改文章为C语言实现井字棋小游戏的完整代码,可供大家自行参考喔
完整代码如下:
/*井字棋小游戏*/
#include<stdio.h>
#include<string.h>
#define ROW 3
#define COL 3
char chessBoard[ROW][COL]={0};
void menu()
{
printf("*******************************************\n");
printf("****** 1.play 0.exit *******\n");
printf("*******************************************\n");
}
int begin(){
int input=0;
int flag=-1;
do{
menu();
printf("请选择->");
scanf("%d",&input);
switch(input){
case 1:
printf("井字棋\n");
flag=1;
break;
case 0:
printf("退出游戏!\n");
flag=0;
break;
default:
printf("选择错误,请重新选择\n");
// break;
}
}while(input!=1 && input!=0);
return flag;
}
void initchessBoard(char chessBoard[ROW][COL]){ //初始化棋盘
for(int row=0;row<ROW;row++){
for(int col=0;col<COL;col++){
chessBoard[row][col]=' ';
}
}
}
void printchessBoard(){
printf("\t+---+---+---+\n");
for(int row=0;row<ROW;row++){
printf("\t| %c | %c | %c |\n",chessBoard[row][0],chessBoard[row][1],chessBoard[row][2]);
printf("\t+---+---+---+\n");
}
}
void xplay(){
while(1){
int row=0;
int col=0;
printf("x玩家请下棋:");
scanf("%d%d",&row,&col);
if(row<0 || row>=ROW || col<0 || col>=COL){
printf("您输入的坐标不合法!,请重新输入\n");
continue;
}
if(chessBoard[row][col]!=' '){
printf("该位置已有棋子!\n");
continue;
}
chessBoard[row][col]='x';
break;
}
printchessBoard();
}
void oplay(){
while(1){
int row=0;
int col=0;
printf("o玩家请下棋:");
scanf("%d%d",&row,&col);
if(row<0 || row>=ROW || col<0 || col>=COL){
printf("您输入的坐标不合法!,请重新输入\n");
continue;
}
if(chessBoard[row][col]!=' '){
printf("该位置已有棋子!\n");
continue;
}
chessBoard[row][col]='o';
break;
}
printchessBoard();
}
int isfull(char chessBoard[ROW][COL]){
int flag=1;
for(int row=0;row<ROW;row++){
for(int col;col<COL;col++){
if(chessBoard[ROW][COL]==' '){
flag=0;
}
}
}
return flag; //如果返回1则棋盘已经下满,如果返回0则表示棋盘还未下满
}
int iswin(char chessBoard[ROW][COL])
{
int col,row;
int win=2;
for(int row=0;row<ROW;row++){
if(chessBoard[row][0]!=' ' && chessBoard[row][0]==chessBoard[row][1] && chessBoard[row][1]==chessBoard[row][2]){
if(chessBoard[row][0]=='x'){
win=1; //返回1表示x玩家赢
}
if(chessBoard[row][0]=='o'){
win=0;
}
return win;
}
}
for(int col=0;col<COL;col++){
if(chessBoard[0][col]!=' ' && chessBoard[0][col]==chessBoard[1][col] && chessBoard[1][col]==chessBoard[2][col]){
if(chessBoard[0][col]=='x'){
win=1;
}
if(chessBoard[0][col]=='o'){
win=0;
}
return win;
}
}
if(chessBoard[0][0]!=' ' && chessBoard[0][0]==chessBoard[1][1] && chessBoard[1][1]==chessBoard[2][2]){
if(chessBoard[0][col]=='x'){
win=1;
}
if(chessBoard[0][col]=='o'){
win=0;
}
return win;
}
if(chessBoard[2][0]!=' ' && chessBoard[2][0]==chessBoard[1][1] && chessBoard[1][1]==chessBoard[0][2]){
if(chessBoard[0][col]=='x'){
win=1;
}
if(chessBoard[0][col]=='o'){
win=0;
}
return win;
}
if(isfull(chessBoard)){
return win;
}
}
void judge(){
do{
xplay();
if(iswin(chessBoard)==1){
printf("\n\t恭喜x玩家获胜!");
break;
}
if(iswin(chessBoard)==0){
printf("\n\t恭喜o玩家获胜!");
break;
}
oplay();
if(iswin(chessBoard)==1){
printf("\n\t恭喜x玩家获胜!");
break;
}
if(iswin(chessBoard)==0){
printf("\n\t恭喜o玩家获胜!");
break;
}
}while(iswin(chessBoard)==2);
}
int main(){
int xflag;
xflag=begin();
if(xflag==1){
initchessBoard(chessBoard); //初始化棋盘
printchessBoard(); //打印棋盘形状
judge();
}
return 0;
}
运行结果:
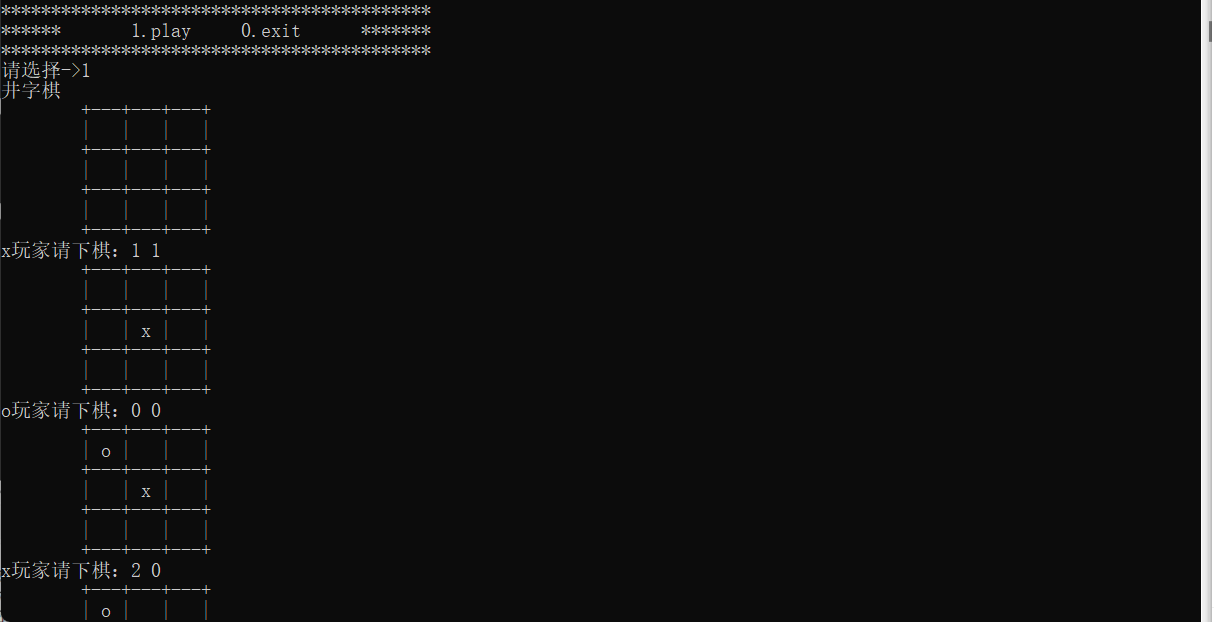
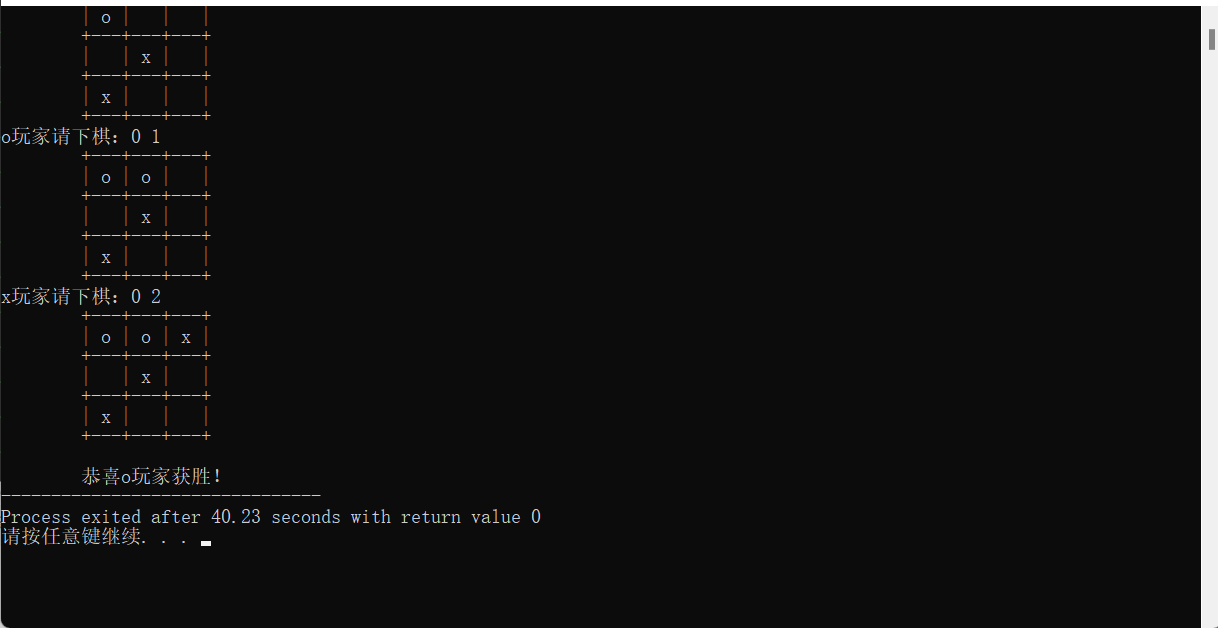
谢谢大家咯,如有改进欢迎指点喔