<?php
namespace app\api\controller\Test;
use app\api\controller\Common;
use think\facade\Cache;
class Jim extends Common
{
public function getSubjectsByIds()
{
$ids = [1, 2, 22,33,44,3, 4, 5];
$cacheKeyPrefix = $this->getRedisKey('shopProductList');
$cached = [];
$missIds = [];
$cacheKeys = array_map(fn($id) => $cacheKeyPrefix . $id, $ids);
$cacheData = Cache::store('redis')->mget($cacheKeys);
$cacheData = array_combine($cacheKeys, $cacheData);
dump($cacheData);
foreach ($ids as $i => $id) {
$key = $cacheKeys[$i];
$item = $cacheData[$key] ?? null;
if ($item) {
$cached[$id] = is_array($item) ? $item : json_decode($item, true);
} else {
$missIds[] = $id;
}
}
dump($missIds);
if (!empty($missIds)) {
$dbList = [
['id'=>1, 'name'=>'Product 1'],
['id'=>2, 'name'=>'Product 2'],
['id'=>3, 'name'=>'Product 3'],
['id'=>4, 'name'=>'Product 4'],
['id'=>5, 'name'=>'Product 5'],
];
foreach ($dbList as $item) {
$key = $cacheKeyPrefix . $item['id'];
Cache::store('redis')->set($key, json_encode($item), 60);
$cached[$item['id']] = $item;
}
}
$result = [];
foreach ($ids as $id) {
if (isset($cached[$id])) {
$result[] = $cached[$id];
}
}
dd($result);
}
public function getRedisKey(string $prefix, $suffix = ''): string
{
$app = config('app.app_name') ?? 'app';
$env = config('app.env') ?? 'prod';
$key = "{$app}:{$env}:{$prefix}";
if (!empty($suffix)) {
if (is_array($suffix)) {
$suffix = implode("_", $suffix);
}
$key .= ":{$suffix}";
}
return $key;
}
}
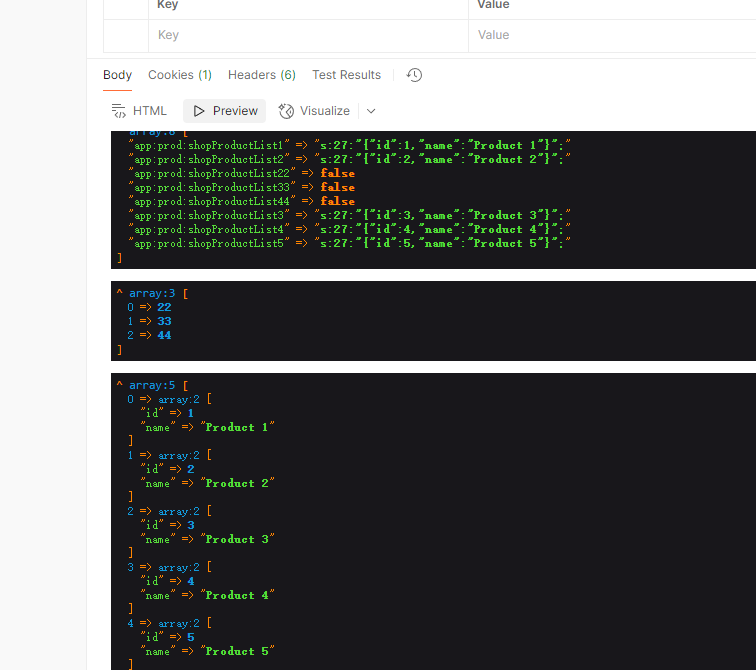