把数据库与文档联系起来,并实现通过编写代码进行数据的存入、显示、查找、修改、删除等的功能。
首先要新建一个Studentshujvbiao的类。
//Studentshijvbiao.h
#if !defined(AFX_STUDENTSHUJVBIAO_H__5156FC39_F34E_4428_82BC_9BFA9C3DC022__INCLUDED_)
#define AFX_STUDENTSHUJVBIAO_H__5156FC39_F34E_4428_82BC_9BFA9C3DC022__INCLUDED_
#if _MSC_VER > 1000
#pragma once
#endif // _MSC_VER > 1000
// Studentshujvbiao.h : header file
//
/
// Studentshujvbiao recordset
#include<afxdb.h>
class Studentshujvbiao : public CRecordset
{
public:
Studentshujvbiao(CDatabase* pDatabase = NULL);
DECLARE_DYNAMIC(Studentshujvbiao)
// Field/Param Data
//{{AFX_FIELD(Studentshujvbiao, CRecordset)
CString m_ID;
CString m_name;
CString m_chengji;
//}}AFX_FIELD
// Overrides
// ClassWizard generated virtual function overrides
//{{AFX_VIRTUAL(Studentshujvbiao)
public:
virtual CString GetDefaultConnect(); // Default connection string
virtual CString GetDefaultSQL(); // Default SQL for Recordset
virtual void DoFieldExchange(CFieldExchange* pFX); // RFX support
//}}AFX_VIRTUAL
// Implementation
#ifdef _DEBUG
virtual void AssertValid() const;
virtual void Dump(CDumpContext& dc) const;
#endif
};
//{{AFX_INSERT_LOCATION}}
// Microsoft Visual C++ will insert additional declarations immediately before the previous line.
#endif // !defined(AFX_STUDENTSHUJVBIAO_H__5156FC39_F34E_4428_82BC_9BFA9C3DC022__INCLUDED_)
//Studentshujvbiao.cpp
#include "stdafx.h"
#include "7.h"
#include "Studentshujvbiao.h"
#ifdef _DEBUG
#define new DEBUG_NEW
#undef THIS_FILE
static char THIS_FILE[] = __FILE__;
#endif
/
// Studentshujvbiao
IMPLEMENT_DYNAMIC(Studentshujvbiao, CRecordset)
Studentshujvbiao::Studentshujvbiao(CDatabase* pdb)
: CRecordset(pdb)
{
//{{AFX_FIELD_INIT(Studentshujvbiao)
m_ID = _T("");
m_name = _T("");
m_chengji = _T("");
m_nFields = 3;
//}}AFX_FIELD_INIT
m_nDefaultType = snapshot;
}
CString Studentshujvbiao::GetDefaultConnect()
{
return _T("ODBC;DSN=Mysource");
}
CString Studentshujvbiao::GetDefaultSQL()
{
return _T("[studenttable]");
}
void Studentshujvbiao::DoFieldExchange(CFieldExchange* pFX)
{
//{{AFX_FIELD_MAP(Studentshujvbiao)
pFX->SetFieldType(CFieldExchange::outputColumn);
RFX_Text(pFX, _T("[ID]"), m_ID);
RFX_Text(pFX, _T("[name]"), m_name);
RFX_Text(pFX, _T("[chengji]"), m_chengji);
//}}AFX_FIELD_MAP
}
/
// Studentshujvbiao diagnostics
#ifdef _DEBUG
void Studentshujvbiao::AssertValid() const
{
CRecordset::AssertValid();
}
void Studentshujvbiao::Dump(CDumpContext& dc) const
{
CRecordset::Dump(dc);
}
#endif //_DEBUG
//view.h
#if !defined(AFX_7VIEW_H__A7AFAC7A_5458_4331_BDD8_35946C1AA08D__INCLUDED_)
#define AFX_7VIEW_H__A7AFAC7A_5458_4331_BDD8_35946C1AA08D__INCLUDED_
#if _MSC_VER > 1000
#pragma once
#endif // _MSC_VER > 1000
#include "Studentshujvbiao.h"
class CMy7View : public CView
{
protected: // create from serialization only
CMy7View();
DECLARE_DYNCREATE(CMy7View)
// Attributes
public:
CMy7Doc* GetDocument();
// Operations
public:
// Overrides
// ClassWizard generated virtual function overrides
//{{AFX_VIRTUAL(CMy7View)
public:
virtual void OnDraw(CDC* pDC); // overridden to draw this view
virtual BOOL PreCreateWindow(CREATESTRUCT& cs);
protected:
virtual BOOL OnPreparePrinting(CPrintInfo* pInfo);
virtual void OnBeginPrinting(CDC* pDC, CPrintInfo* pInfo);
virtual void OnEndPrinting(CDC* pDC, CPrintInfo* pInfo);
//}}AFX_VIRTUAL
// Implementation
public:
virtual ~CMy7View();
#ifdef _DEBUG
virtual void AssertValid() const;
virtual void Dump(CDumpContext& dc) const;
#endif
protected:
// Generated message map functions
protected:
//{{AFX_MSG(CMy7View)
afx_msg void OnLButtonDown(UINT nFlags, CPoint point);
afx_msg void OnRButtonDown(UINT nFlags, CPoint point);
//}}AFX_MSG
DECLARE_MESSAGE_MAP()
};
#ifndef _DEBUG // debug version in 7View.cpp
inline CMy7Doc* CMy7View::GetDocument()
{ return (CMy7Doc*)m_pDocument; }
#endif
//view.cpp
#include "stdafx.h"
#include "7.h"
#include "7Doc.h"
#include "7View.h"
#ifdef _DEBUG
#define new DEBUG_NEW
#undef THIS_FILE
static char THIS_FILE[] = __FILE__;
#endif
/
// CMy7View
IMPLEMENT_DYNCREATE(CMy7View, CView)
BEGIN_MESSAGE_MAP(CMy7View, CView)
//{{AFX_MSG_MAP(CMy7View)
ON_WM_LBUTTONDOWN()
ON_WM_RBUTTONDOWN()
//}}AFX_MSG_MAP
// Standard printing commands
ON_COMMAND(ID_FILE_PRINT, CView::OnFilePrint)
ON_COMMAND(ID_FILE_PRINT_DIRECT, CView::OnFilePrint)
ON_COMMAND(ID_FILE_PRINT_PREVIEW, CView::OnFilePrintPreview)
END_MESSAGE_MAP()
/
// CMy7View construction/destruction
CMy7View::CMy7View()
{
// TODO: add construction code here
}
CMy7View::~CMy7View()
{
}
BOOL CMy7View::PreCreateWindow(CREATESTRUCT& cs)
{
// TODO: Modify the Window class or styles here by modifying
// the CREATESTRUCT cs
return CView::PreCreateWindow(cs);
}
/
// CMy7View drawing
void CMy7View::OnDraw(CDC* pDC)
{
CMy7Doc* pDoc = GetDocument();
ASSERT_VALID(pDoc);
}
/
// CMy7View printing
BOOL CMy7View::OnPreparePrinting(CPrintInfo* pInfo)
{
// default preparation
return DoPreparePrinting(pInfo);
}
void CMy7View::OnBeginPrinting(CDC* /*pDC*/, CPrintInfo* /*pInfo*/)
{
// TODO: add extra initialization before printing
}
void CMy7View::OnEndPrinting(CDC* /*pDC*/, CPrintInfo* /*pInfo*/)
{
// TODO: add cleanup after printing
}
/
// CMy7View diagnostics
#ifdef _DEBUG
void CMy7View::AssertValid() const
{
CView::AssertValid();
}
void CMy7View::Dump(CDumpContext& dc) const
{
CView::Dump(dc);
}
CMy7Doc* CMy7View::GetDocument() // non-debug version is inline
{
ASSERT(m_pDocument->IsKindOf(RUNTIME_CLASS(CMy7Doc)));
return (CMy7Doc*)m_pDocument;
}
#endif //_DEBUG
/
// CMy7View message handlers
void CMy7View::OnLButtonDown(UINT nFlags, CPoint point) //单击左键实现以下功能
{
// TODO: Add your message handler code here and/or call default
Studentshujvbiao s3; //存数据
s3.Open();
s3.AddNew();
s3.m_ID="003";
s3.m_name="小tu";
s3.m_chengji="100";
if(!s3.Update())
{
MessageBox("error");
}
s3.Close();
/*Studentshujvbiao s; //修改数据
s.Open();
s.m_strFilter="ID='001'";
s.Edit();
s.m_name="小驴";
s.Update();
s.Close();*/
CView::OnLButtonDown(nFlags, point);
}
void CMy7View::OnRButtonDown(UINT nFlags, CPoint point) //单击右键实现以下功能
{
// TODO: Add your message handler code here and/or call default
/*Studentshujvbiao s2; //显示数据
s2.Open();
CClientDC dc(this);
CString str;
int y=20;
while(!s2.IsEOF())
{
str.Format("ID:%s,name:%s,chengji:%s",s2.m_ID,s2.m_name,s2.m_chengji);
dc.TextOut(20,y,str);
y+=20;
s2.MoveNext();
}
s2.Close();*/
Studentshujvbiao st; //查找数据
int y=20;
st.Open();
CClientDC dc(this);
st.m_strFilter="chengji='90'";
while(!st.IsEOF())
{
CString str;
str.Format("ID:%s,name:%s,chengji:%s",st.m_ID,st.m_name,st.m_chengji);
dc.TextOut(20,y,str);
y+=20;
st.MoveNext();
}
st.Close();
/*Studentshujvbiao s2; //删除数据方法1
s2.Open();
while(!s2.IsEOF())
{
s2.Delete();
s2.MoveNext();
}
s2.Close();*/
/*CDatabase db; //删除数据方法2
Studentshujvbiao s(&db);
CString str="delete from studenttable";
s.Open();
db.ExecuteSQL(str);
s.Close();*/
CView::OnRButtonDown(nFlags, point);
}
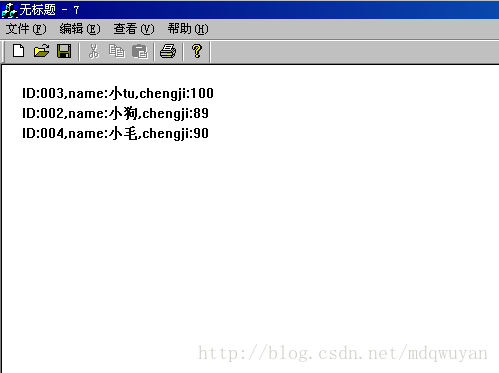