代码布局
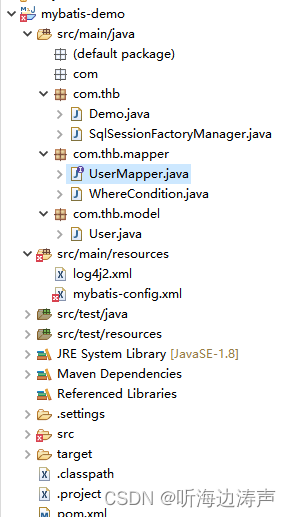
数据库user表
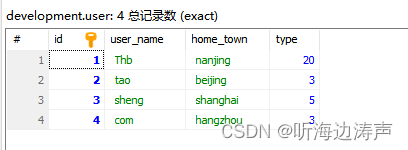
pom.xml文件中的依赖
<dependencies>
<!-- https://mvnrepository.com/artifact/org.mybatis/mybatis -->
<dependency>
<groupId>org.mybatis</groupId>
<artifactId>mybatis</artifactId>
<version>3.5.14</version>
</dependency>
<!-- https://mvnrepository.com/artifact/com.mysql/mysql-connector-j -->
<dependency>
<groupId>com.mysql</groupId>
<artifactId>mysql-connector-j</artifactId>
<version>8.2.0</version>
</dependency>
<dependency>
<groupId>org.apache.logging.log4j</groupId>
<artifactId>log4j-core</artifactId>
<version>2.20.0</version>
</dependency>
</dependencies>
mybatis-config.xml文件
<?xml version="1.0" encoding="UTF-8"?>
<!DOCTYPE configuration
PUBLIC "-//mybatis.org//DTD Config 3.0//EN"
"https://mybatis.org/dtd/mybatis-3-config.dtd">
<configuration>
<properties>
<property name="driver" value="com.mysql.cj.jdbc.Driver"/>
<property name="url" value="jdbc:mysql://localhost:3306/development"/>
<property name="username" value="development_user"/>
<property name="password" value="test0009"/>
</properties>
<environments default="development">
<environment id="development">
<transactionManager type="JDBC"/>
<dataSource type="POOLED">
<property name="driver" value="${driver}"/>
<property name="url" value="${url}"/>
<property name="username" value="${username}"/>
<property name="password" value="${password}"/>
</dataSource>
</environment>
</environments>
<mappers>
<mapper class="com.thb.mapper.UserMapper"/>
</mappers>
</configuration>
log4j2.xml文件
<?xml version="1.0" encoding="UTF-8"?>
<Configuration status="WARN">
<Appenders>
<Console name="Console" target="SYSTEM_OUT">
<PatternLayout pattern="%d{HH:mm:ss.SSS} [%t] %-5level %logger{36} - %msg%n"/>
</Console>
</Appenders>
<Loggers>
<Root level="trace">
<AppenderRef ref="Console"/>
</Root>
</Loggers>
</Configuration>
UserMapper.java文件
package com.thb.mapper;
import java.util.List;
import java.util.StringJoiner;
import org.apache.ibatis.annotations.SelectProvider;
import org.apache.ibatis.annotations.Param;
import org.apache.ibatis.annotations.Result;
import org.apache.ibatis.annotations.Results;
import org.apache.ibatis.jdbc.SQL;
import com.thb.model.User;
public interface UserMapper {
@Results(value = {
@Result(property = "id", column = "id", id = true),
@Result(property = "userName", column = "user_name"),
@Result(property = "homeTown", column = "home_town"),
@Result(property = "type", column = "type"),
})
@SelectProvider(type = UserSqlBuilder.class, method = "selectManyUsers")
List<User> selectManyUsers(@Param("whereConditions")List<WhereCondition> whereConditions, @Param("offset")int offset, @Param("limit")int limit);
public static class UserSqlBuilder {
public static String selectManyUsers(@Param("whereConditions")final List<WhereCondition> whereConditions, @Param("offset")final int offset, @Param("limit")final int limit) {
return new SQL() {{
SELECT("id, user_name, home_town, type");
FROM("user");
WhereCondition whereCondition = null;
String columnName;
String operator;
String compareValue;
for (int i = 0; i < whereConditions.size(); i++) {
whereCondition = whereConditions.get(i);
if (whereCondition.getOperator() != "in") {
columnName = whereCondition.getColumnName();
operator = whereCondition.getOperator();
compareValue = whereCondition.getValue()[0];
WHERE(columnName + " " + operator + " " + compareValue);
} else {
columnName = whereCondition.getColumnName();
operator = whereCondition.getOperator();
// 设置合成后的字符串元素间以逗号分割,合成的字符串以左小括号开头,以右小括号结尾
// 例如["12", "34"]合成后的字符串是(12, 34)
StringJoiner stringJoiner = new StringJoiner(",", "(", ")");
for (int j = 0; j < whereCondition.getValue().length; j++) {
stringJoiner.add(whereCondition.getValue()[j]);
}
compareValue = stringJoiner.toString();
WHERE(columnName + " " + operator + " " + compareValue);
}
}
OFFSET(offset);
LIMIT(limit);
}}.toString();
}
}
}
WhereCondition.java文件
package com.thb.mapper;
/**
* 存放where查询条件的数据库表的列名、比较操作符、待比较的值
*/
public class WhereCondition {
// 数据数库的列名
private String columnName;
// 比较操作符
private String operator;
// 当比较操作符是"in"的时候数组中可能存在多个元素
//当比较操作符不是"in"的时候,数组中只有一个元素
private String[] value;
public String getColumnName() {
return this.columnName;
}
public void setColumnName(String columnName) {
this.columnName = columnName;
}
public String getOperator() {
return this.operator;
}
public void setOperator(String operator) {
this.operator = operator;
}
public String[] getValue() {
return this.value;
}
public void setValue(String[] value) {
this.value = value;
}
}
User.java
package com.thb.model;
public class User {
private int id;
private String userName;
private String homeTown;
private int type;
public int getId() {
return this.id;
}
public void setId(int id) {
this.id = id;
}
public String getUserName() {
return this.userName;
}
public void setUserName(String name) {
this.userName = name;
}
public String getHomeTown () {
return this.homeTown;
}
public void setHomeTown(String hometown) {
this.homeTown = hometown;
}
public int getType() {
return this.type;
}
public void setType(int type) {
this.type = type;
}
}
调用类Demo.java
package com.thb;
import java.io.IOException;
import java.util.ArrayList;
import java.util.List;
import org.apache.ibatis.session.SqlSession;
import org.apache.ibatis.session.SqlSessionFactory;
import com.thb.mapper.UserMapper;
import com.thb.mapper.WhereCondition;
import com.thb.model.User;
public class Demo {
public static void main(String[] args) throws IOException {
Demo demo = new Demo();
demo.dataProcess();
}
public void dataProcess() throws IOException {
SqlSessionFactory sqlSessionFactory = SqlSessionFactoryManager.getSqlSessionFactory();
// 取得一个SqlSession
try (SqlSession session = sqlSessionFactory.openSession()) {
UserMapper userMapper = session.getMapper(UserMapper.class);
String columnName = "type";
String operator = "in";
String[] value = new String[] {"3", "5"};
WhereCondition whereCondition = new WhereCondition();
whereCondition.setColumnName(columnName);
whereCondition.setOperator(operator);
whereCondition.setValue(value);
List<WhereCondition> whereConditions = new ArrayList<>();
whereConditions.add(whereCondition);
int offset = 0;
int limit = 100;
List<User> users = userMapper.selectManyUsers(whereConditions, offset, limit);
for (User user : users) {
System.out.println("*".repeat(60));
System.out.println("id = " + user.getId());
System.out.println("userName = " + user.getUserName());
System.out.println("homeTown = " + user.getHomeTown());
System.out.println("type = " + user.getType());
}
}
}
}
提供SqlSessionFactory单例的SqlSessionFactoryManager
package com.thb;
import java.io.IOException;
import java.io.InputStream;
import org.apache.ibatis.io.Resources;
import org.apache.ibatis.session.SqlSessionFactory;
import org.apache.ibatis.session.SqlSessionFactoryBuilder;
public class SqlSessionFactoryManager {
private static SqlSessionFactory sqlSessionFactory = null;
private static String resource = "mybatis-config.xml";
public static synchronized SqlSessionFactory getSqlSessionFactory() {
if (sqlSessionFactory == null) {
InputStream inputStream;
try {
inputStream = Resources.getResourceAsStream(resource);
sqlSessionFactory = new SqlSessionFactoryBuilder().build(inputStream);
} catch (IOException e) {
e.printStackTrace();
}
}
return sqlSessionFactory;
}
}
运行输出
D:\temp\eclipse-workspace\java_work\mybatis-demo>mvn exec:java -Dexec.mainClass=com.thb.Demo
[INFO] Scanning for projects...
[INFO]
[INFO] ------------------------< com.thb:mybatis-demo >------------------------
[INFO] Building mybatis-demo 0.0.1-SNAPSHOT
[INFO] from pom.xml
[INFO] --------------------------------[ jar ]---------------------------------
[INFO]
[INFO] --- exec-maven-plugin:3.1.0:java (default-cli) @ mybatis-demo ---
18:47:01.265 [com.thb.Demo.main()] DEBUG org.apache.ibatis.logging.LogFactory - Logging initialized using 'class org.apache.ibatis.logging.log4j2.Log4j2Impl' adapter.
18:47:01.275 [com.thb.Demo.main()] DEBUG org.apache.ibatis.datasource.pooled.PooledDataSource - PooledDataSource forcefully closed/removed all connections.
18:47:01.275 [com.thb.Demo.main()] DEBUG org.apache.ibatis.datasource.pooled.PooledDataSource - PooledDataSource forcefully closed/removed all connections.
18:47:01.276 [com.thb.Demo.main()] DEBUG org.apache.ibatis.datasource.pooled.PooledDataSource - PooledDataSource forcefully closed/removed all connections.
18:47:01.277 [com.thb.Demo.main()] DEBUG org.apache.ibatis.datasource.pooled.PooledDataSource - PooledDataSource forcefully closed/removed all connections.
18:47:01.320 [com.thb.Demo.main()] DEBUG org.apache.ibatis.transaction.jdbc.JdbcTransaction - Opening JDBC Connection
18:47:01.667 [com.thb.Demo.main()] DEBUG org.apache.ibatis.datasource.pooled.PooledDataSource - Created connection 1642644333.
18:47:01.668 [com.thb.Demo.main()] DEBUG org.apache.ibatis.transaction.jdbc.JdbcTransaction - Setting autocommit to false on JDBC Connection [com.mysql.cj.jdbc.ConnectionImpl@61e8c36d]
18:47:01.674 [com.thb.Demo.main()] DEBUG com.thb.mapper.UserMapper.selectManyUsers - ==> Preparing: SELECT id, user_name, home_town, type FROM user WHERE (type in (3,5)) LIMIT 100 OFFSET 0
18:47:01.703 [com.thb.Demo.main()] DEBUG com.thb.mapper.UserMapper.selectManyUsers - ==> Parameters:
18:47:01.724 [com.thb.Demo.main()] TRACE com.thb.mapper.UserMapper.selectManyUsers - <== Columns: id, user_name, home_town, type
18:47:01.725 [com.thb.Demo.main()] TRACE com.thb.mapper.UserMapper.selectManyUsers - <== Row: 2, tao, beijing, 3
18:47:01.729 [com.thb.Demo.main()] TRACE com.thb.mapper.UserMapper.selectManyUsers - <== Row: 3, sheng, shanghai, 5
18:47:01.736 [com.thb.Demo.main()] TRACE com.thb.mapper.UserMapper.selectManyUsers - <== Row: 4, com, hangzhou, 3
18:47:01.736 [com.thb.Demo.main()] DEBUG com.thb.mapper.UserMapper.selectManyUsers - <== Total: 3
************************************************************
id = 2
userName = tao
homeTown = beijing
type = 3
************************************************************
id = 3
userName = sheng
homeTown = shanghai
type = 5
************************************************************
id = 4
userName = com
homeTown = hangzhou
type = 3
18:47:01.763 [com.thb.Demo.main()] DEBUG org.apache.ibatis.transaction.jdbc.JdbcTransaction - Resetting autocommit to true on JDBC Connection [com.mysql.cj.jdbc.ConnectionImpl@61e8c36d]
18:47:01.765 [com.thb.Demo.main()] DEBUG org.apache.ibatis.transaction.jdbc.JdbcTransaction - Closing JDBC Connection [com.mysql.cj.jdbc.ConnectionImpl@61e8c36d]
18:47:01.765 [com.thb.Demo.main()] DEBUG org.apache.ibatis.datasource.pooled.PooledDataSource - Returned connection 1642644333 to pool.
[WARNING] thread Thread[#35,mysql-cj-abandoned-connection-cleanup,5,com.thb.Demo] was interrupted but is still alive after waiting at least 15000msecs
[WARNING] thread Thread[#35,mysql-cj-abandoned-connection-cleanup,5,com.thb.Demo] will linger despite being asked to die via interruption
[WARNING] NOTE: 1 thread(s) did not finish despite being asked to via interruption. This is not a problem with exec:java, it is a problem with the running code. Although not serious, it should be remedied.
[INFO] ------------------------------------------------------------------------
[INFO] BUILD SUCCESS
[INFO] ------------------------------------------------------------------------
[INFO] Total time: 16.400 s
[INFO] Finished at: 2024-01-06T18:47:16+08:00
[INFO] ------------------------------------------------------------------------