导入jwt依赖
<dependency>
<groupId>io.jsonwebtoken</groupId>
<artifactId>jjwt</artifactId>
<version>0.9.1</version>
</dependency>
token实体类
@Data
public class TokenDto implements Serializable {
private String token;
private String expire;
}
封装jwt生成token工具类
public class JwtUtils {
private static final long EXPIRE = 1000 * 60 * 60 * 24 * 7;
private static final String SECRET = "PVjALNPPuVzHQaPrGQJJ94r3u0ZoL2hcnEUPAcOAwkM=";
private static final String SUBJECT = "enterprise";
public static final String CUSTOM_PAYLOAD_PHONE_KEY = "phone";
public static final String CUSTOM_PAYLOAD_TYPE_KEY = "type";
public static final String CUSTOM_PAYLOAD_TENANTID_KEY = "tenantId";
public static final String TYPE_APPLETS = "applets";
public static final String TYPE_WEB = "web";
public static TokenDto getToken(String type, String tenantId, String phone){
SignatureAlgorithm signatureAlgorithm = SignatureAlgorithm.HS256;
TokenDto dto = new TokenDto();
Date exp = new Date(System.currentTimeMillis()+EXPIRE);
String token = Jwts.builder()
.setSubject(SUBJECT)
.claim(CUSTOM_PAYLOAD_TYPE_KEY,type)
.claim(CUSTOM_PAYLOAD_TENANTID_KEY,tenantId)
.claim(CUSTOM_PAYLOAD_PHONE_KEY,phone)
.setIssuedAt(new Date())
.setExpiration(exp)
.signWith(signatureAlgorithm,getSecretKey())
.compact();
dto.setToken(token);
dto.setExpire(TimeUtil.dateToStamp2(exp));
return dto;
}
public static Claims checkJwt(String token){
SecretKey key = getSecretKey();
Claims claims;
try {
claims = Jwts.parser()
.setSigningKey(key)
.parseClaimsJws(token).getBody();
} catch (Exception e) {
claims = null;
}
return claims;
}
private static SecretKey getSecretKey() {
byte[] encodeKey = Base64.decodeBase64(SECRET);
SecretKey key = new SecretKeySpec(encodeKey, 0, encodeKey.length, "AES");
return key;
}
登录过滤器实现Filter接口
@AllArgsConstructor
@WebFilter(urlPatterns = "/app/api/v1/pri/*",filterName = "loginFilter")
public class LoginFilter implements Filter {
private final RedisUtil redisUtil ;
@Override
public void init(FilterConfig filterConfig) throws ServletException {
System.out.println("login filter init...");
}
@Override
public void doFilter(ServletRequest servletRequest, ServletResponse servletResponse, FilterChain filterChain) throws IOException, ServletException {
System.out.println("login filter doFilter...");
HttpServletRequest req = (HttpServletRequest) servletRequest;
HttpServletResponse resp = (HttpServletResponse) servletResponse;
String token = req.getHeader(AppConstant.APP_TOKEN_HEADER);
if(StringUtils.isNotEmpty(token)){
Claims claims = JwtUtils.checkJwt(token);
if(null != claims){
String phone = (String)claims.get(JwtUtils.CUSTOM_PAYLOAD_PHONE_KEY);
if(StringUtils.isNotEmpty(phone)){
String redisToken = redisUtil.get(AppConstant.APP_TOKEN_REDIS_PREFIX + phone);
if(StringUtils.isNotEmpty(redisToken) && StringUtils.equals(token,redisToken)){
redisUtil.setEx(AppConstant.APP_TOKEN_REDIS_PREFIX+phone,token,AppConstant.APP_TOKEN_EXPIRE);
filterChain.doFilter(servletRequest,servletResponse);
}else {
renderJson(resp,R.fail(401,"请求未授权"));
}
}else {
renderJson(resp,R.fail(401,"请求未授权"));
}
}else {
renderJson(resp,R.fail(401,"请求未授权"));
}
}else {
renderJson(resp,R.fail(401,"请求未授权"));
}
}
@Override
public void destroy() {
System.out.println("login filter destroy...");
}
private void renderJson(HttpServletResponse response, R<Object> json){
response.setCharacterEncoding("UTF-8");
response.setContentType("application/json");
try (PrintWriter writer = response.getWriter()){
writer.print(JSON.toJSONString(json));
} catch (IOException e) {
e.printStackTrace();
}
}
}
启动类上要加@ServletComponentScan注解
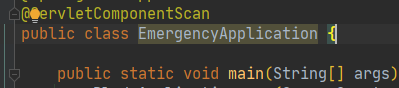
登录接口示列
@PostMapping("/login")
@ApiOperationSupport(order = 2)
@ApiOperation(value = "物业APP登录", notes = "手机号和密码")
@ApiImplicitParams({
@ApiImplicitParam(name = "data", value = "手机号和密码加密后的字符串", paramType = "query", dataType = "string", required = true),
@ApiImplicitParam(name = "tenantId", value = "租户ID", paramType = "header", dataType = "string", required = true)
})
public String loginApp(HttpServletRequest request,String data) {
PropertyAppUser appUser = AesPkcs5Util.pareData(data, new PropertyAppUser(),apiCryptoProperties.getAesKey());
String tenantId = getTenantId(request);
if (null == appUser || StringUtils.isEmpty(tenantId) || StringUtils.isEmpty(appUser.getAccount()) || StringUtils.isEmpty(appUser.getPassword())) {
return AesPkcs5Util.encryptResponseData(R.fail("参数错误!"),apiCryptoProperties.getAesKey());
}
PropertyAppUser user = propertyAppUserService.getByPhone(appUser);
if (null == user) {
return AesPkcs5Util.encryptResponseData(R.fail("用户名或密码错误!"),apiCryptoProperties.getAesKey());
}
String lowerPwd = DigestUtil.encrypt(appUser.getPassword());
if (StringUtils.equals(user.getPassword(), lowerPwd)) {
String oldToken = redisUtil.get(AppConstant.APP_TOKEN_REDIS_PREFIX + appUser.getAccount());
if (StringUtils.isNotEmpty(oldToken)) {
redisUtil.del(AppConstant.APP_TOKEN_REDIS_PREFIX + appUser.getAccount());
}
TokenDto dto = JwtUtils.getToken(JwtUtils.TYPE_APP, tenantId, appUser.getAccount());
redisUtil.setEx(AppConstant.APP_TOKEN_REDIS_PREFIX + appUser.getAccount(), dto.getToken(), AppConstant.APP_TOKEN_EXPIRE);
Map<String,Object> map = propertyAppUserService.loginAppUser(user);
map.put("token",dto.getToken());
map.put("expire",dto.getExpire());
return AesPkcs5Util.encryptResponseData(R.data(map,"登录成功!"),apiCryptoProperties.getAesKey());
} else {
return AesPkcs5Util.encryptResponseData(R.fail("用户名或密码错误!"),apiCryptoProperties.getAesKey());
}
}