(javaEE的SSM框架maven项目入门简单图书管理-新手期末复习
#本项目仅用于新手参考和入门参考,实现超级简单的登录注册和图书的查找差选删改的功能,大牛请绕道,第一篇文章献给了java(前端人的遗憾)
小小的导航
1.配置文件
1. jdbc.properties
jdbc.driver=com.mysql.jdbc.Driver
jdbc.url=jdbc:mysql://localhost:3306/test
jdbc.username=root
jdbc.password=123456
jdbc.maxTotal=30
jdbc.maxIdle=10
jdbc.initialSize=5
2.mybatis-config.xml
<?xml version="1.0" encoding="UTF-8" standalone="no"?>
<!DOCTYPE configuration PUBLIC "-//mybatis.org//DTD Config 3.0//EN"
"http://mybatis.org/dtd/mybatis-3-config.dtd">
<configuration>
<typeAliases>
<package name="com.lsnu.demo.domain"></package>
</typeAliases>
<mappers>
<package name="com.lsnu.demo.mapper"></package>
</mappers>
</configuration>
3.log4j.properties
# Global logging configuration
log4j.rootLogger=ERROR, stdout
# MyBatis logging configuration...
log4j.logger.com.lsnu.cross.mapper=DEBUG #此处的应该改为对应的mapper文件的路径
# Console output...
log4j.appender.stdout=org.apache.log4j.ConsoleAppender
log4j.appender.stdout.layout=org.apache.log4j.PatternLayout
log4j.appender.stdout.layout.ConversionPattern=%5p [%t] - %m%n
4.springmvc-config.xml
<?xml version="1.0" encoding="UTF-8"?>
<beans xmlns="http://www.springframework.org/schema/beans"
xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xmlns:mvc="http://www.springframework.org/schema/mvc"
xmlns:context="http://www.springframework.org/schema/context"
xsi:schemaLocation="http://www.springframework.org/schema/beans
http://www.springframework.org/schema/beans/spring-beans-4.3.xsd
http://www.springframework.org/schema/mvc
http://www.springframework.org/schema/mvc/spring-mvc-4.3.xsd
http://www.springframework.org/schema/context
http://www.springframework.org/schema/context/spring-context-4.3.xsd">
<!--controller注解-->
<context:component-scan base-package="demo.controller"></context:component-scan>
<!-- Spring MVC注解驱动支持 -->
<mvc:annotation-driven></mvc:annotation-driven>
<!-- 前端视图解析器的前缀目录和后缀文件名配置 -->
<bean class="org.springframework.web.servlet.view.InternalResourceViewResolver">
<property name="prefix" value="/WEB-INF/jsp"></property>
<property name="suffix" value=".jsp"></property>
</bean>
<!--配置静态资源的访问映射,此配置中的文件,将不被前端控制器拦截 -->
<mvc:resources mapping="/static/**" location="/static/"></mvc:resources>
<!--解决乱码-->
<bean class="org.springframework.web.multipart.commons.CommonsMultipartResolver">
<property name="defaultEncoding" value="UTF-8"></property>
<property name="maxUploadSize" value="1048576600"></property>
</bean>
<!--拦截器-->
</beans>
5.applicationContext.xml
<?xml version="1.0" encoding="UTF-8"?>
<beans xmlns="http://www.springframework.org/schema/beans"
xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xmlns:aop="http://www.springframework.org/schema/aop"
xmlns:tx="http://www.springframework.org/schema/tx"
xmlns:context="http://www.springframework.org/schema/context"
xsi:schemaLocation="http://www.springframework.org/schema/beans
http://www.springframework.org/schema/beans/spring-beans-4.3.xsd
http://www.springframework.org/schema/tx
http://www.springframework.org/schema/tx/spring-tx-4.3.xsd
http://www.springframework.org/schema/context
http://www.springframework.org/schema/context/spring-context-4.3.xsd
http://www.springframework.org/schema/aop
http://www.springframework.org/schema/aop/spring-aop-4.3.xsd">
<!--读取db.properties-->
<context:property-placeholder location="classpath:db.properties"></context:property-placeholder>
<!--配置数据源-->
<bean id="dataSource" class="org.apache.commons.dbcp2.BasicDataSource">
<!--数据可驱动-->
<property name="driverClassName" value="com.mysql.jdbc.Driver"></property>
<!--链接url-->
<property name="url" value="jdbc:mysql://localhost:3306/test"></property>
<!--连接用户名-->
<property name="username" value="root"></property>
<!--连接mima-->
<property name="password" value="123456"></property>
<!--连接zuidashu-->
<property name="maxTotal" value="30"></property>
<!--连接最大空闲-->
<property name="maxIdle" value="10"></property>
<!--连接初始化连接数-->
<property name="initialSize" value="5"></property>
</bean>
<!--事务管理依赖于数据源-->
<bean id="transactionManager" class="org.springframework.jdbc.datasource.DataSourceTransactionManager">
<!--注入数据源-->
<property name="dataSource" ref="dataSource"></property>
</bean>
<!-- 开启事务注解 -->
<tx:annotation-driven transaction-manager="transactionManager"></tx:annotation-driven>
<!-- 配置mybatis工厂SqlSessionFactory -->
<bean id="sqlSessionFactory" class="org.mybatis.spring.SqlSessionFactoryBean">
<!--注入数据源-->
<property name="dataSource" ref="dataSource"></property>
<!--指定mybatis文件-->
<property name="configLocation" value="classpath:mybatis-config.xml"></property>
</bean>
<!-- 配置mapper扫描器 -->
<bean id="mapperScannerConfigurer" class="org.mybatis.spring.mapper.MapperScannerConfigurer">
<property name="basePackage" value="com.lsnu.demo.mapper"></property>
</bean>
<!-- 扫描Service -->
<context:component-scan base-package="demo.service"></context:component-scan>
</beans>
2.Main文件
目录结构
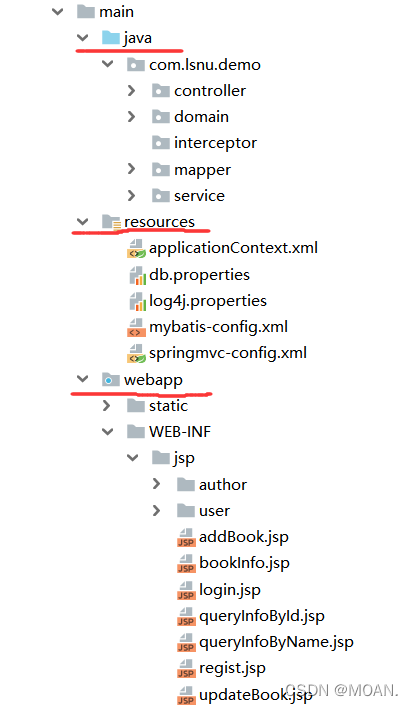
开始写java文件下的代码(jaava下面的com.lsnu.demo文件名根据项目的需求进行更改)
1.首先写domain(相当于pojo)文件
先在domain中建立所需要的实体类,创建对应的class文件,例如这个简单的图书管理项目,需要用到User和Book两个实体类
User
getter、setter,ToString和有参构造无参构造方法可以通过generate(IDEA)来一键生成
package com.lsnu.demo.domain;
import lombok.Data;
import lombok.ToString;
@Data
@ToString
public class User {
private Integer userId;
private String userName;
private String password;
private String email;
public User() {
super();
}
public User(Integer userId, String userName, String password, String email) {
this.userId = userId;
this.userName = userName;
this.password = password;
this.email = email;
}
public Integer getUserId() {
return userId;
}
public void setUserId(Integer userId) {
this.userId = userId;
}
public String getUserName() {
return userName;
}
public void setUserName(String userName) {
this.userName = userName;
}
public String getPassword() {
return password;
}
public void setPassword(String password) {
this.password = password;
}
public String getEmail() {
return email;
}
public void setEmail(String email) {
this.email = email;
}
@Override
public String toString() {
return "User{" +
"userId=" + userId +
", userName='" + userName + '\'' +
", password='" + password + '\'' +
", email='" + email + '\'' +
'}';
}
}
Book
这边建议亲亲一个功能一个功能的实现
package com.lsnu.demo.domain;
import lombok.Data;
import lombok.ToString;
@Data
@ToString
public class Book {
private int bookid;
private String bookname;
private double price;
public int getBookid() {
return bookid;
}
public void setBookid(int bookid) {
this.bookid = bookid;
}
public String getBookname() {
return bookname;
}
public void setBookname(String bookname) {
this.bookname = bookname;
}
public double getPrice() {
return price;
}
public void setPrice(double price) {
this.price = price;
}
public Book(int bookid, String bookname, double price) {
this.bookid = bookid;
this.bookname = bookname;
this.price = price;
}
@Override
public String toString() {
return "Book{" +
"bookid=" + bookid +
", bookname='" + bookname + '\'' +
", price=" + price +
'}';
}
}
2.接下来写mapper中的代码
首先mapper我自己理解的是可以对数据库进行数据的操作,所有每个实体类对应的mapper文件有一个Iterface和xml文件构成,接口文件中写的是需要的方法,xml文件中写的是对数据库的操作
UserMapper
package com.lsnu.demo.mapper;
import com.lsnu.demo.domain.User;
import org.apache.ibatis.annotations.Param;
import org.springframework.stereotype.Repository;
import java.util.List;
public interface UserMapper {
User login(String username,String password);
int register(User user);
}
UserMapper.xml
<?xml version="1.0" encoding="UTF-8" standalone="no"?>
<!DOCTYPE mapper PUBLIC "-//mybatis.org//DTD Mapper 3.0//EN" "http://mybatis.org/dtd/mybatis-3-mapper.dtd">
<mapper namespace="com.lsnu.demo.mapper.UserMapper">
<select id="login" parameterType="String" >
select * from user where user_name = #{userName} and password = #{password}
</select>
<insert id="register" parameterType="com.lsnu.demo.domain.User">
insert into user(userId,user_name,password) values (#{userId},#{userName},#{password})
</insert>
</mapper>
3.BookMapper
package com.lsnu.demo.mapper;
import com.lsnu.demo.domain.Book;
import org.apache.ibatis.session.RowBounds;
import org.springframework.stereotype.Repository;
import java.util.List;
@Repository
public interface BookMapper {
// 查找所有书
// 按照id查找
// 按照名字查找
// 添加
// 删除
// 更新
public List<Book> getAllBooks();
public List<Book> queryByBookid(int bookid);
public List<Book> queryByBookname(String bookname);
public int addBook(Book book);
public int delBook(int bookid);
public int updateBook(Book book);
public int save(Book book);
}
BookMapper.xml
<?xml version="1.0" encoding="UTF-8" standalone="no"?>
<!DOCTYPE mapper
PUBLIC "-//mybatis.org//DTD Mapper 3.0//EN"
"http://mybatis.org/dtd/mybatis-3-mapper.dtd">
<mapper namespace="com.lsnu.demo.mapper.BookMapper">
<!--查询所有书籍-->
<select id="findAllBooks" resultType="com.lsnu.demo.domain.Book">
select * from book
</select>
<!--通过id查询-->
<select id="queryByBookid" resultType="com.lsnu.demo.domain.Book" parameterType="Integer" >
select * from book where bookid=#{bookid}
</select>
<!--通过书名模糊查询-->
<select id="queryByBookid" resultType="com.lsnu.demo.domain.Book" parameterType="String" >
select * from book where bookname like concat('%',#{bookname},'%')
</select>
<insert id="addBook" parameterType="com.lsnu.demo.domain.Book" >
insert into book(bookname,price) values (#{bookname},#{price})
</insert>
<delete id="delBook" parameterType="Integer">
delete from book where bookid=#{bookid}
</delete>
<update id="updateBook" parameterType="com.lsnu.demo.domain.Book" >
update book set bookname=#{bookname},pirce = #{price} where bookid = #{bookid}
</update>
<insert id="save" parameterType="Book" >
insert into book(bookname,price) values (#{bookname},#{price})
</insert>
</mapper>
4.再写Service层
Service中每个实体类也对应了一个接口文件和一个实现类
UserService(Iterface)
package com.lsnu.demo.service;
import com.lsnu.demo.domain.User;
public interface UserService {
User login(String username,String password);
int register(User user);
}
UserMapperImpl(class)
package com.lsnu.demo.service;
import com.lsnu.demo.domain.Book;
import com.lsnu.demo.mapper.BookMapper;
import org.apache.ibatis.session.RowBounds;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.stereotype.Service;
import org.springframework.transaction.annotation.Transactional;
import java.util.List;
@Service
@Transactional
public class BookServiceImpl implements BookService{
@Autowired
BookMapper bookMapper;
@Override
public List<Book> getAllBooks() {
return bookMapper.getAllBooks();
}
@Override
public List<Book> queryByBookid(int bookid) {
return bookMapper.queryByBookid(bookid);
}
@Override
public List<Book> queryByBookname(String bookname) {
return bookMapper.queryByBookname(bookname);
}
@Override
public int addBook(Book book) {
return bookMapper.addBook(book);
}
@Override
public int delBook(int bookid) {
return bookMapper.delBook(bookid);
}
@Override
public int updateBook(Book book) {
return bookMapper.updateBook(book);
}
@Override
public List<Book> selectAuthors() {
return bookMapper.selectAuthors();
}
@Override
public List<Book> selectAuthors(RowBounds rowBounds) {
return bookMapper.selectAuthors(rowBounds);
}
BookService(Iterface)
package com.lsnu.demo.service;
import com.lsnu.demo.domain.Book;
import org.apache.ibatis.session.RowBounds;
import java.util.List;
public interface BookService {
public List<Book> getAllBooks();
public List<Book> queryByBookid(int bookid);
public List<Book> queryByBookname(String bookname);
public int addBook(Book book);
public int delBook(int bookid);
public int updateBook(Book book);
List<Book> selectAuthors();
List<Book> selectAuthors(RowBounds rowBounds);
int getCount();
}
BookServiceImpl(class)
package com.lsnu.demo.service;
import com.lsnu.demo.service.Page;
import com.lsnu.demo.domain.Book;
import com.lsnu.demo.mapper.BookMapper;
import org.apache.ibatis.session.RowBounds;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.stereotype.Service;
import org.springframework.transaction.annotation.Transactional;
import java.util.List;
@Service
@Transactional
public class BookServiceImpl implements BookService{
@Autowired
BookMapper bookMapper;
@Override
public List<Book> getAllBooks() {
return bookMapper.getAllBooks();
}
@Override
public List<Book> queryByBookid(int bookid) {
return bookMapper.queryByBookid(bookid);
}
@Override
public List<Book> queryByBookname(String bookname) {
return bookMapper.queryByBookname(bookname);
}
@Override
public int addBook(Book book) {
return bookMapper.addBook(book);
}
@Override
public int delBook(int bookid) {
return bookMapper.delBook(bookid);
}
@Override
public int updateBook(Book book) {
return bookMapper.updateBook(book);
}
@Override
public List<Book> selectAuthors() {
return bookMapper.selectAuthors();
}
@Override
public List<Book> selectAuthors(RowBounds rowBounds) {
return bookMapper.selectAuthors(rowBounds);
}
@Override
public int getCount() {
return 0;
}
public Page<Book> findPage(Integer pageNo,Integer pageSize){
int count = bookMapper.getCount();
if(pageNo == null || pageNo <= 1)
pageNo=1;
if(pageSize==null)
pageSize=5;
int pageCount = Integer.parseInt(String.valueOf(count/pageSize));
if(pageCount<pageNo)
pageNo=pageCount;
Integer start = (pageNo-1)*pageSize;
if(start<0)
start=0;
RowBounds rowBounds = new RowBounds(start,pageSize);
List<Book> books=bookMapper.selectAuthors(rowBounds);
Page<Book> page = new Page<Book>();
page.setPageNo(pageNo);
page.setResults(books);
page.setTotal(count);
return page;
}
}
5.Controller层
UserController
package com.lsnu.demo.controller;
import com.lsnu.demo.domain.User;
import com.lsnu.demo.service.UserService;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.stereotype.Controller;
import org.springframework.ui.Model;
import org.springframework.web.bind.annotation.RequestMapping;
import javax.jws.WebParam;
import javax.servlet.http.HttpSession;
@Controller
@RequestMapping("/user")
public class userController {
@Autowired
UserService userService;
@RequestMapping("/login")
public String login(User user, Model model, HttpSession session){
//获取用户名和密码
String username =user.getUserName();
String userPassword=user.getPassword();
//判断
if(username != null && userPassword != null){
User user1 = userService.login(username,userPassword);
session.setAttribute("user",user1.getUserName());
return "redirect:/book/getAllBook";
}
model.addAttribute("msg","用户名或者密码为空");
return "redirect:/book/getAllBook";
}
@RequestMapping("/register")
public String register(User u, Model model){
int result = userService.register(u);
model.addAttribute("msg","注册成功");
return "login";
}
}
BookController
package com.lsnu.demo.controller;
import com.github.pagehelper.PageHelper;
import com.github.pagehelper.PageInfo;
import com.lsnu.demo.domain.Book;
import com.lsnu.demo.service.BookService;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.stereotype.Controller;
import org.springframework.ui.Model;
import org.springframework.web.bind.annotation.RequestMapping;
import org.springframework.web.bind.annotation.RequestParam;
import java.util.List;
@Controller
@RequestMapping("/book")
public class BookController {
@Autowired
BookService bookService;
@RequestMapping("/getAllBooks")
public String findAllBooks(Model model, @RequestParam(defaultValue = "1") int pageNo,@RequestParam(defaultValue = "5")int pageSize){
PageHelper.startPage(pageNo,pageSize);
List<Book> book = bookService.getAllBooks();
PageInfo<Book> p = new PageInfo<Book>(book);
model.addAttribute( "booklist",book);
model.addAttribute("pageInfo",p);
return "bookInfo";
}
@RequestMapping("/queryById")
public String queryById(int bookid,Model model, @RequestParam(defaultValue = "1") int pageNum,@RequestParam(defaultValue = "5")int pageSize){
if(pageNum == 0)
pageNum = 1;
PageHelper.startPage(pageNum,pageSize);
List<Book> book = bookService.queryByBookid(bookid);
PageInfo<Book> p = new PageInfo<Book>(book);
model.addAttribute( "booklist",book);
model.addAttribute("pageInfo",p);
model.addAttribute("bookid",bookid);
return "queryInfoById";
}
@RequestMapping("/queryByNmme")
public String queryByName(String name,Model model, @RequestParam(defaultValue = "1") int pageNo,@RequestParam(defaultValue = "5")int pageSize){
if(pageNo == 0)
pageNo = 1;
PageHelper.startPage(pageNo,pageSize);
List<Book> book = bookService.queryByBookname(name);
PageInfo<Book> p = new PageInfo<Book>(book);
model.addAttribute( "booklist",book);
model.addAttribute("pageInfo",p);
model.addAttribute("bookname",name);
return "queryInfoByName";
}
@RequestMapping("/delBook")
public String delBook(int bookid,Model model){
int res = bookService.delBook(bookid);
if(res > 0){
return "forword:findAllBooks";
}else {
model.addAttribute("msg","删除失败!");
return "bookInfo";
}
}
@RequestMapping("/toAddBook")
public String toAddBook(){return "addBook";};
@RequestMapping("/addBook")
public String addBook(Book book,Model model){
int res = bookService.addBook(book);
if(res > 0){
return "forword:findAllBooks";
}else {
model.addAttribute("msg","添加失败!");
return "bookInfo";
}
}
@RequestMapping("/toupdateBook")
public String toUpdateBook(){return "updateBook";};
@RequestMapping("/updateBook")
public String updateBook(Book book,Model model){
int res = bookService.updateBook(book);
if(res > 0){
return "forword:findAllBooks";
}else {
model.addAttribute("msg","更新失败!");
return "bookInfo";
}
}
}
3.Jsp页面
最后再写上jsp文件就可以了,再WEB-INF下面创建两个文件夹,用来存放jsp文件和引入的jq和bootstrap文件
1.login.jsp
不要忘记引入外部的css样式和js文件 可以使用cdn链接或者本地导入 这个项目的css样式是从bootstrap官网导入的
<%@ page contentType="text/html;charset=UTF-8" language="java" %>
<%@taglib prefix="c" uri="http://java.sun.com/jsp/jstl/core" %>
<%@taglib prefix="fmt" uri="http://java.sun.com/jsp/jstl/fmt"%>
<html>
<head>
<link rel="${pageContext.request.contextPath}/static/css/bootstrap.css">
<title>用户登录</title>
</head>
<body>
<form action="${pageContext.request.contextPath}/user/login" method="post">
<div class="form-group">
<label for="exampleInputEmail1">用户名</label>
<input type="text" class="form-control" id="exampleInputEmail1" aria-describedby="emailHelp" name="username">
</div>
<div class="form-group">
<label for="exampleInputPassword1">密码</label>
<input type="password" class="form-control" id="exampleInputPassword1">
</div>
<button type="submit" class="btn btn-primary">登陆</button>
<a class="btn btn-primary" href="#" role="button" id="regist">注册</a>
</form>
</body>
<script src="${pageContext.request.contextPath}/static/js/bootstrap.js"></script>
<script src="${pageContext.request.contextPath}/static/js/jquery-1.11.3.min.js"></script>
<script>
var regis = document.querySelector("#regist");
regis.addEventListener("click",function (evt) {
window.location.href="regist.jsp"
})
</script>
</html>
2.regist.jsp
和login差不多
<%@ page contentType="text/html;charset=UTF-8" language="java" %>
<%@taglib prefix="c" uri="http://java.sun.com/jsp/jstl/core" %>
<%@taglib prefix="fmt" uri="http://java.sun.com/jsp/jstl/fmt"%>
<html>
<head>
<link rel="${pageContext.request.contextPath}/static/css/bootstrap.css">
<title>用户注册</title>
</head>
<body>
<form action="${pageContext.request.contextPath}/user/register" method="post">
<div class="form-group">
<label for="exampleInputEmail1">用户名</label>
<input type="text" class="form-control" id="exampleInputEmail1" aria-describedby="emailHelp" name="username">
</div>
<div class="form-group">
<label for="exampleInputPassword1">密码</label>
<input type="password" class="form-control" id="exampleInputPassword1">
</div>
<button type="submit" class="btn btn-primary" id="login">登陆</button>
<a class="btn btn-primary" href="#" role="button" id="regist">注册</a>
</form>
</body>
<script src="${pageContext.request.contextPath}/static/css/bootstrap.js"></script>
<script src="${pageContext.request.contextPath}/static/css/jquery-1.11.3.min.js"></script>
<script>
var login = document.querySelector("#login");
login.addEventListener("click",function (evt) {
window.location.href="login.jsp"
})
</script>
</html>
3.bookInfo.jsp
<%@ page contentType="text/html;charset=UTF-8" language="java" %>
<%@taglib prefix="c" uri="http://java.sun.com/jsp/jstl/core" %>
<%@taglib prefix="fmt" uri="http://java.sun.com/jsp/jstl/fmt" %>
<html>
<head>
<link rel="${pageContext.request.contextPath}/static/css/bootstrap.css">
<title>Title</title>
</head>
<body>
欢迎你,<%=session.getAttribute("user")%>>
${msg}
<center>
<form method="post" action="${pageContext.request.contextPath}/book/queryByNmme">
<input type="text" name="name" placeholder="请输入关键字查询"/>
<input type="submit" value="提交"/>
<a href="${pageContext.request.contextPath}/book/toAddBook">添加</a>
</form><br>
<form method="post" action="${pageContext.request.contextPath}/book/queryById">
<input type="text" name="bookid" placeholder="请输入书编号查询"/>
<input type="submit" value="提交"/>
</form><br>
<table border="1px" width="500px">
<tr>
<th>编号</th>
<th>书名</th>
<th>价格</th>
<th>操作</th>
</tr>
<c:choose>
<c:when test="${ empty booklist} " >
<tr><td>没有查到相关信息!</td></tr>
</c:when>
<c:otherwise>
<c:forEach items="${booklist}" var="x">
<tr>
<td style="text-align: center;">${x.bookid}</td>
<td style="text-align: center;">${x.name}</td>
<td style="text-align: center;">${x.price}</td>
<td style="text-align: center;">
<a href="${pageContext.request.contextPath}/book/toupdateBook">修改</a>/
<a href="${pageContext.request.contextPath}/book/delBook?bookid=${x.bookid}">删除</a></td>
</tr>
</c:forEach>
</c:otherwise>
</c:choose>
</table>
第${pageinfo.pageNum }页/共${pageinfo.pages}页<br>
<a href="${pageContext.request.contextPath }/book/getAllBook?pageNum=${pageinfo.prePage}">上一页</a>
<c:forEach begin="1" end="${pageinfo.pages}" step="1" var="y" items="pageInfo">
<a href="${pageContext.request.contextPath }/book/getAllBook?pageNum=${y}">${y}</a>
</c:forEach>
<a href="${pageContext.request.contextPath }/book/getAllBook?pageNum=${pageinfo.nextPage}">下一页</a>
</center>
</body>
<script src="${pageContext.request.contextPath}/static/js/bootstrap.js"></script>
<script src="${pageContext.request.contextPath}/static/js/jquery-1.11.3.min.js"></script>
</html>
4.addBook.jsp
<%@ page contentType="text/html;charset=UTF-8" language="java" %>
<%@taglib prefix="c" uri="http://java.sun.com/jsp/jstl/core" %>
<%@taglib prefix="fmt" uri="http://java.sun.com/jsp/jstl/fmt" %>
<html>
<head>
<link rel="${pageContext.request.contextPath}/static/css/bootstrap.css">
<title>添加书籍</title>
</head>
<body>
<form action="${pageContext.request.contextPath}/book/addBook" method="post">
书名:<input type="text" name="name" /><br>
价格:<input type="text" name="price" /><br><br>
<input type="submit" value="提交" /><br>
</form>
</body>
<script src="${pageContext.request.contextPath}/static/js/bootstrap.js"></script>
<script src="${pageContext.request.contextPath}/static/js/jquery-1.11.3.min.js"></script>
</html>
updateBook.jsp
<%@ page contentType="text/html;charset=UTF-8" language="java" %>
<%@taglib prefix="c" uri="http://java.sun.com/jsp/jstl/core" %>
<%@taglib prefix="fmt" uri="http://java.sun.com/jsp/jstl/fmt" %>
<html>
<head>
<link rel="${pageContext.request.contextPath}/static/css/bootstrap.css">
<title>更新书籍</title>
</head>
<body>
<form action="${pageContext.request.contextPath}/book/updateBook" method="post">
编号:<input type="text" name="bookid" value="<%=request.getParameter("bookid")%>" readonly="readonly"/><br>
书名:<input type="text" name="name" /><br>
价格:<input type="text" name="price" /><br><br>
<input type="submit" value="提交" /><br>
</form>
</body>
<script src="${pageContext.request.contextPath}/static/js/bootstrap.js"></script>
<script src="${pageContext.request.contextPath}/static/js/jquery-1.11.3.min.js"></script>
</html>
5.queryInfoById.jsp
<%@ page contentType="text/html;charset=UTF-8" language="java" %>
<%@taglib prefix="c" uri="http://java.sun.com/jsp/jstl/core" %>
<%@taglib prefix="fmt" uri="http://java.sun.com/jsp/jstl/fmt" %>
<html>
<head>
<title>根据ID查找书籍</title>
<link rel="${pageContext.request.contextPath}/static/css/bootstrap.css">
</head>
<body>
<a href="bookInfo.jsp">返回主页面,请点击</a>
<table class="table">
<thead>
<tr>
<th scope="col">bookId</th>
<th scope="col">bookName</th>
<th scope="col">bookPrice</th>
<th scope="col">操作</th>
</tr>
</thead>
<tbody>
<c:forEach items="${booklist}" var="book">
<tr>
<th scope="row">${book.bookid}</th>
<td>${book.bookname}</td>
<td>${book.price}</td>
<td>
<a href="${pageContext.request.contextPath}/book/toupdateBook">修改</a>
<a href="${pageContext.request.contextPath}/book/delBook?bookid=${book.bookid}">删除</a>
</td>
</tr>
</c:forEach>
<nav aria-label="Page navigation example">
<ul class="pagination">
<li class="page-item">
<a class="page-link" href="${pageContext.request.contextPath}/book/queryById?pageNum=${pageInfo.prePage}" aria-label="Previous">
<span aria-hidden="true">«</span>
</a>
</li>
<c:forEach items="${pageInfo}" var="p" begin="1" end="${pageInfo.pages}" step="1">
<li class="page-item"><a class="page-link" href="${pageContext.request.contextPath}/book/queryById?pageNum=${p}">${p}</a></li>
</c:forEach>
<li class="page-item">
<a class="page-link" href="${pageContext.request.contextPath}/book/queryById?pageNum=${pageInfo.nextPage}" aria-label="Next">
<span aria-hidden="true">»</span>
</a>
</li>
</ul>
</nav>
</tbody>
</table>
</body>
<script src="${pageContext.request.contextPath}/static/js/bootstrap.js"></script>
<script src="${pageContext.request.contextPath}/static/js/jquery-1.11.3.min.js"></script>
</html>
queryInfoByName.jsp
<%@ page contentType="text/html;charset=UTF-8" language="java" %>
<%@taglib prefix="c" uri="http://java.sun.com/jsp/jstl/core" %>
<%@taglib prefix="fmt" uri="http://java.sun.com/jsp/jstl/fmt" %>
<html>
<head>
<title>根据ID查找书籍</title>
<link rel="${pageContext.request.contextPath}/static/css/bootstrap.css">
</head>
<body>
<a href="bookInfo.jsp">返回主页面,请点击</a>
<table class="table">
<thead>
<tr>
<th scope="col">bookId</th>
<th scope="col">bookName</th>
<th scope="col">bookPrice</th>
<th scope="col">操作</th>
</tr>
</thead>
<tbody>
<c:forEach items="${booklist}" var="book">
<tr>
<th scope="row">${book.bookid}</th>
<td>${book.bookname}</td>
<td>${book.price}</td>
<td>
<a href="${pageContext.request.contextPath}/book/toupdateBook">修改</a>
<a href="${pageContext.request.contextPath}/book/delBook?bookid=${book.bookid}">删除</a>
</td>
</tr>
</c:forEach>
<nav aria-label="Page navigation example">
<ul class="pagination">
<li class="page-item">
<a class="page-link" href="${pageContext.request.contextPath}/book/queryByNmme?pageNum=${pageInfo.prePage}" aria-label="Previous">
<span aria-hidden="true">«</span>
</a>
</li>
<c:forEach items="${pageInfo}" var="p" begin="1" end="${pageInfo.pages}" step="1">
<li class="page-item"><a class="page-link" href="${pageContext.request.contextPath}/book/queryByNmme?pageNum=${p}">${p}</a></li>
</c:forEach>
<li class="page-item">
<a class="page-link" href="${pageContext.request.contextPath}/book/queryByNmme?pageNum=${pageInfo.nextPage}" aria-label="Next">
<span aria-hidden="true">»</span>
</a>
</li>
</ul>
</nav>
</tbody>
</table>
</body>
<script src="${pageContext.request.contextPath}/static/js/bootstrap.js"></script>
<script src="${pageContext.request.contextPath}/static/js/jquery-1.11.3.min.js"></script>
</html>
祝福
都看到(滑到)这儿,不如给个大指拇的点赞吧!!!小M祝大家学习顺利,考试顺利,工作顺利,每天都能开心的敲代码,还有圣诞快乐!
转载:欢迎转载,但未经作者同意,必须保留此段声明;必须在文章中给出原文连接;否则必究法律责任(虽然但是应该不会有人会看上这篇小破文章)
有大佬瞧见或者发现不对的地方可以私信或者评论区。
勿杠,互联网打工人都不容易。