BM42 用两个栈实现队列
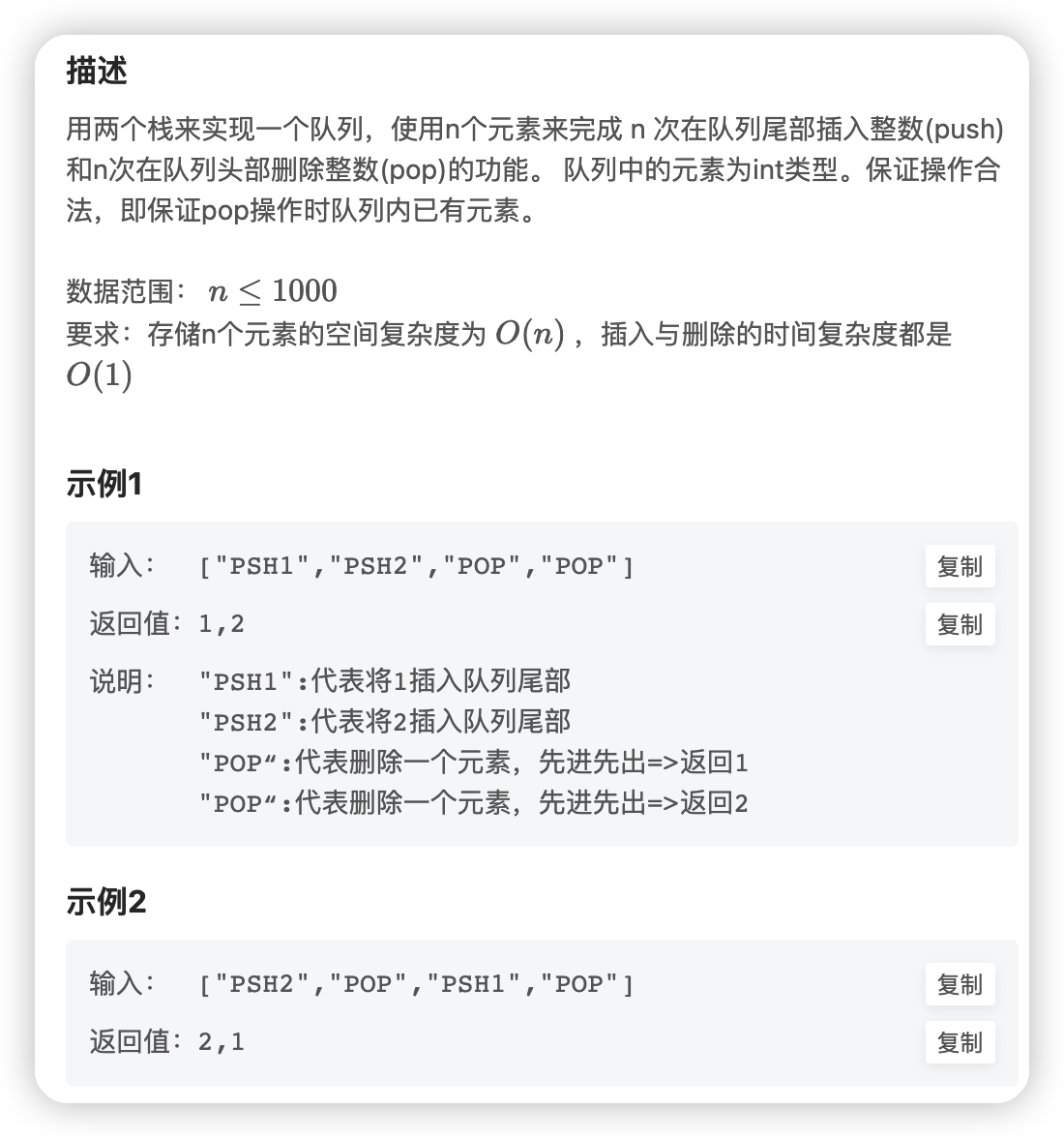
let stack1=[],stack2=[];
function push(node)
{
stack1.push(node);
}
function pop()
{
if(stack2.length==0){
while(stack1.length!==0){
stack2.push(stack1.pop());
}
}
return stack2.pop()
}
module.exports = {
push : push,
pop : pop
};
BM43 包含min函数的栈
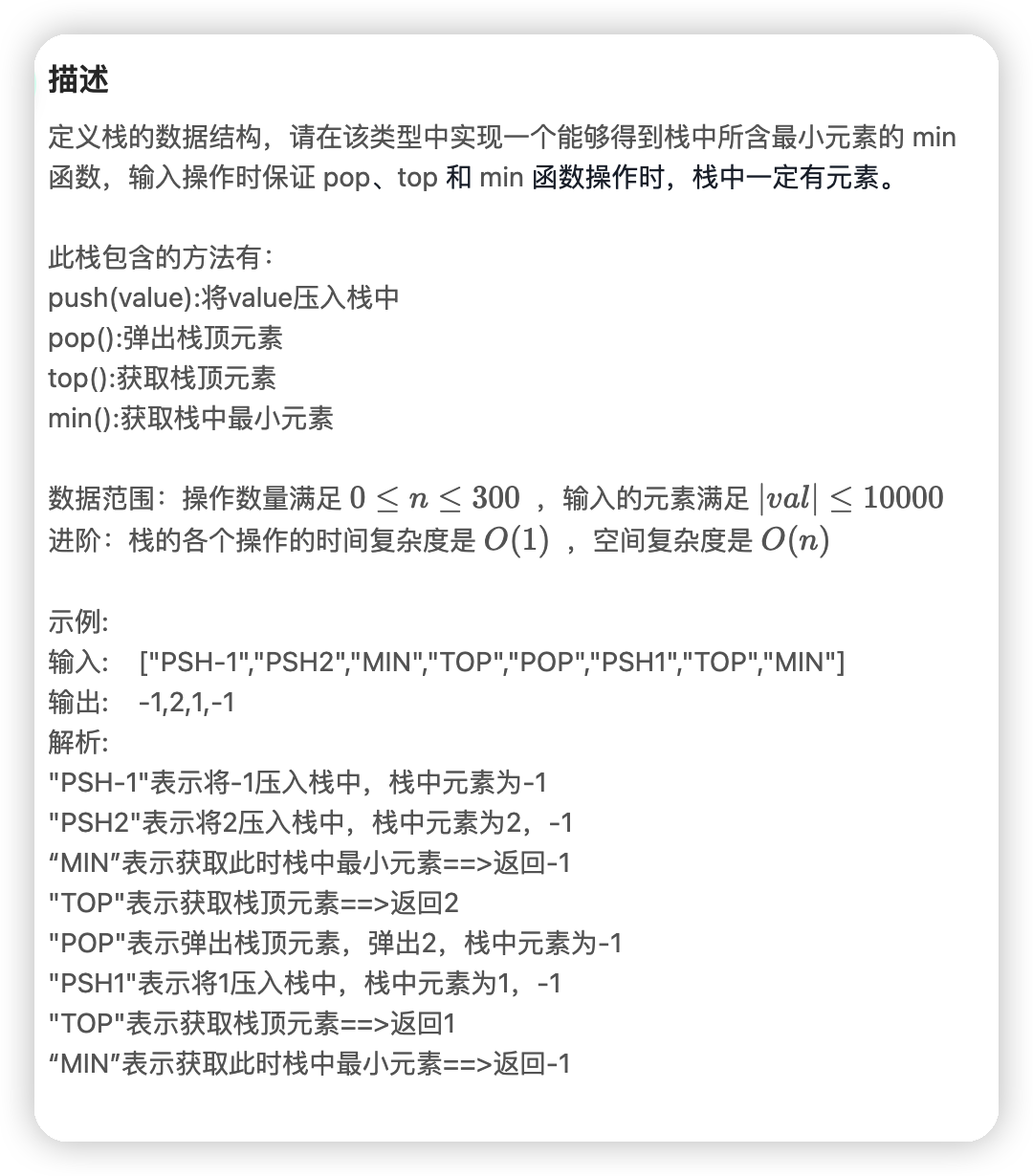
BM43 包含min函数的栈
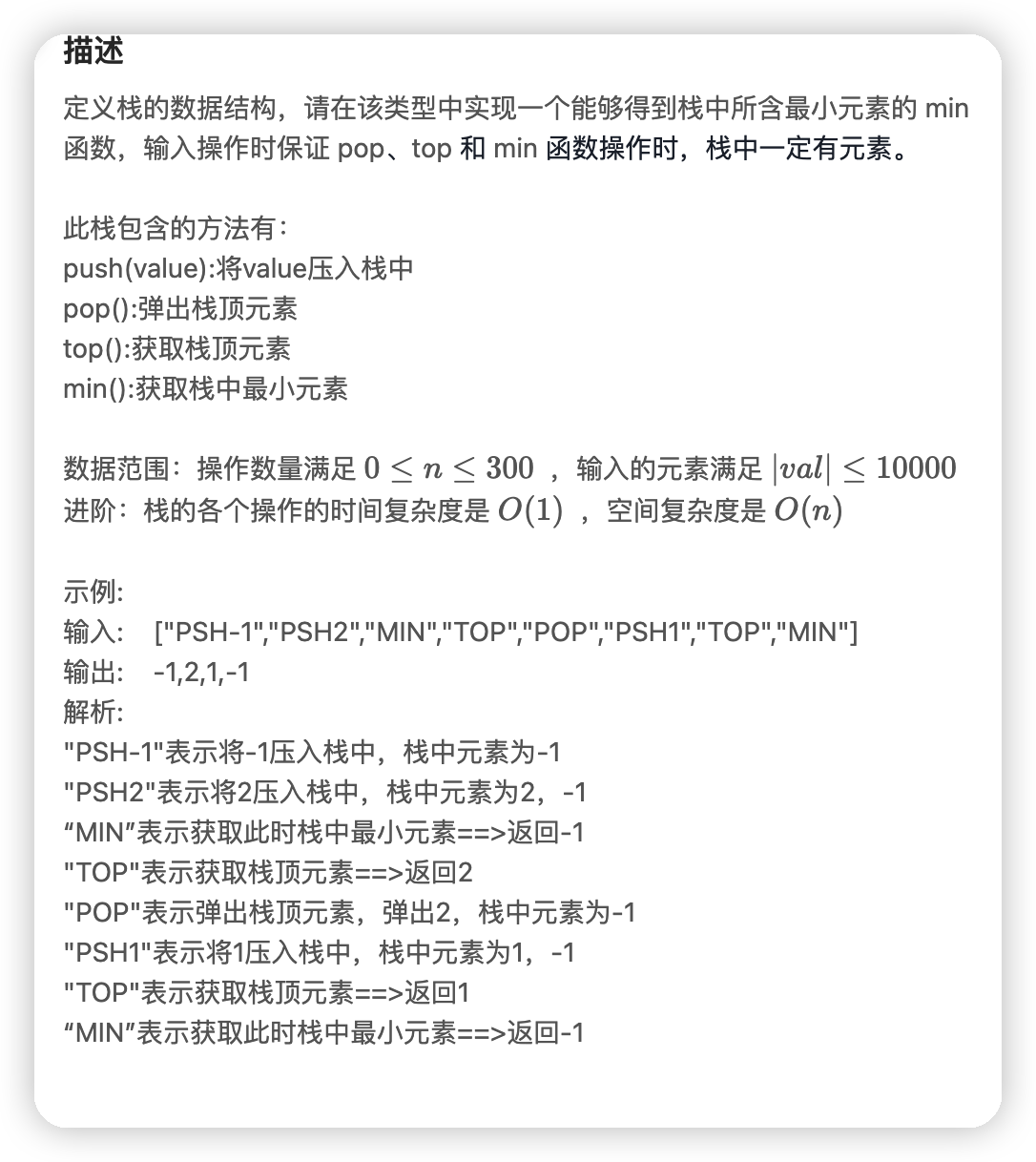
let stack1=[];
function push(node)
{
stack1.push(node);
}
function pop()
{
stack1.pop();
}
function top()
{
return stack1[stack1.length-1];
}
function min()
{
let min=stack1[0];
for(let i=1;i<stack1.length;i++){
min=min>stack1[i]?stack1[i]:min
}
return min
}
module.exports = {
push : push,
pop : pop,
top : top,
min : min
};
BM44 有效括号序列
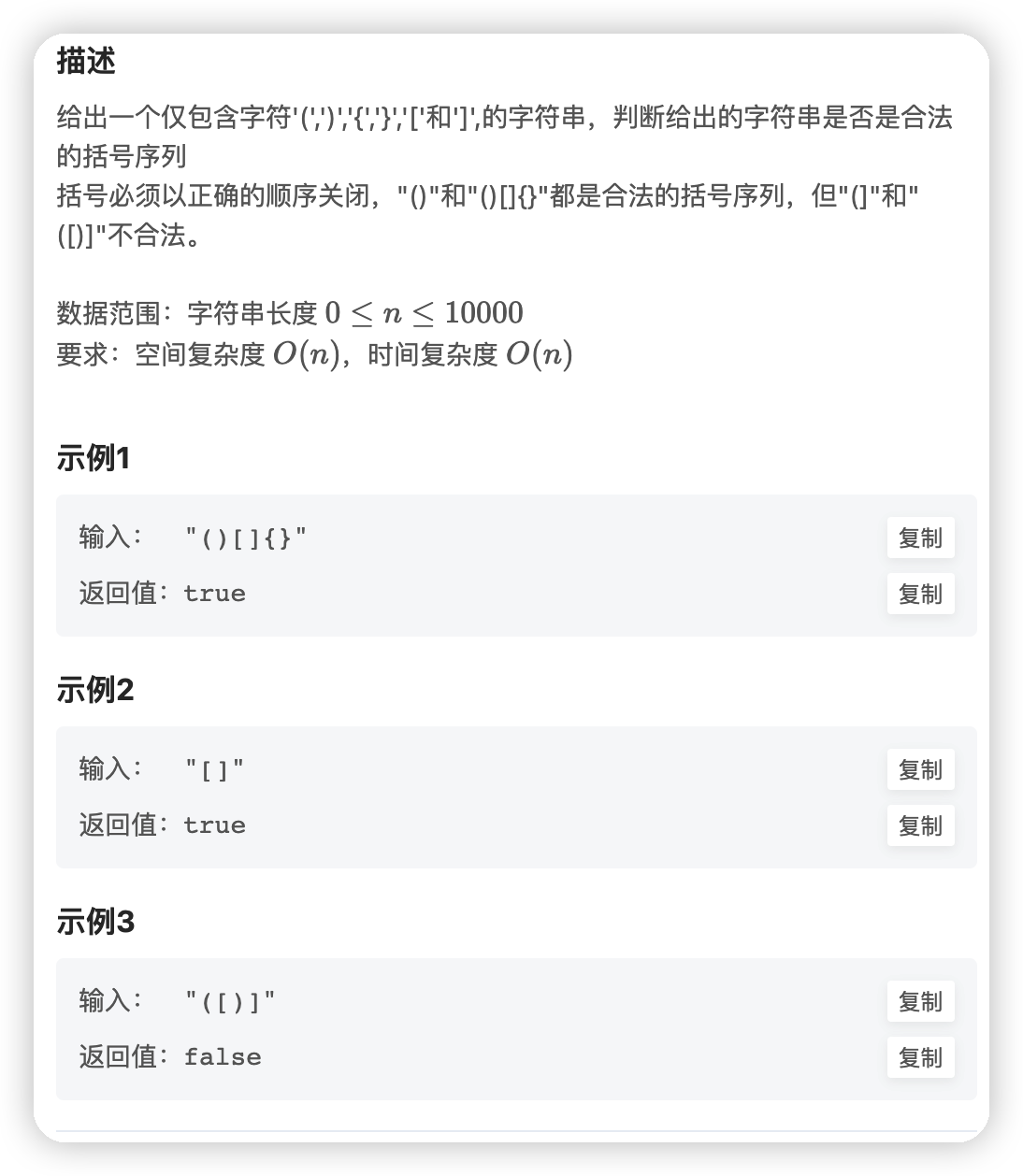
function isValid( s ) {
let stack=[];
for(let i=0;i<s.length;i++){
if(s[i]==='('||s[i]==='{'||s[i]==='['){
stack.push(s[i]);
}else{
if(s[i]===')'&&stack.pop()==='('||
s[i]===']'&&stack.pop()==='['||
s[i]==='}'&&stack.pop()==='{'){
continue;
}else{
return false;
}
}
}
if(stack.length!==0){
return false
}
return true
}
module.exports = {
isValid : isValid
};
BM46 最小的K个数
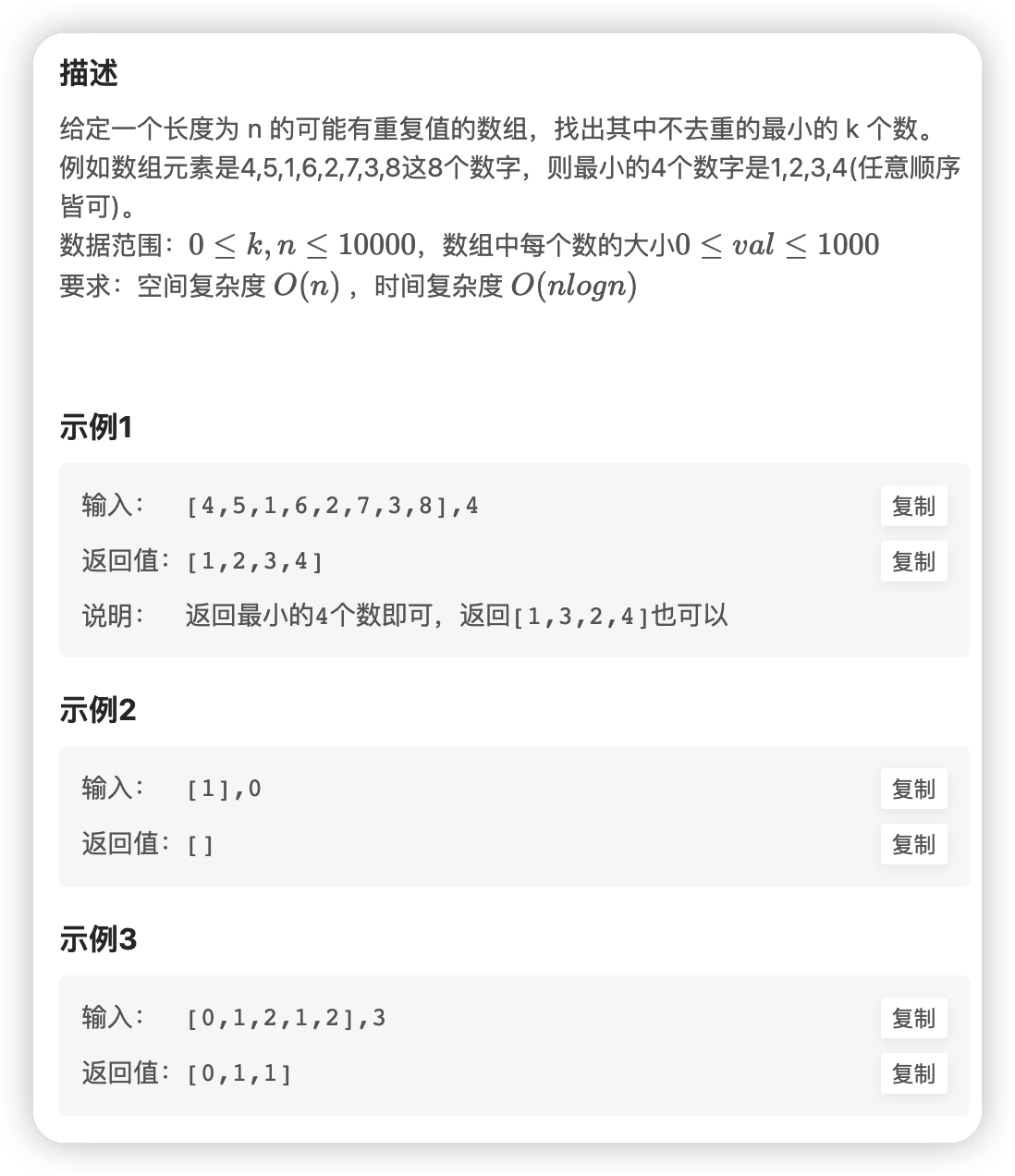
function GetLeastNumbers_Solution(input, k)
{
function swap(i,j){
let temp = heap[i];
heap[i] = heap[j];
heap[j] = temp;
}
function percDown(index,N){
let root = heap[index];
let parent,child;
for( parent=index; parent*2+1<N; parent=child){
child = parent*2+1;
if(child+1<N && heap[child+1]>heap[child])
child++;
if( root > heap[child] )
break;
else
heap[parent] = heap[child];
}
heap[parent] = root;
}
let heap = input.slice(0,k);
for(let i=Math.floor(k/2)-1; i>=0; i-- ){
percDown(i,k);
}
for(let i=k; i<input.length; i++){
if(input[i]<heap[0]){
heap[0] = input[i];
percDown(0,k);
}
}
return heap;
}
module.exports = {
GetLeastNumbers_Solution : GetLeastNumbers_Solution
};
BM47 寻找第K大
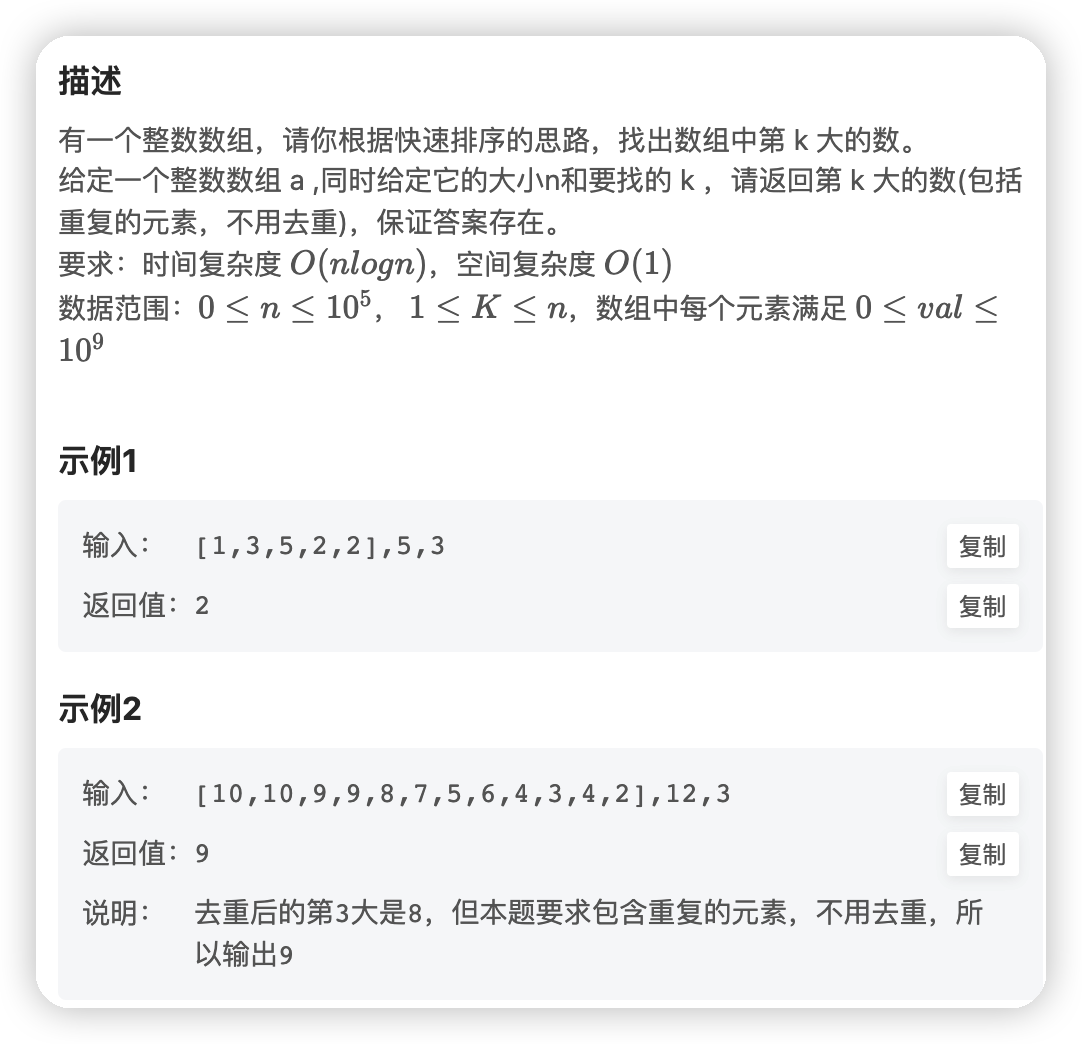
function findKth( a , n , K ) {
if(a.length===0) return 0;
return sortArray(a)[n-K]
}
function sortArray(nums){
let len=nums.length;
if(len===0) return nums;
const midIndex=Math.floor(len/2);
const midValue=nums.splice(midIndex,1)[0];
const left=[];
const right=[];
for(let i=0;i<nums.length;i++){
const n=nums[i];
if(n<midValue) left.push(n);
else right.push(n);
}
return sortArray(left).concat([midValue],sortArray(right))
}
module.exports = {
findKth : findKth
};
BM48 数据流中的中位数
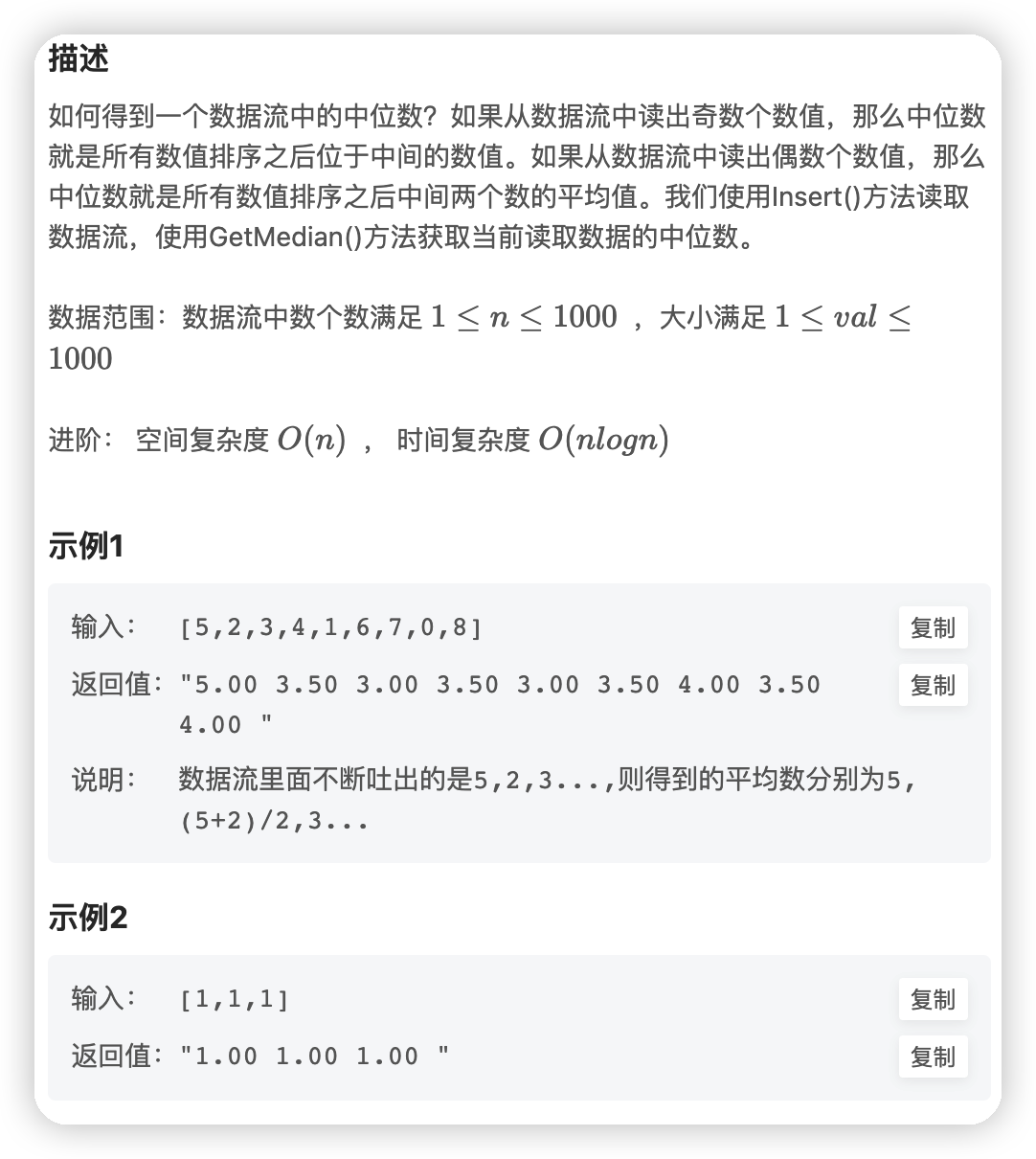
let arr=[];
function Insert(num)
{
let i=0;
while(arr[i]<num) i++;
arr.splice(i,0,num);
}
function GetMedian(){
let index = Math.floor( arr.length/2 )
if(arr.length%2){
return arr[index];
}else{
return ( arr[index] + arr[index-1] )/2 ;
}
}
module.exports = {
Insert : Insert,
GetMedian : GetMedian
};
BM49 表达式求值
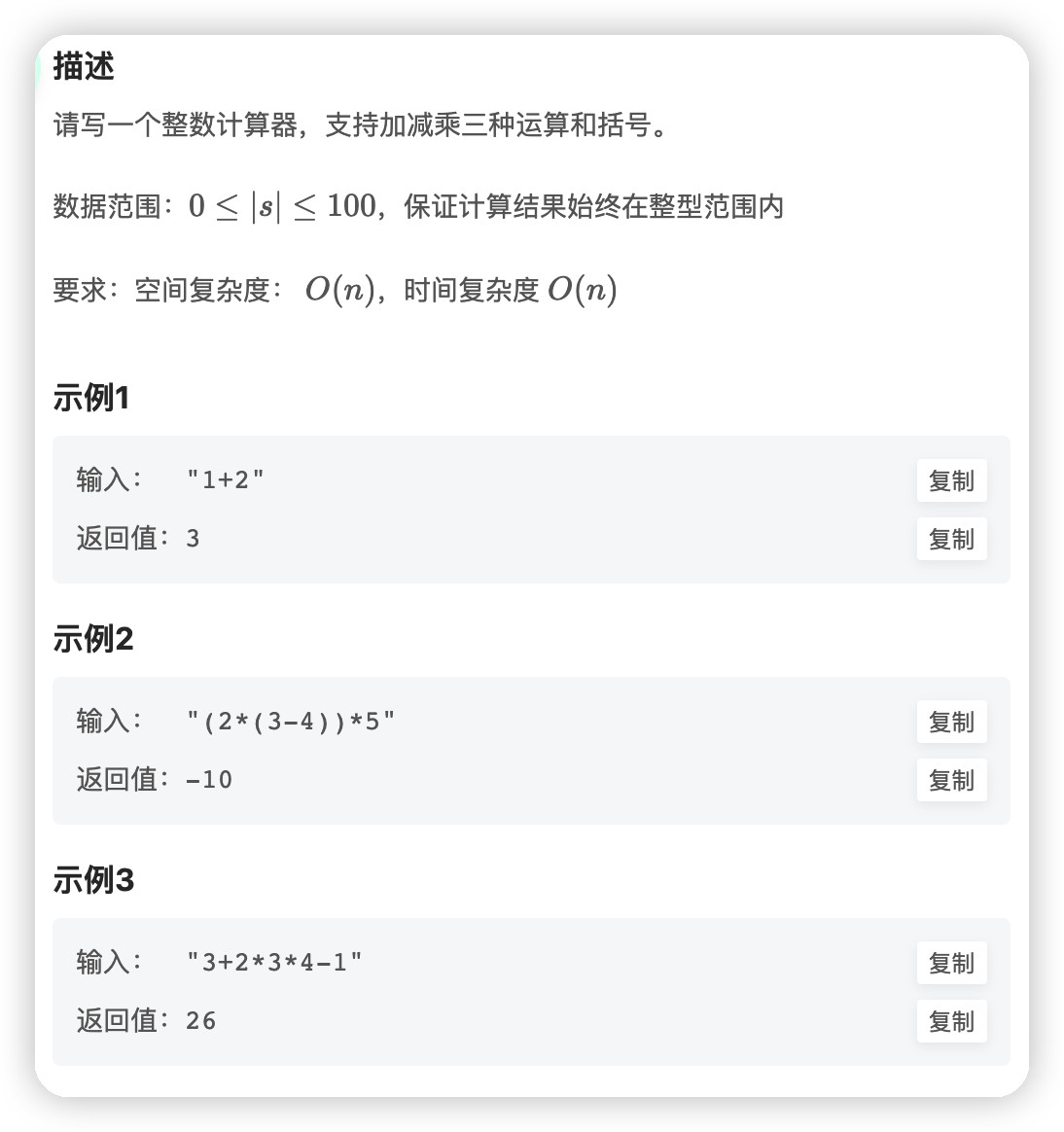