效果:
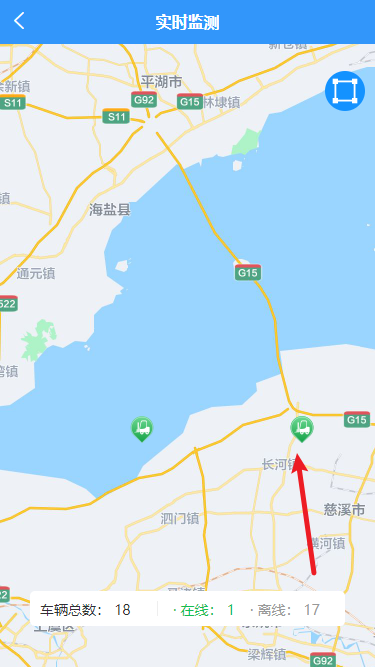
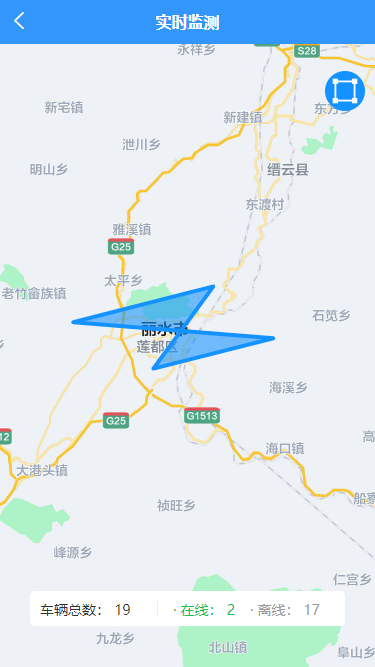
官网: https://uniapp.dcloud.net.cn/component/map.html#map
// 回显的数据格式
polygons: [
{
points: [
{
latitude: 28.51888245793022,
longitude: 119.98906378916045,
},
{
latitude: 28.475729153565684,
longitude: 119.7966929814844,
},
{
latitude: 28.456177380060186,
longitude: 120.07130302831642,
},
{
latitude: 28.41960240564566,
longitude: 119.90682381395553,
},
],
strokeWidth: 4, // 描边的宽度
strokeColor: '#1492ff', // 描边的颜色
fillColor: '#1492ff94', // 填充颜色
},
{
points: [
{
latitude: 36.79364322167569,
longitude: 118.07446541195007,
},
{
latitude: 33.440106715216565,
longitude: 109.72120044240603,
},
{
latitude: 30.27022820630607,
longitude: 119.5096078516515,
},
{
latitude: 37.47082862133101,
longitude: 118.7737041476045,
},
],
strokeWidth: 4, // 描边的宽度
strokeColor: '#1492ff', // 描边的颜色
fillColor: '#1492ff94', // 填充颜色
}
], // 多边形回显数据格式
covers: [
{
latitude: 39.909,
longitude: 116.39742,
iconPath: iconPath
}, {
latitude: 39.90,
longitude: 116.39,
iconPath: iconPath
}
] // 点位的数据格式
功能实现
<map ref="map"
id="map"
:latitude="latitude"
:longitude="longitude"
:markers="covers" // 地图标点
:scale="scale"
:min-scale="minScale"
:max-scale="maxScale"
:show-location="true"
:polygons="polygons" // 地图上画不规则图形
@regionchange="regionChange"
>
</map>
var iconPath = require('../../../../static/images/icon/forklift.png'); // 图标位置
export default {
data() {
return {
scale: 10, // 17
minScale: 3, // 最小缩放级别
maxScale: 20, // 最大缩放级别
latitude: 30.25, // 中心点
longitude: 121.21, // 中心点
covers: [], // 点位数据 // 可用 markers 代替
params: {
mapLevel: 11,
bottomLeftLatitude: 29.85357527749677,
bottomLeftLongitude: 120.95250793457029,
topRightLatitude: 30.644831586175155,
topRightLongitude: 121.46749206542967,
}, // 请求接口的参数(获取点位 --- 点聚合)
polygons: [], // 多边形选框数据
polygonsFlag: false, // 用户是否展示选框的标志
}
},
methods: {
/**
* @Interface 获取所有的点位数据
* */
getPointers() {
this.$u.api.realTimeMonitoring.selectCarPoints(this.params).then(res => {
if (res.code === 1) {
if (res.data !== []) {
res.data.forEach((item, index) => {
this.covers.push({
latitude: item.y,
longitude: item.x,
iconPath,
})
})
}
}
})
},
/**
* @Interface 展示边界
* */
showBorder() {
this.polygonsFlag = !this.polygonsFlag;
if (this.polygonsFlag) {
this.$u.api.realTimeMonitoring.allLists().then(res => {
if (res.code === 1) {
this.polygons = res.data.polygons
}
})
} else {
this.polygons = []
}
},
/**
* 获取可视区的经纬度
* */
regionChange() {
let map = uni.createMapContext('map');
map.getRegion({
success: res => {
this.params = {
mapLevel: this.scale,
bottomLeftLatitude: res.southwest.latitude,
bottomLeftLongitude: res.southwest.longitude,
topRightLatitude: res.northeast.latitude,
topRightLongitude: res.northeast.longitude,
}
setTimeout(() => {
this.getPointers()
}, 500)
},
fail: (data, code) => {
console.log('fail' + JSON.stringify(data));
}
})
},
},
onLoad() {
this.getPointers()
}
}