几个常用的处理流
ByteArrayInputStream和ByteArrayOutputStream
字节数组输入流在内存中创建一个字节数组缓冲区,从输入流读取的数据保存在该字节数组缓冲区中。
字节数组输出流在内存中创建一个字节数组缓冲区,所有发送到输出流的数据保存在该字节数组缓冲区中。
package com.buerc.file;
import java.io.ByteArrayInputStream;
import java.io.ByteArrayOutputStream;
import java.io.IOException;
import org.junit.Test;
public class ByteArrayInputOutPutStream {
@Test
public void input() {//这样read进去后,中文有可能发生乱码
ByteArrayInputStream bais=new ByteArrayInputStream("ByteArrayInputStream总结".getBytes());
byte[] b=new byte[1024];
int len;
try {
while((len=bais.read(b))!=-1) {
System.out.print(new String(b,0,len));
}
} catch (IOException e) {
e.printStackTrace();
}
}
@Test
public void test() throws IOException {
ByteArrayOutputStream baos=new ByteArrayOutputStream(12);
while(baos.size()!=10) {
baos.write(System.in.read());
}
byte[] b=baos.toByteArray();
for(int i=0;i<b.length;i++) {
System.out.println((char)b[i]);
}
}
}
缓冲流BufferedInputStream;BufferedOutputStream;BufferedReader;BufferedWriter;
package com.buerc.file;
import java.io.BufferedInputStream;
import java.io.BufferedOutputStream;
import java.io.BufferedReader;
import java.io.BufferedWriter;
import java.io.FileInputStream;
import java.io.FileNotFoundException;
import java.io.FileOutputStream;
import java.io.FileReader;
import java.io.FileWriter;
import java.io.IOException;
import org.junit.Test;
public class BufferedTest {
@Test
public void input_output() {
BufferedInputStream bis=null;
BufferedOutputStream bos=null;
try {
long start=System.currentTimeMillis();
bis = new BufferedInputStream(new FileInputStream("C:\\Users\\buerc\\Desktop\\1.rar"));
bos=new BufferedOutputStream(new FileOutputStream("C:\\Users\\buerc\\Desktop\\2.rar"));
byte[] b=new byte[1024];
int len;
try {
while((len=bis.read(b))!=-1) {
bos.write(b,0,len);
// bos.flush();
}
} catch (IOException e) {
e.printStackTrace();
}
long end =System.currentTimeMillis();
System.out.println(end-start);
} catch (FileNotFoundException e) {
e.printStackTrace();
}finally {
try {
bos.close();
} catch (IOException e1) {
e1.printStackTrace();
}
try {
bis.close();
} catch (IOException e) {
e.printStackTrace();
}
}
}
@Test
public void reader_writer() {
BufferedReader br=null;
BufferedWriter bw=null;
try {
br = new BufferedReader(new FileReader("hello.txt"));//纯文本文件适合Reader和Writer
bw =new BufferedWriter(new FileWriter("hello1.txt"));
String str;
while((str=br.readLine())!=null) {
bw.write(str+"\n");
}
} catch (IOException e) {
e.printStackTrace();
}finally {
try {
bw.close();
} catch (IOException e1) {
e1.printStackTrace();
}
try {
br.close();
} catch (IOException e) {
e.printStackTrace();
}
}
}
}
转换流InputStreamReader、OutputStreamWriter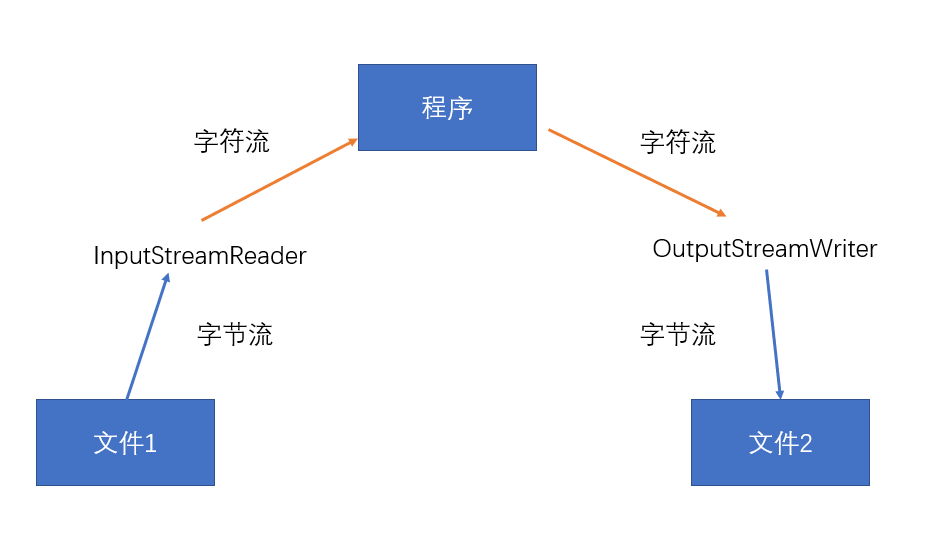
转换流将字节流转换为字符流,将字符流转为字节流,如上图所示。
package com.buerc.file;
import java.io.FileInputStream;
import java.io.FileNotFoundException;
import java.io.FileOutputStream;
import java.io.IOException;
import java.io.InputStreamReader;
import java.io.OutputStreamWriter;
import org.junit.Test;
public class InputOuptPutStreamReaderWriter {
@Test
public void test() {
InputStreamReader isr=null;
OutputStreamWriter osw=null;
try {
isr = new InputStreamReader(new FileInputStream("hello.txt"));
osw=new OutputStreamWriter(new FileOutputStream("hello2.txt"));
char[] c=new char[1024];
int len;
try {
while((len=isr.read(c))!=-1) {
osw.write(String.valueOf(c, 0, len));
}
} catch (IOException e) {
e.printStackTrace();
}
} catch (FileNotFoundException e) {
e.printStackTrace();
}finally {
try {
osw.close();
} catch (IOException e1) {
e1.printStackTrace();
}
try {
isr.close();
} catch (IOException e) {
e.printStackTrace();
}
}
}
}
InputStreamReader:输入时,实现字节流到字符流的转换,提高操作的效率(前提是,数据是文本文件)
===>解码:字节数组--->字符串
OutputStreamWriter:输出时,实现字符流到字节流的转换。 ===>编码: 字符串---->字节数组
对象流ObjectInputStream;ObjectOutputStream
对象的序列化机制:允许把内存中的Java对象转换成平台无关的二进制流,从而允许把这种二进制流持久地保存在磁盘上,或通过网络将这种二进制流传输到另一个网络节点。当其它程序获取了这种二进制流,就可以恢复成原来的Java对象.
实现序列化机制的对象对应的类的要求:
①要求类要实现Serializable接口
②同样要求类的所有属性也必须实现Serializable接口
③要求给类提供一个序列版本号:private static final long serialVersionUID;
④属性声明为static 或transient的,不可以实现序列化
package com.buerc.file;
import java.io.FileInputStream;
import java.io.FileNotFoundException;
import java.io.FileOutputStream;
import java.io.IOException;
import java.io.ObjectInputStream;
import java.io.ObjectOutputStream;
import org.junit.Test;
public class ObjectInputOutputStream {
@Test
public void test1() {
ObjectOutputStream oos=null;
try {
oos = new ObjectOutputStream(new FileOutputStream("person.txt"));
oos.writeObject(new Person("张三", 20));//将person对象序列化保存到文件中
} catch (FileNotFoundException e) {
e.printStackTrace();
} catch (IOException e) {
e.printStackTrace();
}finally {
try {
oos.close();
} catch (IOException e) {
e.printStackTrace();
}
}
}
@Test
public void test2() {
ObjectInputStream ois=null;
try {
ois = new ObjectInputStream(new FileInputStream("person.txt"));
try {
//从文件读取信息进行反序列化进而实例化,如果Person类有个Address属性,
//则Adderss类对应的属性也应该满足序列化条件,否则Person类无法序列化
Person person=(Person)ois.readObject();
System.out.println(person.toString());
} catch (ClassNotFoundException e) {
e.printStackTrace();
}
} catch (FileNotFoundException e) {
e.printStackTrace();
} catch (IOException e) {
e.printStackTrace();
}finally {
try {
ois.close();
} catch (IOException e) {
e.printStackTrace();
}
}
}
}
打印流 (都是输出流) PrintStream(处理字节) PrintWriter(处理字符)
package com.buerc.file;
import java.io.File;
import java.io.FileNotFoundException;
import java.io.FileWriter;
import java.io.IOException;
import java.io.PrintStream;
import java.io.PrintWriter;
import org.junit.Test;
public class PrintTest {
@Test
public void test1() {
PrintStream printStream=null;
try {
printStream = new PrintStream(new File("print.txt"));
System.setOut(printStream);//改变输出方式
System.out.println("不会输出到控制台了,而是文件中");
System.out.println((char)new byte[] {97,98,99}[1]);
} catch (FileNotFoundException e) {
e.printStackTrace();
}finally {
printStream.close();
}
}
@Test
public void test2() {
PrintWriter printWriter=null;
try {
printWriter = new PrintWriter(new FileWriter("writer.txt"));
printWriter.print("我是中国人");
} catch (IOException e) {
e.printStackTrace();
}finally {
printWriter.close();
}
}
}
DataInputStream数据输入流允许应用程序以与机器无关方式从底层输入流中读取基本 Java 数据类型。
DataOutputStream数据输出流允许应用程序以与机器无关方式将Java基本数据类型写到底层输出流。
package com.buerc.file;
import java.io.BufferedReader;
import java.io.DataInputStream;
import java.io.DataOutputStream;
import java.io.FileInputStream;
import java.io.FileNotFoundException;
import java.io.FileOutputStream;
import java.io.IOException;
import java.io.InputStreamReader;
import org.junit.Test;
public class DataTest {
@Test
public void test1() {
DataInputStream dis = null;
try {
dis = new DataInputStream(new FileInputStream("test.txt"));
byte[] b = new byte[1024];
int len;
try {
while ((len = dis.read(b)) != -1) {
System.out.println(new String(b, 0, len));
}
} catch (IOException e) {
e.printStackTrace();
}
} catch (FileNotFoundException e) {
e.printStackTrace();
} finally {
try {
dis.close();
} catch (IOException e) {
e.printStackTrace();
}
}
}
@Test
public void test2() {
DataOutputStream out = null;
BufferedReader br = null;
try {
DataInputStream in = new DataInputStream(new FileInputStream("test.txt"));
out = new DataOutputStream(new FileOutputStream("test1.txt"));
br = new BufferedReader(new InputStreamReader(in));
String count;
while ((count = br.readLine()) != null) {
String u = count.toUpperCase();
System.out.println(u);
out.writeBytes(u + " ,");
}
} catch (FileNotFoundException e) {
e.printStackTrace();
} catch (IOException e) {
e.printStackTrace();
} finally {
try {
br.close();
} catch (IOException e) {
e.printStackTrace();
}
try {
out.close();
} catch (IOException e) {
e.printStackTrace();
}
}
}
}
随机存取文件流:RandomAccessFile
既可以充当一个输入流,又可以充当一个输出流:public RandomAccessFile(File file, String mode)支持从文件的开头读取、写入。若输出的文件不存在,直接创建。若存在,则是对原有文件内容的覆盖。
支持任意位置的“插入”。