Hibernate对JDBC进行了非常轻量级的对象封装,利用Hibernate框架可以让我们用面向对象的思想来操作数据对象。以下将说明一个简单的学生信息管理系统例子,结合了Java图形界面和hibernate框架。这里将不再说明hibernate的配置,请读者先自行配好,下图是运行效果。
**1.首先是数据持久层,即写好JavaBean类**
Student.java
package com.edu.entity;
public class Student {
private Integer id;
private Integer no;
private String name;
private String sex;
private String dept;
private Integer age;
private String address;
public Student(){}
public Integer getId() {
return id;
}
public void setId(Integer id) {
this.id = id;
}
public Integer getNo() {
return no;
}
public void setNo(Integer no) {
this.no = no;
}
public String getName() {
return name;
}
public void setName(String name) {
this.name = name;
}
public String getSex() {
return sex;
}
public void setSex(String sex) {
this.sex = sex;
}
public String getDept() {
return dept;
}
public void setDept(String dept) {
this.dept = dept;
}
public Integer getAge() {
return age;
}
public void setAge(Integer age) {
this.age = age;
}
public String getAddress() {
return address;
}
public void setAddress(String address) {
this.address = address;
}
}
**2.利用hiberante的eclipse插件可以很方便生成对象文件,右击Student类,new->others->hibernate,选择相关选项,如下图所示**
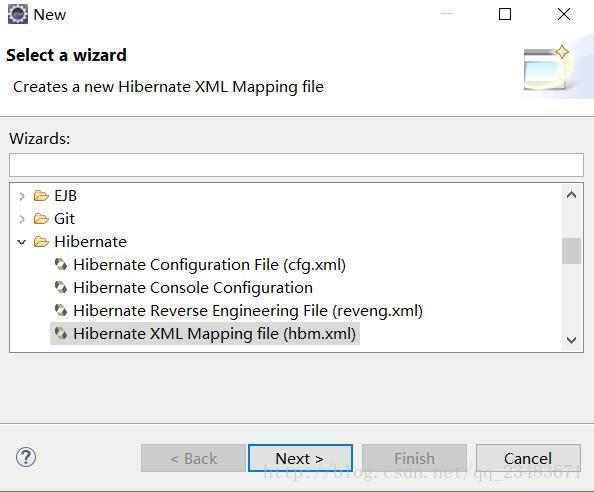
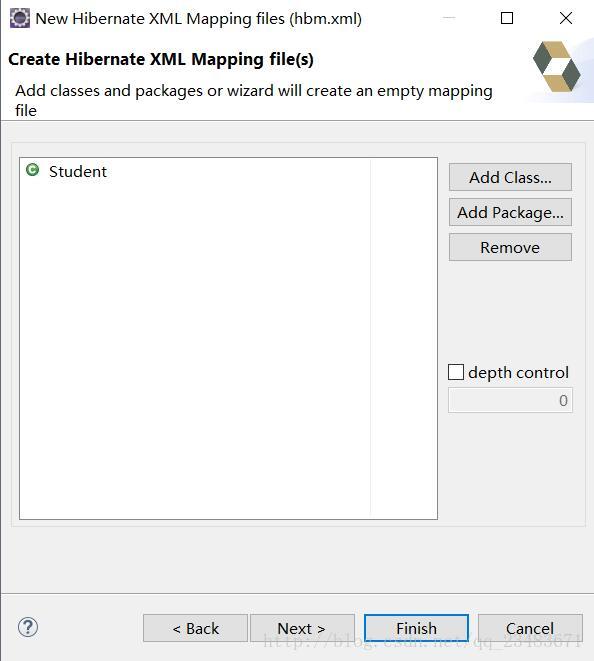
按Finish按钮即可生成 Student.hbm.xml
<?xml version="1.0"?>
<!DOCTYPE hibernate-mapping PUBLIC "-//Hibernate/Hibernate Mapping DTD 3.0//EN"
"http://hibernate.sourceforge.net/hibernate-mapping-3.0.dtd">
<hibernate-mapping>
<class name="com.edu.entity.Student" table="STUDENT">
<id name="id" type="java.lang.Integer">
<column name="ID" />
<generator class="native" />
</id>
<property name="no" type="java.lang.Integer">
<column name="NO" />
</property>
<property name="name" type="java.lang.String">
<column name="NAME" />
</property>
<property name="sex" type="java.lang.String">
<column name="SEX" />
</property>
<property name="dept" type="java.lang.String">
<column name="DEPT" />
</property>
<property name="age" type="java.lang.Integer">
<column name="AGE" />
</property>
<property name="address" type="java.lang.String">
<column name="ADDRESS" />
</property>
</class>
</hibernate-mapping>
**3.配置Hibernate.cfg.xml**
<?xml version="1.0" encoding="UTF-8"?>
<!DOCTYPE hibernate-configuration PUBLIC
"-//Hibernate/Hibernate Configuration DTD 3.0//EN"
"http://hibernate.sourceforge.net/hibernate-configuration-3.0.dtd">
<hibernate-configuration>
<session-factory>
<property name="connection.driver_class">com.mysql.jdbc.Driver</property>
<property name="connection.url">jdbc:mysql://localhost:3306/homework?useUnicode=true&characterEncoding=utf-8</property>
<property name="connection.username">root</property>
<property name="connection.password">root</property>
<property name="dialect">org.hibernate.dialect.MySQL5InnoDBDialect</property>
<property name="hbm2ddl.auto">update</property>
<property name="show_sql">true</property>
<property name="format_sql">true</property>
<mapping resource="com/edu/entity/Student.hbm.xml"/>
</session-factory>
</hibernate-configuration>
**4.编写Hibernate工具类HibernateUtils.java**
package com.edu.entity;
import org.hibernate.Session;
import org.hibernate.SessionFactory;
import org.hibernate.boot.registry.StandardServiceRegistryBuilder;
import org.hibernate.cfg.Configuration;
import org.hibernate.service.ServiceRegistry;
public class HibernateUtils {
private static SessionFactory factory;
private static ThreadLocal threadLocal=new ThreadLocal();
static {
try {
Configuration cfg = new Configuration().configure();
ServiceRegistry serviceRegistry = new StandardServiceRegistryBuilder()
.applySettings(cfg.getProperties()).build();
factory = cfg.buildSessionFactory(serviceRegistry);
}catch(Exception e) {
e.printStackTrace();
}
}
public HibernateUtils() {
}
public static Session getSession() {
Session session=(Session)threadLocal.get();
if(session==null){
session=factory.openSession();
threadLocal.set(session);
}
return session;
}
public static void closeSession() {
Session s=(Session)threadLocal.get();
if(s!=null){
s.close();
threadLocal.set(null);
}
}
public static SessionFactory getSessionFactory() {
return factory;
}
}
**5.最后,编写主类ImageMain.java.这里完成各种操作,包括图形界面的实现**
package com.edu.entity;
import javax.swing.*;
import javax.swing.border.TitledBorder;
import javax.swing.event.ChangeEvent;
import javax.swing.table.DefaultTableModel;
import java.awt.*;
import java.util.List;
import java.awt.event.*;
import java.util.*;
public class ImageMain extends JFrame implements ActionListener{
private LogPane log;
JSplitPane vsplitPane;
static JPanel controlPane;
static JTabbedPane tabPane;
static CardLayout card;
public JPanel selectPane;
private JPanel updatePane;
private JPanel deletePane;
private JPanel insertPane;
Font font=new Font("微软雅黑",Font.PLAIN,16);
private Vector<Vector> vector;
private JTable table;
private JScrollPane jsp,jsp1,jsp2,jsp3;
private DefaultTableModel tableModel,tableModel1;
private Vector columnsName;
private JButton queryButton,updateButton,deleteButton,insertButton,newButton,delButton;
String[] columns = {"序号","学号","姓名","性别","年龄","专业","地址"};
public ImageMain(){
super("Author@夜空--学生信息管理系统");
try {
UIManager
.setLookAndFeel("com.sun.java.swing.plaf.windows.WindowsLookAndFeel");
} catch (Exception e) {
e.printStackTrace();
}
try {
init();
} catch (Exception e) {
log.addLog(e.toString());
}
}
private void setBorder(TitledBorder titledBorder) {
}
public void init(){
initTable();
initUI();
initListener();
setSize(800,650);
setLocationRelativeTo(null);
setBackground(Color.LIGHT_GRAY);
setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
setVisible(true);
setResizable(false);
}
public void initTable(){
columnsName=new Vector();
for(int i=0;i<columns.length;i++){
columnsName.add(columns[i]);
}
vector=new Vector<Vector>();
tableModel=new DefaultTableModel(vector,columnsName);
table = new JTable(tableModel);
table.setRowHeight(30);
}
public void initUI(){
queryButton=new JButton("查询");
newButton=new JButton("新增");
insertButton=new JButton("插入");
updateButton=new JButton("更改");
deleteButton=new JButton("删除");
queryButton.addActionListener(this);
newButton.addActionListener(this);
insertButton.addActionListener(this);
updateButton.addActionListener(this);
deleteButton.addActionListener(this);
JPanel pane=new JPanel();
pane.add(new JLabel("学生信息管理系统"));
pane.setLayout(new BorderLayout());
selectPane=new JPanel(new BorderLayout());
deletePane=new JPanel();
updatePane=new JPanel();
insertPane=new JPanel();
jsp = new JScrollPane(table);
JPanel buttonPane=new JPanel(new GridLayout(1,5));
buttonPane.add(queryButton);
buttonPane.add(newButton);
buttonPane.add(insertButton);
buttonPane.add(updateButton);
buttonPane.add(deleteButton);
selectPane.add(buttonPane,BorderLayout.NORTH);
selectPane.add(jsp,BorderLayout.CENTER);
controlPane=new JPanel();
controlPane.setLayout(new BorderLayout());
controlPane.add(pane, BorderLayout.NORTH);
tabPane=new JTabbedPane(JTabbedPane.TOP);
tabPane.add("查询",selectPane);
tabPane.add("插入",insertPane);
tabPane.add("更新",updatePane);
tabPane.add("删除",deletePane);
controlPane.add(tabPane,BorderLayout.CENTER);
log=LogPane.getLog();
log.addLog("欢迎来到学生信息管理系统!",Color.blue);
vsplitPane=new JSplitPane(JSplitPane.VERTICAL_SPLIT);
vsplitPane.setTopComponent(new JScrollPane(controlPane));
vsplitPane.setBottomComponent(log);
vsplitPane.setDividerLocation(400);
vsplitPane.setEnabled(false);
add(vsplitPane);
}
public void initListener(){
tabPane.addChangeListener(new javax.swing.event.ChangeListener() {
@Override
public void stateChanged(ChangeEvent e) {
JTabbedPane tabbedPane = (JTabbedPane)e.getSource();
int selectedIndex = tabbedPane.getSelectedIndex();
switch(selectedIndex){
case 0:
log.addLog("进入查询记录面板");
break;
case 1:
log.addLog("进入插入记录面板");
break;
case 2:
log.addLog("进入更新记录面板");
break;
case 3:
log.addLog("进入删除记录面板");
break;
}
}
});
}
@Override
public void actionPerformed(ActionEvent e) {
if(e.getSource()==queryButton){
List<Student> list=DataOperate.queryStudent();
vector = JTableUtils.query(list);
tableModel = (DefaultTableModel)table.getModel();
tableModel.setDataVector(vector, columnsName);
table.setModel(tableModel);
table.validate();
table.repaint();
}else if(e.getSource()==newButton){
tableModel.addRow(new Vector());
}else if(e.getSource()==insertButton){
int row=table.getSelectedRow();
Integer no=Integer.parseInt(table.getValueAt(row, 1).toString());
String name=(String)table.getValueAt(row, 2);
String sex=(String)table.getValueAt(row, 3);
Integer age=Integer.parseInt(table.getValueAt(row, 4).toString());
String major=(String)table.getValueAt(row, 5);
String address=(String)table.getValueAt(row, 6);
Student student=new Student();
student.setNo(no);
student.setName(name);
student.setSex(sex);
student.setAge(age);
student.setDept(major);
student.setAddress(address);
int result=DataOperate.insertStudent(student);
if(result>0){
log.addLog("插入数据成功!",Color.BLACK);
cutRow();
}
}else if(e.getSource()==updateButton){
System.out.println("sdfs");
int row=table.getSelectedRow();
System.out.println(row);
if(row>=0){
Integer id=Integer.parseInt(table.getValueAt(row, 0).toString());
Integer no=Integer.parseInt(table.getValueAt(row, 1).toString());
String name=(String)table.getValueAt(row, 2);
String sex=(String)table.getValueAt(row, 3);
Integer age=Integer.parseInt(table.getValueAt(row, 4).toString());
String major=(String)table.getValueAt(row, 5);
String address=(String)table.getValueAt(row, 6);
Student student=new Student();
student.setId(id);
student.setNo(no);
student.setName(name);
student.setSex(sex);
student.setAge(age);
student.setDept(major);
student.setAddress(address);
int result=DataOperate.updateStudent(student);
if(result>0){
log.addLog("更新数据成功!",Color.BLACK);
}
}
}else if(e.getSource()==deleteButton){
int row=table.getSelectedRow();
if(row>=0){
Integer id=Integer.parseInt(table.getValueAt(row, 0).toString());
Integer no=Integer.parseInt(table.getValueAt(row, 1).toString());
String name=(String)table.getValueAt(row, 2);
String sex=(String)table.getValueAt(row, 3);
Integer age=Integer.parseInt(table.getValueAt(row, 4).toString());
String major=(String)table.getValueAt(row, 5);
String address=(String)table.getValueAt(row, 6);
Student student=new Student();
student.setId(id);
student.setNo(no);
student.setName(name);
student.setSex(sex);
student.setAge(age);
student.setDept(major);
student.setAddress(address);
int result=DataOperate.deleteStudent(student);
if(result>0){
log.addLog("删除数据成功!",Color.BLACK);
}
}
}
}
public void cutRow(){
int rowCount=tableModel.getRowCount()-1;
if(rowCount>=0){
tableModel.removeRow(rowCount);
tableModel.setRowCount(rowCount);
}
}
public static void main(String[] args) {
new ImageMain();
}
}
**另外,在程序中结合了一个专门打印操作信息的面板LogPane,这里将其封装成一个类,方便操作 **
package com.edu.entity;
import java.awt.*;
import javax.swing.*;
import java.awt.event.*;
import java.util.Date;
public class LogPane extends JTabbedPane implements ActionListener{
private JScrollPane scroll;
private JTextArea textArea;
static final LogPane log=new LogPane();
public LogPane() {
try {
UIManager
.setLookAndFeel("com.sun.java.swing.plaf.windows.WindowsLookAndFeel");
} catch (Exception e) {
e.printStackTrace();
}
textArea=new JTextArea();
textArea.setLineWrap(true);
textArea.setFont(new Font("TimesRoman",Font.PLAIN,18));
scroll=new JScrollPane(textArea);
add(scroll,BorderLayout.CENTER);
this.setTitleAt(0, "Log");
this.setFont(new Font("微软雅黑",Font.BOLD,16));
this.setEnabled(false);
textArea.setEditable(false);
}
@Override
public void actionPerformed(ActionEvent arg0) {
}
public void addLog(String log){
Date current = new Date(System.currentTimeMillis());
textArea.append(log+"\t\t"+current+"\n");
}
public static LogPane getLog(){
return log;
}
public void addLog(String str,Color color){
Date date=new Date();
textArea.setForeground(color);
textArea.append(str+"\t\t"+date+"\n");
}
}
**谢谢浏览 **