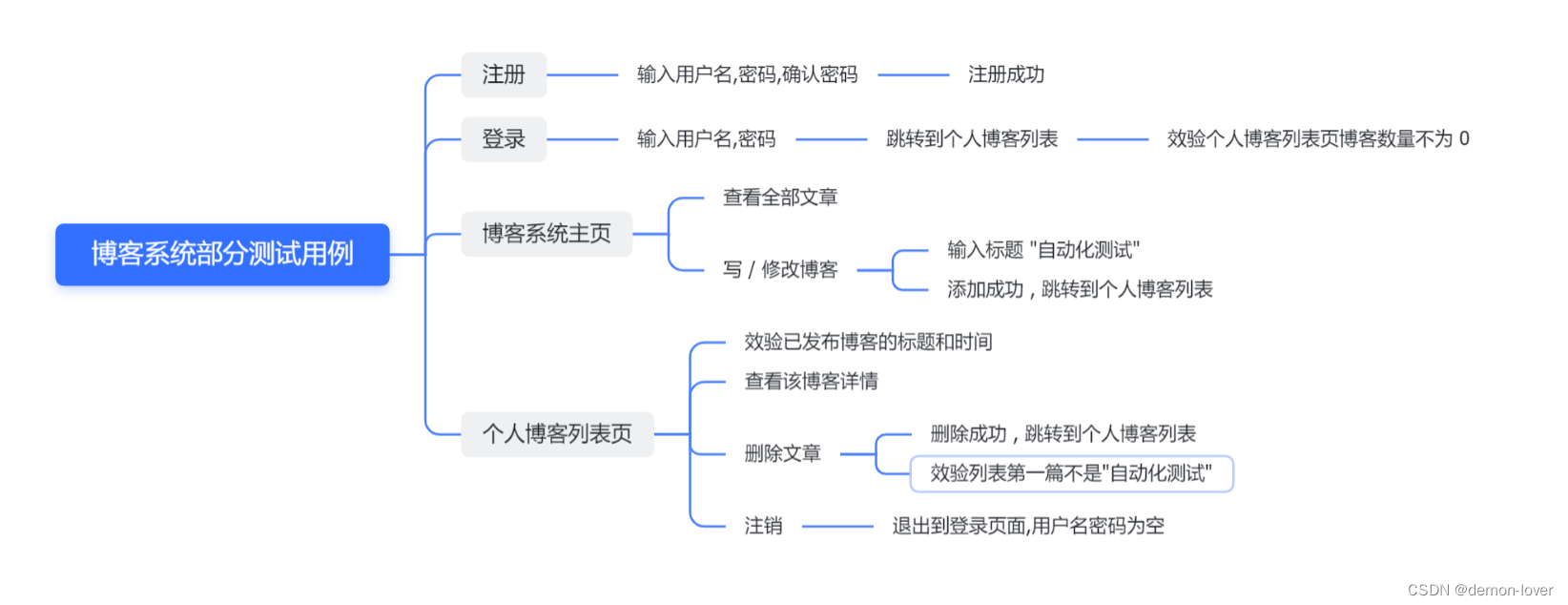
用户注册
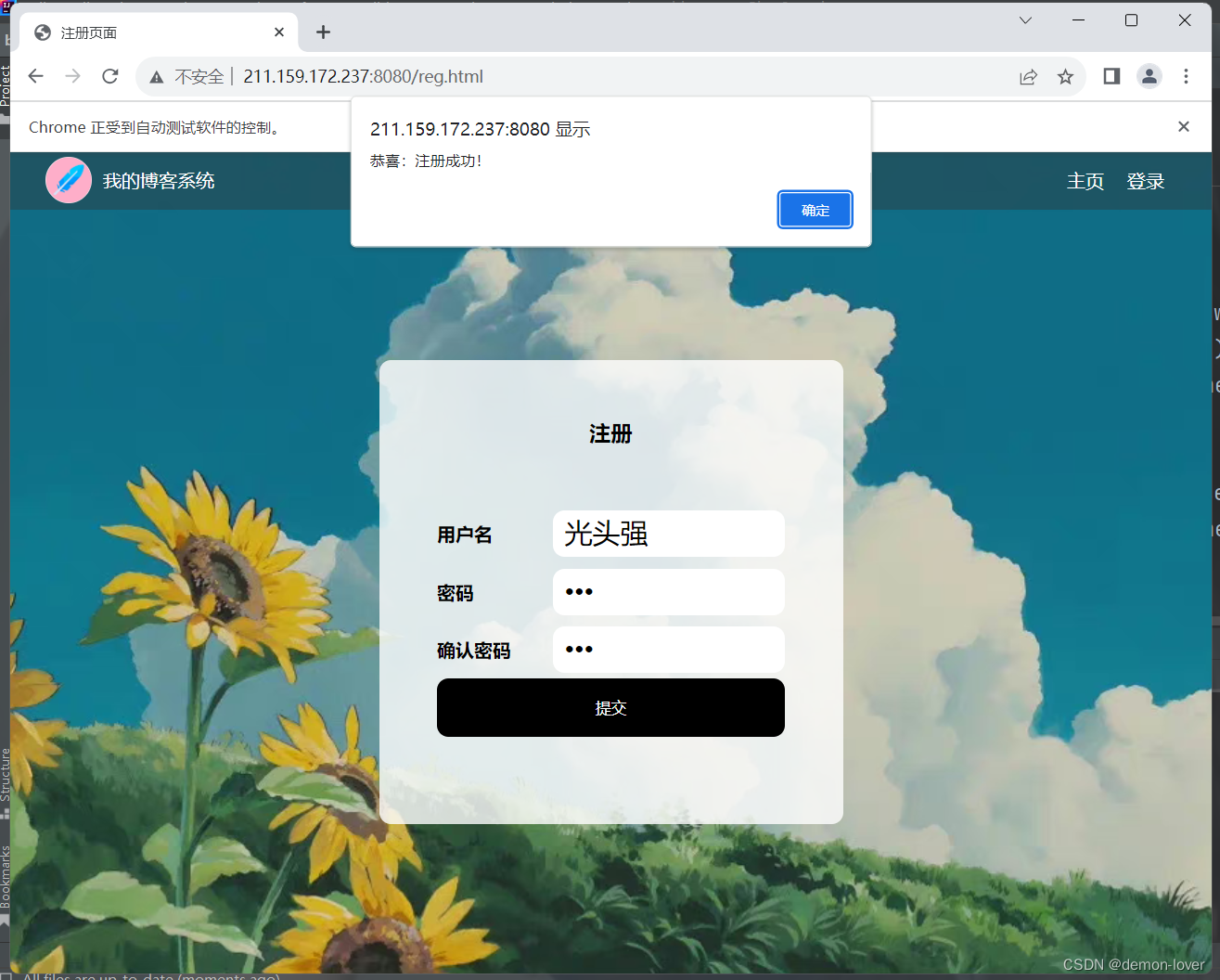
@Order(1)
@ParameterizedTest
@CsvFileSource(resources = "regmes.csv")
void Reg(String username , String password , String password2) throws InterruptedException {
webDriver.get("http://211.159.172.237:8080/reg.html");
webDriver.manage().timeouts().implicitlyWait(3,TimeUnit.SECONDS);
webDriver.findElement(By.cssSelector("#username")).sendKeys(username);
webDriver.manage().timeouts().implicitlyWait(3,TimeUnit.SECONDS);
webDriver.findElement(By.cssSelector("#password")).sendKeys(password);
webDriver.manage().timeouts().implicitlyWait(3,TimeUnit.SECONDS);
webDriver.findElement(By.cssSelector("#password2")).sendKeys(password2);
webDriver.manage().timeouts().implicitlyWait(3,TimeUnit.SECONDS);
sleep(2000);
webDriver.findElement(By.cssSelector("#submit")).click();
webDriver.manage().timeouts().implicitlyWait(3,TimeUnit.SECONDS);
sleep(2000);
webDriver.switchTo().alert().accept();
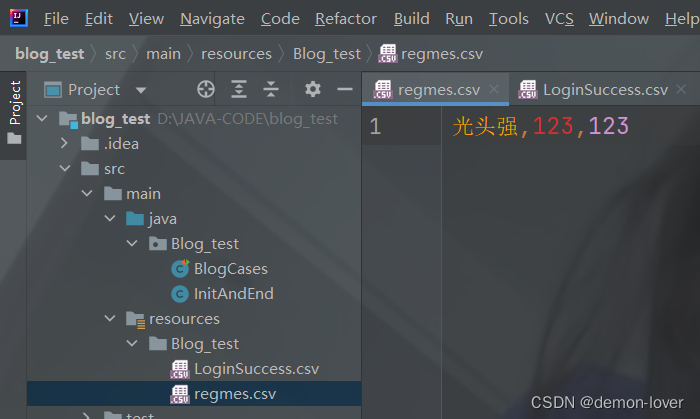
登录验证
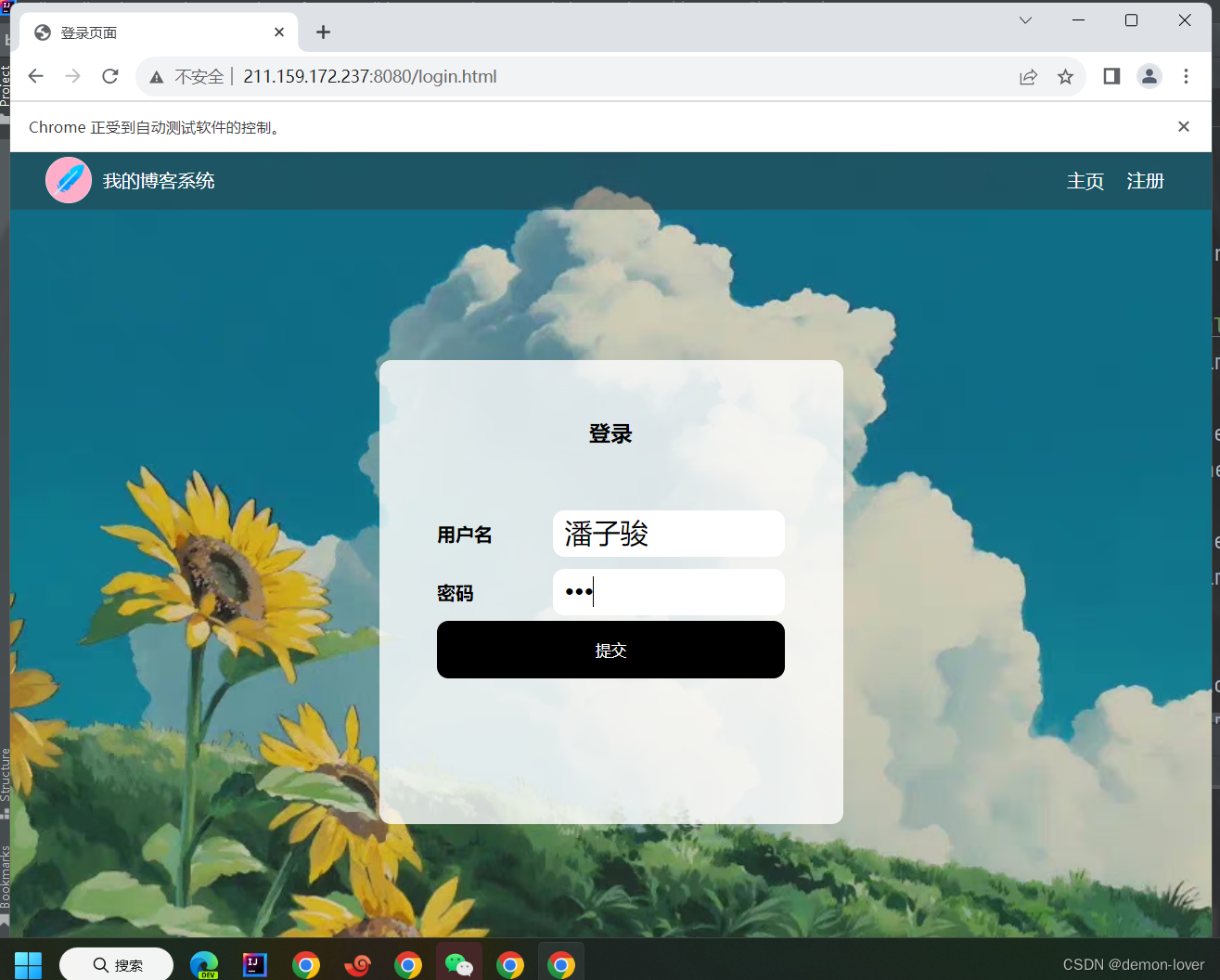
@Order(2)
@ParameterizedTest
@CsvFileSource(resources = "LoginSuccess.csv")
void LoginSuccess(String username , String password , String blog_list_url) throws InterruptedException {
webDriver.get("http://211.159.172.237:8080/login.html");
webDriver.manage().timeouts().implicitlyWait(3, TimeUnit.SECONDS);
webDriver.findElement(By.cssSelector("#username")).sendKeys(username);
webDriver.manage().timeouts().implicitlyWait(3,TimeUnit.SECONDS);
webDriver.findElement(By.cssSelector("#password")).sendKeys(password);
webDriver.manage().timeouts().implicitlyWait(3, TimeUnit.SECONDS);
sleep(3000);
webDriver.findElement(By.cssSelector("#submit")).click();
webDriver.manage().timeouts().implicitlyWait(3, TimeUnit.SECONDS);
sleep(1000);
String cur_url = webDriver.getCurrentUrl();
Assertions.assertEquals(blog_list_url, cur_url);
}
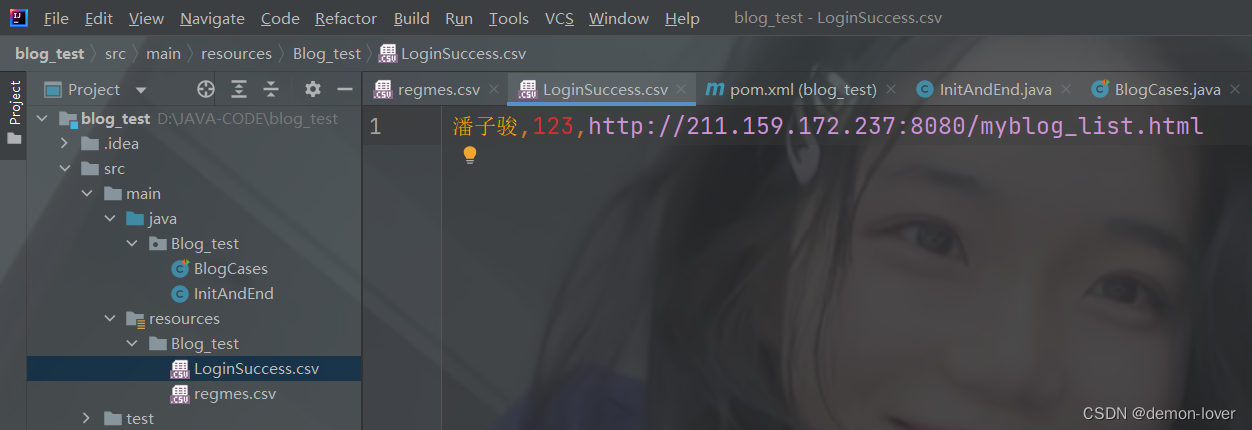
效验个人博客列表页博客数量不为 0
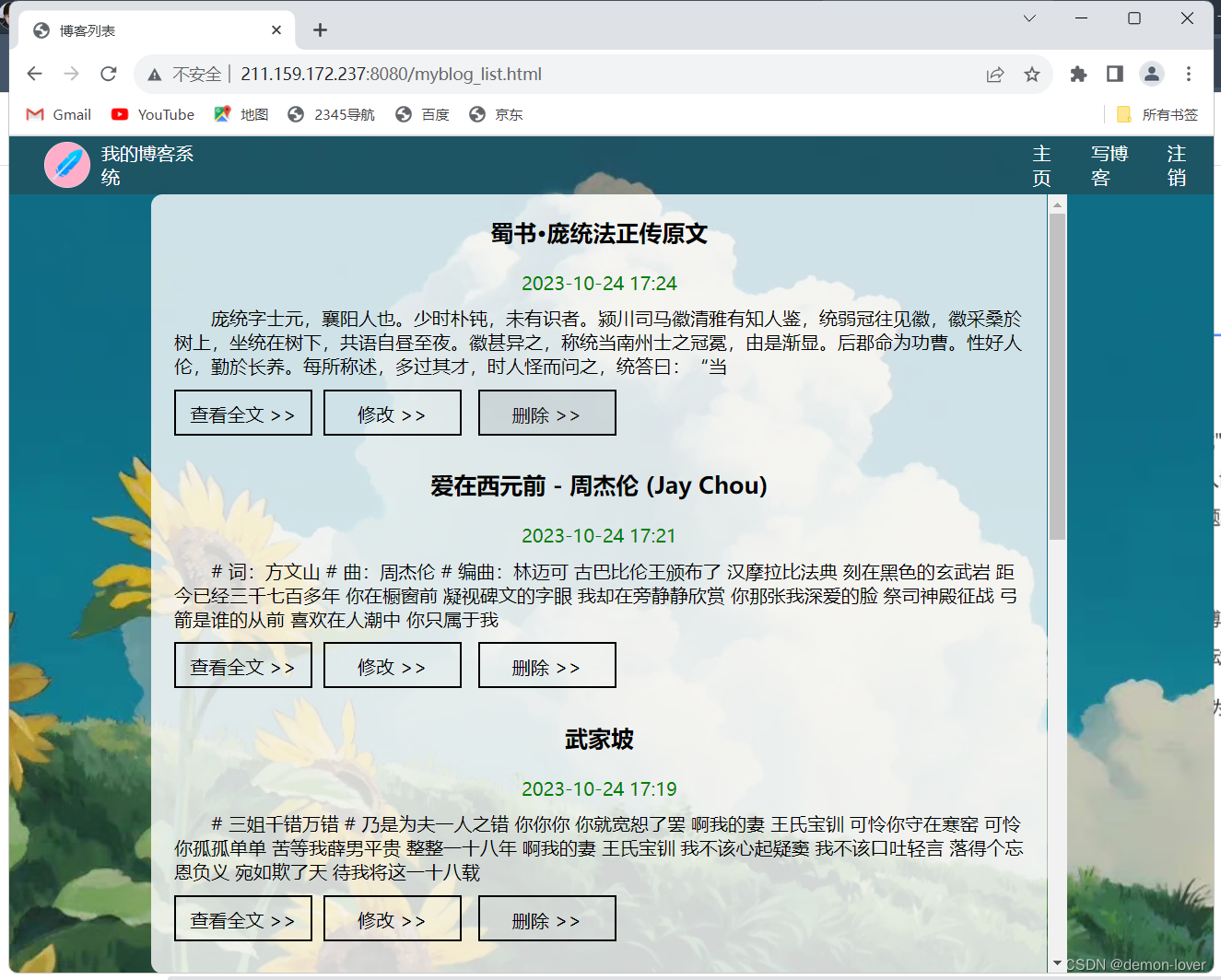
@Order(3)
@Test
void MyBlogList() throws InterruptedException {
webDriver.manage().timeouts().implicitlyWait(3,TimeUnit.SECONDS);
sleep(3000);
int title_num = webDriver.findElements(By.cssSelector(".title")).size();
Assertions.assertNotEquals(0,title_num);
}
博客系统主页
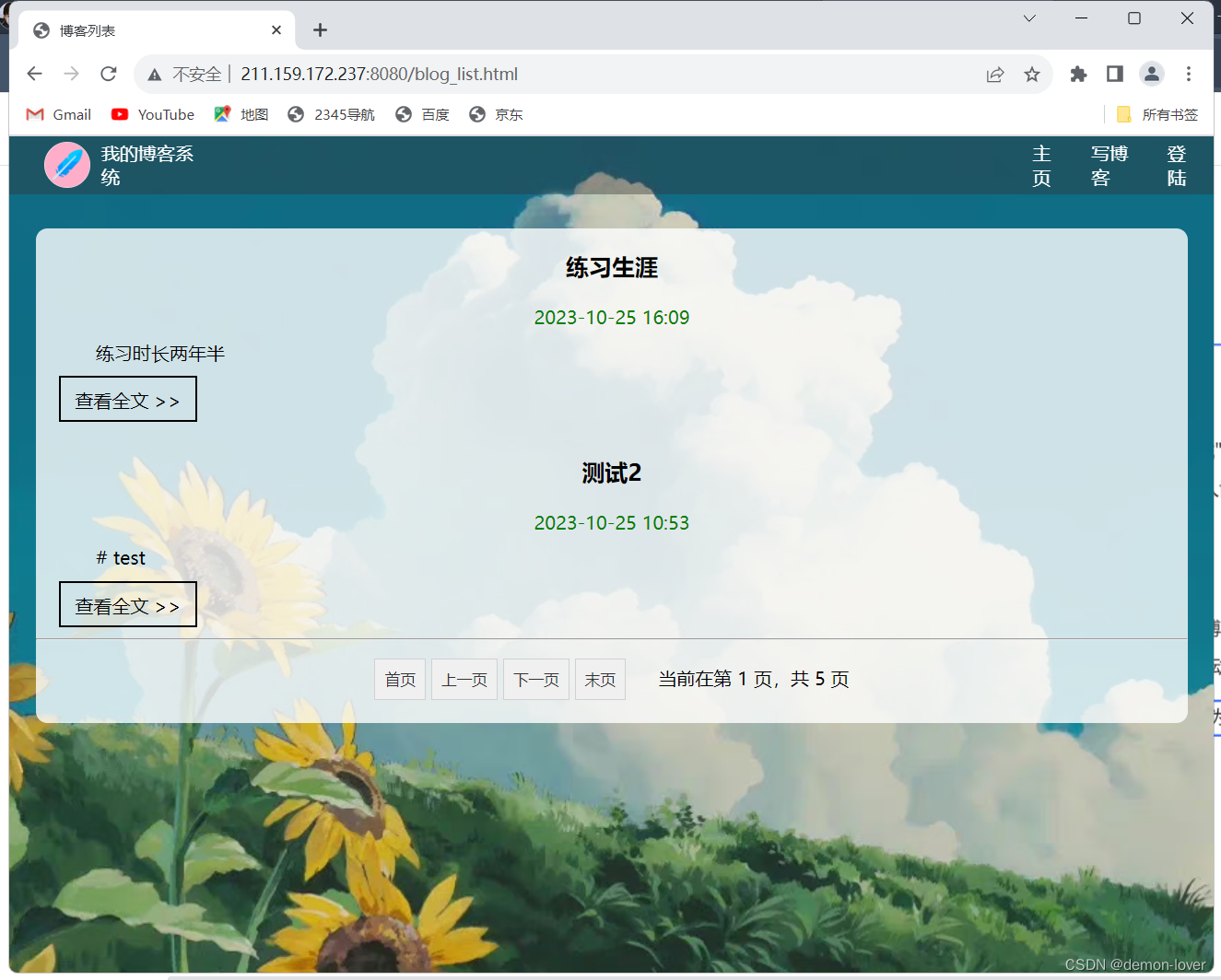
写博客
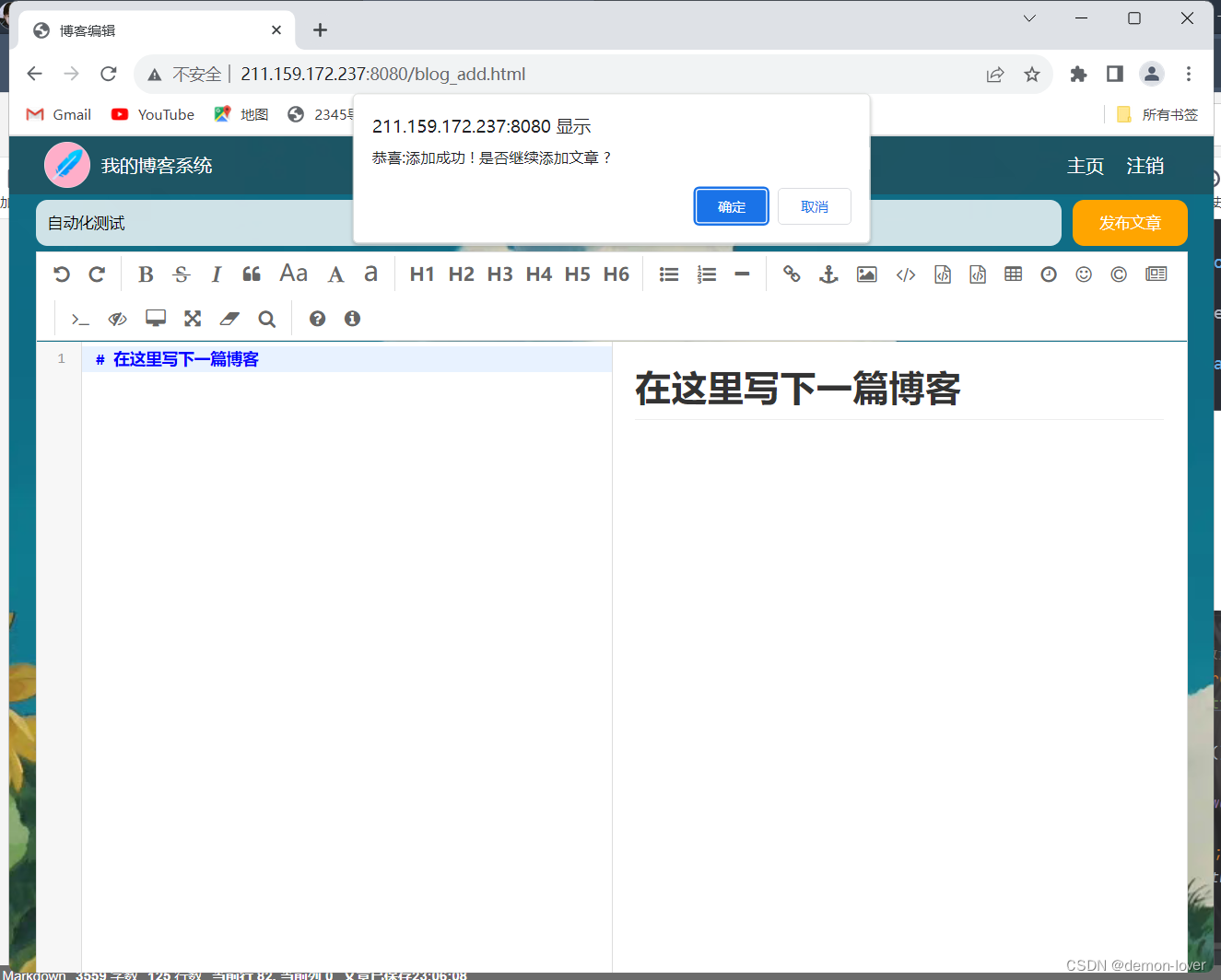
@Order(4)
@Test
void AddBlog() throws InterruptedException {
webDriver.findElement(By.cssSelector("body > div.nav > a:nth-child(4)")).click();
webDriver.manage().timeouts().implicitlyWait(3,TimeUnit.SECONDS);
sleep(3000);
webDriver.findElement(By.cssSelector("body > div.nav > a:nth-child(5)")).click();
webDriver.manage().timeouts().implicitlyWait(3,TimeUnit.SECONDS);
((JavascriptExecutor)webDriver).executeScript("document.getElementById(\"title\").value=\"自动化测试\"");
sleep(1500);
webDriver.findElement(By.cssSelector("body > div.blog-edit-container > div.title > button")).click();
sleep(1500);
webDriver.manage().timeouts().implicitlyWait(3,TimeUnit.SECONDS);
webDriver.switchTo().alert().dismiss();
String cur_url = webDriver.getCurrentUrl();
Assertions.assertEquals("http://211.159.172.237:8080/myblog_list.html",cur_url);
sleep(1500);
}
我的博客列表页
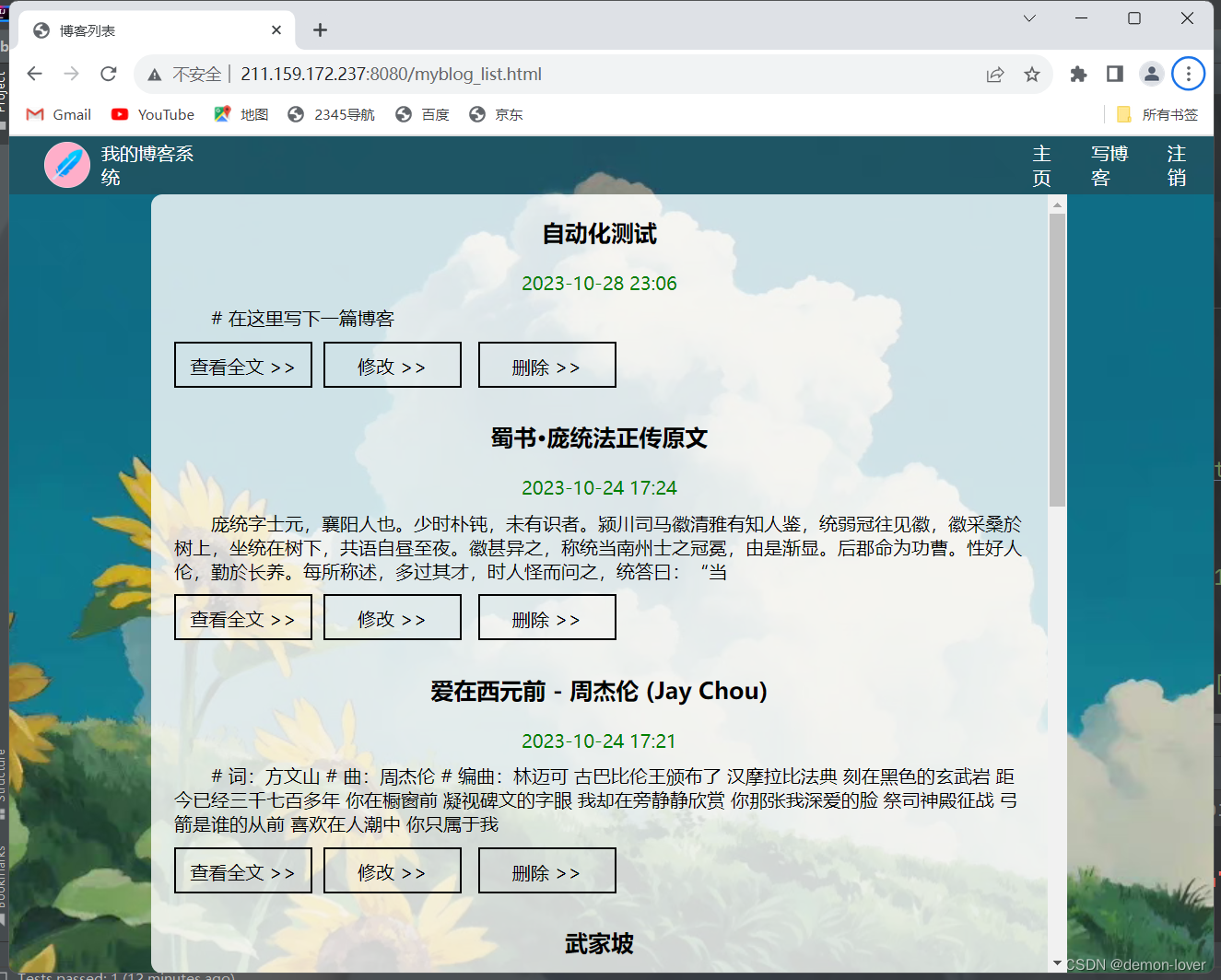
效验 刚发布的博客的标题和时间
@Order(5)
@Test
void BlogInfoChecked() throws InterruptedException {
webDriver.get("http://211.159.172.237:8080/myblog_list.html");
String first_blog_title = webDriver.findElement
(By.cssSelector("#artListDiv > div:nth-child(1) > div.title")).getText();
String first_blog_time = webDriver.findElement
(By.xpath("//*[@id=\"artListDiv\"]/div[1]/div[2]")).getText();
Assertions.assertEquals("自动化测试",first_blog_title);
sleep(3000);
if(first_blog_time.contains("2023-10-28")) {
System.out.println("时间正确");
}else {
System.out.println("当前时间是 :" + first_blog_time);
System.out.println("时间错误");
}
}
查看 文章详情页
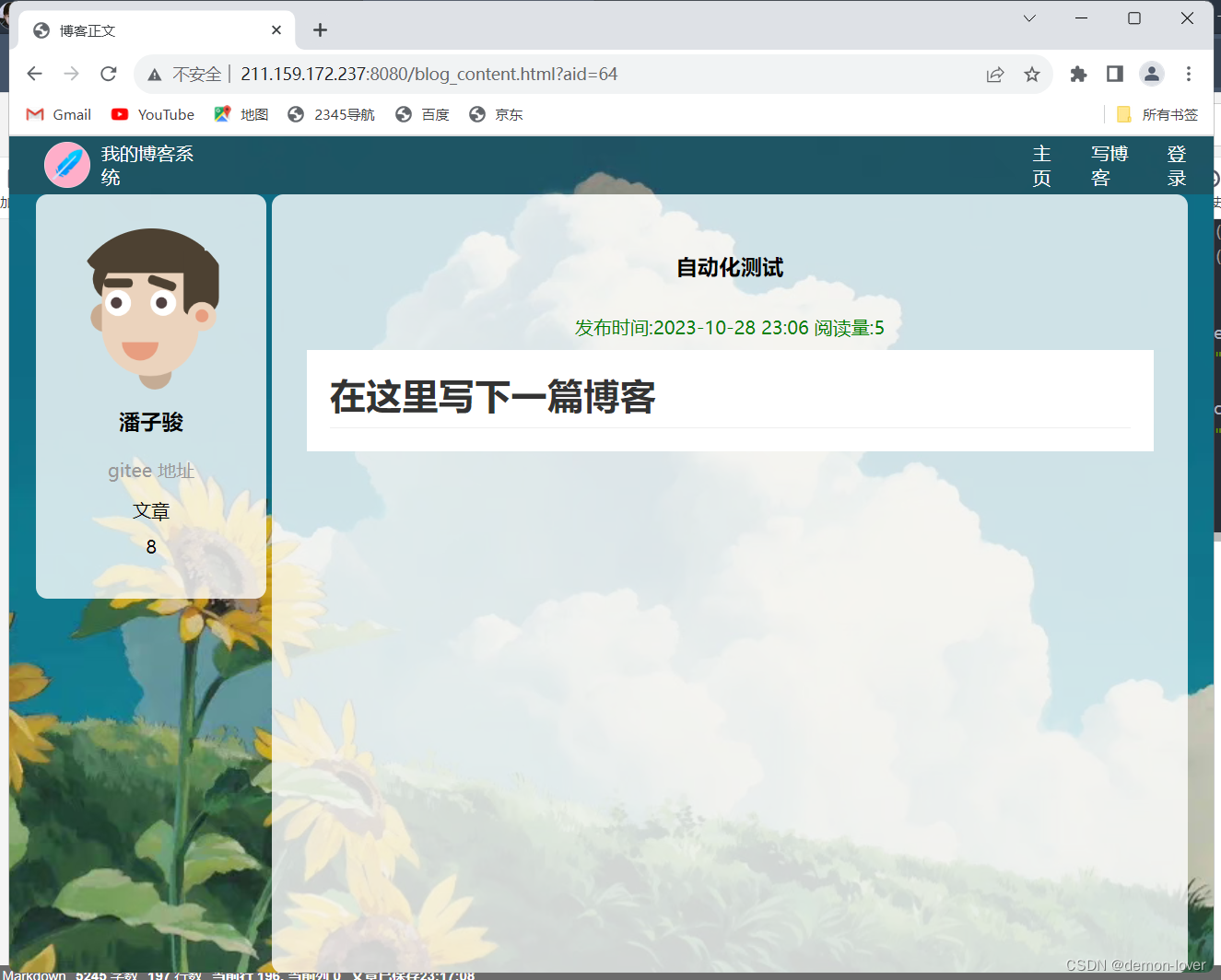
@TestMethodOrder(MethodOrderer.OrderAnnotation.class)
public class BlogCases extends InitAndEnd{
public static Stream<Arguments> Generator() {
return Stream.of(Arguments.arguments("http://211.159.172.237:8080/blog_content.html",
"博客正文","自动化测试"));
}
@Order(6)
@ParameterizedTest
@MethodSource("Generator")
void BlogContent(String expected_url , String expected_title , String expected_blog_title) throws InterruptedException {
webDriver.findElement(By.xpath("//*[@id=\"artListDiv\"]/div[1]/a[1]")).click();
sleep(3000);
String cur_url = webDriver.getCurrentUrl();
webDriver.manage().timeouts().implicitlyWait(3,TimeUnit.SECONDS);
String cur_title = webDriver.getTitle();
webDriver.manage().timeouts().implicitlyWait(3,TimeUnit.SECONDS);
String blog_title = webDriver.findElement(By.cssSelector("#title")).getText();
webDriver.manage().timeouts().implicitlyWait(3,TimeUnit.SECONDS);
Assertions.assertEquals(expected_title , cur_title);
Assertions.assertEquals(expected_blog_title , blog_title);
if(cur_url.contains(expected_url)) {
System.out.println("博客详情正确");
} else {
System.out.println(cur_url);
System.out.println("博客详情失败");
}
sleep(1500);
}
删除文章
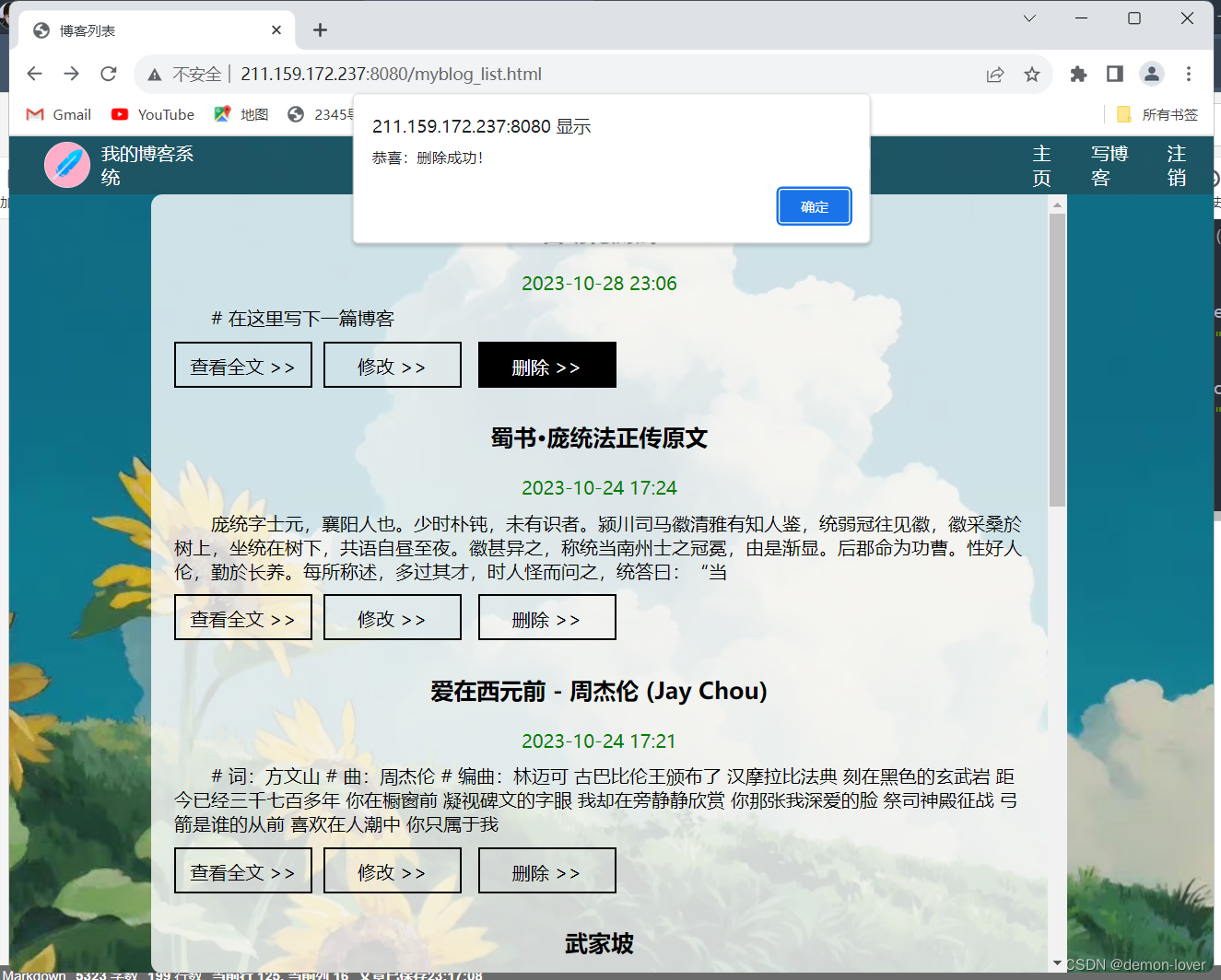
效验第一篇博客 不是 “自动化测试”
@Order(7)
@Test
void DeleteBlog() throws InterruptedException {
webDriver.get("http://211.159.172.237:8080/myblog_list.html");
webDriver.manage().timeouts().implicitlyWait(3,TimeUnit.SECONDS);
webDriver.findElement(By.cssSelector("#artListDiv > div:nth-child(1) > a:nth-child(6)")).click();
webDriver.manage().timeouts().implicitlyWait(3,TimeUnit.SECONDS);
sleep(3000);
webDriver.switchTo().alert().accept();
String first_blog_title = webDriver.findElement
(By.cssSelector("#artListDiv > div:nth-child(1) > div.title")).getText();
webDriver.manage().timeouts().implicitlyWait(3,TimeUnit.SECONDS);
Assertions.assertNotEquals("自动化测试",first_blog_title);
sleep(3000);
}
注销
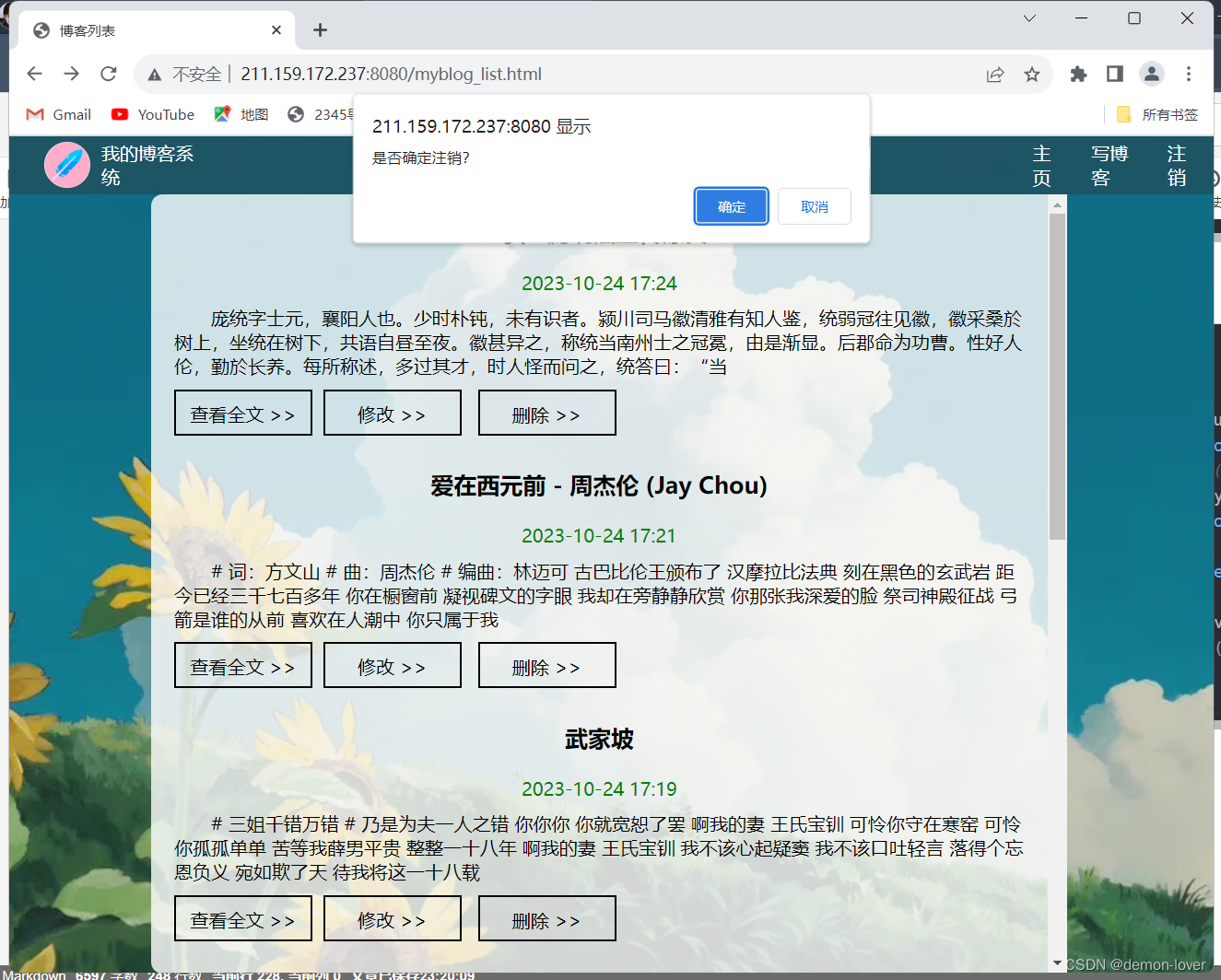
@Order(8)
@Test
void Logout() throws InterruptedException {
webDriver.manage().timeouts().implicitlyWait(3, TimeUnit.SECONDS);
webDriver.findElement(By.xpath("/html/body/div[1]/a[3]")).click();
webDriver.manage().timeouts().implicitlyWait(3, TimeUnit.SECONDS);
webDriver.switchTo().alert().accept();
sleep(3000);
String cur_url = webDriver.getCurrentUrl();
Assertions.assertEquals("http://211.159.172.237:8080/login.html",cur_url);
}
退出到登录页面,用户名密码为空
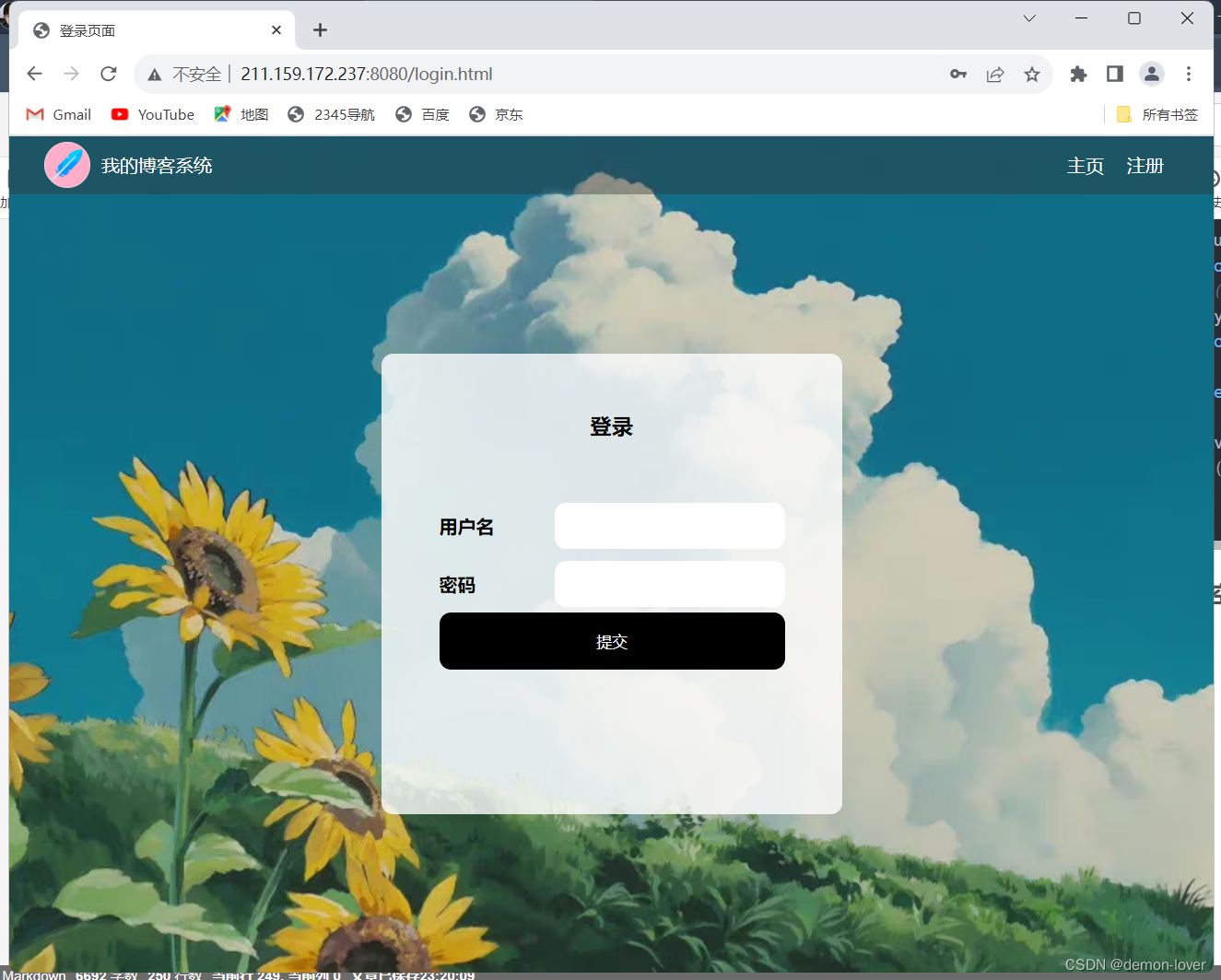