某网站提供了Javadoc中文版,Google译文和百度译文。阅读ClassLoader类的文件时候发现两个文档说法不一。所以干脆自己啃一啃英文。
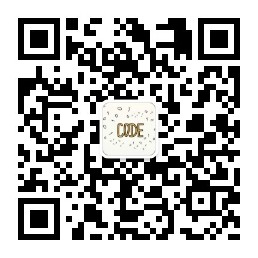
类加载器是一个对象,负责加载class文件。类加载器是抽象类。给定一个二进制文件名,类加载器应该尝试去定位或产生类的数据结构。然后把二进制文件名作为class文件名,去文件系统中找对应的“class 文件”是常用的做法。
每一个Class类对象都包含一个定义该Class类对象的ClassLoader的引用。(Class类对象可以获取加载它的加载器)
数组类的对象不是通过类加载器创建,它是根据请求在Java运行时自动创建的。数组类的类加载器,可以通过 Class.getClassLoader()获取,数组类加载器和数组元素的 类加载器是一样的。如果数组元素类型是原生类型,数组类没有加载器。(素组类加载器是和元素类型加载器是一样的)
为了扩展Java虚拟机动态加载类,应用程序需继承ClassLoader类。(自定义类加载器,需要实现CLassLoader类)
ClassLoader类使用委派模型来搜索类和资源。每一个ClassLoader的实例有一个相关联的父类加载器。当请求一个类或资源的时候,类加载器实例会委托它的父类加载器尝试加载。虚拟机内置的类加载器,被称为启动类加载器,它没有父类,但是可以作为类加载器实例的父类。
支持并发加载类的类加载器称为并行加载类加载器,需要通过调用ClassLoader.registerAsParallelCapable方法在类初始化时注册自己。请注意,ClassLoader类默认注册为并行。 但是,如果它们具有并行能力,它的子类仍然需要注册自己。
在委托模型不是严格分层的环境中,类加载器需要具有并行能力,否则类加载会导致死锁,因为加载器锁在类加载过程的持续时间内保持(请参阅loadClass方法)。
通常,Java虚拟机以与平台相关的方式从本地文件系统加载类。 例如,在UNIX系统上,虚拟机从CLASSPATH环境变量定义的目录中加载类。
但是,某些类可能不是源自文件; 它们可能来自其他来源,例如网络,或者它们可以由应用程序构建。 方法defineClass将字节数组转换为类Class的实例。 可以使用Class.newInstance创建此新定义的类的实例。
由类加载器创建的对象的方法和构造函数可以引用其他类。 要确定所引用的类,Java虚拟机将调用最初创建该类的类加载器的loadClass方法。
例子:
/**
* 自定义类加载器
*/
public class myClassLoader extends ClassLoader{
public String classLoaderName;
public final String fileExtentionName = ".class";
/**
* 调用父类的构造方法,返回父类加载器。默认是系统加载器。
*
*/
public myClassLoader(String classLoaderName){
super();
this.classLoaderName = classLoaderName;
}
/**
* 指定父类的加载器。
*/
public myClassLoader(ClassLoader parent,String classLoaderName){
super(parent);
this.classLoaderName = classLoaderName;
}
/**
* 返回Class对象
*/
@Override
protected Class<?> findClass(String name) throws ClassNotFoundException {
byte[] data = this.loadClassDate(name);
this.defineClass(name,data,0,data.length);
return super.findClass(name);
}
/**
* 通过传入类的目录。获取数据。
*/
public byte[] loadClassDate(String className){
InputStream input = null;
byte[] data = null;
ByteArrayOutputStream byteOutPut = null;
try{
this.classLoaderName = this.classLoaderName.replace(".","/");
input = new FileInputStream(new File(className+this.fileExtentionName));
byteOutPut = new ByteArrayOutputStream();
int ch = 0;
while (-1 !=(ch = input.read())){
byteOutPut.write(ch);
}
data = byteOutPut.toByteArray();
}catch (Exception e){
}finally {
try {
input.close();
byteOutPut.close();
} catch (IOException e) {
e.printStackTrace();
}
}
return data;
}
/**
* 虽然没有显示的调用上面的方法。
*/
public static void test(ClassLoader classLoader) throws Exception {
/**
* 这里并没有直接去调用上面的方法。而是通过回调上面的方法。当调用loadClass的时候。
*/
Class<?> aClass = classLoader.loadClass("com.example.demo.constPro.myTest001");
/**
* 创建实例
*/
Object obj = aClass.newInstance();
System.out.println(obj);
}
/**
* 创建实例
*/
public static void main(String[] args) throws Exception {
/**
* 类加载器添加一个名字。
*/
myClassLoader myLoad = new myClassLoader("myLoader0000000999");
test(myLoad);
}
}
原文:
public abstract class ClassLoader
extends Object
A class loader is an object that is responsible for loading classes. The class ClassLoader is an abstract class. Given the binary name of a class, a class loader should attempt to locate or generate data that constitutes a definition for the class. A typical strategy is to transform the name into a file name and then read a "class file" of that name from a file system.
Every Class object contains a reference to the ClassLoader that defined it.
Class objects for array classes are not created by class loaders, but are created automatically as required by the Java runtime. The class loader for an array class, as returned by Class.getClassLoader() is the same as the class loader for its element type; if the element type is a primitive type, then the array class has no class loader.
Applications implement subclasses of ClassLoader in order to extend the manner in which the Java virtual machine dynamically loads classes.
Class loaders may typically be used by security managers to indicate security domains.
The ClassLoader class uses a delegation model to search for classes and resources. Each instance of ClassLoader has an associated parent class loader. When requested to find a class or resource, a ClassLoader instance will delegate the search for the class or resource to its parent class loader before attempting to find the class or resource itself. The virtual machine's built-in class loader, called the "bootstrap class loader", does not itself have a parent but may serve as the parent of a ClassLoader instance.
Class loaders that support concurrent loading of classes are known as parallel capable class loaders and are required to register themselves at their class initialization time by invoking the ClassLoader.registerAsParallelCapable method. Note that the ClassLoader class is registered as parallel capable by default. However, its subclasses still need to register themselves if they are parallel capable.
In environments in which the delegation model is not strictly hierarchical, class loaders need to be parallel capable, otherwise class loading can lead to deadlocks because the loader lock is held for the duration of the class loading process (see loadClass methods).
Normally, the Java virtual machine loads classes from the local file system in a platform-dependent manner. For example, on UNIX systems, the virtual machine loads classes from the directory defined by the CLASSPATH environment variable.
However, some classes may not originate from a file; they may originate from other sources, such as the network, or they could be constructed by an application. The method defineClass converts an array of bytes into an instance of class Class. Instances of this newly defined class can be created using Class.newInstance.
The methods and constructors of objects created by a class loader may reference other classes. To determine the class(es) referred to, the Java virtual machine invokes the loadClass method of the class loader that originally created the class.
For example, an application could create a network class loader to download class files from a server. Sample code might look like:
ClassLoader loader = new NetworkClassLoader(host, port);
Object main = loader.loadClass("Main", true).newInstance();
. . .
The network class loader subclass must define the methods findClass and loadClassData to load a class from the network. Once it has downloaded the bytes that make up the class, it should use the method defineClass to create a class instance. A sample implementation is:
class NetworkClassLoader extends ClassLoader {
String host;
int port;
public Class findClass(String name) {
byte[] b = loadClassData(name);
return defineClass(name, b, 0, b.length);
}
private byte[] loadClassData(String name) {
// load the class data from the connection
. . .
}
}
Any class name provided as a String parameter to methods in ClassLoader must be a binary name as defined by The Java™ Language Specification.
Examples of valid class names include:
"java.lang.String"
"javax.swing.JSpinner$DefaultEditor"
"java.security.KeyStore$Builder$FileBuilder$1"
"java.net.URLClassLoader$3$1"