What’s React-Router
React Router 是一个基于 React 之上的强大路由库,它可以让你向应用中快速地添加视图和数据流,同时保持页面与 URL 间的同步。
前言
继续上一篇文章的延续<<React教程之create-react-app+webpack打包运行项目(react-webpack-starter)>>
https://blog.csdn.net/moshowgame/article/details/91984076
官方文档
https://react-guide.github.io/react-router-cn/docs/Introduction.html
开源项目
https://github.com/moshowgame/react-study
npm install
执行以下安装命令
npm install --save react-route react-router-dom
确认package.json里面有以下依赖,且大版本号基本一致(版本相差过大)
"dependencies": {
"react": "^16.8.6",
"react-dom": "^16.8.6",
"react-route": "^1.0.3",
"react-router-dom": "^5.0.1",
"react-scripts": "3.0.1"
},
目录结构
基本核心代码都放在src
下的components
目录,首页index.html无需改变,保证有个root
的div
即可,再index.js
中通过ReactDOM.render(<App />, document.getElementById('root'));
来进行渲染
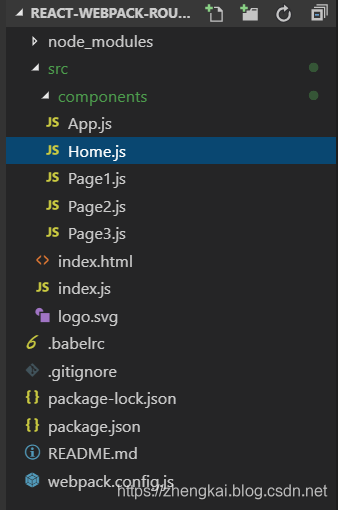
index.js
import React from 'react';
import ReactDOM from 'react-dom';
import App from './components/App';
ReactDOM.render(<App />, document.getElementById('root'));
App.js
这里引发了一个HashRouter
vs BrowserRouter
的问题,请见文分解
import React from 'react';
//HashRouter,支持#识别,http://localhost:8080/#/Page3/
import { HashRouter as Router,Route} from 'react-router-dom';
//单页BrowserRouter
//import { BrowserRouter as Router,Route} from 'react-router-dom';
import Home from './home';
import Page1 from './page1';
import Page2 from './page2';
import Page3 from './page3';
class App extends React.Component {
render(){
return(
<Router >
<div>
<Route exact path="/" component={Home} />
<Route path="/Page1/:name" component={Page1} />
<Route path="/Page2" component={Page2} />
<Route path="/Page3" component={Page3} />
</div>
</Router>
)
}
}
export default App;
Home.js
import React from 'react';
import { Link } from 'react-router-dom';
import { Router, Route, Switch } from "react-router";
class Home extends React.Component{
render(){
return(
<div>
<p>This is Home!</p>
<Link to="/Page1/Moshow" style={{color:'black'}}>
<div>点击跳转到Page1</div>
</Link>
<Link to="/Page2/" style={{color:'black'}}>
<div>点击跳转到Page2</div>
</Link>
<Link to="/Page3/" style={{color:'black'}}>
<div>点击跳转到Page3</div>
</Link>
</div>
);
}
}
export default Home;
Page1.js
import React from 'react';
import App from './App';
class Page1 extends React.Component {
render() {
let mystyle = {
width: '100%',
height: '80px',
backgroundColor: 'pink',
fontSize: '24px',
textAlign: 'center',//垂直居中
lineHeight: '80px'//水平居中
};
console.log(this, this.props);
return (
<div>
<h1 style={mystyle}>This is Page 111111! your staff-id :{this.props.match.params.name}</h1>
</div>
);
}
}
export default Page1;
Page2.js
import React from 'react';
class Page2 extends React.Component{
render(){
let mystyle={
width: '100%',
height:'80px',
backgroundColor:'greenyellow',
fontSize:'24px',
textAlign: 'center',//垂直居中
lineHeight: '80px'//水平居中
};
return(
<div>
<h1 style={mystyle}>This is Page 222222!</h1>
</div>
);
}
}
export default Page2;
Page3.js
import React from 'react';
const Page3 = ({match}) => {
let mystyle={
width: '100%',
height:'80px',
backgroundColor:'burlywood',
fontSize:'34px',
textAlign: 'center',//垂直居中
lineHeight: '80px'//水平居中
};
return(
<div>
<h1 style={mystyle}>hello {match.params.id} This is Page 333333!</h1>
</div>
);
}
export default Page3;
npm start
现在让我们开始运行项目把.输入
npm start
运行效果如下
http://localhost:8080/#
http://localhost:8080/#/Page1/:yourname
http://localhost:8080/#/Page2/
http://localhost:8080/#/Page3/
以下内容为补充部分 : BrowserRouter vs HashRouter
BrowserRouter
It uses history API, i.e. it’s unavailable for legacy browsers (IE 9 and lower and contemporaries). Client-side React application is able to maintain clean routes like example.com/react/route but needs to be backed by web server. Usually this means that web server should be configured for single-page application, i.e. same index.html is served for /react/route path or any other route on server side. On client side, window.location.pathname is parsed by React router. React router renders a component that it was configured to render for /react/route.
它使用浏览历史history API
,所以他不适用于传统浏览器(IE 9及更低版本)。 客户端的React应用程序应该能够维护像example.com/react/route这样的干净路由,但需要由Web服务器支持。 通常这意味着Web服务器应该配置为单页面应用程序
,即为/ react / route路径或服务器端的任何其他路由提供相同的index.html。 在客户端,window.location.pathname
由React路由器解析。 React路由器呈现一个组件,它被配置为为/ react / route呈现。
HashRouter
It uses URL hash, it puts no limitations on supported browsers or web server. Server-side routing is independent from client-side routing.
Backward-compatible single-page application can use it as example.com/#/react/route. The setup cannot be backed up by server-side rendering because it’s / path that is served on server side, #/react/route URL hash cannot be read from server side. On client side, window.location.hash is parsed by React router. React router renders a component that it was configured to render for /react/route, similarly to BrowserRouter.
Most importantly, HashRouter use cases aren’t limited to SPA. A website may have legacy or search engine-friendly server-side routing, while React application may be a widget that maintains its state in URL like example.com/server/side/route#/react/route. Some page that contains React application is served on server side for /server/side/route, then on client side React router renders a component that it was configured to render for /react/route, similarly to previous scenario.
它使用URL Hash
哈希,它对受支持的浏览器或Web服务器没有限制。服务器端路由独立于客户端路由。
向后兼容的单页面应用程序可以将其用作example.com/#/react/route。服务器端渲染无法备份设置,因为它是服务器端提供的/path,#/react /route URL hash无法从服务器端读取。在客户端,window.location.hash
由React路由器解析。 React路由器呈现一个组件,它被配置为为/ react / route呈现,类似于BrowserRouter。
最重要的是,HashRouter用例不仅限于SPA。一个网站可能具有遗留或搜索引擎友好的服务器端路由,而React应用程序可能是一个小部件,它在URL中保持其状态,如example.com/server/side/route#/react/route。一些包含React应用程序的页面在服务器端为/ server / side / route提供,然后在客户端React路由器呈现一个组件,它被配置为为/ react / route呈现,与之前的方案类似。(简单的讲,就是可以使用http://localhost:8080/#/Page1/Moshow
这种方式来访问URL,无刷新页面,切换视图,用hash 实现路由切换,本身附带history记录,简单舒服。)