1.ServletContext的定义
1.1 ServletContext 是一个接口,表示Servlet 上下文对象
1.2 一个web工程,只有一个ServletContext对象实例
1.3 ServletContext对象是一个域对象
什么是域对象?
- 域对象,是可以像 Map一样存取数据的对象
- 这里的域指的的存取数据的操作范围

1.4 ServletContext是在web工程部署启动的时候创建;在web工程停止的时候销毁
2. ServletContext的4个作用
获取 web.xml 中配置的上下文参数 context-param
获取当前的工程路径,格式为: /工程路径
获取工程部署在服务器端硬盘的绝对路径
像 Map一样存取数据
举例如下
Servlet实现类
package com.lchh.servlet;
import java.io.IOException;
import javax.servlet.ServletContext;
import javax.servlet.ServletException;
import javax.servlet.http.HttpServlet;
import javax.servlet.http.HttpServletRequest;
import javax.servlet.http.HttpServletResponse;
@SuppressWarnings("serial")
public class ServletContextTest extends HttpServlet{
@Override
protected void doGet(HttpServletRequest req, HttpServletResponse resp) throws ServletException, IOException {
super.doGet(req, resp);
ServletContext servletContext = getServletConfig().getServletContext();
String imageUrl = servletContext.getInitParameter("imageUrl");
System.out.println("imageUrl="+imageUrl);
String web_desc = servletContext.getInitParameter("web_desc");
System.out.println("web_desc="+web_desc);
String contextPath = servletContext.getContextPath();
System.out.println("contextPath="+contextPath);
String realPath = servletContext.getRealPath("/");
System.out.println("realPath="+realPath);
}
@Override
protected void doPost(HttpServletRequest req, HttpServletResponse resp) throws ServletException, IOException {
super.doPost(req, resp);
}
}
web.xml
<?xml version="1.0" encoding="UTF-8"?>
<web-app xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xmlns="http://xmlns.jcp.org/xml/ns/javaee" xsi:schemaLocation="http://xmlns.jcp.org/xml/ns/javaee http://xmlns.jcp.org/xml/ns/javaee/web-app_3_1.xsd" id="WebApp_ID" version="3.1">
<display-name>servlet_01</display-name>
<welcome-file-list>
<welcome-file>index.html</welcome-file>
<welcome-file>index.htm</welcome-file>
<welcome-file>index.jsp</welcome-file>
<welcome-file>default.html</welcome-file>
<welcome-file>default.htm</welcome-file>
<welcome-file>default.jsp</welcome-file>
</welcome-file-list>
<context-param>
<param-name>imageUrl</param-name>
<param-value>www.baidu.com/image</param-value>
</context-param>
<context-param>
<param-name>web_desc</param-name>
<param-value>这个网站是一个测试网站</param-value>
</context-param>
<servlet>
<servlet-name>ServletContextTest</servlet-name>
<servlet-class>com.lchh.servlet.ServletContextTest</servlet-class>
</servlet>
<servlet-mapping>
<servlet-name>ServletContextTest</servlet-name>
<url-pattern>/servletContext</url-pattern>
</servlet-mapping>
</web-app>
测试结果
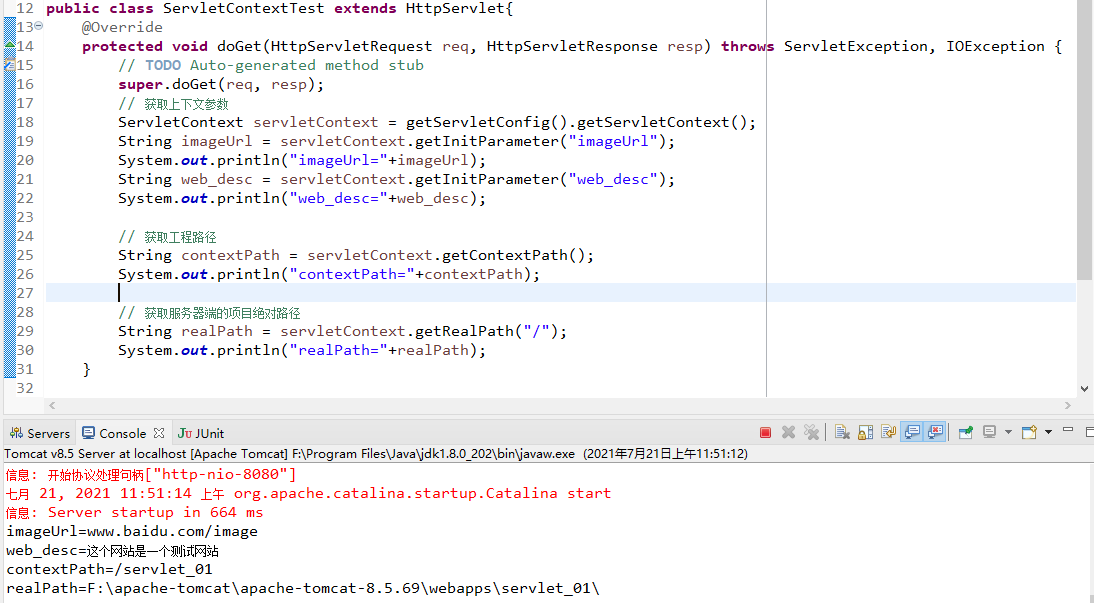