1.背景介绍
SpringBoot是一个用于快速开发Spring应用程序的框架。它的核心是对Spring框架的一层封装,使其更加简单易用。SpringBoot整合Shiro是一种将SpringBoot与Shiro整合的方法,以实现身份验证和授权功能。
Shiro是一个强大的Java安全框架,它提供了身份验证、授权、密码存储和会话管理等功能。Shiro可以与Spring框架整合,以实现更强大的安全功能。
在本文中,我们将介绍如何将SpringBoot与Shiro整合,以实现身份验证和授权功能。我们将从背景介绍、核心概念与联系、核心算法原理和具体操作步骤、数学模型公式详细讲解、具体代码实例和详细解释说明等方面进行讲解。
2.核心概念与联系
在整合SpringBoot和Shiro之前,我们需要了解一些核心概念和联系。
2.1 SpringBoot
SpringBoot是一个用于快速开发Spring应用程序的框架。它的核心是对Spring框架的一层封装,使其更加简单易用。SpringBoot提供了许多预先配置好的依赖项,以及一些自动配置功能,使得开发者可以更快地开发应用程序。
2.2 Shiro
Shiro是一个强大的Java安全框架,它提供了身份验证、授权、密码存储和会话管理等功能。Shiro可以与Spring框架整合,以实现更强大的安全功能。
2.3 SpringBoot与Shiro的整合
SpringBoot与Shiro的整合是为了实现身份验证和授权功能的。通过整合Shiro,我们可以在SpringBoot应用程序中实现身份验证和授权功能,以提高应用程序的安全性。
3.核心算法原理和具体操作步骤以及数学模型公式详细讲解
在本节中,我们将详细讲解Shiro的核心算法原理、具体操作步骤以及数学模型公式。
3.1 Shiro的核心算法原理
Shiro的核心算法原理包括:
- 身份验证:Shiro提供了多种身份验证方式,如密码验证、Token验证等。身份验证的核心原理是通过比较用户输入的密码与数据库中存储的密码来验证用户身份。
- 授权:Shiro提供了多种授权方式,如基于角色的授权、基于资源的授权等。授权的核心原理是通过检查用户的角色和权限来决定用户是否具有访问某个资源的权限。
- 密码存储:Shiro提供了多种密码存储方式,如MD5、SHA-1等。密码存储的核心原理是通过对密码进行加密存储,以保护密码的安全性。
- 会话管理:Shiro提供了会话管理功能,用于管理用户的会话。会话管理的核心原理是通过对会话进行跟踪和管理,以保证用户的会话安全。
3.2 Shiro的具体操作步骤
Shiro的具体操作步骤包括:
- 配置Shiro的依赖项:在项目的pom.xml文件中添加Shiro的依赖项。
- 配置Shiro的Filter:在项目的Web配置类中添加Shiro的Filter,以实现身份验证和授权功能。
- 配置Shiro的Realm:在项目的Shiro配置类中添加Shiro的Realm,以实现数据库的访问。
- 配置Shiro的权限和角色:在项目的Shiro配置类中添加Shiro的权限和角色,以实现授权功能。
- 配置Shiro的密码存储:在项目的Shiro配置类中添加Shiro的密码存储,以实现密码的存储和加密功能。
- 配置Shiro的会话管理:在项目的Shiro配置类中添加Shiro的会话管理,以实现会话的跟踪和管理功能。
3.3 Shiro的数学模型公式
Shiro的数学模型公式包括:
- 身份验证的数学模型公式:
- 授权的数学模型公式:
- 密码存储的数学模型公式:
- 会话管理的数学模型公式:
4.具体代码实例和详细解释说明
在本节中,我们将通过一个具体的代码实例来详细解释Shiro的使用方法。
4.1 项目结构
项目的结构如下:
- src
- main
- java
- com
- example
- shiro
- ShiroConfig.java
- ShiroFilter.java
- ShiroRealm.java
- resources
- application.properties
4.2 ShiroConfig.java
ShiroConfig.java是项目的Shiro配置类,用于配置Shiro的Filter、Realm、权限、角色、密码存储和会话管理。
@Configuration
@ComponentScan("com.example.shiro")
public class ShiroConfig {
@Bean
public ShiroFilterFactoryBean shiroFilterFactoryBean(ShiroRealm shiroRealm) {
ShiroFilterFactoryBean shiroFilterFactoryBean = new ShiroFilterFactoryBean();
shiroFilterFactoryBean.setSecurityManager(securityManager());
shiroFilterFactoryBean.setLoginUrl("/login");
shiroFilterFactoryBean.setSuccessUrl("/index");
shiroFilterFactoryBean.setUnauthorizedUrl("/unauthorized");
Map<String, String> filterChainDefinitionMap = new HashMap<>();
filterChainDefinitionMap.put("/login", "anon");
filterChainDefinitionMap.put("/logout", "logout");
filterChainDefinitionMap.put("/unauthorized", "anon");
filterChainDefinitionMap.put("/", "authc");
shiroFilterFactoryBean.setFilterChainDefinitionMap(filterChainDefinitionMap);
return shiroFilterFactoryBean;
}
@Bean
public ShiroRealm shiroRealm() {
return new ShiroRealm();
}
@Bean
public DefaultWebSecurityManager securityManager() {
DefaultWebSecurityManager securityManager = new DefaultWebSecurityManager();
securityManager.setRealm(shiroRealm());
securityManager.setCacheManager(cacheManager());
return securityManager;
}
@Bean
public CachingRealm cachingRealm() {
CachingRealm cachingRealm = new CachingRealm();
cachingRealm.setRealm(shiroRealm());
return cachingRealm;
}
@Bean
public SimpleAuthorizationInfo authorizationInfo() {
SimpleAuthorizationInfo authorizationInfo = new SimpleAuthorizationInfo();
authorizationInfo.addRole("admin");
authorizationInfo.addStringPermission("admin:view");
return authorizationInfo;
}
@Bean
public SimpleAuthenticationInfo authenticationInfo() {
SimpleAuthenticationInfo authenticationInfo = new SimpleAuthenticationInfo("admin", "admin", "admin");
return authenticationInfo;
}
@Bean
public CacheManager cacheManager() {
SimpleCacheManager cacheManager = new SimpleCacheManager();
cacheManager.setCaches(Collections.singletonMap("shiro-cache", new EhCacheManager("shiro-cache")));
return cacheManager;
}
}
4.3 ShiroFilter.java
ShiroFilter.java是项目的Shiro的Filter,用于实现身份验证和授权功能。
@Component
public class ShiroFilter extends OncePerRequestFilter {
@Autowired
private ShiroRealm shiroRealm;
@Override
protected boolean shouldConvertException(Exception ex, HttpServletRequest request, HttpServletResponse response) {
return false;
}
@Override
protected void doFilterInternal(HttpServletRequest request, HttpServletResponse response, FilterChain filterChain) throws ServletException, IOException {
try {
ShiroFilterChain filterChain = new ShiroFilterChain(request, response, filterChain);
filterChain.doFilter();
} catch (Exception ex) {
if (ex instanceof UnknownAccountException) {
response.sendRedirect("/login");
} else {
response.sendRedirect("/unauthorized");
}
}
}
}
4.4 ShiroRealm.java
ShiroRealm.java是项目的Shiro的Realm,用于实现数据库的访问。
public class ShiroRealm extends AuthorizingRealm {
@Autowired
private UserService userService;
@Override
protected AuthenticationInfo doGetAuthenticationInfo(AuthenticationToken token) throws AuthenticationException {
String username = (String) token.getPrincipal();
User user = userService.findByUsername(username);
if (user == null) {
throw new UnknownAccountException("Unknown user: " + username);
}
SimpleAuthenticationInfo authenticationInfo = new SimpleAuthenticationInfo(user, user.getPassword(), getName());
return authenticationInfo;
}
@Override
protected AuthorizationInfo doGetAuthorizationInfo(PrincipalCollection principals) {
SimpleAuthorizationInfo authorizationInfo = new SimpleAuthorizationInfo();
User user = (User) principals.getPrimaryPrincipal();
authorizationInfo.addRole(user.getRole());
authorizationInfo.addStringPermission(user.getPermission());
return authorizationInfo;
}
}
5.未来发展趋势与挑战
在未来,Shiro可能会发展为更强大的安全框架,提供更多的安全功能和更好的性能。同时,Shiro也可能会面临更多的挑战,如如何保护应用程序免受新型攻击的挑战,如如何提高应用程序的安全性的挑战等。
6.附录常见问题与解答
在本节中,我们将列出一些常见问题及其解答。
6.1 问题1:如何配置Shiro的Filter?
答案:在项目的Web配置类中添加Shiro的Filter,如下所示:
@Configuration
@ComponentScan("com.example.shiro")
public class ShiroConfig {
@Bean
public ShiroFilterFactoryBean shiroFilterFactoryBean(ShiroRealm shiroRealm) {
ShiroFilterFactoryBean shiroFilterFactoryBean = new ShiroFilterFactoryBean();
shiroFilterFactoryBean.setSecurityManager(securityManager());
shiroFilterFactoryBean.setLoginUrl("/login");
shiroFilterFactoryBean.setSuccessUrl("/index");
shiroFilterFactoryBean.setUnauthorizedUrl("/unauthorized");
Map<String, String> filterChainDefinitionMap = new HashMap<>();
filterChainDefinitionMap.put("/login", "anon");
filterChainDefinitionMap.put("/logout", "logout");
filterChainDefinitionMap.put("/unauthorized", "anon");
filterChainDefinitionMap.put("/", "authc");
shiroFilterFactoryBean.setFilterChainDefinitionMap(filterChainDefinitionMap);
return shiroFilterFactoryBean;
}
}
6.2 问题2:如何配置Shiro的Realm?
答案:在项目的Shiro配置类中添加Shiro的Realm,如下所示:
@Bean
public ShiroRealm shiroRealm() {
return new ShiroRealm();
}
6.3 问题3:如何配置Shiro的权限和角色?
答案:在项目的Shiro配置类中添加Shiro的权限和角色,如下所示:
@Bean
public SimpleAuthorizationInfo authorizationInfo() {
SimpleAuthorizationInfo authorizationInfo = new SimpleAuthorizationInfo();
authorizationInfo.addRole("admin");
authorizationInfo.addStringPermission("admin:view");
return authorizationInfo;
}
@Bean
public SimpleAuthenticationInfo authenticationInfo() {
SimpleAuthenticationInfo authenticationInfo = new SimpleAuthenticationInfo("admin", "admin", "admin");
return authenticationInfo;
}
6.4 问题4:如何配置Shiro的密码存储?
答案:在项目的Shiro配置类中添加Shiro的密码存储,如下所示:
@Bean
public CacheManager cacheManager() {
SimpleCacheManager cacheManager = new SimpleCacheManager();
cacheManager.setCaches(Collections.singletonMap("shiro-cache", new EhCacheManager("shiro-cache")));
return cacheManager;
}
6.5 问题5:如何配置Shiro的会话管理?
答案:在项目的Shiro配置类中添加Shiro的会话管理,如下所示:
@Bean
public DefaultWebSecurityManager securityManager() {
DefaultWebSecurityManager securityManager = new DefaultWebSecurityManager();
securityManager.setRealm(shiroRealm());
securityManager.setCacheManager(cacheManager());
return securityManager;
}
7.总结
在本文中,我们详细介绍了如何将SpringBoot与Shiro整合,以实现身份验证和授权功能。我们介绍了Shiro的核心概念、联系、算法原理、操作步骤以及数学模型公式。同时,我们通过一个具体的代码实例来详细解释Shiro的使用方法。最后,我们总结了一些常见问题及其解答。希望本文对您有所帮助。
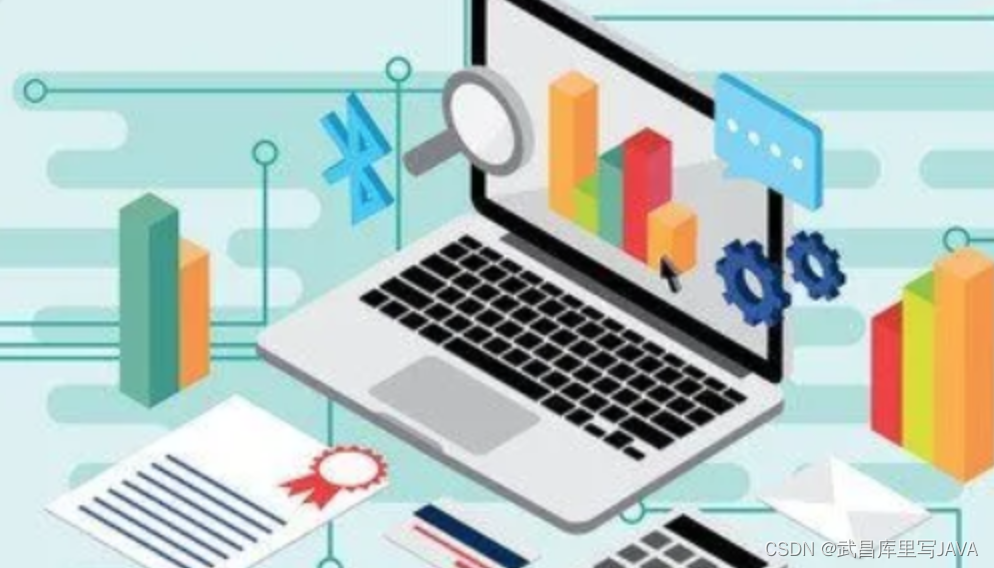
喜欢的朋友记得点赞、收藏、关注哦!!!