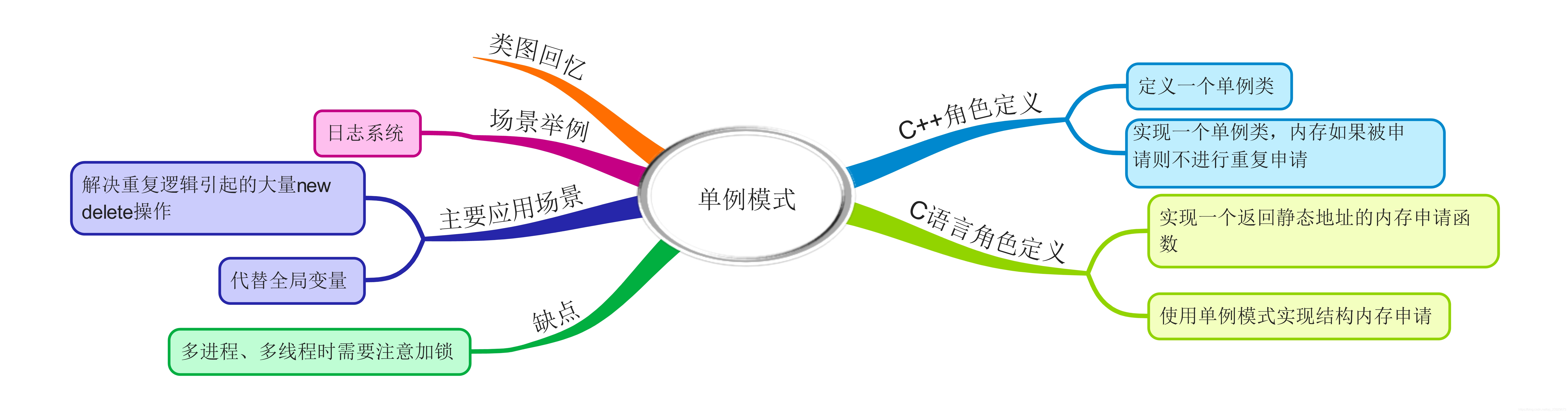
1、场景举例
日志系统
2、主要应用场景
1、解决重复操作引起的大量new delete问题
2、代替全局变量
3、类图
4、用C语言实现原型模式
角色定义
1、实现一个返回静态地址的内存申请函数
案例描述
使用单例模式实现申请具体结构内存,下次调用申请函数时,如果内存已被申请,则直接返回地址
案例实现
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
typedef struct DATA{
char data[12];
}Data;
struct DATA* get_data()
{
static Data* data = NULL;
if(NULL != data){
printf("I have being...\n");
return data;
}
data = (DATA*)malloc(sizeof(Data));
if (data == NULL){
return NULL;
}
printf("new malloc\n");
return data;
}
int main()
{
struct DATA* data = NULL;
data = get_data();
data = get_data();
data = get_data();
if (data != NULL) {
free(data);
}
return 0;
}
5、使用C++实现单例模式
角色定义
1、定义一个单例类
注意私有变量函数指针为静态,且需要初始化。
案例描述
实现一个单例类,内存如果被申请则不进行重复申请
函数实现
#include <iostream>
using namespace std;
class Singleton {
public:
static Singleton* GetInstance()
{
if (m_instance == NULL) {
m_instance = new Singleton();
}
return m_instance;
}
static void DestoryInstance()
{
if (m_instance == NULL)
{
delete m_instance;
m_instance = NULL;
}
}
int get_value()
{
return m_test;
}
private:
Singleton() { m_test = 10; };
static Singleton* m_instance;
int m_test;
};
Singleton* Singleton::m_instance = NULL;
int main()
{
Singleton* singleton = Singleton::GetInstance();
cout << singleton->get_value() << endl;
Singleton::DestoryInstance();
return 0;
}
6、缺点
1、多进程、多线程场景,需要注意加锁