目录
后端部分
mapper层
package com.woniu.community.mapper;
import com.woniu.community.entity.User;
import java.util.List;
public interface UserMapper {
User selectByNameAndPwd(String username, String passwd);
List<User> selectAll(int start, int size);
int selectCount();
int insertUser(User user);
int updateUser(User user);
int deleteUser(int id);
User selectUserById(int id);
}
mapper sql文件
<insert id="insertUser">
insert into userinfo(username,password) values(#{username},#{password})
</insert>
<update id="updateUser">
update userinfo set username=#{username},password=#{password} where id=#{id}
</update>
<delete id="deleteUser">
delete from userinfo where id=#{id}
</delete>
<select id="selectUserById" resultMap="userResult">
select * from userinfo where id=#{id}
</select>
Service层
接口
/**
* 添加用户
* @param user
* @return
*/
HttpResult addUser(User user);
/**
* 删除用户,根据id
* @param id
* @return
*/
HttpResult removeUser(int id);
/**
* 修改用户
* @param user
* @return
*/
HttpResult updateUser(User user);
/**
* 查询用户,根据id
* @param id
* @return
*/
HttpResult getUserInfo(int id);
@Override
public HttpResult addUser(User user) {
int count = userMapper.insertUser(user);//添加用户
HttpResult result = null;
if (count > 0 ) {
result = new HttpResult(null, 0, 200, "新增成功");
} else {
result = new HttpResult(null, 0, 500, "新增失败");
}
return result;
}
@Override
public HttpResult removeUser(int id) {
int count = userMapper.deleteUser(id);//添加用户
HttpResult result = null;
if (count > 0 ) {
result = new HttpResult(null, 0, 200, "删除成功");
} else {
result = new HttpResult(null, 0, 500, "删除失败");
}
return result;
}
@Override
public HttpResult updateUser(User user) {
int count = userMapper.updateUser(user);//添加用户
HttpResult result = null;
if (count > 0 ) {
result = new HttpResult(null, 0, 200, "修改成功");
} else {
result = new HttpResult(null, 0, 500, "修改失败");
}
return result;
}
@Override
public HttpResult getUserInfo(int id) {
User user = userMapper.selectUserById(id);
HttpResult result = null;
if (user != null ) {
result = new HttpResult(user, 0, 200, null);
} else {
result = new HttpResult(null, 0, 500, "没有更多数据");
}
return result;
}
Controller层
@PostMapping("/add")
public HttpResult addUser(@RequestBody UserVO vo) {
User user = new User();
user.setUsername(vo.getUsername());
user.setPassword(vo.getPassword());
return userService.addUser(user);
}
@RequestMapping("/remove")
public HttpResult removeUser(int id) {
return userService.removeUser(id);
}
@PostMapping("/update")
public HttpResult updateUser(@RequestBody User user) {
return userService.updateUser(user);
}
@RequestMapping("/info")
public HttpResult getUserInfo(int id) {
return userService.getUserInfo(id);
}
前端部分
源码
列表(增加和修改的跳转,删除功能)
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<title>Title</title>
<link href="assets/bootstrap-3.3.7-dist/css/bootstrap.min.css" rel="stylesheet">
<link href="assets/css/right.css" rel="stylesheet">
<script src="assets/jquery-3.5.1.min.js"></script>
<script src="assets/bootstrap-3.3.7-dist/js/bootstrap.min.js"></script>
<script src="assets/vue.min-v2.5.16.js"></script>
<script src="assets/vue-router.min-2.7.0.js"></script>
<script src="assets/axios.min.js"></script>
</head>
<body>
<div id="app" class="container">
<div class="row">
<div class="col-md-12" style="height: 50px; line-height: 50px;">
<button class="btn btn-info"@click="doAdd">新增</button>
</div>
</div>
<div class="row">
<div class="col-md-12">
<table class="table table-striped">
<caption>用户列表</caption>
<thead>
<tr>
<th>ID</th>
<th>用户名</th>
<th>操作</th>
</tr>
</thead>
<tbody>
<tr v-for="u in userList">
<td>{{u.id}}</td>
<td>{{u.username}}</td>
<td>
<button class="btn btn-link"@Click="doUpdate(u.id)">修改</button>
<button class="btn btn-link"@Click="doDelete(u.id)">删除</button>
</td>
</tr>
</tbody>
</table>
<ul class="pagination" v-for="p in pageNum">
<li v-if="p==pageIndex" class="active"><a @click="doGo(p)">{{p}}</a></li>
<li v-else="p==pageIndex" ><a @click="doGo(p)">{{p}}</a></li>
</ul>
</div>
</div>
</div>
<script>
new Vue({
el: '#app',
data: {
userList: null,//用户列表
//用于分页
pageIndex: 1,//当前页数
pageSize: 10,//每页显示的条数
pageTotle: 0,//总条数
pageNum: 0,//页数(分页器)
},
methods: {
//请求用户列表
requestUserList(p) {
//通过axios发送请求获取用户列表
axios.get("http://localhost:8080/user/list?pageIndex=" + p + "&pageSize=" + this.pageSize).then(response => {
console.log(response.data);
this.userList = response.data.data;//给用户列表赋值
this.pageTotle = response.data.pageTotle;//给总条数赋值
//计算页数,通过Math.ceil函数,小数取整,向上取整
this.pageNum = Math.ceil(this.pageTotle / this.pageSize);
});
},
//点击分页按钮
doGo(p){
this.pageIndex=p;
this.requestUserList(p);//调用请求用户列表的函数
},
//点击添加按钮时执行
doAdd() {
//页面跳转
window.parent.main_right.location.href="user_add_update.html";
},
//点击修改按钮时执行
doUpdate(id) {
window.parent.main_right.location.href="user_add_update.html?id="+id;
},
//点击删除按钮时执行
doDelete(id) {
//通过axios发送请求
axios.get("http://localhost:8080/user/remove?id="+id).then(response=>{
if (response.data.code==200){//删除成功
this.pageIndex=1;//返回第一页
this.requestUserList(this.pageIndex);//调用请求用户列表的函数
}else{//删除失败
alert(response.data.msg)
}
});
}
},
created: function () {
this.requestUserList(this.pageIndex);//调用请求用户列表的函数
}
});
</script>
</body>
</html>
删除功能当删除成功的时候直接返回到第一页
修改和增加功能
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<title>Title</title>
<link href="assets/bootstrap-3.3.7-dist/css/bootstrap.min.css" rel="stylesheet">
<link href="assets/css/right.css" rel="stylesheet">
<script src="assets/jquery-3.5.1.min.js"></script>
<script src="assets/bootstrap-3.3.7-dist/js/bootstrap.min.js"></script>
<script src="assets/vue.min-v2.5.16.js"></script>
<script src="assets/vue-router.min-2.7.0.js"></script>
<script src="assets/axios.min.js"></script>
<script src="assets/date_picker.js"></script>
</head>
<body>
<div id="app" class="container">
<div class="row">
<div class="col-md-8 col-md-offset-2">
<div class="row">
<div class="col-md-12"
style="text-align: center; font-weight: bold; font-size: 18px; height: 80px; line-height: 80px;">
{{title}}
</div>
</div>
<div class="row">
<div class="col-md-6 col-md-offset-3" style="height: 80px;">
<label>用户名</label>
<input type="text" class="form-control" v-model="userName">
</div>
</div>
<div class="row">
<div class="col-md-6 col-md-offset-3" style="height: 80px;">
<button class="btn btn-primary" @Click="doSave">保存</button>
<button class="btn btn-default" @Click="doCancel">取消</button>
</div>
</div>
</div>
</div>
</div>
<script>
new Vue({
el: '#app',
data: {
title: null,
userId: null,//接受页面跳转传参
userName: null,
passwd: null,
},
methods: {
//保存按钮
doSave() {
if (this.userId == null) {//添加
axios.post("http://localhost:8080/user/add", {
username: this.userName,
password: "12345",
}).then(response => {
if (response.data.code == 200) {//添加成功
//跳转到用户列表页面
window.parent.main_right.location.href="user_list.html";
} else {//添加失败
alert(response.data.msg);
}
});
} else {//修改
//通过axios发送请求,修改用户
axios.post("http://localhost:8080/user/update",{
id:this.userId,
username: this.userName,
password: this.passwd,
}).then(response => {
if (response.data.code == 200) {//添加成功
//跳转到用户列表页面
window.parent.main_right.location.href="user_list.html";
} else {//添加失败
alert(response.data.msg);
}
});
}
},
//取消按钮
doCancel() {
//返回上一个页面
history.go(-1);
},
},
created: function () {
//获取页面跳转传的id
var url = window.location.href;
if (url.indexOf("id") != -1) {//是否传递了id
this.userId = url.substring(url.indexOf("=") + 1);
}
console.log(this.userId);
if (this.userId == null) {//添加
this.title = "添加用户";
} else {//修改
this.title = "修改用户";
//发送请求获取用户信息,回显数据
axios.get("http://localhost:8080/user/info?id="+this.userId).then(response=>{
this.userName=response.data.data.username;
this.passwd=response.data.data.password;
});
}
}
});
</script>
</body>
</html>
先判断我们是点击的新增还是修改,之后当我们新增和修改取消的时候,我们直接返回上一级
效果图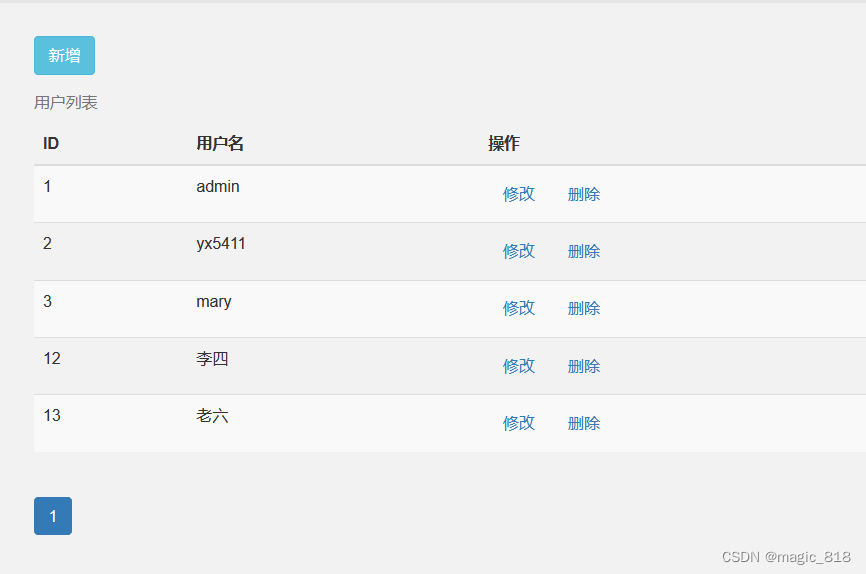