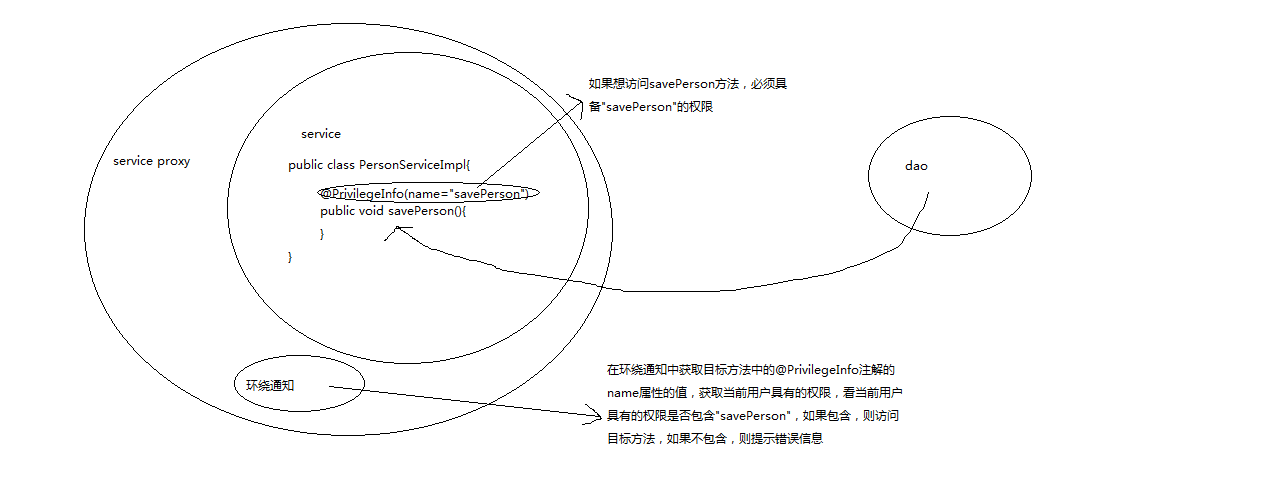
思路:
1、写dao层和service层的类和接口
2、自定义的注解@PrivilegeInfo
3、注解解析器:解析目标方法上面的注解的name属性的值
4、写一个权限类Privilege(name)
5、写一个关于权限的判断的切面,在切面中写一个环绕通知
/**
* dao 接口
* @author w7
*
*/
public interface PersonDao {
public void savePerson();
public void updatePerson();
}
/**
* dao 实现类
* @author w7
*
*/
public class PersonDaoImpl implements PersonDao{
public void savePerson() {
System.out.println("save person");
}
public void updatePerson() {
System.out.println("update person");
}
}
@Target(ElementType.METHOD)
@Retention(RetentionPolicy.RUNTIME)
public @interface PrivilegeInfo {
String name() default "";
}
/**
* 注解的解析
* @author w7
*
*/
public class AnnotationParse {
/**
*
* @param classt 目标类
* @param methodName 目标的方法名子
* @return
* @throws Exception
*/
public static String parse(Class classt,String methodName) throws Exception{
String privilegeName = "";
Method method = classt.getMethod(methodName);
if(method.isAnnotationPresent(PrivilegeInfo.class)){
PrivilegeInfo privilegeInfo = method.getAnnotation(PrivilegeInfo.class);
privilegeName = privilegeInfo.name();
}
return privilegeName;
}
}
/**
* service 接口
* @author w7
*
*/
public interface PersonService {
public void savePerson();
public void updatePerson();
}
/**
* service 实现类
* @author w7
*
*/
public class PersonServiceImpl implements PersonService{
private PersonDao personDao;
public void setPersonDao(PersonDao personDao) {
this.personDao = personDao;
}
/**
* 设置 权限的注解 标记
*/
@PrivilegeInfo(name="savePerson")
public void savePerson() {
this.personDao.savePerson();
}
@PrivilegeInfo(name="updatePerson")
public void updatePerson() {
this.personDao.updatePerson();
}
}
/**
* 封装权限的对象
* @author w7
*
*/
public class Privilege {
private String name;
public String getName() {
return name;
}
public void setName(String name) {
this.name = name;
}
}
/**
* 切面:权限的访问
* @author w7
*
*/
public class PrivilegeAspect {
/**
* 该用户能够访问到的权限集合
*/
private List<Privilege> privileges = new ArrayList<Privilege>();
public List<Privilege> getPrivileges() {
return privileges;
}
public void setPrivileges(List<Privilege> privileges) {
this.privileges = privileges;
}
public void controlTargetMethod(ProceedingJoinPoint joinPoint) throws Throwable{
/**
* 解析注解:
*
* 1、获取用户能够访问到的权限
* 2、获取访问目标方法的权限
* 3、检查用户的权限中是否包含目标方法的权限
* 如果包含
* 则访问目标方法
* 如果不包含
* 则提示不能访问
*/
Class targetClass = joinPoint.getTarget().getClass();
String methodName = joinPoint.getSignature().getName();
String privilegeName = AnnotationParse.parse(targetClass, methodName);
boolean flag = false;
for(Privilege privilege:this.privileges){
if(privilege.getName().equals(privilegeName)){
flag = true;
break;
}
}
if(flag){
joinPoint.proceed();
}else{
System.out.println("权限不足");
}
}
}
<bean id="personDao" class="com.itheima09.springaop.xml.privilege.dao.PersonDaoImpl"></bean>
<bean id="personService" class="com.itheima09.springaop.xml.privilege.service.PersonServiceImpl">
<property name="personDao">
<ref bean="personDao"/>
</property>
</bean>
<bean id="privilegeAspect" class="com.itheima09.springaop.xml.privilege.aspect.PrivilegeAspect">
</bean>
<aop:config>
<aop:pointcut
expression="execution(* com.itheima09.springaop.xml.privilege.service.*.*(..))"
id="perform"/>
<aop:aspect ref="privilegeAspect">
<aop:around method="controlTargetMethod" pointcut-ref="perform"/>
</aop:aspect>
</aop:config>
@Test
public void test(){
ApplicationContext context = new ClassPathXmlApplicationContext("applicationContext.xml");
PrivilegeAspect privilegeAspect = (PrivilegeAspect)context.getBean("privilegeAspect");
/**
* 给用户初始化权限值
*/
Privilege privilege1 = new Privilege();
privilege1.setName("asdf");
Privilege privilege2 = new Privilege();
privilege2.setName("updatePerson");
/**
* 将用户的所有权限添加到权限切面的集合中
*/
privilegeAspect.getPrivileges().add(privilege1);
privilegeAspect.getPrivileges().add(privilege2);
/**
* 执行目标方法
*/
PersonService personService = (PersonService)context.getBean("personService");
personService.savePerson();
}