目录
题目:
1.
首次打开页面,展示所有汽车信息列表,如图 1 所示。
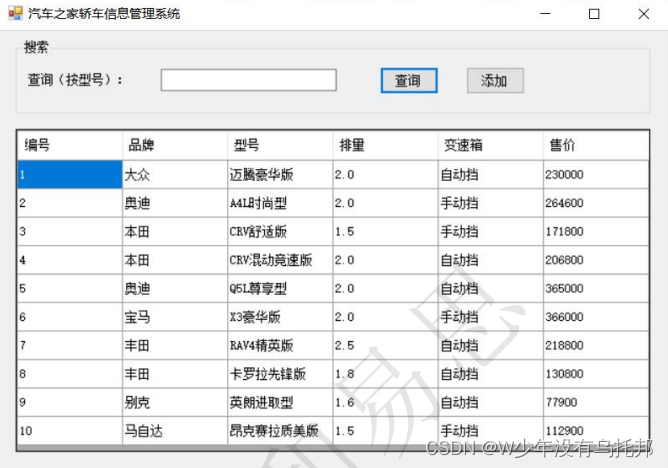
图 1 页面初始化效果
2. 系统能根据汽车型号进行检索,点击“搜索”,在列表中展示符合所选状态的数据,如图 2 所示。
图 2 按条件查询轿车信息
3. 鼠标右键在列表页单击某一行,弹出删除菜单,如图 3
图3 删除菜单
单击删除后,弹出确定对话框,如图 4,用户单击“是”则删除当前选中行,并刷新列表,
单击否则关闭提示框,不执行删除。
图4 删除确定对话框
4. 点击“添加”按钮,打开添加汽车信息窗体,如图5所示
5. 在添加汽车信息窗体中输入相关的信息, 点击“确定”按钮, 将数据添加至数据库表中。点
击取消,关闭添加汽车信息窗口。添加完成后,在列表页面点击“查询”按钮, 可以看见新添加
的汽车信息。
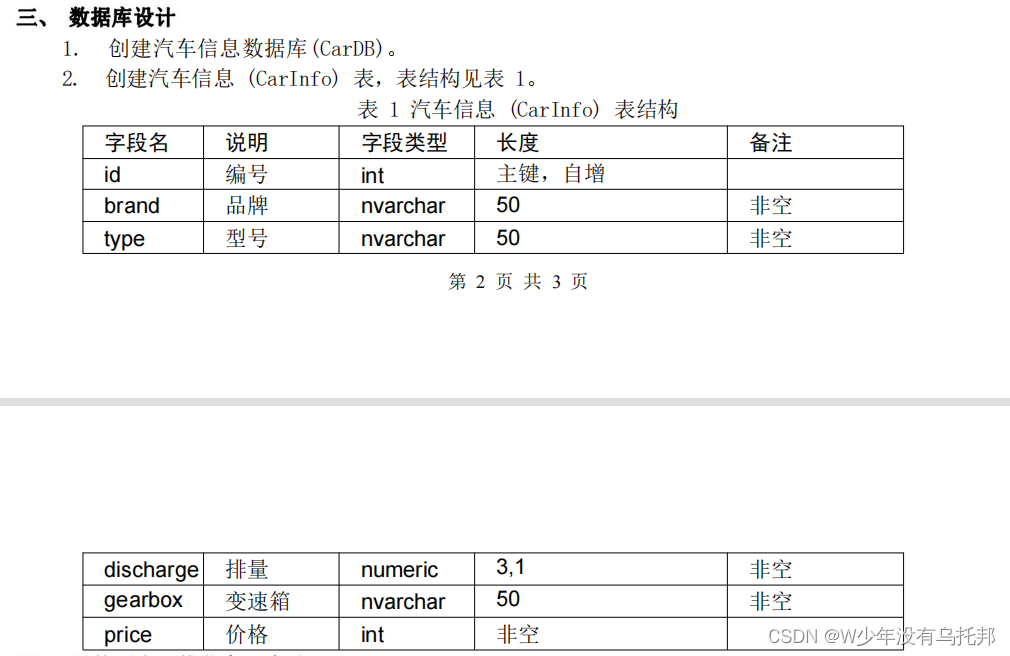
效果图:
做法:
首先设置datagridview的这三个属性
1 AutoSizeColumnsMode = Fill 设置每列宽度平均分布
2 RowHeaderVisible = false 取消最左侧空⽩列
3 SelectionMode = FullRowSelect 设置单元格选中模式为整⾏选中
代码部分:
DBHelper类
using System;
using System.Collections.Generic;
using System.Data.SqlClient;
using System.Data;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
using System.Windows.Forms;
namespace car
{
internal class DBHelper
{
public static string ConnString = "server=.;database=CarDB;uid=sa;pwd=123456";
public static SqlConnection Conn = null;
//初始化数据库连接
public static void InitConnection()
{
if (Conn == null)
{
Conn = new SqlConnection(ConnString);
}
if (Conn.State == ConnectionState.Closed)
{
Conn.Open();
}
if (Conn.State == ConnectionState.Broken)
{
Conn.Close();
Conn.Open();
}
}
//查询,返回值为DataSet
public static DataSet ds(string sql)
{
InitConnection();
DataSet t=new DataSet();
SqlDataAdapter d=new SqlDataAdapter(sql,Conn);
d.Fill(t);
Conn.Close();
return t;
}
//增删改操作
public static bool ExecuteNonQuery(string sqlStr)
{
int result = 0;
try
{
InitConnection();
SqlCommand cmd = new SqlCommand(sqlStr, Conn);
result = cmd.ExecuteNonQuery();
}
catch (Exception e)
{
MessageBox.Show("重新输入" + e.Message);
}
finally
{
Conn.Close();
}
return result > 0;
}
}
}
From1主窗体代码
//using car.登录;
using car;
using System;
using System.Collections.Generic;
using System.ComponentModel;
using System.Data;
using System.Data.SqlClient;
using System.Drawing;
using System.Linq;
using System.Security.Cryptography;
using System.Text;
using System.Threading.Tasks;
using System.Windows.Forms;
namespace car
{
public partial class Form1 : Form
{
public Form1()
{
InitializeComponent();
}
//该方法专门用来更新datagirdview表的展示数据库表中所有数据,一共引用了两次
private void zhanshiall()
{
string sql = "select * from CarInfo";
DataSet ds = DBHelper.ds(sql);
DataTable dsC = ds.Tables[0];
this.dataGridView1.DataSource = dsC;
}
private void Form1_Load(object sender, EventArgs e)
{
this.dataGridView1.AutoGenerateColumns = false;//取消自动生成列
zhanshiall();
}
private void button1_Click(object sender, EventArgs e)
{
//模糊查询姓名sql语句,如果为空则展示数据库表的所有值
string s = string.Format("select * from CarInfo where type like '%{0}%'", textBox1.Text);
DataSet ds = DBHelper.ds(s);
DataTable dsC = ds.Tables[0];
this.dataGridView1.DataSource = dsC;
}
//点击生成新窗体
private void button2_Click(object sender, EventArgs e)
{
ti t =new ti();
t.ShowDialog();
//如果在新窗体中添加成功则会展示数据库表中所有的数据
if (t.DialogResult==DialogResult.OK)
{
string s = "select * from CarInfo";
dataGridView1.DataSource = DBHelper.ds(s).Tables[0] ;
}
}
private void 删除ToolStripMenuItem_Click(object sender, EventArgs e)
{
//获取选定行的编号的列
string shan = dataGridView1.CurrentRow.Cells[0].Value.ToString();
//执行删除操作
string s = string.Format("delete CarInfo where id='{0}'",shan);
if (DBHelper.ExecuteNonQuery(s))
{ //删除后直接更新datagirdview
zhanshiall();
}
else
{
MessageBox.Show("删除失败");
}
}
}
}
添加代码:
using System;
using System.Collections.Generic;
using System.ComponentModel;
using System.Data;
using System.Drawing;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
using System.Windows.Forms;
namespace car
{
public partial class ti : Form
{
public ti()
{
InitializeComponent();
}
private void button1_Click(object sender, EventArgs e)
{
//直接调用DBHelper的增删减方法进行添加就好
string q = string.Format("insert CarInfo(brand,type,discharge,gearbox,price) values ('{0}','{1}','{2}','{3}','{4}') ",textBox1.Text, textBox2.Text, textBox3.Text, textBox4.Text, textBox5.Text);
if (DBHelper.ExecuteNonQuery(q))
{
MessageBox.Show("添加成功");
this.DialogResult=DialogResult.OK;
}
else
{
MessageBox.Show("添加失败");
}
}
private void button2_Click(object sender, EventArgs e)
{
this.Close();
}
private void ti_Load(object sender, EventArgs e)
{
}
}
}