文件描述
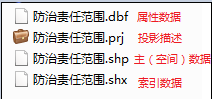
maven必备jar包 pom.xml
<dependency>
<groupId>com.vividsolutions</groupId>
<artifactId>jts-core</artifactId>
<version>1.14.0</version>
</dependency>
<dependency>
<groupId>org.geotools</groupId>
<artifactId>gt-metadata</artifactId>
<version>${geotools.version}</version>
</dependency>
<dependency>
<groupId>org.geotools</groupId>
<artifactId>gt-main</artifactId>
<version>${geotools.version}</version>
</dependency>
<dependency>
<groupId>org.geotools</groupId>
<artifactId>gt-shapefile</artifactId>
<version>${geotools.version}</version>
</dependency>
<dependency>
<groupId>org.geotools</groupId>
<artifactId>gt-api</artifactId>
<version>${geotools.version}</version>
</dependency>
<dependency>
<groupId>org.geotools</groupId>
<artifactId>gt-geojson</artifactId>
<version>${geotools.version}</version>
</dependency>
<dependency>
<groupId>org.geotools</groupId>
<artifactId>gt-geometry</artifactId>
<version>${geotools.version}</version>
</dependency>
<dependency>
<groupId>org.geotools</groupId>
<artifactId>gt-opengis</artifactId>
<version>${geotools.version}</version>
</dependency>
<dependency>
<groupId>org.geotools</groupId>
<artifactId>gt-data</artifactId>
<version>${geotools.version}</version>
</dependency>
<dependency>
<groupId>org.geotools</groupId>
<artifactId>gt-referencing</artifactId>
<version>${geotools.version}</version>
</dependency>
<!-- https://mvnrepository.com/artifact/com.googlecode.json-simple/json-simple -->
<dependency>
<groupId>com.googlecode.json-simple</groupId>
<artifactId>json-simple</artifactId>
</dependency>
<!-- https://mvnrepository.com/artifact/org.jscience/jscience -->
<dependency>
<groupId>org.jscience</groupId>
<artifactId>jscience</artifactId>
<version>4.3.1</version>
</dependency>
<!-- https://mvnrepository.com/artifact/com.googlecode.efficient-java-matrix-library/ejml -->
<dependency>
<groupId>com.googlecode.efficient-java-matrix-library</groupId>
<artifactId>ejml</artifactId>
<version>0.25</version>
</dependency>
解析.shp.dbf文件
package com.kero99.utils;
import java.io.File;
import java.io.StringWriter;
import java.nio.charset.Charset;
import java.util.ArrayList;
import java.util.HashMap;
import java.util.LinkedHashMap;
import java.util.List;
import java.util.Map;
import org.geotools.data.shapefile.ShapefileDataStore;
import org.geotools.data.shapefile.ShapefileDataStoreFactory;
import org.geotools.data.shapefile.dbf.DbaseFileHeader;
import org.geotools.data.shapefile.dbf.DbaseFileReader;
import org.geotools.data.shapefile.files.ShpFiles;
import org.geotools.data.simple.SimpleFeatureCollection;
import org.geotools.data.simple.SimpleFeatureIterator;
import org.geotools.data.simple.SimpleFeatureSource;
import org.geotools.geojson.feature.FeatureJSON;
import org.geotools.geojson.geom.GeometryJSON;
import org.opengis.feature.simple.SimpleFeature;
import org.opengis.feature.type.AttributeDescriptor;
import net.sf.json.JSONArray;
import net.sf.json.JSONObject;
public class ShapeUtils {
/**
* 解析.shp 点线面文件
* @param path
* @return
* @throws Exception
* @author ygc
*/
public static Map<String, String> ParasShapeFileData(String path) throws Exception {
Map<String, String> map = new HashMap<String, String>();
try {
File file = new File(path);
String fileName = file.getName();
ShapefileDataStoreFactory sfdsf = new ShapefileDataStoreFactory();
ShapefileDataStore sfds = (ShapefileDataStore) sfdsf.createDataStore(file.toURI().toURL());
sfds.setCharset(Charset.forName("GBK"));
SimpleFeatureSource featureSource = sfds.getFeatureSource();
List<AttributeDescriptor> attrList = sfds.getSchema().getAttributeDescriptors();
JSONObject pFieldJSON = new JSONObject();
for (AttributeDescriptor attributeDescriptor : attrList) {
String pFieldName = attributeDescriptor.getName().toString();
String pTypeName = attributeDescriptor.getType().getBinding().getSimpleName();
// System.out.println(attributeDescriptor.getType().getBinding().getName());
if (pFieldName == "the_geom") {
map.put("type", pTypeName);
pFieldName = "Shape";
pTypeName = "Geometry";
}
pFieldJSON.put(pFieldName, getShapeType(pTypeName));
}
// System.out.println(pFieldJSON);
SimpleFeatureCollection feacollection = featureSource.getFeatures();
SimpleFeatureIterator iterator = feacollection.features();
GeometryJSON gjson = new GeometryJSON(15);
FeatureJSON fjson = new FeatureJSON(gjson);
StringWriter writer = new StringWriter();
while (iterator.hasNext()) {
SimpleFeature sFeature = iterator.next();
if (writer.toString().isEmpty()) {
fjson.writeFeature(sFeature, writer);
} else {
writer.write(',');
fjson.writeFeature(sFeature, writer);
}
}
iterator.close();
writer.flush();
writer.close();
sfds.dispose();
String jsonData = "[" + writer.toString() + "]";
// System.err.println(jsonData);
JSONObject jsonObject = new JSONObject();
jsonObject.put("type", "FeatureCollection");
jsonObject.put("features", JSONArray.fromObject(jsonData));
map.put("features", jsonObject.toString());
map.put("fields", pFieldJSON.toString());
map.put("LayerName", file.getName().replace(".shp", ""));
map.put("op", "sucess");
// System.out.println(map);
} catch (Exception e) {
// TODO: handle exception
e.printStackTrace();
map.put("op", "fail");
}
return map;
}
/**
* 解析.dbf
* @param path
* @return
* @throws Exception
* @author ygc
*/
public static List<Object> readDbfFile(String path){
List<Object> list=new ArrayList<Object>();
Map<Object, Object> values = null;
DbaseFileReader reader = null;
try {
reader = new DbaseFileReader(new ShpFiles(path), false, Charset.forName("GBK"));
DbaseFileHeader header = reader.getHeader();
int numFields = header.getNumFields();
//迭代读取记录
while (reader.hasNext()) {
try {
Object[] entry = reader.readEntry();
values=new LinkedHashMap<Object, Object>();
for (int i=0; i<numFields; i++) {
String title = header.getFieldName(i);
Object value = entry[i];
values.put(title, value);
System.out.println(title+"="+value);
}
} catch (Exception e) {
e.printStackTrace();
}
list.add(values);
}
} catch (Exception e) {
e.printStackTrace();
} finally {
if (reader != null) {
//关闭
try {reader.close();} catch (Exception e) {}
}
}
return list;
}
public static String getShapeType(String type) {
String pDbFieldType = "";
switch (type) {
case "Integer":
pDbFieldType = "INTEGER";
break;
case "Double":
pDbFieldType = "DOUBLE";
break;
case "String":
pDbFieldType = "VARCHAR";
break;
case "Date":
pDbFieldType = "DATETIME";
break;
case "Boolean":
pDbFieldType = "BOOLEAN";
break;
case "Geometry":
pDbFieldType = "Geometry";
break;
default:
pDbFieldType = "TEXT";
break;
}
return pDbFieldType;
}
//投影转换 prj
public static void main(String[] args) throws Exception {
String path="E:\\shape文件\\设计图斑\\设计图斑-线状\\设计图斑-线状.dbf";
ShapeUtils.readDbfFile(path);
System.out.println(ShapeUtils.ParasShapeFileData(path));
}
}