实现效果:
在手机号栏,输入手机号后点击获取验证码,等待片刻就会收到验证码,填写收到的验证码后点击验证注册,就可以实现注册功能。
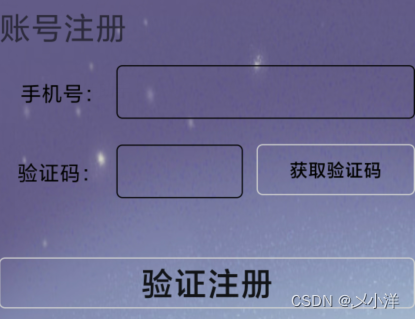
按照提示,输入手机号和验证码就可以实现登录功能。
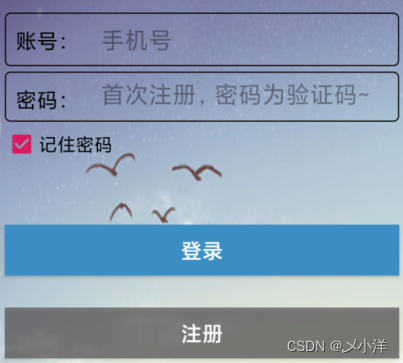
实现过程:
- 注册MobTech
在功能实现之前需要注册MobTech,用来接收和验证验证码,新用户注册有1000条免费额度。
注册MobTech
注册完成之后,在导航栏找到开发者服务下的SMSSDK。点击进入后开始使用。首先需要创建应用,应用LOGO以及应用名称随意填写。填写完后,选择SMSSDK服务,然后就是确认确认完成。打开我们刚创建的应用,我们会看到自动生成的App Key和App Secret,这个在后面配置的时候需要用到。不需要应用登记也可以使用。 - 添加配置
在项目Gradle的文件中注册MobSDK:
buildscript {
repositories {
maven {
url "https://mvn.mob.com/android"
}
...
}
dependencies {
...
classpath "com.mob.sdk:MobSDK:2018.0319.1724"
}
}
在项目App Module的Gradle文件中添加插件和扩展:
apply plugin: 'com.mob.sdk'
MobSDK {
appKey "您的appkey"
appSecret "您的appsecrt"
SMSSDK {
}
}
在gradle.properties中添加代码 :
MobSDK.spEdition=FP
在AndroidManifest.xml文件添加以下代码:
<uses-permission android:name="android.permission.READ_CONTACTS" />
<uses-permission android:name="android.permission.READ_PHONE_STATE" />
<uses-permission android:name="android.permission.WRITE_EXTERNAL_STORAGE" />
<uses-permission android:name="android.permission.ACCESS_NETWORK_STATE" />
<uses-permission android:name="android.permission.ACCESS_WIFI_STATE" />
<uses-permission android:name="android.permission.INTERNET" />
<uses-permission android:name="android.permission.RECEIVE_SMS" />
<uses-permission android:name="android.permission.GET_TASKS" />
<uses-permission android:name="android.permission.ACCESS_FINE_LOCATION" />
- 创建数据库
利用Sqlite数据库储存注册的账号和密码。
创建一个java类MyDBHelper继承SQLiteOpenHelper
public class MyDBHelper extends SQLiteOpenHelper {
private static final String DBNAME="输入你想创建的数据库名";
private static final int VERSION=1;
private SQLiteDatabase db;
public MyDBHelper(Context context) {
super(context, DBNAME, null, VERSION);
}
@Override
public void onCreate(SQLiteDatabase db) {
db.execSQL("create table yonghu(id integer primary key autoincrement,zhanghao varchar(10),mima varchar(15))");
}
@Override
public void onUpgrade(SQLiteDatabase db, int oldVersion, int newVersion) {
}
}
根据实现的效果需要创建三个Activity,分别为账号注册(register),登录(MainActivity)和登录进去之后的显示界面(login)。
- 创建register账号注册界面
首先创建一个空的Activity,命名为register,在生成的activity_register.xml文件做以下修改
<?xml version="1.0" encoding="utf-8"?>
<RelativeLayout xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:app="http://schemas.android.com/apk/res-auto"
xmlns:tools="http://schemas.android.com/tools"
android:layout_width="match_parent"
android:layout_height="match_parent"
android:background="选择你的背景图片"
tools:context=".register">
<TextView
android:id="@+id/textView1"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_alignParentStart="true"
android:layout_alignParentLeft="true"
android:layout_alignParentBottom="true"
android:layout_marginStart="20dp"
android:layout_marginLeft="20dp"
android:layout_marginBottom="615dp"
android:text="手机号:"
android:textColor="#000"
android:textSize="20dp" />
<EditText
android:id="@+id/phone"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_alignBaseline="@+id/textView1"
android:layout_alignParentStart="true"
android:layout_marginStart="111dp"
android:layout_marginLeft="10dp"
android:layout_toRightOf="@+id/textView1"
android:background="@drawable/shape"
android:ems="11"
android:inputType="phone"
android:maxLength="11"
android:padding="10dp">
<requestFocus />
</EditText>
<TextView
android:id="@+id/textView3"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_below="@+id/phone"
android:layout_alignLeft="@+id/textView1"
android:layout_marginLeft="-3dp"
android:layout_marginTop="37dp"
android:text="验证码:"
android:textColor="#000"
android:textSize="20dp" />
<EditText
android:id="@+id/cord"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_alignBaseline="@+id/textView3"
android:layout_alignLeft="@+id/phone"
android:layout_alignBottom="@+id/textView3"
android:layout_alignParentStart="true"
android:layout_marginStart="111dp"
android:layout_marginLeft="2dp"
android:layout_marginTop="20dp"
android:layout_marginBottom="-4dp"
android:background="设计你的EditText样式"
android:ems="4"
android:inputType="phone"
android:maxLength="6"
android:padding="10dp" />
<Button
android:id="@+id/getcord"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:layout_alignParentBottom="true"
android:background="@drawable/shape2"
android:layout_alignBottom="@+id/textView3"
android:layout_marginLeft="13dp"
android:layout_marginTop="-6dp"
android:layout_marginBottom="532dp"
android:layout_toRightOf="@+id/cord"
android:text="获取验证码"
android:textColor="#000"
android:visibility="visible" />
<Button
android:id="@+id/savecord"
android:layout_width="fill_parent"
android:layout_height="wrap_content"
android:layout_alignParentBottom="true"
android:layout_marginBottom="425dp"
android:background="设计你的Button样式"
android:textSize="25sp"
android:text="验证注册" />
<TextView
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_alignParentBottom="true"
android:layout_marginTop="10dp"
android:layout_marginBottom="670dp"
android:text="账号注册"
android:textSize="30dp" />
</RelativeLayout>
- 创建登录界面
登录界面是主界面Mactivity,在activity_main_xml做以下修改
<?xml version="1.0" encoding="utf-8"?>
<LinearLayout xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:app="http://schemas.android.com/apk/res-auto"
xmlns:tools="http://schemas.android.com/tools"
android:layout_width="match_parent"
android:layout_height="match_parent"
android:orientation="vertical"
android:background="选择你的背景图片"
tools:context=".MainActivity">
<TextView
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_marginStart="75dp"
android:layout_marginTop="100dp"
android:padding="10dp"
android:text="这是登录界面"
android:textColor="#000000"
android:textSize="40dp" />
<LinearLayout
android:id="@+id/number"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:layout_marginBottom="5dp"
android:layout_marginLeft="10dp"
android:layout_marginRight="10dp"
android:layout_marginTop="100dp"
android:background="设计的样式"
android:orientation="horizontal">
<TextView
android:id="@+id/tv_number"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:padding="10dp"
android:text="账号:"
android:textColor="#000"
android:textSize="20dp" />
<EditText
android:id="@+id/et_zhanghao"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:background="@null"
android:layout_marginLeft="5dp"
android:hint="手机号"
android:padding="10dp" >
</EditText>
</LinearLayout>
<LinearLayout
android:id="@+id/password"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:layout_below="@+id/number"
android:layout_centerVertical="true"
android:layout_marginLeft="10dp"
android:layout_marginRight="10dp"
android:background="设计的样式">
<TextView
android:id="@+id/tv_password"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:padding="10dp"
android:text="密码:"
android:textSize="20dp"
android:textColor="#000" />
<EditText
android:id="@+id/et_mima"
android:layout_width="match_parent"
android:layout_height="46dp"
android:layout_marginLeft="5dp"
android:layout_toRightOf="@id/tv_password"
android:hint="首次注册,密码为验证码~"
android:background="@null"
android:inputType="textPassword"
android:padding="10dp" />
</LinearLayout>
<CheckBox
android:checked="true"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="记住密码"
android:id="@+id/checkBox"
android:layout_marginLeft="10dp"
android:layout_marginTop="5dp"/>
<Button
android:id="@+id/bt_denglu"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:layout_below="@id/password"
android:layout_marginLeft="10dp"
android:layout_marginRight="10dp"
android:layout_marginTop="60dp"
android:background="#3c8dc4"
android:text="登录"
android:textColor="#ffffff"
android:textSize="20dp" />
<Button
android:id="@+id/bt_zhuce"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:layout_below="@id/bt_denglu"
android:layout_marginLeft="10dp"
android:layout_marginRight="10dp"
android:layout_marginTop="30dp"
android:background="#b7585556"
android:text="注册"
android:textColor="#ffffff"
android:textSize="20dp" />
</LinearLayout>
- 登录成功后的界面
创建一个空的Activity即可,这里创建的Activity命名为login。
- java代码
通过以上操作,界面已设计好,接下来就是实现代码。
创建一个java类User
public class User {
private static String zhanghao;
private static String mima;
public static String getZhanghao() {
return zhanghao;
}
public void setZhanghao(String zhanghao) {
this.zhanghao = zhanghao;
}
public static String getMima() {
return mima;
}
public void setMima(String mima) {
this.mima = mima;
}
public User(String zhanghao,String mima){
super();
this.zhanghao = zhanghao;
this.mima = mima;
}
}
创建一个用来检测登录的java类UserService
public class UserService {
private MyDBHelper dbHelper;
public UserService(Context context){
dbHelper=new MyDBHelper(context);
}
public boolean login(String zhanghao,String mima){
SQLiteDatabase sdb=dbHelper.getReadableDatabase();
String sql="select * from yonghu where zhanghao=? and mima=?";
Cursor cursor=sdb.rawQuery(sql, new String[]{zhanghao,mima});
if(cursor.moveToFirst()==true){
cursor.close();
return true;
}
return false;
}
}
在register.java做以下修改,以实现验证码验证注册
public class register extends AppCompatActivity {
EditText phone;
EditText cord;
Button getCord;
Button saveCord;
MyDBHelper mhelper;
SQLiteDatabase db;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_register);
init();
MobSDK.submitPolicyGrantResult(true, null);
btnOnClick();
}
public void regist(){
EventHandler eh = new EventHandler() {
@Override
public void afterEvent(int event, int result, Object data) {
if (result == SMSSDK.RESULT_COMPLETE) {
if (event == SMSSDK.EVENT_SUBMIT_VERIFICATION_CODE) {
runOnUiThread(new Runnable() {
@Override
public void run() {
ContentValues values=new ContentValues();
values.put("zhanghao",phone.getText().toString());
values.put("mima",cord.getText().toString());
db.insert("yonghu",null,values);
Toast.makeText(getApplication(), "您已验证成功,注册成功,请登录~~", Toast.LENGTH_SHORT).show();
Intent intent = new Intent(register.this,MainActivity.class);
startActivity(intent);
finish();
}
});
} else if (event == SMSSDK.EVENT_GET_VERIFICATION_CODE) {
runOnUiThread(new Runnable() {
@Override
public void run() {
Toast.makeText(getApplication(), "已发送验证码,请注意查收", Toast.LENGTH_SHORT).show();
}
});
} else if (event == SMSSDK.EVENT_GET_SUPPORTED_COUNTRIES) {}
} else {
runOnUiThread(new Runnable() {
@Override
public void run() {
Toast.makeText(getApplication(), "操作失败,重新获取验证码", Toast.LENGTH_SHORT).show();
}
});
((Throwable) data).printStackTrace();
}
}
};
SMSSDK.registerEventHandler(eh);
}
private void btnOnClick() {
getCord.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View view) {
regist();
String a = phone.getText().toString().trim();
if (TextUtils.isEmpty(a))
{
Toast.makeText(getApplicationContext(), "输入的手机号不能为空",Toast.LENGTH_LONG).show();
} else {
SMSSDK.getVerificationCode("86", a);
}
}
});
saveCord.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View view) {
String phoneNumber = phone.getText().toString().trim();
String code = cord.getText().toString().trim();
if (TextUtils.isEmpty(phoneNumber) && TextUtils.isEmpty(code)) {
Toast.makeText(getApplicationContext(), "请检验您输入的信息", Toast.LENGTH_LONG).show();
}
else
{
SMSSDK.submitVerificationCode("86",phoneNumber,code);
}
}
});
}
private void init() {
phone = (EditText) findViewById(R.id.phone);
cord = (EditText) findViewById(R.id.cord);
getCord = (Button) findViewById(R.id.getcord);
saveCord = (Button) findViewById(R.id.savecord);
mhelper=new MyDBHelper(register.this);
db=mhelper.getWritableDatabase();
}
}
在MainActivity.java做以下修改,以实现登录功能
public class MainActivity extends AppCompatActivity {
EditText et_zhanghao,et_mima;
Button bt_denglu,bt_zhuce;
SharedPreferences preferences;
SharedPreferences.Editor editor;
CheckBox remember;
MyDBHelper mhelper;
SQLiteDatabase db;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
init();
preferences= PreferenceManager.getDefaultSharedPreferences(this);
boolean isRemember=preferences.getBoolean("remember_password",false);
if (isRemember){
String account=preferences.getString("account","");
String password=preferences.getString("password","");
et_zhanghao.setText(account);
et_mima.setText(password);
remember.setChecked(true);
}
bt_onclick();
}
private void bt_onclick() {
bt_zhuce.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View view) {
Intent intent = new Intent(MainActivity.this,register.class);
startActivity(intent);
}
});
bt_denglu.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View view) {
String zhanghao = et_zhanghao.getText().toString().trim();
String mima = et_mima.getText().toString().trim();
if (!TextUtils.isEmpty(zhanghao) && !TextUtils.isEmpty(mima)) {
UserService uService=new UserService(MainActivity.this);
boolean flag=uService.login(zhanghao, mima);
if(flag){
Toast.makeText(MainActivity.this, "登录成功", Toast.LENGTH_LONG).show();
editor=preferences.edit();
if (remember.isChecked()){
editor.putBoolean("remember_password",true);
editor.putString("account", zhanghao);
editor.putString("password",mima);
}else{
editor.clear();
}
editor.apply();
Intent intent = new Intent(MainActivity.this,login.class);
intent.putExtra("zh",zhanghao);
startActivity(intent);
finish();
}else{
Toast.makeText(MainActivity.this, "密码或者账号错误,请重新登录", Toast.LENGTH_LONG).show();
}
} else{ Toast.makeText(MainActivity.this, "账号或者密码不能为空" , Toast.LENGTH_SHORT).show();}
}
});
}
private void init() {
et_zhanghao = findViewById(R.id.et_zhanghao);
et_mima = findViewById(R.id.et_mima);
bt_denglu = findViewById(R.id.bt_denglu);
bt_zhuce = findViewById(R.id.bt_zhuce);
remember=(CheckBox) findViewById(R.id.checkBox);
mhelper=new MyDBHelper(MainActivity.this);
db=mhelper.getWritableDatabase();
}
}
修改完之后,本次Android Studio实现短信验证码注册/登录功能就已经实现了。
实现过程中用拼音当各种控件的ID和数据库的表名属性名,希望大家不要像我学习!!
由于第一次发CSDN,还不够熟练使用其编辑功能,编辑模式不要在意~ 程序中若有错误的地方,希望大家寄给我指正批评!!
还有就是希望给个赞,以提高我的创作动力,谢谢大家啦~~~