0);
cin >> t;
while (t--){
long long xx, yy;
cin >> xx >> yy >> n;
for (int i = 1; i <= n; i++){
cin >> a[i][0] >> a[i][1] >> a[i][2];
}
a[0][0] = 0, a[0][1] = 0, a[0][2] = 1;
for (int i = 0; i <= n; i++){
fa[i] = i;
}
for (int i = 0; i <= n; i++){
for (int j = i + 1; j <= n; j++){
long long temp = (a[j][1] - a[i][1]) * (a[j][1] - a[i][1]) + (a[j][0] - a[i][0]) * (a[j][0] - a[i][0]);
if (temp <= (a[i][2] + a[j][2]) * (a[i][2] + a[j][2])){
int fi = getfa(i), fj = getfa(j);
if (fi == fj){
continue;
}
fa[fi] = fj;
}
}
}
int idx = -1;
for (int i = 0; i <= n; i++){
long long temp = (a[i][0] - xx) * (a[i][0] - xx) + (a[i][1] - yy) * (a[i][1] - yy);
if (temp <= a[i][2] * a[i][2]){
idx = i;
}
}
if (idx != -1 && getfa(0) == getfa(idx)){
cout << "1" << '\n';
}
else {
cout << "0" << '\n';
}
}
}