6.AnticipateInterpolator 回荡秋千插值器
这个插值器的值变化过程,可以想像成荡秋千时的一个段过程。(此时秋千已经在比较上面的位置了,一放手就可以荡下来)。你开始用力推向更上面,然后秋千终将荡回下面。
tension值就好比推力的大小。
源代码如下:
01 | package android.view.animation; |
03 | import android.content.Context; |
04 | import android.content.res.TypedArray; |
05 | import android.util.AttributeSet; |
10 | * An interpolator where the change starts backward then flings forward. |
12 | public class AnticipateInterpolator implements Interpolator { |
13 | private final float mTension; |
15 | public AnticipateInterpolator() { |
21 | * 绷紧程度,当绷紧程序为0.0f时,也就没有了反向作用力。插值器将退化成一个y=x^3的加速插值器。 |
23 | * Amount of anticipation. When tension equals 0.0f, there is |
24 | * no anticipation and the interpolator becomes a simple |
25 | * acceleration interpolator. |
27 | public AnticipateInterpolator( float tension) { |
31 | public AnticipateInterpolator(Context context, AttributeSet attrs) { |
32 | TypedArray a = context.obtainStyledAttributes(attrs, |
33 | com.android.internal.R.styleable.AnticipateInterpolator); |
36 | a.getFloat(com.android.internal.R.styleable.AnticipateInterpolator_tension, 2 .0f); |
42 | public float getInterpolation( float t) { |
44 | return t * t * (((mTension + 1 ) * t) - mTension); |
根据getInterpolation()方法。
当tension为默认值2.0f时,曲线图如下:
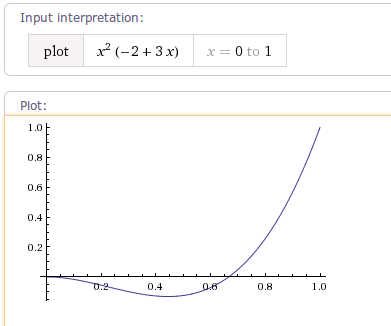
当tension值为4.0f时,曲线图如下:
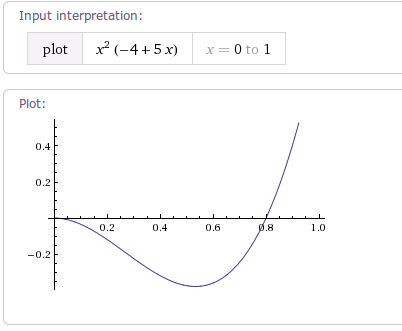
7. AnticipateOvershootInterpolator
源代码如下:
01 | package android.view.animation; |
03 | import android.content.Context; |
04 | import android.content.res.TypedArray; |
05 | import android.util.AttributeSet; |
08 | * 一个插值器它开始向上推,然后向下荡,荡过最低线。然后再回到最低线。 |
10 | * An interpolator where the change starts backward then flings forward and overshoots |
11 | * the target value and finally goes back to the final value. |
13 | public class AnticipateOvershootInterpolator implements Interpolator { |
14 | private final float mTension; |
16 | public AnticipateOvershootInterpolator() { |
17 | mTension = 2 .0f * 1 .5f; |
22 | * anticipation/overshoot的比值。当和tension值为0.0f时, |
23 | * 也就没有anticipation/overshoot的比值了,插值器退化为一个加速/减速插值器。 |
25 | * Amount of anticipation/overshoot. When tension equals 0.0f, |
26 | * there is no anticipation/overshoot and the interpolator becomes |
27 | * a simple acceleration/deceleration interpolator. |
29 | public AnticipateOvershootInterpolator( float tension) { |
30 | mTension = tension * 1 .5f; |
34 | * @param tension Amount of anticipation/overshoot. When tension equals 0.0f, |
35 | * there is no anticipation/overshoot and the interpolator becomes |
36 | * a simple acceleration/deceleration interpolator. |
38 | * 乘以tension的值。例如,在上面构造函数中extraTension的值为1.5f |
40 | * Amount by which to multiply the tension. For instance, |
41 | * to get the same overshoot as an OvershootInterpolator with |
42 | * a tension of 2.0f, you would use an extraTension of 1.5f. |
44 | public AnticipateOvershootInterpolator( float tension, float extraTension) { |
45 | mTension = tension * extraTension; |
48 | public AnticipateOvershootInterpolator(Context context, AttributeSet attrs) { |
49 | TypedArray a = context.obtainStyledAttributes(attrs, AnticipateOvershootInterpolator); |
51 | mTension = a.getFloat(AnticipateOvershootInterpolator_tension, 2 .0f) * |
52 | a.getFloat(AnticipateOvershootInterpolator_extraTension, 1 .5f); |
57 | private static float a( float t, float s) { |
58 | return t * t * (((s + 1 ) * t) - s); |
61 | private static float o( float t, float s) { |
62 | return t * t * (((s + 1 ) * t) + s); |
66 | public float getInterpolation( float t) { |
71 | if (t < 0 .5f) return 0 .5f * a(t * 2 .0f, mTension); |
72 | else return 0 .5f * (o((t * 2 .0f) - 2 .0f, mTension) + 2 .0f); |
根据getInterpolation()方法,
可以得到当tension为默认值时,曲线图为:
plot Piecewise[{{0.5((2x)*(2x)*((2+1)*2x-2)), 0<x<0.5}, {0.5*(((2x-2)*(2x-2)*((2+1)*(2x-2)+2))+2),0.5<=x<=1}}]
(不知道我的plot函数写对了没?)
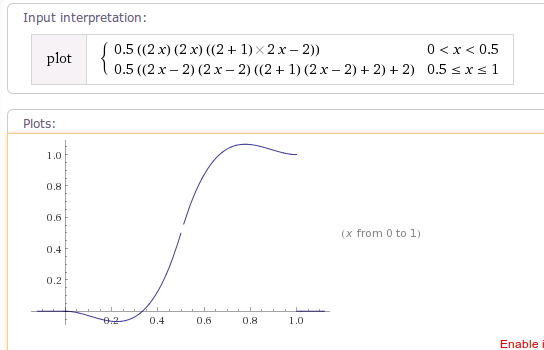
8. CycleInterpolator 正弦周期变化插值器
源代码:
01 | package android.view.animation; |
03 | import android.content.Context; |
04 | import android.content.res.TypedArray; |
05 | import android.util.AttributeSet; |
09 | * 以指定的周期重复动画。变化率曲线为正弦。 |
11 | * Repeats the animation for a specified number of cycles(周期). The |
12 | * rate of change follows a sinusoidal(正弦) pattern. |
15 | public class CycleInterpolator implements Interpolator { |
18 | * @param cycles 要重复的周期数 |
20 | public CycleInterpolator( float cycles) { |
24 | public CycleInterpolator(Context context, AttributeSet attrs) { |
26 | context.obtainStyledAttributes(attrs, com.android.internal.R.styleable.CycleInterpolator); |
28 | mCycles = a.getFloat(com.android.internal.R.styleable.CycleInterpolator_cycles, 1 .0f); |
34 | public float getInterpolation( float input) { |
35 | return ( float )(Math.sin( 2 * mCycles * Math.PI * input)); |
38 | private float mCycles; |
当cycle时为1时,即变化一周时,曲线图如下:
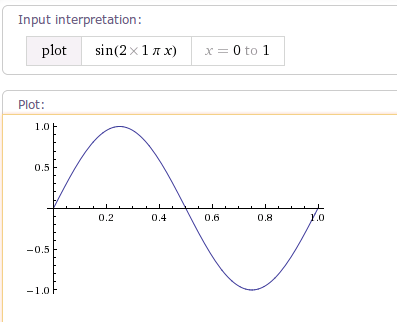
9. OvershootInterpolator
源代码:
01 | package android.view.animation; |
03 | import android.content.Context; |
04 | import android.content.res.TypedArray; |
05 | import android.util.AttributeSet; |
08 | * An interpolator where the change flings forward and overshoots the last value |
11 | public class OvershootInterpolator implements Interpolator { |
12 | private final float mTension; |
14 | public OvershootInterpolator() { |
19 | * @param tension Amount of overshoot. When tension equals 0.0f, there is |
20 | * no overshoot and the interpolator becomes a simple |
21 | * deceleration interpolator. |
23 | public OvershootInterpolator( float tension) { |
27 | public OvershootInterpolator(Context context, AttributeSet attrs) { |
28 | TypedArray a = context.obtainStyledAttributes(attrs, |
29 | com.android.internal.R.styleable.OvershootInterpolator); |
32 | a.getFloat(com.android.internal.R.styleable.OvershootInterpolator_tension, 2 .0f); |
38 | public float getInterpolation( float t) { |
42 | return (t * t * (((mTension + 1 ) * t) + mTension)) + 1 .0f; |
当tension为默认值2时,曲线图如下:
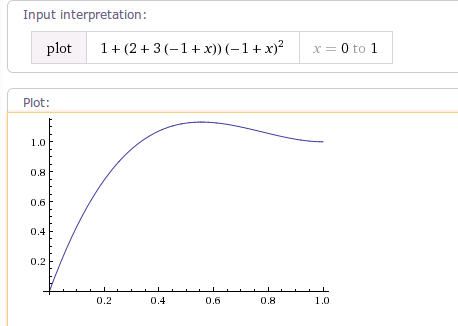
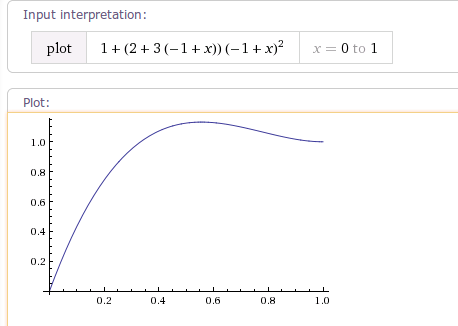
当tension的值为4时,曲线图如下:
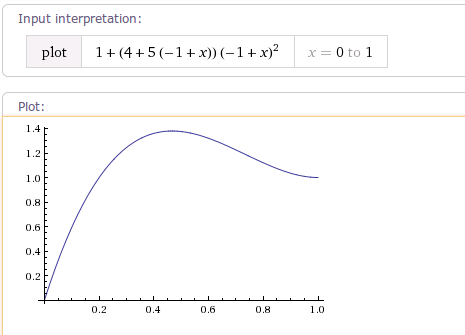
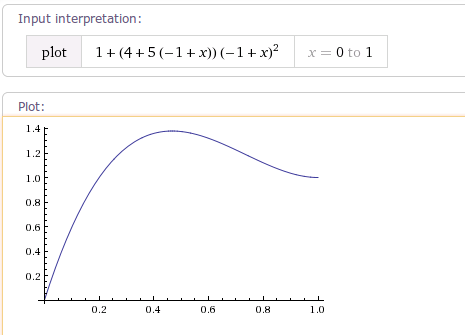
通过学习了解Android自带的这些Interpolator,我们可以很好的根据自己的使用场景使用这些Interpolator了。也可以很容易的写出我们自己的Interpolator。