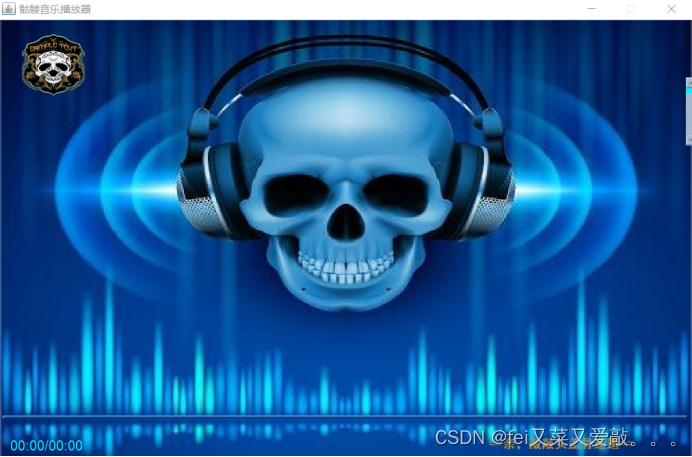
作品名:
骷髅音乐播放器
作品功能与使用说明:
1.播放/暂停音乐:鼠标点击骷髅头盖骨任意区域(播放/暂停按键共用同一个按钮,为了不影响整个音乐背景的风格,我尝试隐藏了播放按钮)。为了能够反馈用户,我添加了一些特色功能:只有当音乐播放时,骷髅眼睛会变色,左右两侧赛博骷髅也将会随之闪动,一些控件也将会展示出来,下面的几点功能才得以使用。而当音乐暂停时,眼睛颜色淡去,其他控件的功能也不允许使用。
2.播放下一首音乐:点击骷髅右侧发光的眼睛
3.播放上一首音乐:点击骷髅左侧发光的眼睛
4.回放当前音乐:点击骷髅中间发光的鼻孔处.
4.音乐列表:在右上角处,音乐正在播放的歌曲名将被高亮标志,当然用户也可以点击音乐列表中的选项进行选择自己喜欢的音乐进行播放。歌曲比较多,只展示全四首歌曲名,其他歌曲用户可下拉右侧滚动条寻.
5.搜索框:用户点击右上角处的放大镜图标即可打开搜索框,输入要搜索的音乐,再点击右侧放大镜图标(或者选择点击回车键),如果音乐库中存在用户要搜索的歌曲,播放器便可以自动播放该歌曲,否则将弹出“暂未找到”提示窗口。
6.时间计时器/音乐总时长,播放器左下侧,会随着音乐播放进行计时(可以随着音乐播放暂停,继续,归零)
7.音乐进度条:会随着音乐播放而增长(暂停,继续,归零)。
8.歌词播放器:能够滚动展示播放中的音乐所对应的歌词,并且只有音乐正在播放的内容被高亮,播放时间误差大约为1~2秒。
9.可在随意位置播放:用户可以点击进度条的任意一位置,即可随意从音乐的某一刻开始播放。
10.自动循环播放、顺序播放、重复播放:点击右下角提示按钮
11.点击左上角logo,可打开歌曲对应的网页资源,不同的歌曲会打开不同的网页让播发器有更多资源,音乐没播放前开始点击时会打开自己做的html网页。
上图为一个简单的html网页,用于介绍骷髅播放器的主题。
播放音乐时的界面:
搜索:(右上角)
未找到歌曲的提示窗口:
点击左上角logo弹出来的网页:
Java实现
工具与材料:
需要播放器所用到的图片、你喜欢的音乐(.wav文件,暂不支持.mp4文件)以及音乐歌词(.txt文件)、IntelliJ IDEA Community Edition 2021.2(或其他版本)。
图片放到image文件夹、音乐以及歌词文件放到music文件夹中。player.java为可执行文件,Audio Playe.java和MyExtendJFrame.java文件为类文件。
代码:
player.java:
/**
* @Auther: Fei
* @Date: 2023/7/2 - 07 - 02 - 21:06
* @Description: MyCoolPlayer
* @version: 1.0
*/
public class player {
public static void main(String[] args) throws IOException {
MyExtendsJFrame my =new MyExtendsJFrame("C:\\Users\\86133\\Downloads\\demo\\MyCoolPlayer\\src\\MyCoolPlayer");
}
}
Audio Playe.java:
package MyCoolPlayer;
import javax.sound.sampled.AudioFileFormat;
import javax.sound.sampled.AudioInputStream;
import javax.sound.sampled.AudioSystem;
import javax.sound.sampled.Clip;
import java.io.File;
import java.net.URL;
import java.util.ArrayList;
public class AudioPlaye {
private ArrayList<File> soundList;
private AudioInputStream audioInput;
private Clip clip;
private URL url;
private int currentSoundIndex;
private long currentFrame;
private boolean isPlaying = false;
private boolean isPaused = false;
public AudioPlaye(ArrayList<File> fileList) {
soundList = fileList;
currentSoundIndex = 0;
try {
audioInput = AudioSystem.getAudioInputStream(soundList.get(currentSoundIndex));
clip = AudioSystem.getClip();
clip.open(audioInput);
} catch (Exception e) {
System.out.println("Error: Unable to open audio file.");
e.printStackTrace();
}
}
public void play() {
try {
if (!isPlaying) {
if (isPaused) {
clip.setMicrosecondPosition(currentFrame);
isPaused = false;
} else {
clip.setFramePosition(0);
}
isPlaying = true;
clip.start();
}
} catch (Exception e) {
System.out.println("Error: Unable to start audio clip.");
e.printStackTrace();
}
}
public int play(int index) {//对play函数进行重写
try {
if (!isPlaying) {
if (isPaused) {
clip.setMicrosecondPosition(currentFrame);
isPaused = false;
} else {
clip.setFramePosition(0);
}
isPlaying = true;
clip.start();
}
} catch (Exception e) {
System.out.println("Error: Unable to start audio clip.");
e.printStackTrace();
}
int totalDuration = 0;
if (index >= 0 && index < soundList.size()) {
try {
currentSoundIndex = index;
audioInput = AudioSystem.getAudioInputStream(soundList.get(currentSoundIndex));
AudioFileFormat fileFormat = AudioSystem.getAudioFileFormat(soundList.get(currentSoundIndex));
totalDuration = (int) (fileFormat.getFrameLength() / fileFormat.getFormat().getFrameRate());
clip.close();
clip = AudioSystem.getClip();
clip.open(audioInput);
clip.start();
} catch (Exception ex) {
ex.printStackTrace();
}
}
return totalDuration;
}
public void jmp(long position){
try {
if (clip != null && clip.isRunning()) {
clip.stop();
clip.setMicrosecondPosition(position);
clip.start();
}
} catch (Exception e) {
System.out.println("Error: Unable to start audio clip.");
e.printStackTrace();
}
}
public void latter(){
try {
if (clip != null && clip.isRunning()) {
long point = clip.getMicrosecondPosition();
clip.stop();
clip.setMicrosecondPosition(point+5000000);
clip.start();
}
} catch (Exception e) {
System.out.println("Error: Unable to start audio clip.");
e.printStackTrace();
}
}
public void former(){
try {
if (clip != null && clip.isRunning()) {
long point = clip.getMicrosecondPosition();
clip.stop();
clip.setMicrosecondPosition(point-5000000);
clip.start();
}
} catch (Exception e) {
System.out.println("Error: Unable to start audio clip.");
e.printStackTrace();
}
}
public void pause() {
try {
if (isPlaying && !isPaused) {
isPaused = true;
currentFrame = clip.getMicrosecondPosition();
clip.stop();
}
} catch (Exception e) {
System.out.println("Error: Unable to pause audio clip.");
e.printStackTrace();
}
}
public void resume() {
try {
if (isPlaying && isPaused) {
isPaused = false;
clip.setMicrosecondPosition(currentFrame);
clip.start();
}
} catch (Exception e) {
System.out.println("Error: Unable to resume audio clip.");
e.printStackTrace();
}
}
public void stop() {
try {
if (isPlaying) {
isPlaying = false;
isPaused = false;
currentFrame = 0;
clip.stop();
clip.setFramePosition(0);
}
} catch (Exception e) {
System.out.println("Error: Unable to stop audio clip.");
e.printStackTrace();
}
}
public int next() {
stop();
int totalDuration = 0;
try {
if (currentSoundIndex < sou