用js写一个轮播效果
-
1思路如下:1.自动轮播(重点偏移量)
1-1.如果需要左右轮播的话则将图片排成一行。
1-2.如果需要上下轮播的话则将图片排成一列. -
2.左右两侧耳朵点击进行位移
3.通过点击下方小圆点进行任意位置轮播
1.先静态后动态的开发模式。
2.动态JS的开发思路(原生的JS)
3.首先HTML和js关联起来。
4.核心重点是:偏移量(取自于图片的宽度或者规定每次移动的像素值)
5.HTML和js结合式的书写 变量名.style.left = 数值。 (取默认的左偏移初始值)
要用到的知识有:
- 1.周期性定时器
2.数组(存图片)
3. if…else…(判断首尾变化)
4.清空定时器
5.单击事件
6.js选择器(id,集合)
7.偏移量(非常重要)
代码如下
1.周期性定时器
2.数组(存图片)
3. if...else...(判断首尾变化)
4.清空定时器
5.单击事件
6.js选择器(id,集合)
7.偏移量(非常重要)
css部分:
<style>
#container {width: 600px;height: 300px;
border:2px solid black;
position: relative;overflow: hidden;}
#list img{width: 600px;height:300px;float: left;}
#list{width: 3600px;height:300px;position:absolute;
/* z-index:1px ;solid red */}
/* 耳朵 */
.arrow{position: absolute;top: 110px;text-decoration: none;
z-index:2;
/* 调节层级与层级之间的关系,必须放在定位后使用} */
display: none; /* 隐藏 */
width: 40px;height: 40px;font-size: 36px;font-weight: bold;
line-height: 39px;text-align: center;color: #FFFFFF; background-color: rgba(0,0,0,1);cursor: pointer;}
.arrow:hover{background-color: rgba(0,0,0,0.8);}
#container:hover .arrow{display: block;}
#prev{right: 20px;}
#next{left: 20px;}
#list{transition: left 1s;};
.list{transition: left 0.1s;};
/* 第三种点击轮播 */
元素 {
}
/* #buttons {
position: absolute;
left: 250px;
bottom: 20px;
z-index: 2;
height: 10px;
width: 120px;
} */
#buttons{position: absolute;
left: 250px;
bottom: 20px;
z-index:2;
height: 10px;
width: 120px}
#buttons span{
float:left;
margin-right: 5px;
width: 10px;
height: 10px;
border: 1px solid #FFFFFF;
border-radius: 5px;
background: #333;
cursor: pointer;}
#buttons .on{background: orangered;}
</style>
`
HTML部分:
-- 1,首先规定盒子引入6张图片 -->
<div id="container">
<!-- 将6张图片包裹在一个很宽的div中 -->
<div id="list" style="left:0px;">
<img src="image/index1.jpg" alt="1" />
<img src="image/index2.jpg" alt="2" />
<img src="image/index3.jpg" alt="3" />
<img src="image/index4.jpg" alt="4" />
<img src="image/index5.jpg" alt="5" />
<img src="image/index6.jpg" alt="6" />
</div>
<!-- 2,写两侧的耳朵链接 -->
<a href="javascript:;" id="next" class="arrow"><</a>
<a href="javascript:;" id="prev" class="arrow">></a>
<!--3, 六个小圆点 -->
<div id="buttons">
<span index='1' class="on"></span>
<span index='2'></span>
<span index='3'></span>
<span index='4'></span>
<span index='5'></span>
<span index='6'></span>
</div>
</div>`
js部分:
<script type="text/javascript">
// 11,页面加载完成时的时候执行里面的定时器
// 首先进行关联(一般id的都会关联起来)
window.onload = function() {
var container = document.getElementById("container");
var list = document.getElementById("list")
var prev = document.getElementById("prev")
var next = document.getElementById("next")
var newlist = parseInt(list.style.left);
console.log(newlist);
var buttons = document.getElementById("buttons").getElementsByTagName('span')
console.log(buttons)
var timer
var index = 1; //移动的速度为1毫秒
// 22,开始操作偏移量的方法
function animals(offset) {
var newlist = parseInt(list.style.left) + offset;
list.style.left = newlist + 'px';
console.log(list.style.left)
// 4如何解决偏过头的问题?
// 判断偏移量大于最后一张的时候时,返回到第一张
if (newlist < -3001) {
list.style.left = 0 + 'px'
list.setAttribute('class', 'list');
}
if (newlist > 0) {
list.style.left = -3000 + 'px';
list.setAttribute('class', 'list');
}
}
// 5,点击左右两侧耳朵进行移动
prev.onclick = function() {
animals(600)
}
next.onclick = function() {
animals(-600)
}
// 5,把定时器包裹在自定义方法中,,用于后边的的使用
function play() {
timer = setInterval(function() {
prev.onclick()
}, 2000);
}
play();
// 6,清空定时器
function stop() {
clearInterval(timer);
}
// 7,鼠标划入到框中进行清空定时器,方便点击切换的过程
// 鼠标划出时触发定时器,方便自动了轮播
container.onmouseenter = stop;
container.onmouseleave = play;
// 第三种任意点击轮播
// 1.需要先获取点击某一个小圆圈后的下标值
// 2判断到达两头是反向移动位置
// 3.将点击的值和图片进行关联(自调函数【闭包】)
// 这是用来清空当前的div的classname
function buttonsShow() {
for (var i = 0; i < buttons.length; i++) {
if (buttons[i].className == 'on') {
buttons[i].className = ''
}
console.log(buttons[i]);
}
buttons[index - 1].className = 'on'
}
// 这是用来判断光标到两头是的过程
prev.onclick = function() {
index += 1;
console.log(index)
if (index > 6) {
index = 1
}
buttonsShow()
animals(600)
console.log(index)
}
next.onclick = function() {
index -= 1;
if (index < 1) {
index = 6
}
buttonsShow()
animals(-600)
}
// 通过圆点的长度循环,使用自调函数进行操作
for (var i = 0; i < buttons.length; i++) {
(function(i) {
// 获取被点击的圆圈中的index的指向
buttons[i].onclick = function() {
var newindex = this.getAttribute('index')
console.log(newindex);
// 通过该值进行调用animals(offset)方法 计算偏移量
offset = 600 * (index - newindex);
console.log(offset);
animals(offset);
// 去掉默认的带色小圆点,将点击的小圆点上色
index = newindex
console.log(index);
buttonsShow();
}
})
}
}
</script>
最后6张图片: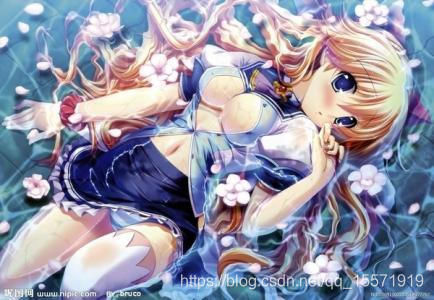
最后的总体效果视频:
27.0.0.1:8848/轮播/轮播/轮播练习.html
后期再上传