final = []
bs = BeautifulSoup(html_text, “html.parser”) # 创建BeautifulSoup对象
body = bs.body #获取body
data = body.find(‘div’,{‘id’: ‘7d’})
ul = data.find(‘ul’)
li = ul.find_all(‘li’)
for day in li:
temp = []
date = day.find(‘h1’).string
temp.append(date) #添加日期
inf = day.find_all(‘p’)
weather = inf[0].string #天气
temp.append(weather)
temperature_highest = inf[1].find(‘span’).string #最高温度
temperature_low = inf[1].find(‘i’).string # 最低温度
temp.append(temperature_low)
temp.append(temperature_highest)
final.append(temp)
print(‘getDate success’)
return final
**上面的解析其实就是按照 HTML 的规则解析的。可以打开 杭州天气 在开发者模式中(F12),看一下页面的元素分布。**
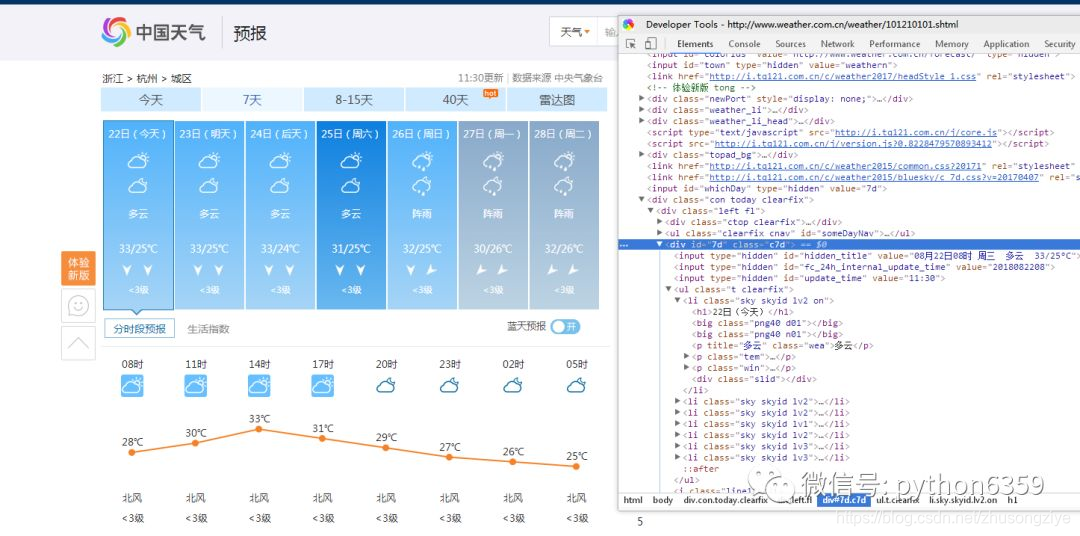
**在 main 方法中调用:**
if name == ‘main’:
url =‘http://www.weather.com.cn/weather/101210101.shtml’
html = getContent(url) # 获取网页信息
result = getData(html) # 解析网页信息,拿到需要的数据
print(‘my frist python file’)
**数据写入excel**
现在我们已经在 Python 中拿到了想要的数据,对于这些数据我们可以先存放起来,比如把数据写入 csv 中。
**定义一个 writeDate 方法:**
import csv #导入包
def writeData(data, name):
with open(name, ‘a’, errors=‘ignore’, newline=‘’) as f:
f_csv = csv.writer(f)
f_csv.writerows(data)
print(‘write_csv success’)
**在 main 方法中调用:**
if name == ‘main’:
url =‘http://www.weather.com.cn/weather/101210101.shtml’
html = getContent(url) # 获取网页信息
result = getData(html) # 解析网页信息,拿到需要的数据
writeData(result, ‘D:/py_work/venv/Include/weather.csv’) #数据写入到 csv文档中
print(‘my frist python file’)
执行之后呢,再指定路径下就会多出一个 weather.csv 文件,可以打开看一下内容。
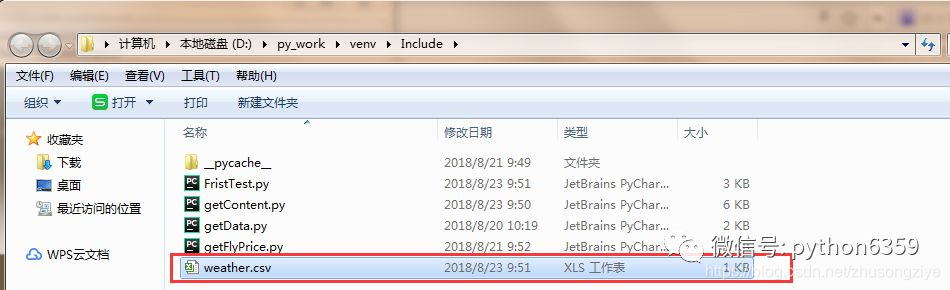
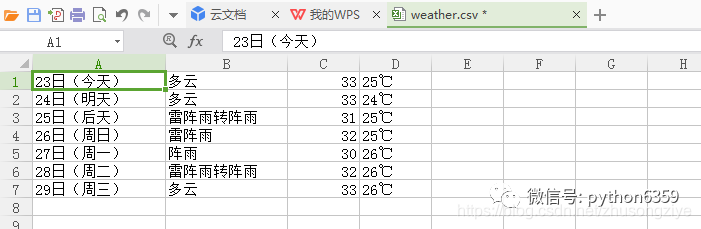
到这里最简单的数据抓取--储存就完成了。
**数据写入数据库**
因为一般情况下都会把数据存储在数据库中,所以我们以 mysql 数据库为例,尝试着把数据写入到我们的数据库中。
**第一步创建WEATHER 表:**
创建表可以在直接在 mysql 客户端进行操作,也可能用 python 创建表。在这里 我们使用 python 来创建一张 WEATHER 表。
定义一个 createTable 方法:(之前已经导入了 import pymysql 如果没有的话需要导入包)
def createTable():
# 打开数据库连接
db = pymysql.connect(“localhost”, “zww”, “960128”, “test”)
# 使用 cursor() 方法创建一个游标对象 cursor
cursor = db.cursor()
# 使用 execute() 方法执行 SQL 查询
cursor.execute(“SELECT VERSION()”)
# 使用 fetchone() 方法获取单条数据.
data = cursor.fetchone()
print("Database version : %s " % data) # 显示数据库版本(可忽略,作为个栗子)
# 使用 execute() 方法执行 SQL,如果表存在则删除
cursor.execute(“DROP TABLE IF EXISTS WEATHER”)
# 使用预处理语句创建表
sql = “”“CREATE TABLE WEATHER (
w_id int(8) not null primary key auto_increment,
w_date varchar(20) NOT NULL ,
w_detail varchar(30),
w_temperature_low varchar(10),
w_temperature_high varchar(10)) DEFAULT CHARSET=utf8"”" # 这里需要注意设置编码格式,不然中文数据无法插入
cursor.execute(sql)
# 关闭数据库连接
db.close()
print(‘create table success’)
**在 main 方法中调用:**
if name == ‘main’:
url =‘http://www.weather.com.cn/weather/101210101.shtml’
html = getContent(url) # 获取网页信息
result = getData(html) # 解析网页信息,拿到需要的数据
writeData(result, ‘D:/py_work/venv/Include/weather.csv’) #数据写入到 csv文档中
createTable() #表创建一次就好了,注意
print(‘my frist python file’)
执行之后去检查一下数据库,看一下 weather 表是否创建成功了。
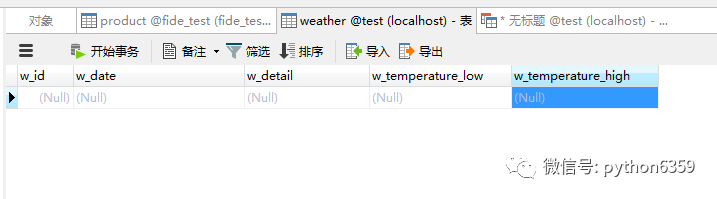
**第二步批量写入数据至 WEATHER 表:**
**定义一个 insertData 方法:**
def insert_data(datas):
# 打开数据库连接
db = pymysql.connect(“localhost”, “zww”, “960128”, “test”)
# 使用 cursor() 方法创建一个游标对象 cursor
cursor = db.cursor()
try:
# 批量插入数据
cursor.executemany(‘insert into WEATHER(w_id, w_date, w_detail, w_temperature_low, w_temperature_high) value(null, %s,%s,%s,%s)’, datas)
# sql = “INSERT INTO WEATHER(w_id,
# w_date, w_detail, w_temperature)
# VALUES (null, ‘%s’,‘%s’,‘%s’)” %
# (data[0], data[1], data[2])
# cursor.execute(sql) #单条数据写入
# 提交到数据库执行
db.commit()
except Exception as e:
print(‘插入时发生异常’ + e)
# 如果发生错误则回滚
db.rollback()
# 关闭数据库连接
db.close()
**在 main 方法中调用:**
if name == ‘main’:
url =‘http://www.weather.com.cn/weather/101210101.shtml’
html = getContent(url) # 获取网页信息
result = getData(html) # 解析网页信息,拿到需要的数据
writeData(result, ‘D:/py_work/venv/Include/weather.csv’) #数据写入到 csv文档中
# createTable() #表创建一次就好了,注意
insertData(result) #批量写入数据
print(‘my frist python file’)
检查:执行这段 Python 语句后,看一下数据库是否有写入数据。有的话就大功告成了。
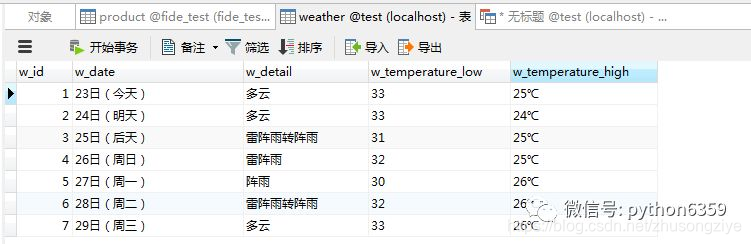
**全部代码看这里:**
# 导入相关联的包
import requests
import time
import random
import socket
import http.client
import pymysql
from bs4 import BeautifulSoup
import csv
def getContent(url , data = None):
header={
‘Accept’: ‘text/html,application/xhtml+xml,application/xml;q=0.9,image/webp,/;q=0.8’,
‘Accept-Encoding’: ‘gzip, deflate, sdch’,
‘Accept-Language’: ‘zh-CN,zh;q=0.8’,
‘Connection’: ‘keep-alive’,
‘User-Agent’: ‘Mozilla/5.0 (Windows NT 6.3; WOW64) AppleWebKit/537.36 (KHTML, like Gecko) Chrome/43.0.235’
} # request 的请求头
timeout = random.choice(range(80, 180))
while True:
try:
rep = requests.get(url,headers = header,timeout = timeout) #请求url地址,获得返回 response 信息
rep.encoding = ‘utf-8’
break
except socket.timeout as e: # 以下都是异常处理
print( ‘3:’, e)
time.sleep(random.choice(range(8,15)))
except socket.error as e:
print( ‘4:’, e)
time.sleep(random.choice(range(20, 60)))
except http.client.BadStatusLine as e:
print( ‘5:’, e)
time.sleep(random.choice(range(30, 80)))
except http.client.IncompleteRead as e:
print( ‘6:’, e)
time.sleep(random.choice(range(5, 15)))
print(‘request success’)
return rep.text # 返回的 Html 全文
def getData(html_text):
final = []
bs = BeautifulSoup(html_text, “html.parser”) # 创建BeautifulSoup对象
body = bs.body #获取body
data = body.find(‘div’,{‘id’: ‘7d’})
ul = data.find(‘ul’)
li = ul.find_all(‘li’)
for day in li:
temp = []
date = day.find(‘h1’).string
temp.append(date) #添加日期
inf = day.find_all(‘p’)
weather = inf[0].string #天气
temp.append(weather)
temperature_highest = inf[1].find(‘span’).string #最高温度
temperature_low = inf[1].find(‘i’).string # 最低温度
temp.append(temperature_highest)
temp.append(temperature_low)
final.append(temp)
print(‘getDate success’)
return final
def writeData(data, name):
with open(name, ‘a’, errors=‘ignore’, newline=‘’) as f:
f_csv = csv.writer(f)
f_csv.writerows(data)
print(‘write_csv success’)
def createTable():
# 打开数据库连接
db = pymysql.connect(“localhost”, “zww”, “960128”, “test”)
# 使用 cursor() 方法创建一个游标对象 cursor
cursor = db.cursor()
# 使用 execute() 方法执行 SQL 查询
cursor.execute(“SELECT VERSION()”)
# 使用 fetchone() 方法获取单条数据.
data = cursor.fetchone()
print("Database version : %s " % data) # 显示数据库版本(可忽略,作为个栗子)
# 使用 execute() 方法执行 SQL,如果表存在则删除
cursor.execute(“DROP TABLE IF EXISTS WEATHER”)
# 使用预处理语句创建表
sql = “”“CREATE TABLE WEATHER (
w_id int(8) not null primary key auto_increment,
w_date varchar(20) NOT NULL ,
w_detail varchar(30),
w_temperature_low varchar(10),
w_temperature_high varchar(10)) DEFAULT CHARSET=utf8"”"
cursor.execute(sql)
# 关闭数据库连接
db.close()
print(‘create table success’)
def insertData(datas):
# 打开数据库连接
db = pymysql.connect(“localhost”, “zww”, “960128”, “test”)
# 使用 cursor() 方法创建一个游标对象 cursor
cursor = db.cursor()
try:
# 批量插入数据
cursor.executemany(‘insert into WEATHER(w_id, w_date, w_detail, w_temperature_low, w_temperature_high) value(null, %s,%s,%s,%s)’, datas)
# 提交到数据库执行
db.commit()
except Exception as e:
print(‘插入时发生异常’ + e)
# 如果发生错误则回滚
db.rollback()
# 关闭数据库连接
db.close()
print(‘insert data success’)
if name == ‘main’:
url =‘http://www.weather.com.cn/weather/101210101.shtml’
html = getContent(url) # 获取网页信息
result = getData(html) # 解析网页信息,拿到需要的数据
writeData(result, ‘D:/py_work/venv/Include/weather.csv’) #数据写入到 csv文档中
做了那么多年开发,自学了很多门编程语言,我很明白学习资源对于学一门新语言的重要性,这些年也收藏了不少的Python干货,对我来说这些东西确实已经用不到了,但对于准备自学Python的人来说,或许它就是一个宝藏,可以给你省去很多的时间和精力。
别在网上瞎学了,我最近也做了一些资源的更新,只要你是我的粉丝,这期福利你都可拿走。
我先来介绍一下这些东西怎么用,文末抱走。
(1)Python所有方向的学习路线(新版)
这是我花了几天的时间去把Python所有方向的技术点做的整理,形成各个领域的知识点汇总,它的用处就在于,你可以按照上面的知识点去找对应的学习资源,保证自己学得较为全面。
最近我才对这些路线做了一下新的更新,知识体系更全面了。
(2)Python学习视频
包含了Python入门、爬虫、数据分析和web开发的学习视频,总共100多个,虽然没有那么全面,但是对于入门来说是没问题的,学完这些之后,你可以按照我上面的学习路线去网上找其他的知识资源进行进阶。
(3)100多个练手项目
我们在看视频学习的时候,不能光动眼动脑不动手,比较科学的学习方法是在理解之后运用它们,这时候练手项目就很适合了,只是里面的项目比较多,水平也是参差不齐,大家可以挑自己能做的项目去练练。
(4)200多本电子书
这些年我也收藏了很多电子书,大概200多本,有时候带实体书不方便的话,我就会去打开电子书看看,书籍可不一定比视频教程差,尤其是权威的技术书籍。
基本上主流的和经典的都有,这里我就不放图了,版权问题,个人看看是没有问题的。
(5)Python知识点汇总
知识点汇总有点像学习路线,但与学习路线不同的点就在于,知识点汇总更为细致,里面包含了对具体知识点的简单说明,而我们的学习路线则更为抽象和简单,只是为了方便大家只是某个领域你应该学习哪些技术栈。
(6)其他资料
还有其他的一些东西,比如说我自己出的Python入门图文类教程,没有电脑的时候用手机也可以学习知识,学会了理论之后再去敲代码实践验证,还有Python中文版的库资料、MySQL和HTML标签大全等等,这些都是可以送给粉丝们的东西。
这些都不是什么非常值钱的东西,但对于没有资源或者资源不是很好的学习者来说确实很不错,你要是用得到的话都可以直接抱走,关注过我的人都知道,这些都是可以拿到的。
网上学习资料一大堆,但如果学到的知识不成体系,遇到问题时只是浅尝辄止,不再深入研究,那么很难做到真正的技术提升。
一个人可以走的很快,但一群人才能走的更远!不论你是正从事IT行业的老鸟或是对IT行业感兴趣的新人,都欢迎加入我们的的圈子(技术交流、学习资源、职场吐槽、大厂内推、面试辅导),让我们一起学习成长!