C/C++字符串操作
一、 C++字符串操作
头文件#include<string> using std::string
1、 构造函数
default (1) | string(); |
copy (2) | string (const string& str); |
substring (3) | string (const string& str, size_t pos, size_t len = npos); |
from c-string (4) | string (const char* s); |
from buffer (5) | string (const char* s, size_t n); |
fill (6) | string (size_t n, char c); |
2、 常用函数
string str = "copy";
char *ch = "chars";
//1 constructor
string str1;//default (1) string();
string str2(str); //copy (2) string (const string& str);
string str3("hello",1,2);//substring (3) string (const string& str, size_t pos,size_t len = npos);
string str4(ch);//from c-string (4) string(const char* s);
string str5(ch, 2); //from buffer (5) string(const char* s, size_t n);
string str6(10, 'c');// fill(6) string(size_tn, char c);
C++官网函数介绍
介绍几个常用的
1)c_str
// strings and c-strings
//补充strtok知识:
//函数原型:char *strtok(char *s,const char *delim)
//函数功能:分解字符串为一组字符串,s为要分解的字符串,delim为分隔字//符串
#include <iostream>
#include <cstring>
#include <string>
int main ()
{
std::string str ("Please split this sentence into tokens");
char * cstr = new char [str.length()+1];
std::strcpy (cstr, str.c_str());
// cstr now contains a c-string copy of str
char * p = std::strtok (cstr," ");
while (p!=0)
{
std::cout << p << '\n';
p = std::strtok(NULL," ");
}
delete[] cstr;
getchar();
return 0;
}
输出打印:
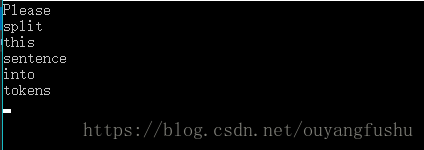
2)data
// string::data
//补充:C 库函数:
int memcmp(const void *str1, const void *str2, size_t n))
把存储区 str1 和存储区 str2 的前 n 个字节进行
如果返回值 < 0,则表示 str1 小于 str2。
如果返回值 > 0,则表示 str2 小于 str1。
如果返回值 = 0,则表示 str1 等于 str2。
#include <iostream>
#include <string>
#include <cstring>
int main ()
{
int length;
std::string str = "Test string";
char* cstr = "Test string";
if ( str.length() == std::strlen(cstr) )
{
std::cout << "str and cstr have the same length.\n";
if ( memcmp (cstr, str.data(), str.length() ) == 0 )
std::cout << "str and cstr have the same content.\n";
}
return 0;
}
Output:
str and cstr have the same length. str and cstr have the same content. |
3)copy()
size_t copy (char* s, size_t len, size_tpos = 0) const;
s: 指向要复制到的字符数组指针,目标字符数组
len:需要复制的长度
pos: 复制的起始位置
// string::copy
#include <iostream>
#include <string>
int main (){
char buffer[20];
std::string str ("Teststring...");
std::size_t length = str.copy(buffer,6,5);
buffer[length]='\0';
std::cout << "buffercontains: " << buffer<< '\n';
return 0;
}
输出:
buffer contains: string
4)find
string (1) | size_t find (const string& str, size_t pos = 0) const noexcept; |
c-string (2) | size_t find (const char* s, size_t pos = 0) const; |
buffer (3) | size_t find (const char* s, size_t pos, size_type n) const; |
character (4) | size_t find (char c, size_t pos = 0) const noexcept; |
str:需要查找的目标字符串
pos:查找起始位置
s:指向要查找字符数组的指针
n:要查找的字符数组的长度
c:要查找的单个字符
返回:The position of the first characterof the first match.
If no matches were found, the function returns string::npos.
// string::find
#include <iostream> // std::cout
#include <string> // std::string
int main (){
std::string str ("Thereare two needles in this haystack with needles.");
std::string str2 ("needle");
// different member versions of find in thesame order as above:
std::size_t found = str.find(str2);
if (found!=std::string::npos)
std::cout << "first'needle' found at: "<< found << '\n';
found=str.find("needlesare small",found+1,6);
if (found!=std::string::npos)
std::cout << "second'needle' found at: "<< found << '\n';
found=str.find("haystack");
if (found!=std::string::npos)
std::cout << "'haystack'also found at: "<< found << '\n';
found=str.find('.');
if (found!=std::string::npos)
std::cout << "Periodfound at: " << found<< '\n';
// let's replace the first needle:
str.replace(str.find(str2),str2.length(),"preposition");
std::cout << str << '\n';
return 0;
}
输出:
first 'needle' found at: 14
second 'needle' found at: 44
'haystack' also found at: 30
Period found at: 51
There are two prepositions in this haystack with needles.
5) find first of
string (1) | size_t find_first_of (const string& str, size_t pos = 0) const noexcept; | |
c-string(2) | size_t find_first_of (const char* s, size_t pos = 0) const; | |
buffer (3) | size_t find_first_of (const char* s, size_t pos, size_t n) const; | |
character (4) | size_t find_first_of (char c, size_t pos = 0) const noexcept; |
参数:
Str 需要查找的目标字符
Pos 查找的起始位置
S 指向字符数组的指针
N 需要查找的字符个数
C 需要查找的单个字符
返回:返回匹配到的第一个字符的索引号.
size_t is an unsigned integral type (the sameas member type string::size_type).
#include <iostream> // std::cout
#include <string> // std::string
#include <cstddef> // std::size_t
int main ()
{
std::string str ("Please, replace the vowels in this sentence by asterisks.");
std::size_t found = str.find_first_of("aeiou");
while (found!=std::string::npos)
{
str[found]='*';
found=str.find_first_of("aeiou",found+1);
}
std::cout << str << '\n';
return 0;
}
注意该函数与find的区别:如果str1中含有str2中的任何字符,则就会查找成功,而find则不同。
6) find_last_of
string (1) | size_t find_last_of (const string& str, size_t pos = 0) const noexcept; | |
c-string(2) | size_t find_last_of (const char* s, size_t pos = 0) const; | |
buffer (3) | size_t find_last_of (const char* s, size_t pos, size_t n) const; | |
character (4) | size_t find_last_of (char c, size_t pos = 0) const noexcept; |
该函数参数与find_first_of 基本一致,顾名思义返回的是查找到与目标字符最后一个匹配成功的索引。
// string::find_last_of
#include <iostream> // std::cout
#include <string> // std::string
#include <cstddef> // std::size_t
//分割字符串,路径与文件名的分割
void SplitFilename (const std::string& str, std::string splittext )
{
std::cout << "Splitting: " << str << '\n';
std::size_t found = str.find_last_of(splittext);
std::cout << " path: " << str.substr(0,found) << '\n';
std::cout << " file: " << str.substr(found+1) << '\n';
}
int main ()
{
std::string str1 ("/usr/bin/man","/\\");
std::string str2 ("c:\\windows\\winhelp.exe","/\\");
SplitFilename (str1);
SplitFilename (str2);
return 0;
}
输出:
Splitting: /usr/bin/man
path:/usr/bin
file:man
Splitting: c:\windows\winhelp.exe
path:c:\windows
file:winhelp.exe
7)std::string::substr
string substr (size_t pos = 0, size_t len = npos) const;
参数:
Pos 子串在字符串的其实位置
Len 子串的长度,字符个数 默认为以pos为起始的剩下所有字符
返回:该函数返回字符串的子串
// string::substr
#include <iostream>
#include <string>
int main ()
{
std::string str="We think in generalities, but we live in details."; // (quoting Alfred N. Whitehead)
std::string str2 = str.substr (3,5); // "think"
std::size_t pos = str.find("live"); // position of "live" in str
std::string str3 = str.substr (pos); // get from "live" to the end
std::cout << str2 << ' ' << str3 << '\n';
return 0;
}
7)std::string::compare
string (1) | int compare (const string& str) const noexcept; |
substrings (2) | int compare (size_t pos, size_t len, const string& str) const; int compare (size_t pos, size_t len, const string& str, size_t subpos, size_t sublen) const; |
c-string (3) | int compare (const char* s) const; int compare (size_t pos, size_t len, const char* s) const; |
buffer (4) | int compare (size_t pos, size_t len, const char* s, size_t n) const; |
Str 需要比较的目标字符串
Pos 字符串起始位置,该起始位置为待比较的字符串
Len 待比较的字符串相对Pos的长度(单位:单个字符)
subpos,sublen 参数中字符串的起始位置以及长度
s 指向字符数组的指针
n 需要比较的字符的个数
// comparing apples with apples
#include <iostream>
#include <string>
int main ()
{
std::string str1 ("green apple");
std::string str2 ("red apple");
if (str1.compare(str2) != 0)
std::cout << str1 << " is not " << str2 << '\n';
if (str1.compare(6,5,"apple") == 0)
std::cout << "still, " << str1 << " is an apple\n";
if (str2.compare(str2.size()-5,5,"apple") == 0)
std::cout << "and " << str2 << " is also an apple\n";
if (str1.compare(6,5,str2,4,5) == 0)
std::cout << "therefore, both are apples\n";
return 0;
}
输出;
green apple is not red apple
still, green apple is an apple
and red apple is also an apple
therefore, both are apples
二、 C语言字符串操作
有点懒惰了,参考这一篇博客(比较全面的总结):https://blog.csdn.net/xfjjs_net/article/details/79329030
摘抄出函数接口:
1)字符串操作
strcpy(p, p1) 复制字符串
strncpy(p, p1, n) 复制指定长度字符串
strcat(p, p1) 附加字符串
strncat(p, p1, n) 附加指定长度字符串
strlen(p) 取字符串长度
strcmp(p, p1) 比较字符串
strcasecmp忽略大小写比较字符串
strncmp(p, p1, n) 比较指定长度字符串
strchr(p, c) 在字符串中查找指定字符
strrchr(p, c) 在字符串中反向查找
strstr(p, p1) 查找字符串
strpbrk(p, p1) 以目标字符串的所有字符作为集合,在当前字符串查找该集合的任一元素
strspn(p, p1) 以目标字符串的所有字符作为集合,在当前字符串查找不属于该集合的任一元素的偏移
strcspn(p, p1) 以目标字符串的所有字符作为集合,在当前字符串查找属于该集合的任一元素的偏移
* 具有指定长度的字符串处理函数在已处理的字符串之后填补零结尾符
2)字符串到数值类型的转换 (我比较关心这个,数据)
strtod(p, ppend) 从字符串 p 中转换 double 类型数值,并将后续的字符串指针存储到 ppend 指向的 char* 类型存储。
strtol(p, ppend, base) 从字符串 p 中转换 long 类型整型数值,base 显式设置转换的整型进制,设置为 0 以根据特定格式判断所用进制,0x, 0X 前缀以解释为十六进制格式整型,0 前缀以解释为八进制格式整型
atoi(p) 字符串转换到 int 整型
atof(p) 字符串转换到 double 符点数
atol(p) 字符串转换到 long 整型
3)字符检查
isalpha() 检查是否为字母字符
isupper() 检查是否为大写字母字符
islower() 检查是否为小写字母字符
isdigit() 检查是否为数字
isxdigit() 检查是否为十六进制数字表示的有效字符
isspace() 检查是否为空格类型字符
iscntrl() 检查是否为控制字符
ispunct() 检查是否为标点符号
isalnum() 检查是否为字母和数字
isprint() 检查是否是可打印字符
isgraph() 检查是否是图形字符,等效于 isalnum() |ispunct()
每个函数详细介绍见上面的参考链接:https://blog.csdn.net/xfjjs_net/article/details/79329030