//miner用来对worker进行管理, 订阅外部事件,控制worker的启动和停止。
// Backend wraps all methods required for mining.
type Backend interface {
AccountManager() *accounts.Manager//账户管理
BlockChain() *core.BlockChain
TxPool() *core.TxPool
ChainDb() ethdb.Database
}
// Miner creates blocks and searches for proof-of-work values.矿工创建块和搜索工作量证明值。
type Miner struct {
mux *event.TypeMux
worker *worker
coinbase common.Address
mining int32
eth Backend
engine consensus.Engine
canStart int32 // can start indicates whether we can start the mining operation可以启动指示是否可以启动采矿操作。
shouldStart int32 // should start indicates whether we should start after sync应该开始指示我们是否应该在同步之后开始
}
//构造, 创建了一个CPU agent 启动了miner的update goroutine
func New(eth Backend, config *params.ChainConfig, mux *event.TypeMux, engine consensus.Engine) *Miner {
miner := &Miner{
eth: eth,
mux: mux,
engine: engine,
worker: newWorker(config, engine, common.Address{}, eth, mux),
canStart: 1,
}
miner.Register(NewCpuAgent(eth.BlockChain(), engine))
go miner.update()
return miner
}
//update订阅了downloader的事件, 注意这个goroutine是一个一次性的循环,
// 只要接收到一次downloader的downloader.DoneEvent或者 downloader.FailedEvent事件,
// 就会设置canStart为1. 并退出循环, 这是为了避免黑客恶意的 DOS攻击,让你不断的处于异常状态
// update keeps track of the downloader events. Please be aware that this is a one shot type of update loop.
// It's entered once and as soon as `Done` or `Failed` has been broadcasted the events are unregistered and
// the loop is exited. This to prevent a major security vuln where external parties can DOS you with blocks
// and halt your mining operation for as long as the DOS continues.
func (self *Miner) update() {
events := self.mux.Subscribe(downloader.StartEvent{}, downloader.DoneEvent{}, downloader.FailedEvent{})
out:
for ev := range events.Chan() {
switch ev.Data.(type) {
case downloader.StartEvent:
atomic.StoreInt32(&self.canStart, 0)
if self.Mining() {
self.Stop()
atomic.StoreInt32(&self.shouldStart, 1)
log.Info("Mining aborted due to sync")
}
case downloader.DoneEvent, downloader.FailedEvent:
shouldStart := atomic.LoadInt32(&self.shouldStart) == 1
atomic.StoreInt32(&self.canStart, 1)
atomic.StoreInt32(&self.shouldStart, 0)
if shouldStart {
self.Start(self.coinbase)
}
// unsubscribe. we're only interested in this event once
//退订。 我们只对这个活动感兴趣
events.Unsubscribe()
// stop immediately and ignore all further pending events
//立即停止并忽略所有进一步的未决事件
break out
}
}
}
//开始挖矿
func (self *Miner) Start(coinbase common.Address) {
atomic.StoreInt32(&self.shouldStart, 1)//shouldStart 是是否应该启动
self.SetEtherbase(coinbase)
if atomic.LoadInt32(&self.canStart) == 0 {//canStart是否能够启动,
log.Info("Network syncing, will start miner afterwards")
return
}
atomic.StoreInt32(&self.mining, 1)
log.Info("Starting mining operation")
self.worker.start()// 启动worker 开始挖矿
self.worker.commitNewWork()//提交新的挖矿任务。
}
//停止挖矿
func (self *Miner) Stop() {
self.worker.stop()
atomic.StoreInt32(&self.mining, 0)
atomic.StoreInt32(&self.shouldStart, 0)
}
//注册agent
func (self *Miner) Register(agent Agent) {
if self.Mining() {
agent.Start()
}
self.worker.register(agent)
}
func (self *Miner) Unregister(agent Agent) {
self.worker.unregister(agent)
}
//是否在挖矿
func (self *Miner) Mining() bool {
return atomic.LoadInt32(&self.mining) > 0
}
func (self *Miner) HashRate() (tot int64) {
if pow, ok := self.engine.(consensus.PoW); ok {
tot += int64(pow.Hashrate())
}
// do we care this might race? is it worth we're rewriting some
// aspects of the worker/locking up agents so we can get an accurate
// hashrate?
for agent := range self.worker.agents {
if _, ok := agent.(*CpuAgent); !ok {
tot += agent.GetHashRate()
}
}
return
}
//设置额外数据
func (self *Miner) SetExtra(extra []byte) error {
if uint64(len(extra)) > params.MaximumExtraDataSize {
return fmt.Errorf("Extra exceeds max length. %d > %v", len(extra), params.MaximumExtraDataSize)
}
self.worker.setExtra(extra)
return nil
}
//挂起返回当前挂起的块和关联状态。
// Pending returns the currently pending block and associated state.
func (self *Miner) Pending() (*types.Block, *state.StateDB) {
return self.worker.pending()
}
// PendingBlock returns the currently pending block.
//
// Note, to access both the pending block and the pending state
// simultaneously, please use Pending(), as the pending state can
// change between multiple method calls
// PendingBlock返回当前挂起的块。
//
//注意,要访问待处理块和待处理状态
//同时,请使用Pending()作为挂起状态
//在多个方法调用之间切换
func (self *Miner) PendingBlock() *types.Block {
return self.worker.pendingBlock()
}
//设置挖矿者地址
func (self *Miner) SetEtherbase(addr common.Address) {
self.coinbase = addr
self.worker.setEtherbase(addr)
}
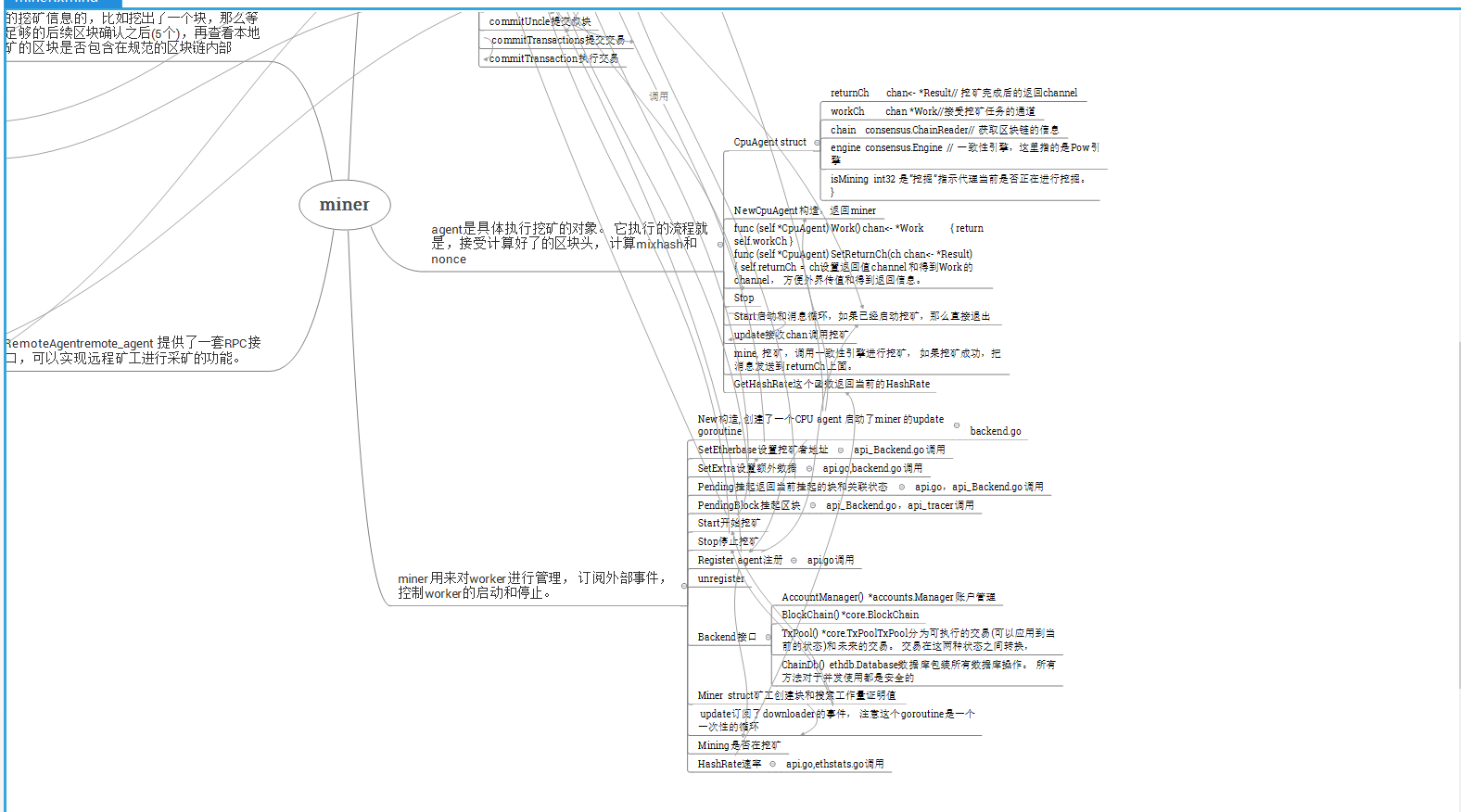
赞助99元可以获得miner包完整Xmind,赞助199元可以获得miner包完整翻译源码,欢迎留言。按留言决定分析模块顺序。