#include<iostream>
#include<cstdio>
#include<queue>
#include<cstring>
#include<algorithm>
/* 构造完成标志 */
int sign; //0代表false,1代表true
/* 创建数独矩阵 */
int num[9][9];
/* 函数声明 */
void Input();
void Output();
int Check(int n, int key);//返回0代表false,1代表true
int DFS(int n);
/* 主函数 */
int main()
{
int test,i,j;
scanf("%d",&test);
while(test--)
{
sign=0;//设初始值为0
Input();//输入
DFS(0);
Output();//输出
}
}
/* 读入数独矩阵 */
void Input()
{
int i,j;
for (i = 0; i < 9; i++)
for (j = 0; j < 9; j++)
scanf("%1d",&num[i][j]);//一个数一个数的读
}
/* 输出数独矩阵 */
void Output()
{
int i,j;
for (i = 0; i < 9; i++)
{
for (j = 0; j < 9; j++)
{
printf("%d",num[i][j]);
}
printf("\n");
}
}
/* 判断key填入n时是否满足条件 */
int Check(int n, int key)
{
/* 判断n所在横列是否合法 */
int i,j;
int x,y;
for (j = 0; j < 9; j++)
{
/* i为n竖坐标 */
i = n / 9;
//如果有相同的数,返回0(不合法)
if (num[i][j] == key) return 0;
}
/* 判断n所在竖列是否合法 */
for (i = 0; i < 9; i++)
{
/* j为n横坐标 */
j = n % 9;
//如果有相同的数,返回0(不合法)
if (num[i][j] == key) return 0;
}
/* x为n所在的小九宫格左顶点竖坐标 */
x = n / 9 / 3 * 3;//如:38/9/3*3=3
/* y为n所在的小九宫格左顶点横坐标 */
y = n % 9 / 3 * 3;//如:38%9/3*3=6
/* 判断n所在的小九宫格是否合法 */
for (i = x; i < x + 3; i++)
{
for (j = y; j < y + 3; j++)
{
if (num[i][j] == key) return 0;
}
}
/* 全部合法,返回正确 */
return 1;
}
/* 深搜构造数独 */
int DFS(int n)
{
/* 所有的都符合,退出递归 */
int i;
if (n > 80)
{
sign = 1;
return 0;
}
/* 当前位不为空时跳过 */
if (num[n/9][n%9] != 0)
{
DFS(n+1);
}
else
{
/* 否则对当前位进行枚举测试 */
for (i = 1; i <= 9; i++)
{
/* 满足条件时填入数字 */
if (Check(n, i) == 1)
{
num[n/9][n%9] = i;
/* 继续搜索 */
DFS(n+1);
/* 返回时如果构造成功,则直接退出 */
if (sign == 1) return 0;
/* 如果构造不成功,还原当前位 */
num[n/9][n%9] = 0;
}
}
}
}
Description
Sudoku is a very simple task. A square table with 9 rows and 9 columns is divided to 9 smaller squares 3x3 as shown on the Figure. In some of the cells are written decimal digits from 1 to 9. The other cells are empty. The goal is to fill the empty cells with decimal digits from 1 to 9, one digit per cell, in such way that in each row, in each column and in each marked 3x3 subsquare, all the digits from 1 to 9 to appear. Write a program to solve a given Sudoku-task.
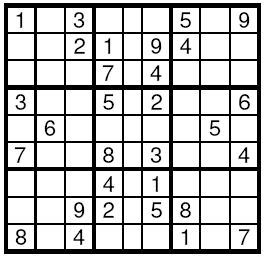
Input
The input data will start with the number of the test cases. For each test case, 9 lines follow, corresponding to the rows of the table. On each line a string of exactly 9 decimal digits is given, corresponding to the cells in this line. If a cell is empty it is represented by 0.
Output
For each test case your program should print the solution in the same format as the input data. The empty cells have to be filled according to the rules. If solutions is not unique, then the program may print any one of them.
Sample Input
1 103000509 002109400 000704000 300502006 060000050 700803004 000401000 009205800 804000107
Sample Output
143628579 572139468 986754231 391542786 468917352 725863914 237481695 619275843 854396127
以下代码用时更短。。。。。
#include <iostream> #include <cstring> #include <cstdio> using namespace std; int map[10][10],count1; bool row[10][10],coloumn[10][10],cr[10][10];//用来标记行,竖,小方格 struct p { int x,y; }point[100];//标记每个格子的横纵坐标 int cxbfine(int i,int j)//规定每个3*3方格的号码 { return ((i-1)/3)*3+(j-1)/3; } int dfs(int n)//进行深搜,符合条件往下,填不下就回溯 { if(n>count1) return 1; for(int i=1;i<=9;i++) if(!row[point[n].x][i] && !coloumn[point[n].y][i] && !cr[cxbfine(point[n].x,point[n].y)][i]) { row[point[n].x][i]=true; coloumn[point[n].y][i]=true; cr[cxbfine(point[n].x,point[n].y)][i]=true; map[point[n].x][point[n].y]=i; if(dfs(n+1)) return 1; row[point[n].x][i]=false; coloumn[point[n].y][i]=false; cr[cxbfine(point[n].x,point[n].y)][i]=false; } return 0; } int main() { int n,i,j; cin>>n; while(n--) { count1=0; memset(row,false,sizeof(row)); memset(coloumn,false,sizeof(coloumn)); memset(cr,false,sizeof(cr)); for(i=1;i<=9;i++)//输入并判断 for(j=1;j<=9;j++) { scanf("%1d",&map[i][j]); if(map[i][j]) { row[i][map[i][j]]=true; coloumn[j][map[i][j]]=true; cr[cxbfine(i,j)][map[i][j]]=true; } else { count1++; point[count1].x=i; point[count1].y=j; } } dfs(1); for(i=1;i<=9;i++) { for(j=1;j<=9;j++) cout<<map[i][j]; cout<<endl; } } return 0; }