#include<stdio.h>
#include<stdlib.h>
# define NULL 0
struct student
{
int num;
char name[20];
struct student *next;
};
struct student *create()
{
struct student *head,*p,*last;
int num;
printf("请输入学号:");
scanf("%d",&num) ;
if (num==0)
head=NULL;
else
{p=(struct student*)malloc(sizeof(struct student));
head=p;
do
{
printf("请输入学生姓名:");
scanf("%s",p->name);
p->num=num;
p->next=NULL;
printf("请输入学号:");
scanf("%d",&num) ;
if(num!=0)
{p=(struct student*)malloc(sizeof(struct student));
last=head;
while(last)
{
last=last->next;
}
last->next=p;
}
}
while(num!=0);
}
return head;
}
void print(struct student *head)
{
struct student *p;
p=head;
while(p)
{
printf("%d,%s",p->num ,p->next);
p=p->next;
}
}
int main()
{
struct student *head;
head=create();
print(head);
}
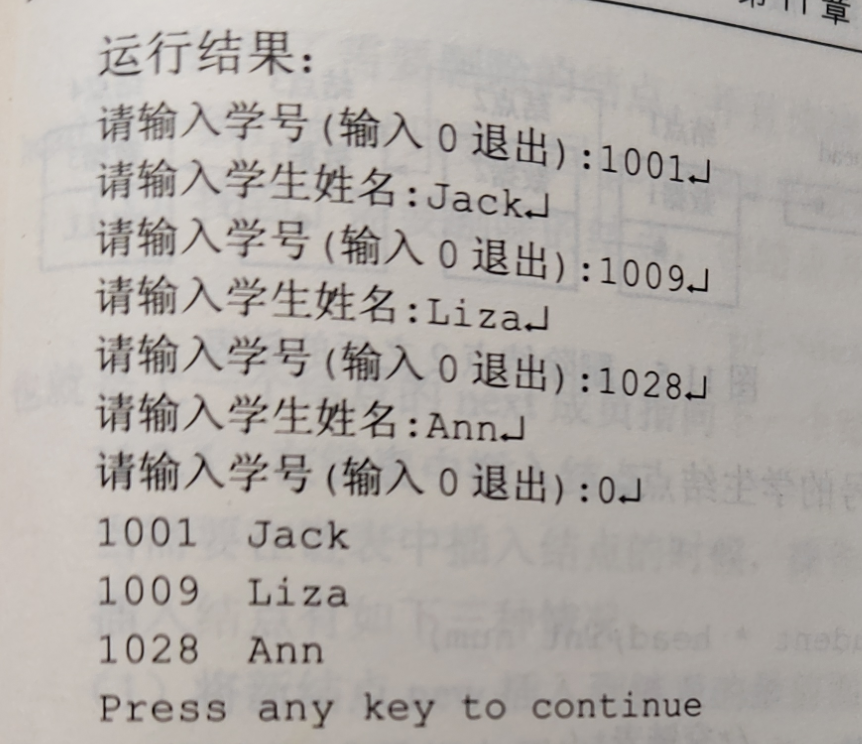