public class SocketUtil {
private static final ThreadLocal<Socket> threadConnect = new ThreadLocal<Socket>();
private static Socket client;
private static OutputStream outStr = null;
private static InputStream inStr = null;
private static Thread tRecv = new Thread(new RecvThread());
private static Thread tKeep = new Thread(new KeepThread());
// 连接以及重新连接
public static void connect(String host, int port) {
try {
client = threadConnect.get();
if (client == null) {
client = new Socket(host, port);
threadConnect.set(client);
tKeep.start();
System.out.println("========链接开始!========");
} else if (client.isClosed()) {
client = new Socket(host, port);
threadConnect.set(client);
tRecv = new Thread(new RecvThread());
tKeep = new Thread(new KeepThread());
tKeep.start();
System.out.println("========链接开始!========");
}
outStr = client.getOutputStream();
inStr = client.getInputStream();
} catch (Exception e) {
e.printStackTrace();
}
}
// 断开连接
public static void disconnect() {
try {
outStr.close();
inStr.close();
client.close();
} catch (IOException e) {
e.printStackTrace();
}
}
// 接收消息
public static void receiveMsg() {
tRecv.start();
}
// 发送消息
public static void sendMsg(String msg) {
try {
outStr.write(msg.getBytes());
outStr.flush();
} catch (IOException e) {
e.printStackTrace();
}
}
private static class KeepThread implements Runnable {
public void run() {
try {
if (!client.getKeepAlive()) client.setKeepAlive(true);//true,若长时间没有连接则断开
if (!client.getOOBInline()) client.setOOBInline(true);//true,允许发送紧急数据,不做处理
while (true) {
try {
Thread.sleep(5000);
} catch (InterruptedException e) {
// TODO Auto-generated catch block
e.printStackTrace();
}
System.out.println("发送心跳数据包");
outStr.write("心跳信息".getBytes());
outStr.flush();
}
} catch (IOException e) {
e.printStackTrace();
}
}
}
private static class RecvThread implements Runnable {
public void run() {
try {
System.out.println("==============开始接收数据===============");
while (true) {
byte[] b = new byte[1024];
int r = inStr.read(b);
if (r > -1) {
String str = new String(b, 0, r);
System.out.println(str);
}
}
} catch (IOException e) {
e.printStackTrace();
}
}
}
}
JAVA完成Socket心跳连接和保持
最新推荐文章于 2024-03-15 09:07:26 发布
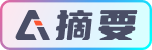