预览
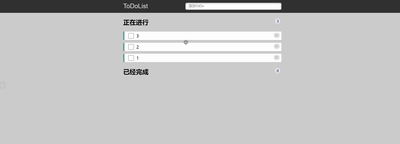
代码
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8" />
<meta http-equiv="X-UA-Compatible" content="IE=edge" />
<meta name="viewport" content="width=device-width, initial-scale=1.0" />
<title>Document</title>
<style>
body {
margin: 0;
padding: 0;
font-size: 16px;
background: #cdcdcd;
}
header {
height: 50px;
background: #333;
background: rgba(47, 47, 47, 0.98);
}
section {
margin: 0 auto;
}
label {
float: left;
width: 100px;
line-height: 50px;
color: #ddd;
font-size: 24px;
cursor: pointer;
font-family: "Helvetica Neue", Helvetica, Arial, sans-serif;
}
header input {
float: right;
width: 60%;
height: 24px;
margin-top: 12px;
text-indent: 10px;
border-radius: 5px;
box-shadow: 0 1px 0 rgba(255, 255, 255, 0.24),
0 1px 6px rgba(0, 0, 0, 0.45) inset;
border: none;
}
input:focus {
outline-width: 0;
}
h2 {
position: relative;
}
span {
position: absolute;
top: 2px;
right: 5px;
display: inline-block;
padding: 0 5px;
height: 20px;
border-radius: 20px;
background: #e6e6fa;
line-height: 22px;
text-align: center;
color: #666;
font-size: 14px;
}
ol,
ul {
padding: 0;
list-style: none;
}
li input {
position: absolute;
top: 2px;
left: 10px;
width: 22px;
height: 22px;
cursor: pointer;
}
p {
margin: 0;
}
li p input {
top: 3px;
left: 40px;
width: 70%;
height: 20px;
line-height: 14px;
text-indent: 5px;
font-size: 14px;
}
li {
height: 32px;
line-height: 32px;
background: #fff;
position: relative;
margin-bottom: 10px;
padding: 0 45px;
border-radius: 3px;
border-left: 5px solid #629a9c;
box-shadow: 0 1px 2px rgba(0, 0, 0, 0.07);
}
ol li {
cursor: move;
}
ul li {
border-left: 5px solid #999;
opacity: 0.5;
}
li a {
position: absolute;
top: 2px;
right: 5px;
display: inline-block;
width: 14px;
height: 12px;
border-radius: 14px;
border: 6px double #fff;
background: #ccc;
line-height: 14px;
text-align: center;
color: #fff;
font-weight: bold;
font-size: 14px;
cursor: pointer;
}
@media screen and (max-device-width: 620px) {
section {
width: 96%;
padding: 0 2%;
}
}
@media screen and (min-width: 620px) {
section {
width: 600px;
padding: 0 10px;
}
}
</style>
</head>
<body>
<header>
<section>
<form action="" id="form" onclick="">
<label for="title">ToDoList</label>
<input
type="text"
id="title"
name="title"
placeholder="添加ToDo"
required="required"
autocomplete="off"
/>
</form>
</section>
</header>
<section>
<h2>正在进行 <span id="todocount"></span></h2>
<ol id="todolist" class="demo-box"></ol>
<h2>已经完成 <span id="donecount"></span></h2>
<ul id="donelist"></ul>
</section>
<script>
loadData();
let input = document.querySelector("input");
let ollist = document.querySelector("#todolist");
let ullist = document.querySelector("#donelist");
// 按回车键将输入框中的内容添加到<正在进行>中
input.onkeydown = function (e) {
if (e.keyCode === 13) {
//如果输入框内容为空就直接返回
if (input.value == "") {
return;
} else {
let todolist = {
title: input.value,
done: false,
};
let arr = getData();
arr.push(todolist);
saveData(arr);
}
}
};
//获取数据并储存
function getData() {
let data = window.localStorage.getItem("todo");
if (data) {
return JSON.parse(data);
} else {
return [];
}
}
//添加数据到列表
function loadData() {
let data = getData();
let ollist = document.querySelector("#todolist");
let ullist = document.querySelector("#donelist");
let todoCount = document.querySelector("#todocount");
let doneCount = document.querySelector("#donecount");
let todocount = 0;
let donecount = 0;
//删除子节点的方法
let childs = ollist.childNodes;
for (let i = childs.length - 1; i >= 0; i--) {
ollist.removeChild(childs[i]);
}
let ulchilds = ullist.childNodes;
for (let i = ulchilds.length - 1; i >= 0; i--) {
ullist.removeChild(ulchilds[i]);
}
data.forEach((item, index) => {
let li = document.createElement("li"); //生成li
li.innerHTML =
"<input type='checkbox' class='change'/><p id='p" +
index +
"'>" +
item.title +
"</p><a href='javascript:;' id=" +
index +
">-</a>";
if (item.done) {
//已完成
ullist.insertBefore(li, ullist.children[0]);
li.children[0].checked = "checked";
donecount++;
} else {
//正在进行中
ollist.insertBefore(li, ollist.children[0]);
todocount++;
}
todoCount.innerText = todocount;
doneCount.innerText = donecount;
});
}
//储存数据
function saveData(arr) {
return window.localStorage.setItem("todo", JSON.stringify(arr));
}
//删除数据
function removeData() {
ollist.addEventListener(
"click",
function (e) {
if (e.target.nodeName === "A") {
//找到对应的元素节点属性
let index = e.target.getAttribute("id");
console.log(index);
let data = getData();
data.splice(index, 1);
saveData(data);
loadData();
}
},
false
);
}
removeData();
//编辑数据
function editData() {
ollist.addEventListener("click", function (e) {
if (e.target.nodeName === "P") {
let id = e.target.getAttribute("id");
let p = document.getElementById(id);
console.log(p);
//替换p中的innerHTML
let title = p.innerHTML;
p.innerHTML =
"<input type='text' value='" + title + "' id=input" + id + "'/>";
//失去焦点的时候
let input = document.getElementById("input" + id);
input.select();
input.addEventListener(
"blur",
function () {
if (input.value != "") {
p.innerHTML = input.value; //把input的value值赋值给p.innerHTML储存新的p.innerHTML
let data = getData();
let index = id.substring(1); //获取当前修改的索引值
data[index].title = p.innerHTML;
saveData(data); //储存新的p.innerHTML
loadData();
} else {
return;
}
},
false
);
}
});
}
editData();
//切换数据
function changeData() {
ollist.addEventListener("click", function (e) {
if (e.target.className === "change") {
console.log(e.target);
if (e.target.nextElementSibling.nextElementSibling) {
console.log(e.target.nextElementSibling);
let id =
e.target.nextElementSibling.nextElementSibling.getAttribute(
"id"
);
let data = getData();
data[id].done = true;
saveData(data); //储存新的p.innerHTML
loadData();
}
}
});
ullist.addEventListener("click", function (e) {
if (e.target.className === "change") {
let id =
e.target.nextElementSibling.nextElementSibling.getAttribute("id");
console.log(id);
let data = getData();
data[id].done = false;
saveData(data); //储存新的p.innerHTML
loadData();
}
});
}
changeData();
</script>
</body>
</html>